Go参考手册
反射 | reflect
反射 | reflect
import "reflect"
- 概述
- 索引
概述
reflect 包了实现运行时反射,允许程序使用任意类型操作对象。典型的用法是用静态类型接口{}取值并通过调用返回类型的 TypeOf 来提取其动态类型信息。
对 ValueOf 的调用返回表示运行时数据的值。Zero 采用 Type 并返回表示该类型的零值的 Value 。
请参阅“ Reflection 的法则”以了解 Go 中的反射:https://golang.org/doc/articles/laws_of_reflection.html
索引
- func Copy(dst, src Value) int
- func DeepEqual(x, y interface{}) bool
- func Select(cases []SelectCase) (chosen int, recv Value, recvOK bool)
- func Swapper(slice interface{}) func(i, j int)
- type ChanDir
- func (d ChanDir) String() string
- type Kind
- func (k Kind) String() string
- type Method
- type SelectCase
- type SelectDir
- type SliceHeader
- type StringHeader
- type StructField
- type StructTag
- func (tag StructTag) Get(key string) string
- func (tag StructTag) Lookup(key string) (value string, ok bool)
- type Type
- func ArrayOf(count int, elem Type) Type
- func ChanOf(dir ChanDir, t Type) Type
- func FuncOf(in, out []Type, variadic bool) Type
- func MapOf(key, elem Type) Type
- func PtrTo(t Type) Type
- func SliceOf(t Type) Type
- func StructOf(fields []StructField) Type
- func TypeOf(i interface{}) Type
- type Value
- func Append(s Value, x ...Value) Value
- func AppendSlice(s, t Value) Value
- func Indirect(v Value) Value
- func MakeChan(typ Type, buffer int) Value
- func MakeFunc(typ Type, fn func(args []Value) (results []Value)) Value
- func MakeMap(typ Type) Value
- func MakeMapWithSize(typ Type, n int) Value
- func MakeSlice(typ Type, len, cap int) Value
- func New(typ Type) Value
- func NewAt(typ Type, p unsafe.Pointer) Value
- func ValueOf(i interface{}) Value
- func Zero(typ Type) Value
- func (v Value) Addr() Value
- func (v Value) Bool() bool
- func (v Value) Bytes() []byte
- func (v Value) Call(in []Value) []Value
- func (v Value) CallSlice(in []Value) []Value
- func (v Value) CanAddr() bool
- func (v Value) CanInterface() bool
- func (v Value) CanSet() bool
- func (v Value) Cap() int
- func (v Value) Close()
- func (v Value) Complex() complex128
- func (v Value) Convert(t Type) Value
- func (v Value) Elem() Value
- func (v Value) Field(i int) Value
- func (v Value) FieldByIndex(index []int) Value
- func (v Value) FieldByName(name string) Value
- func (v Value) FieldByNameFunc(match func(string) bool) Value
- func (v Value) Float() float64
- func (v Value) Index(i int) Value
- func (v Value) Int() int64
- func (v Value) Interface() (i interface{})
- func (v Value) InterfaceData() [2]uintptr
- func (v Value) IsNil() bool
- func (v Value) IsValid() bool
- func (v Value) Kind() Kind
- func (v Value) Len() int
- func (v Value) MapIndex(key Value) Value
- func (v Value) MapKeys() []Value
- func (v Value) Method(i int) Value
- func (v Value) MethodByName(name string) Value
- func (v Value) NumField() int
- func (v Value) NumMethod() int
- func (v Value) OverflowComplex(x complex128) bool
- func (v Value) OverflowFloat(x float64) bool
- func (v Value) OverflowInt(x int64) bool
- func (v Value) OverflowUint(x uint64) bool
- func (v Value) Pointer() uintptr
- func (v Value) Recv() (x Value, ok bool)
- func (v Value) Send(x Value)
- func (v Value) Set(x Value)
- func (v Value) SetBool(x bool)
- func (v Value) SetBytes(x []byte)
- func (v Value) SetCap(n int)
- func (v Value) SetComplex(x complex128)
- func (v Value) SetFloat(x float64)
- func (v Value) SetInt(x int64)
- func (v Value) SetLen(n int)
- func (v Value) SetMapIndex(key, val Value)
- func (v Value) SetPointer(x unsafe.Pointer)
- func (v Value) SetString(x string)
- func (v Value) SetUint(x uint64)
- func (v Value) Slice(i, j int) Value
- func (v Value) Slice3(i, j, k int) Value
- func (v Value) String() string
- func (v Value) TryRecv() (x Value, ok bool)
- func (v Value) TrySend(x Value) bool
- func (v Value) Type() Type
- func (v Value) Uint() uint64
- func (v Value) UnsafeAddr() uintptr
