Go参考手册
文本 | text
text/tabwriter
import "text/tabwriter"
- 概观
- 索引
- 示例
概观
Package tabwriter实现了一个写入过滤器(tabwriter.Writer),它将输入中的选项卡式列转换为正确对齐的文本。
该软件包使用http://nickgravgaard.com/elastictabstops/index.html中描述的弹性 Tabstops 算法。
文本/制表程序包被冻结,并且不接受新功能。
示例(弹性)
package main
import (
"fmt"
"os"
"text/tabwriter"
)
func main() {
// Observe how the b's and the d's, despite appearing in the
// second cell of each line, belong to different columns.
w := tabwriter.NewWriter(os.Stdout, 0, 0, 1, '.', tabwriter.AlignRight|tabwriter.Debug)
fmt.Fprintln(w, "a\tb\tc")
fmt.Fprintln(w, "aa\tbb\tcc")
fmt.Fprintln(w, "aaa\t") // trailing tab
fmt.Fprintln(w, "aaaa\tdddd\teeee")
w.Flush()
}
示例(TrailingTab)
package main
import (
"fmt"
"os"
"text/tabwriter"
)
func main() {
// Observe that the third line has no trailing tab,
// so its final cell is not part of an aligned column.
const padding = 3
w := tabwriter.NewWriter(os.Stdout, 0, 0, padding, '-', tabwriter.AlignRight|tabwriter.Debug)
fmt.Fprintln(w, "a\tb\taligned\t")
fmt.Fprintln(w, "aa\tbb\taligned\t")
fmt.Fprintln(w, "aaa\tbbb\tunaligned") // no trailing tab
fmt.Fprintln(w, "aaaa\tbbbb\taligned\t")
w.Flush()
}
索引
- 常量
- 键入Writer
- func NewWriter(output io.Writer, minwidth, tabwidth, padding int, padchar byte, flags uint) *Writer
- func (b *Writer) Flush() error
- func (b *Writer) Init(output io.Writer, minwidth, tabwidth, padding int, padchar byte, flags uint) *Writer
- func (b *Writer) Write(buf []byte) (n int, err error)
示例
Writer.Init Package (Elastic) Package (TrailingTab)
打包文件
tabwriter.go
常量
格式化可以用这些标志进行控制。
const (
// Ignore html tags and treat entities (starting with '&'
// and ending in ';') as single characters (width = 1).
FilterHTML uint = 1 << iota
// Strip Escape characters bracketing escaped text segments
// instead of passing them through unchanged with the text.
StripEscape
// Force right-alignment of cell content.
// Default is left-alignment.
AlignRight
// Handle empty columns as if they were not present in
// the input in the first place.
DiscardEmptyColumns
// Always use tabs for indentation columns (i.e., padding of
// leading empty cells on the left) independent of padchar.
TabIndent
// Print a vertical bar ('|') between columns (after formatting).
// Discarded columns appear as zero-width columns ("||").
Debug
)
要转义文本段,请使用转义字符进行包围。例如,此字符串中的选项卡“忽略此选项卡: \xff\t\xff ”不会终止单元格,并且为了格式化目的而构成宽度为1的单个字符。
值 0xff 被选中是因为它不能以有效的 UTF-8 序列出现。
const Escape = '\xff'
键入WriterSource
Writer是一个过滤器,用于在其输入中的制表符分隔列中插入填充以将其与输出对齐。
Writer将传入的字节视为由水平 ('\t') 或垂直 ('\v') 制表符以及换行符 ('\n') 或换页符 ('\f') 组成的单元组成的 UTF-8 编码文本。字符; 换行符和换页符都是换行符。
连续行中制表符终止的单元格构成列。 Writer 根据需要插入填充以使列中的所有单元格具有相同的宽度,从而有效地对齐列。它假定所有字符都具有相同的宽度,但必须指定 tabwidth 的制表符除外。列单元格必须是制表符终止的,而不是制表符分隔的:行结尾处的非制表符终止的结尾文本形成单元格,但该单元格不是对齐列的一部分。例如,在本例中(其中|代表水平制表符):