- type ValueError
- func (e *ValueError) Error() string
- Bugs
文件包
deepequal.go makefunc.go swapper.go type.go value.go
func Copy(显示源文件)
func Copy(dst, src Value) int
复制将 src 的内容复制到 dst ,直到 dst 被填充或 src 已用尽。它返回复制元素的数量。Dst 和 src 都必须有 Slice 或 Array 类型,而 dst 和 src 必须具有相同的元素类型。
func DeepEqual(显示源文件)
func DeepEqual(x, y interface{}) bool
DeepEqual 报告 x 和 y 是否 “deeply equal” ,定义如下。如果以下情况之一适用,相同类型的两个值是非常相等的。不同类型的值永远不会相等。
当它们的对应元素非常相等时,数组值是非常相等的。
如果它们的导出和未导出的相应字段深度相等,那么结构值是相等的。
如果两者均为零,则 Func 值非常相等;否则他们并不是很平等。
如果接口值具有深刻的等于具体值,则接口值是相等的。
当满足以下所有条件时,Map 是完全相同的:它们都是零或两者都是非零,它们具有相同的长度,并且它们是相同的地图对象或它们对应的键(使用 Go 相等匹配)映射到深度相等的价值。
如果使用 Go 的==运算符或者指向深度相等的值,则指针值相等,如果它们相等则指针值相等。
当满足以下所有条件时,slice 值是相等的:它们都是零或者都是非零,它们具有相同的长度,并且它们指向相同基础数组的相同初始条目(即&x0 == &y0)或其相应的元素(长度)是非常相等的。请注意,非零空片和零片(例如,[] byte {}和[] []byte(nil))并不完全相等。
其他值 - 数字,布尔,字符串和通道 - 如果使用Go的==运算符相等,则它们是相等的。
一般而言,DeepEqual 是 Go 的==运算符的递归放松。然而,这个想法是不可能实现的,没有一些不一致。具体而言,一个值可能与自身不相等,或者是因为它是 func 类型(通常无法比较),或者因为它是浮点型 NaN 值(在浮点比较中不等于自身),或者因为它是浮点型它是包含这种值的数组,结构或接口。另一方面,指针值总是等于它们自己,即使它们指向或包含这样的有问题的值,因为它们使用 Go 的==运算符比较相等,并且这是一个足够的条件,无论内容如何,它都是完全相等的。DeepEqual 已被定义,以便相同的捷径适用于切片和贴图:如果 x 和 y 是相同的切片或相同的贴图,则无论内容如何,它们都是完全相同的。
当 DeepEqual 遍历数据值时,它可能会找到一个循环。第二次和后来的时间,DeepEqual 比较之前比较过的两个指针值,它将这些值视为相等,而不是检查它们指向的值。这确保了 DeepEqual 终止。
func Select(显示源文件)
func Select(cases []SelectCase) (chosen int, recv Value, recvOK bool)
选择执行由案例列表描述的选择操作。与 Go select 语句一样,它会阻塞,直到至少有一种情况可以继续,进行统一的伪随机选择,然后执行该情况。它返回所选情况的索引,如果是接收操作,则返回接收到的值,并返回一个布尔值,指示该值是否与通道上的发送相对应(与通道关闭时接收的零值相对)。
func Swapper(显示源文件)
func Swapper(slice interface{}) func(i, j int)
Swapper 返回一个函数,它交换提供的 slice 中的元素。
如果提供的接口不是切片,Swapper 会发生混乱。
type ChanDir(显示源文件)
ChanDir 代表了一种渠道类型的方向。
type ChanDir int
const (
RecvDir ChanDir = 1 << iota // <-chan
SendDir // chan<-
BothDir = RecvDir | SendDir // chan
)
func (ChanDir) String(显示源文件)
func (d ChanDir) String() string
type Kind(显示源文件)
类型表示类型代表的特定种类。零种类不是一种有效的种类。
type Kind uint
const (
Invalid Kind = iota
Bool
Int
Int8
Int16
Int32
Int64
Uint
Uint8
Uint16
Uint32
Uint64
Uintptr
Float32
Float64
Complex64
Complex128
Array
Chan
Func
Interface
Map
Ptr
Slice
String
Struct
UnsafePointer
)
func (Kind) String(显示源文件)
func (k Kind) String() string
type Method(显示源文件)
Method 代表一种方法。
type Method struct {
// Name is the method name.
// PkgPath is the package path that qualifies a lower case (unexported)
// method name. It is empty for upper case (exported) method names.
// The combination of PkgPath and Name uniquely identifies a method
// in a method set.
// See https://golang.org/ref/spec#Uniqueness_of_identifiers
Name string
PkgPath string
Type Type // method type
Func Value // func with receiver as first argument
Index int // index for Type.Method
}
type SelectCase(显示源文件)
SelectCase 在选择操作中描述了一个案例。这种情况取决于 Dir ,沟通方向。
如果 Dir 是 SelectDefault ,则表示默认情况。Chan 和 Send 必须为零值。
如果 Dir 是 SelectSend ,则表示发送操作。通常 Chan 的基础值必须是一个通道,并且 Send 的基础值必须可分配给通道的元素类型。作为一种特殊情况,如果 Chan 是零值,则忽略该情况,并且发送字段也将被忽略,并且可以是零或非零。
如果 Dir 是 SelectRecv ,则表示接收操作。通常陈的基础价值必须是一个渠道和发送必须是一个零值。如果 Chan 是零值,则该情况将被忽略,但发送必须仍为零值。当选择接收操作时,接收的值由选择返回。
type SelectCase struct {
Dir SelectDir // direction of case
Chan Value // channel to use (for send or receive)
Send Value // value to send (for send)
}
type SelectDir(显示源文件)
SelectDir 描述选择案例的通信方向。
type SelectDir int
const (
SelectSend SelectDir // case Chan <- Send
SelectRecv // case <-Chan:
SelectDefault // default
)
type SliceHeader(显示源文件)
SliceHeader 是切片的运行时表示。它不能被安全地使用或移植,其代表可能会在以后的版本中发生变化。此外,数据字段不足以保证它所引用的数据不会被垃圾收集,因此程序必须保持一个单独的,正确类型的指向底层数据的指针。
type SliceHeader struct {
Data uintptr
Len int
Cap int
}
type StringHeader(显示源文件)
StringHeader 是字符串的运行时表示。它不能被安全地使用或移植,其代表可能会在以后的版本中发生变化。此外,数据字段不足以保证它所引用的数据不会被垃圾收集,因此程序必须保持一个单独的,正确类型的指向底层数据的指针。
type StringHeader struct {
Data uintptr
Len int
}
type StructField(显示源文件)
StructField 描述结构中的单个字段。
type StructField struct {
// Name is the field name.