aaaa|bbb|d
aa |b |dd
a |
aa |cccc|eee
b 和 c 在不同的列中(b 列在所有方面都不是连续的)。d 和 e 根本不在列中(没有终止标签,列也不是连续的)。
Writer 假定所有的 Unicode 代码点具有相同的宽度;在某些字体中或者字符串包含组合字符时,这可能不是真的。
如果设置了 DiscardEmptyColumns ,则会放弃完全由垂直(或“软”)选项卡终止的空列。水平(或“硬”)选项卡终止的列不受此标志的影响。
如果 Writer 配置为过滤 HTML ,则会传递 HTML 标记和实体。为了格式化目的,标签和实体的宽度被假定为零(标签)和一个(实体)。
一段文字可以通过用 Escape 字符括起来进行转义。制表人通过不变的方式传递转义文本段。特别是,它不解释段中的任何制表符或换行符。如果设置了 StripEscape 标志,则 Escape 字符将从输出中剥离; 否则它们也会通过。为了格式化,转义字符的宽度始终计算,不包括转义字符。
换页字符就像换行符,但它也会终止当前行中的所有列(有效地调用 Flush )。下一行中以制表符结尾的单元格开始新列。除非在 HTML 标签内或转义文本段内发现,否则换页字符在输出中显示为换行符。
写入器必须在内部缓冲输入,因为一条线的适当间距可能取决于未来线中的单元。完成调用写入后,客户端必须调用 Flush 。
type Writer struct {
// contains filtered or unexported fields
}
func NewWriterSource
func NewWriter(output io.Writer, minwidth, tabwidth, padding int, padchar byte, flags uint) *Writer
NewWriter 分配并初始化一个新的 Tabwriter.Writer 。参数与 Init 函数相同。
func (*Writer) FlushSource
func (b *Writer) Flush() error
在上次调用 Write 之后应该调用 Flush ,以确保写入器中缓冲的任何数据都写入输出。出于格式化目的,任何不完整的转义序列都视为完成。
func (*Writer) InitSource
func (b *Writer) Init(output io.Writer, minwidth, tabwidth, padding int, padchar byte, flags uint) *Writer
写入器必须通过对 Init 的调用进行初始化。第一个参数(输出)指定了过滤器输出。其余参数控制格式:
minwidth minimal cell width including any padding
tabwidth width of tab characters (equivalent number of spaces)
padding padding added to a cell before computing its width
padchar ASCII char used for padding
if padchar == '\t', the Writer will assume that the
width of a '\t' in the formatted output is tabwidth,
and cells are left-aligned independent of align_left
(for correct-looking results, tabwidth must correspond
to the tab width in the viewer displaying the result)
flags formatting control
示例
package main
import (
"fmt"
"os"
"text/tabwriter"
)
func main() {
w := new(tabwriter.Writer)
// Format in tab-separated columns with a tab stop of 8.
w.Init(os.Stdout, 0, 8, 0, '\t', 0)
fmt.Fprintln(w, "a\tb\tc\td\t.")
fmt.Fprintln(w, "123\t12345\t1234567\t123456789\t.")
fmt.Fprintln(w)
w.Flush()
// Format right-aligned in space-separated columns of minimal width 5
// and at least one blank of padding (so wider column entries do not
// touch each other).
w.Init(os.Stdout, 5, 0, 1, ' ', tabwriter.AlignRight)
fmt.Fprintln(w, "a\tb\tc\td\t.")
fmt.Fprintln(w, "123\t12345\t1234567\t123456789\t.")
fmt.Fprintln(w)
w.Flush()
}
func (*Writer) WriteSource
func (b *Writer) Write(buf []byte) (n int, err error)
写入器写 buf 给写入器 b 。返回的唯一错误是在写入底层输出流时遇到的错误。
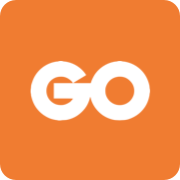
Go 是一种编译型语言,它结合了解释型语言的游刃有余,动态类型语言的开发效率,以及静态类型的安全性。它也打算成为现代的,支持网络与多核计算的语言。要满足这些目标,需要解决一些语言上的问题:一个富有表达能力但轻量级的类型系统,并发与垃圾回收机制,严格的依赖规范等等。这些无法通过库或工具解决好,因此Go也就应运而生了。
主页 | https://golang.org/ |
源码 | https://go.googlesource.com/go |
发布版本 | 1.9.2 |