Name string
// PkgPath is the package path that qualifies a lower case (unexported)
// field name. It is empty for upper case (exported) field names.
// See https://golang.org/ref/spec#Uniqueness_of_identifiers
PkgPath string
Type Type // field type
Tag StructTag // field tag string
Offset uintptr // offset within struct, in bytes
Index []int // index sequence for Type.FieldByIndex
Anonymous bool // is an embedded field
}
type StructTag(显示源文件)
StructTag 是 struct 字段中的标记字符串。
按照惯例,标记字符串是可选空间分隔键:“值”对的串联。每个键都是由空格 (U+0020 ' '),引号(U+0022 '"')和冒号(U+003A ':')以外的非控制字符组成的非空字符串。使用U + 0022'“'字符和 Go 字符串文字语法。
type StructTag string
func (StructTag) Get(显示源文件)
func (tag StructTag) Get(key string) string
获取返回与标记字符串中的键关联的值。如果标签中没有这样的密钥,则 Get 返回空字符串。如果标签不具有传统格式,则 Get 返回的值是未指定的。要确定标记是否显式设置为空字符串,请使用查找。
func (StructTag) Lookup(显示源文件)
func (tag StructTag) Lookup(key string) (value string, ok bool)
查找将返回与标记字符串中的键关联的值。如果密钥存在于标签中,则返回值(可能为空)。否则,返回的值将是空字符串。ok 返回值报告该值是否在标记字符串中明确设置。如果标记不具有传统格式,则查找返回的值未指定。
type Type(显示源文件)
Type 是 Go 类型的表示。
并非所有方法都适用于各种类型。每种方法的文档中都会注明限制(如果有的话)。在调用种类特定的方法之前,使用 Kind 方法找出类型的类型。调用不适合这种类型的方法会导致运行时恐慌。
类型值具有可比性,如使用==运算符。如果两个类型的值表示相同类型,则这两个值相等
type Type interface {
// Align returns the alignment in bytes of a value of
// this type when allocated in memory.
Align() int
// FieldAlign returns the alignment in bytes of a value of
// this type when used as a field in a struct.
FieldAlign() int
// Method returns the i'th method in the type's method set.
// It panics if i is not in the range [0, NumMethod()).
//
// For a non-interface type T or *T, the returned Method's Type and Func
// fields describe a function whose first argument is the receiver.
//
// For an interface type, the returned Method's Type field gives the
// method signature, without a receiver, and the Func field is nil.
Method(int) Method
// MethodByName returns the method with that name in the type's
// method set and a boolean indicating if the method was found.
//
// For a non-interface type T or *T, the returned Method's Type and Func
// fields describe a function whose first argument is the receiver.
//
// For an interface type, the returned Method's Type field gives the
// method signature, without a receiver, and the Func field is nil.
MethodByName(string) (Method, bool)
// NumMethod returns the number of exported methods in the type's method set.
NumMethod() int
// Name returns the type's name within its package.
// It returns an empty string for unnamed types.
Name() string
// PkgPath returns a named type's package path, that is, the import path
// that uniquely identifies the package, such as "encoding/base64".
// If the type was predeclared (string, error) or unnamed (*T, struct{}, []int),
// the package path will be the empty string.
PkgPath() string
// Size returns the number of bytes needed to store
// a value of the given type; it is analogous to unsafe.Sizeof.
Size() uintptr
// String returns a string representation of the type.
// The string representation may use shortened package names
// (e.g., base64 instead of "encoding/base64") and is not
// guaranteed to be unique among types. To test for type identity,
// compare the Types directly.
String() string
// Kind returns the specific kind of this type.
Kind() Kind
// Implements reports whether the type implements the interface type u.
Implements(u Type) bool
// AssignableTo reports whether a value of the type is assignable to type u.
AssignableTo(u Type) bool
// ConvertibleTo reports whether a value of the type is convertible to type u.
ConvertibleTo(u Type) bool
// Comparable reports whether values of this type are comparable.
Comparable() bool
// Bits returns the size of the type in bits.
// It panics if the type's Kind is not one of the
// sized or unsized Int, Uint, Float, or Complex kinds.
Bits() int
// ChanDir returns a channel type's direction.
// It panics if the type's Kind is not Chan.
ChanDir() ChanDir
// IsVariadic reports whether a function type's final input parameter
// is a "..." parameter. If so, t.In(t.NumIn() - 1) returns the parameter's
// implicit actual type []T.
//
// For concreteness, if t represents func(x int, y ... float64), then
//
// t.NumIn() == 2
// t.In(0) is the reflect.Type for "int"
// t.In(1) is the reflect.Type for "[]float64"
// t.IsVariadic() == true
//
// IsVariadic panics if the type's Kind is not Func.
IsVariadic() bool
// Elem returns a type's element type.
// It panics if the type's Kind is not Array, Chan, Map, Ptr, or Slice.
Elem() Type
// Field returns a struct type's i'th field.
// It panics if the type's Kind is not Struct.
// It panics if i is not in the range [0, NumField()).
Field(i int) StructField
// FieldByIndex returns the nested field corresponding
// to the index sequence. It is equivalent to calling Field
// successively for each index i.
// It panics if the type's Kind is not Struct.
FieldByIndex(index []int) StructField
// FieldByName returns the struct field with the given name
// and a boolean indicating if the field was found.
FieldByName(name string) (StructField, bool)
// FieldByNameFunc returns the struct field with a name
// that satisfies the match function and a boolean indicating if
// the field was found.
//
// FieldByNameFunc considers the fields in the struct itself
// and then the fields in any anonymous structs, in breadth first order,
// stopping at the shallowest nesting depth containing one or more
// fields satisfying the match function. If multiple fields at that depth
// satisfy the match function, they cancel each other
// and FieldByNameFunc returns no match.
// This behavior mirrors Go's handling of name lookup in
// structs containing anonymous fields.
FieldByNameFunc(match func(string) bool) (StructField, bool)
// In returns the type of a function type's i'th input parameter.
// It panics if the type's Kind is not Func.
// It panics if i is not in the range [0, NumIn()).
In(i int) Type
// Key returns a map type's key type.
// It panics if the type's Kind is not Map.
Key() Type
// Len returns an array type's length.
// It panics if the type's Kind is not Array.
Len() int
// NumField returns a struct type's field count.
// It panics if the type's Kind is not Struct.
NumField() int
// NumIn returns a function type's input parameter count.
// It panics if the type's Kind is not Func.
NumIn() int
// NumOut returns a function type's output parameter count.
// It panics if the type's Kind is not Func.
NumOut() int
// Out returns the type of a function type's i'th output parameter.
// It panics if the type's Kind is not Func.
// It panics if i is not in the range [0, NumOut()).
Out(i int) Type
// contains filtered or unexported methods
}
func ArrayOf(显示源文件)
func ArrayOf(count int, elem Type) Type
ArrayOf 使用给定的计数和元素类型返回数组类型。例如,如果 t 表示 int ,则 ArrayOf(5, t) 表示 5int 。
如果生成的类型会比可用的地址空间大, ArrayOf 恐慌。
func ChanOf(显示源文件)
func ChanOf(dir ChanDir, t Type) Type
ChanOf 返回具有给定方向和元素类型的通道类型。例如,如果 t 表示 int ,则 ChanOf(RecvDir,t) 表示 <-chan int 。
gc 运行时在通道元素类型上施加了 64 kB 的限制。如果t的大小等于或超过此限制, ChanOf 恐慌。
func FuncOf(显示源文件)
func FuncOf(in, out []Type, variadic bool) Type
FuncOf 返回给定参数和结果类型的函数类型。例如,如果 k 表示 int 并且 e 表示字符串,则 FuncOf([] Type {k},[] Type {e},false) 表示 func(int) 字符串。
可变参数控制函数是否可变。如果 inlen(in)-1 不代表切片并且可变参数为真,则 FuncOf 发生混乱。
func MapOf(显示源文件)
func MapOf(key, elem Type) Type
MapOf 使用给定的键和元素类型返回地图类型。例如,如果 k 表示 int 并且 e 表示字符串,则 MapOf(k, e) 表示 mapintstring 。
如果键类型不是有效的映射键类型(也就是说,如果它不执行 Go 的==运算符),则 MapOf 发生混乱。
func PtrTo(显示源文件)
func PtrTo(t Type) Type
PtrTo 返回指向元素t的指针类型。例如,如果 t 表示类型 Foo ,则 PtrT(t) 表示 * Foo 。
func SliceOf(显示源文件)
func SliceOf(t Type) Type
SliceOf 返回元素类型为 t 的切片类型。例如,如果 t 表示 int , SliceOf(t) 表示 [] int 。
func StructOf(显示源文件)
func StructOf(fields []StructField) Type
StructOf 返回包含字段的结构类型。偏移量和索引字段被忽略和计算,就像编译器一样。
StructOf 目前不会为嵌入字段生成封装器方法。未来版本中可能会取消此限制。
func TypeOf(显示源文件)
func TypeOf(i interface{}) Type
TypeOf 返回表示i的动态类型的反射 Type 。如果我是一个无接口值, TypeOf 返回 nil 。
type Value(显示源文件)
Value 是 Go 值的反射界面。
并非所有方法都适用于各种价值。每种方法的文档中都会注明限制(如果有的话)。在调用种类特定的方法之前,使用 Kind 方法找出这种值。调用不适合这种类型的方法会导致运行时间恐慌。
零值表示没有值。它的 IsValid 方法返回 false ,其 Kind 方法返回Invalid,其 String 方法返回 “<invalid Value>” ,所有其他方法都会出现混乱。大多数函数和方法永远不会返回无效值。如果有的话,其文件明确说明条件。
一个值可以被多个 goroutine 同时使用,前提是 Go 值可以同时用于等价的直接操作。
要比较两个值,请比较 Interface 方法的结果。在两个值上使用==不会比较它们表示的基础值。
type Value struct {
// contains filtered or unexported fields
}
func Append(显示源文件)
func Append(s Value, x ...Value) Value
追加将值 x 附加到切片 s 并返回结果切片。和 Go 一样,每个 x 的值必须可分配给切片的元素类型。
func AppendSlice(显示源文件)
func AppendSlice(s, t Value) Value
AppendSlice 将切片 t 附加到切片 s 并返回结果切片。切片 s 和 t 必须具有相同的元素类型。
func Indirect(显示源文件)
func Indirect(v Value) Value
间接返回 v 指向的值。如果 v 是一个零指针,则间接返回一个零值。如果 v 不是指针,则间接返回 v 。
func MakeChan(显示源文件)
func MakeChan(typ Type, buffer int) Value
MakeChan 使用指定的类型和缓冲区大小创建一个新的通道。
func MakeFunc(显示源文件)
func MakeFunc(typ Type, fn func(args []Value) (results []Value)) Value
MakeFunc 返回一个包装函数 fn 的给定类型的新函数。被调用时,该新功能执行以下操作:
- converts its arguments to a slice of Values.
- runs results := fn(args).
- returns the results as a slice of Values, one per formal result.
实现 fn 可以假定参数 Value slice 具有由 typ 给出的参数的数量和类型。如果 typ 描述了一个可变参数函数,那么最终的值本身就是一个表示可变参数的分片,就像可变参数函数的主体一样。结果由 fn 返回的值切片必须具有由 typ 给出的结果的数量和类型。
Value.Call 方法允许调用者根据值调用类型化函数; 相比之下, MakeFunc 允许调用者根据值实现类型化函数。
文档的 Examples 部分包含了如何使用 MakeFunc 为不同类型构建交换函数的说明。
func MakeMap(显示源文件)
func MakeMap(typ Type) Value
MakeMap 使用指定的类型创建一个新的地图。
func MakeMapWithSize(显示源文件)
func MakeMapWithSize(typ Type, n int) Value
MakeMapWithSize 为约 n 个元素创建一个具有指定类型和初始空间的新映射。
func MakeSlice(显示源文件)
func MakeSlice(typ Type, len, cap int) Value
MakeSlice 为指定的切片类型,长度和容量创建新的零初始化切片值。
func New(显示源文件)
func New(typ Type) Value
New 返回一个 Value ,表示指向指定类型的新零值的指针。也就是说,返回值的类型是 PtrTo(typ) 。
func NewAt(显示源文件)
func NewAt(typ Type, p unsafe.Pointer) Value
NewAt 返回一个表示指向指定类型值的指针的值,使用 p 作为指针。
func ValueOf(显示源文件)
func ValueOf(i interface{}) Value
ValueOf 返回一个新值,初始化为存储在接口 i 中的具体值。ValueOf(nil) 返回零值。
func Zero(显示源文件)
func Zero(typ Type) Value
Zero 返回表示指定类型的零值的值。结果与 Value 结构的零值不同,后者完全不表示任何值。例如, Zero(TypeOf(42)) 返回一个 Value with Kind Int 和值0。返回的值既不可寻址也不可设置。
func (Value) Addr(显示源文件)
func (v Value) Addr() Value
Addr 返回一个代表 v 的地址的指针值,如果 CanAddr() 返回 false ,则会发生混乱。 Addr 通常用于获取指向结构体字段或切片元素的指针,以便调用需要指针接收器的方法。
func (Value) Bool(显示源文件)
func (v Value) Bool() bool
布尔返回 v 的基础价值。如果 v 的种类不是 Bool ,它会感到恐慌。
func (Value) Bytes(显示源文件)
func (v Value) Bytes() []byte
字节返回 v 的基础值。如果 v 的基础值不是一个字节片段,它会发生混乱。
func (Value) Call(显示源文件)
func (v Value) Call(in []Value) []Value
例如,如果 len(in)== 3,v.Call(in) 表示 Go 调用 v(in0,in1,in2) 。如果 v 的 Kind 不是 Func ,则调用 panics 。它将输出结果作为值返回。和 Go 一样,每个输入参数必须可以分配给函数相应输入参数的类型。如果 v 是一个可变参数函数, Call 将自己创建可变参数切片参数,并复制相应的值。
func (Value) CallSlice(显示源文件)
func (v Value) CallSlice(in []Value) []Value
CallSlice 调用带有输入参数的可变参数函数 v ,将片段 inlen(in)-1 分配给 v 的最终可变参数。例如,如果 len(in)== 3 , v.CallSlice(in) 表示 Go 呼叫 v(in0,in1,in2 ...) 。如果 v 的 Kind 不是 Func 或者 v 不是可变参数, CallSlice 会发生混乱。它将输出结果作为值返回。和 Go 一样,每个输入参数必须可以分配给函数相应输入参数的类型。
func (Value) CanAddr(显示源文件)
func (v Value) CanAddr() bool
CanAddr 报告是否可以使用 Addr 获取该值的地址。这些值称为可寻址的。如果值是切片的元素,可寻址数组的元素,可寻址结构的字段或取消引用指针的结果,则该值是可寻址的。如果 CanAddr 返回 false ,调用 Addr 将会发生混乱。
func (Value) CanInterface(显示源文件)
func (v Value) CanInterface() bool
CanInterface 报告 Interface 是否可以在没有恐慌的情况下使用。
func (Value) CanSet(显示源文件)
func (v Value) CanSet() bool
CanSet 报告 v 的值是否可以改变。值只能在可寻址的情况下更改,并且不能通过使用未导出的结构字段获取。如果 CanSet 返回 false ,则调用 Set 或任何类型特定的 setter (例如 SetBool ,SetInt )将会发生混乱。
func (Value) Cap(显示源文件)
func (v Value) Cap() int
Cap 返回 v 的容量。如果 v 的 Kind 不是 Array,Chan 或 Slice ,它会发生混乱。
func (Value) Close(显示源文件)
func (v Value) Close()
关闭关闭频道 v 。如果 v 的 Kind 不是 Chan ,它会发生混乱。
func (Value) Complex(显示源文件)
func (v Value) Complex() complex128
综合体返回 v 的底层价值,作为一个综合体128。如果 v 的 Kind 不是 Complex64 或 Complex128 ,它会发生混乱
func (Value) Convert(显示源文件)
func (v Value) Convert(t Type) Value
转换返回转换为类型 t 的值 v 。如果通常的 Go 转换规则不允许将值 v 转换为类型 t ,则转换恐慌。
func (Value) Elem(显示源文件)
func (v Value) Elem() Value
Elem 返回接口 v 包含的值或指针 v 指向的值。如果 v 的 Kind 不是 Interface 或 Ptr ,它会发生混乱。如果 v 为零,它返回零值。
func (Value) Field(显示源文件)
func (v Value) Field(i int) Value
Field 返回 struct v 的第 i 个字段,如果 v 的 Kind 不是 Struct 或者我超出范围,它就会发生混乱。
func (Value) FieldByIndex(显示源文件)
func (v Value) FieldByIndex(index []int) Value
FieldByIndex 返回索引对应的嵌套字段。如果 v 的 Kind 不是 struct ,它会发生混乱。
func (Value) FieldByName(显示源文件)
func (v Value) FieldByName(name string) Value
FieldByName 返回给定名称的 struct 字段。如果没有找到字段,它将返回零值。如果 v 的 Kind 不是 struct ,它会发生混乱。
func (Value) FieldByNameFunc(显示源文件)
func (v Value) FieldByNameFunc(match func(string) bool) Value
FieldByNameFunc 返回结构字段,其名称满足匹配函数。如果 v 的 Kind 不是 struct ,它会发生混乱。如果没有找到字段,它将返回零值。
func (Value) Float(显示源文件)
func (v Value) Float() float64
Float 返回 v 的基础值,作为 float64 。如果 v 的 Kind 不是 Float32 或 Float64 ,它会发生混乱
func (Value) Index(显示源文件)
func (v Value) Index(i int) Value
索引返回 v 的第 i 个元素。如果 v 的 Kind 不是 Array,Slice 或 String ,或者我超出范围,它会发生混乱。
func (Value) Int(显示源文件)
func (v Value) Int() int64
Int 返回 v 的基础值,如 int64 。如果 v 的 Kind 不是 Int , Int8 , Int16 , Int32 或 Int64 ,则会发生混乱。
func (Value) Interface(显示源文件)
func (v Value) Interface() (i interface{})
接口返回 v 的当前值作为 interface{} 。它相当于:
var i interface{} = (v's underlying value)
如果通过访问未导出的结构体域来获取值,它会发生混乱。
func (Value) InterfaceData(显示源文件)
func (v Value) InterfaceData() [2]uintptr
InterfaceData 以 uintptr 对的形式返回接口 v 的值。如果 v 的 Kind 不是 Interface ,它会发生混乱。
func (Value) IsNil(显示源文件)
func (v Value) IsNil() bool
IsNil 报告其参数 v 是否为零。参数必须是 chan , func ,接口,映射,指针或切片值; 如果不是,IsNil 恐慌。请注意,IsNil 并非总是与 Go 中的零比较。例如,如果通过使用未初始化的接口变量 i 调用 ValueOf 来创建 v ,那么 i == nil 将为 true,但 v.IsNil 会因为 v 将是零值而发生混乱。
func (Value) IsValid(显示源文件)
func (v Value) IsValid() bool
IsValid 报告 v 是否表示一个值。如果 v 是零值,它将返回 false 。如果 IsValid 返回 false ,除了 String panic 之外的所有其他方法。大多数函数和方法永远不会返回无效值。如果有的话,其文件明确说明条件。
func (Value) Kind(显示源文件)
func (v Value) Kind() Kind
Kind返回v的Kind。如果v是零值(IsValid返回false),Kind将返回无效。
func (Value) Len(显示源文件)
func (v Value) Len() int
Len 返回 v 的长度。如果 v 的 Kind 不是 Array,Chan,Map,Slice 或 String,它会发生混乱。
func (Value) MapIndex(显示源文件)
func (v Value) MapIndex(key Value) Value
MapIndex 返回地图 v 中与键相关的值。如果 v 的 Kind 不是 Map ,它会发生混乱。如果在地图中未找到键或者如果 v 表示零映射,它将返回零值。和 Go 一样,键的值必须可以分配给地图的键类型。
func (Value) MapKeys(显示源文件)
func (v Value) MapKeys() []Value
MapKeys 以未指定的顺序返回包含地图中所有键的切片。如果 v 的 Kind 不是 Map ,它会惊慌失措。如果 v 表示一个零映射,它将返回一个空片段。
func (Value) Method(显示源文件)
func (v Value) Method(i int) Value
方法返回对应于 v 的第 i 个方法的函数值。对返回函数调用的参数不应包含接收方; 返回的函数将始终使用v作为接收者。如果我超出范围或者 v 是一个无界面值,方法会发生混乱。
func (Value) MethodByName(显示源文件)
func (v Value) MethodByName(name string) Value
MethodByName 返回与给定名称的v的方法对应的函数值。对返回函数调用的参数不应包含接收方; 返回的函数将始终使用 v 作为接收者。如果没有找到方法,它返回零值。
func (Value) NumField(显示源文件)
func (v Value) NumField() int
NumField 返回 struct v 中的字段数。如果 v 的 Kind 不是 Struct ,则会发生混乱。
func (Value) NumMethod(显示源文件)
func (v Value) NumMethod() int
NumMethod 返回值的方法集中导出方法的数量。
func (Value) OverflowComplex(显示源文件)
func (v Value) OverflowComplex(x complex128) bool
OverflowComplex 报告 complex128 x 是否不能用 v 的类型表示。如果 v 的 Kind 不是 Complex64 或 Complex128,它会发生混乱。
func (Value) OverflowFloat(显示源文件)
func (v Value) OverflowFloat(x float64) bool
OverflowFloat 报告 float64 x 是否不能由 v 的类型表示。如果 v 的 Kind 不是 Float32 或 Float64 ,它会发生混乱。
func (Value) OverflowInt(显示源文件)
func (v Value) OverflowInt(x int64) bool
OverflowInt 报告 int64 x 是否不能用 v 的类型表示。如果 v 的 Kind 不是 Int,Int8,int16,Int32 或 Int64,它会发生混乱。
func (Value) OverflowUint(显示源文件)
func (v Value) OverflowUint(x uint64) bool
OverflowUint 报告 uint64 x 是否不能用 v 的类型表示。如果 v 的 Kind 不是 Uint,Uintptr,Uint8,Uint16,Uint32 或 Uint64,它会发生混乱。
func (Value) Pointer(显示源文件)
func (v Value) Pointer() uintptr
指针返回 v 的值作为 uintptr 。它返回 uintptr 而不是 unsafe.Pointer,以便使用 reflect 的代码无法显式地导入不安全的包而无法获取不安全的指针。如果 v 的 Kind 不是 Chan,Func,Map,Ptr,Slice 或 UnsafePointer,它会发生混乱。
如果 v 的 Kind 是 Func,则返回的指针是底层代码指针,但不一定足以唯一地标识单个函数。唯一的保证是,当且仅当 v 是一个 nil func 值时,结果为零。
如果 v 的种类是切片,返回的指针是切片的第一个元素。如果切片为零,则返回值为0.如果切片为空,但非零,则返回值不为零。
func (Value) Recv(显示源文件)
func (v Value) Recv() (x Value, ok bool)
Recv 接收并返回来自频道 v 的值。如果 v 的 Kind 不是 Chan,它会发生混乱。接收阻塞,直到值准备就绪。如果值 x 对应于通道上的发送,则布尔值 ok;如果由于通道已关闭而接收到零值,则返回 false 。
func (Value) Send(显示源文件)
func (v Value) Send(x Value)
发送在通道 v 上发送 x ,如果 v 的类型不是 Chan,或者 x 的类型与 v 的元素类型不是同一类型,则会发生混乱。和 Go 一样,x 的值必须可分配给通道的元素类型。
func (Value) Set(显示源文件)
func (v Value) Set(x Value)
将 x 赋值给 v 。如果 CanSet 返回 false,则会发生混乱。和 Go 一样, x 的值必须赋值给 v 的类型。
func (Value) SetBool(显示源文件)
func (v Value) SetBool(x bool)
SetBool 设置 v 的基础值。如果 v 的 Kind 不是 Bool 或者 CanSet() 是 false ,它会发生混乱。
func (Value) SetBytes(显示源文件)
func (v Value) SetBytes(x []byte)
SetBytes 设置 v 的基础值。如果 v 的基础值不是一个字节片段,它会发生混乱。
func (Value) SetCap(显示源文件)
func (v Value) SetCap(n int)
SetCap 将 v 的容量设置为 n 。如果 v 的 Kind 不是 Slice,或者 n 小于长度或大于切片的容量,它会发生混乱。
func (Value) SetComplex(显示源文件)
func (v Value) SetComplex(x complex128)
SetComplex 将 v 的基础值设置为 x 。如果 v 的 Kind 不是 Complex64 或 Complex128 ,或者 CanSet() 为 false ,则会发生混乱。
func (Value) SetFloat(显示源文件)
func (v Value) SetFloat(x float64)
SetFloat 将 v 的基础值设置为 x 。如果 v 的 Kind 不是 Float32 或 Float64 ,或者 CanSet() 是 false ,它会发生混乱。
func (Value) SetInt(显示源文件)
func (v Value) SetInt(x int64)
SetInt 将 v 的基础值设置为 x 。如果 v 的 Kind 不是 Int,Int8,Int16,Int32 或 Int64,或者 CanSet() 为 false,它会发生混乱。
func (Value) SetLen(显示源文件)
func (v Value) SetLen(n int)
SetLen 将 v 的长度设置为 n 。如果 v 的 Kind 不是 Slice 或 n 是负值或大于切片的容量,它会发生混乱。
func (Value) SetMapIndex(显示源文件)
func (v Value) SetMapIndex(key, val Value)
SetMapIndex 将与地图 v 中的键关联的值设置为 val 。如果 v 的 Kind 不是 Map ,它会惊慌失措。如果 val 是零值,则 SetMapIndex 从地图中删除键。否则,如果 v 拥有一个零映射, SetMapIndex 将会出现混乱。与 Go 中一样,键的值必须可以分配给地图的键类型,并且 val 的值必须可以分配给地图的值类型。
func (Value) SetPointer(显示源文件)
func (v Value) SetPointer(x unsafe.Pointer)
SetPointer 将 unsafe.Pointer 值 v 设置为 x 。如果 v 的 Kind 不是 UnsafePointer ,它会发生混乱。
func (Value) SetString(显示源文件)
func (v Value) SetString(x string)
SetString 将 v 的基础值设置为 x 。如果 v 的 Kind 不是 String 或者 CanSet() 为 false ,它会发生混乱。
func (Value) SetUint(显示源文件)
func (v Value) SetUint(x uint64)
SetUint 将 v 的基础值设置为 x 。如果 v 的 Kind 不是 Uint,Uintptr,Uint8,Uint16,Uint32 或 Uint64,或者 CanSet() 为 false,它会发生混乱。
func (Value) Slice(显示源文件)
func (v Value) Slice(i, j int) Value
切片返回 vi:j 。如果 v 的 Kind 不是 Array,Slice 或 String,或者如果 v 是不可寻址的数组,或者索引超出范围,它会发生混乱。
func (Value) Slice3(显示源文件)
func (v Value) Slice3(i, j, k int) Value
Slice3 是 slice 操作的3索引形式:它返回 vi:j:k 。如果 v 的 Kind 不是 Array 或 Slice ,或者 v 是不可寻址的数组,或者索引超出范围,它会发生混乱。
func (Value) String(显示源文件)
func (v Value) String() string
字符串以字符串形式返回字符串v的基础值。由于 Go 的 String 方法约定,字符串是一种特殊情况。与其他 getter 不同,如果 v 的 Kind 不是 String,它不会惊慌。相反,它返回一个形式为“<T value>”的字符串,其中 T 是 v 的类型。fmt 包专门处理值。它不隐式地调用它们的 String 方法,而是打印它们保存的具体值。
func (Value) TryRecv(显示源文件)
func (v Value) TryRecv() (x Value, ok bool)
TryRecv 尝试从通道 v 接收一个值,但不会阻塞。如果 v 的 Kind 不是 Chan ,它会惊慌失措。如果接收提供了一个值,x 是传输值,ok 是真实的。如果接收不能没有阻塞地完成,则 x 是零值并且 ok 是假的。如果通道关闭,x 是通道元素类型的零值,ok 是假。
func (Value) TrySend(显示源文件)
func (v Value) TrySend(x Value) bool
TrySend 尝试在通道 v 上发送 x,但不会阻止。如果 v 的 Kind 不是 Chan ,它会惊慌失措。它报告是否发送了该值。和 Go 一样, x 的值必须可分配给通道的元素类型。
func (Value) Type(显示源文件)
func (v Value) Type() Type
类型返回 v 的类型。
func (Value) Uint(显示源文件)
func (v Value) Uint() uint64
Uint 返回 v 的基础值,作为 uint64 。如果 v 的 Kind 不是 Uint,Uintptr,Uint8,Uint16,Uint32 或 Uint64,它会发生混乱。
func (Value) UnsafeAddr(显示源文件)
func (v Value) UnsafeAddr() uintptr
UnsafeAddr 返回一个指向 v 数据的指针。对于那些也导入“不安全”软件包的高级客户来说。如果 v 不可寻址,它会发生混乱。
type ValueError(显示源文件)
如果在不支持 Value 的 Value 上调用 Value 方法,则会发生 ValueError 。这些情况记录在每种方法的描述中。
type ValueError struct {
Method string
Kind Kind
}
func (*ValueError) Error(显示源文件)
func (e *ValueError) Error() string
Bugs
- ☞ 如果名字相同,FieldByName 和相关函数认为结构字段名称是相等的,即使它们是源自不同包中的未导出名称。这样做的实际效果是,如果结构类型t包含多个名为x的字段(从不同的包中嵌入),则 t.FieldByName(“x”) 的结果没有很好地定义。FieldByName 可能会返回其中一个名为x的字段,或者可能会报告没有字段。有关更多详细信息,请参阅 golang.org/issue/4876 。
反射 | reflect | ||
---|---|---|
反射 | reflect | 详细 |
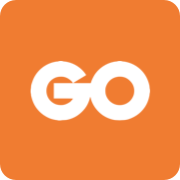
Go 是一种编译型语言,它结合了解释型语言的游刃有余,动态类型语言的开发效率,以及静态类型的安全性。它也打算成为现代的,支持网络与多核计算的语言。要满足这些目标,需要解决一些语言上的问题:一个富有表达能力但轻量级的类型系统,并发与垃圾回收机制,严格的依赖规范等等。这些无法通过库或工具解决好,因此Go也就应运而生了。
主页 | https://golang.org/ |
源码 | https://go.googlesource.com/go |
发布版本 | 1.9.2 |