Go参考手册
net/http
net/http
import "net/http"
- 概述
- 索引
- 示例
- 子目录
概述
http包提供 HTTP 客户端和服务器实现。
Get,Head,Post和 PostForm 发出 HTTP(或HTTPS)请求:
resp, err := http.Get("http://example.com/")
...
resp, err := http.Post("http://example.com/upload", "image/jpeg", &buf)
...
resp, err := http.PostForm("http://example.com/form",
url.Values{"key": {"Value"}, "id": {"123"}})
客户必须在完成后关闭响应主体:
resp, err := http.Get("http://example.com/")
if err != nil {
// 处理错误
}
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
// ...
要控制HTTP客户端标题,重定向策略和其他设置,请创建一个客户端:
client := &http.Client{
CheckRedirect: redirectPolicyFunc,
}
resp, err := client.Get("http://example.com")
// ...
req, err := http.NewRequest("GET", "http://example.com", nil)
// ...
req.Header.Add("If-None-Match", `W/"wyzzy"`)
resp, err := client.Do(req)
// ...
为了控制代理,TLS配置,保活,压缩和其他设置,请创建一个传输:
tr := &http.Transport{
MaxIdleConns: 10,
IdleConnTimeout: 30 * time.Second,
DisableCompression: true,
}
client := &http.Client{Transport: tr}
resp, err := client.Get("https://example.com")
客户端和传输对于多个 goroutine 并发使用是安全的,并且效率应该只创建一次并重新使用。
ListenAndServe 启动一个具有给定地址和处理程序的HTTP服务器。处理程序通常为零,这意味着使用DefaultServeMux 。Handle 和HandleFunc 将处理程序添加到 DefaultServeMux:
http.Handle("/foo", fooHandler)
http.HandleFunc("/bar", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, %q", html.EscapeString(r.URL.Path))
})
log.Fatal(http.ListenAndServe(":8080", nil))
通过创建自定义服务器,可以更好地控制服务器的行为:
s := &http.Server{
Addr: ":8080",
Handler: myHandler,
ReadTimeout: 10 * time.Second,
WriteTimeout: 10 * time.Second,
MaxHeaderBytes: 1 << 20,
}
log.Fatal(s.ListenAndServe())
从Go 1.6开始,http包在使用 HTTPS 时对 HTTP/2 协议有透明的支持。必须禁用 HTTP/2 的程序可以通过将 Transport.TLSNextProto(对于客户端)或 Server.TLSNextProto(对于服务器)设置为非零空映射来实现。或者,当前支持以下 GODEBUG 环境变量:
GODEBUG=http2client=0 # disable HTTP/2 client support
GODEBUG=http2server=0 # disable HTTP/2 server support
GODEBUG=http2debug=1 # enable verbose HTTP/2 debug logs
GODEBUG=http2debug=2 # ... even more verbose, with frame dumps
Go 的 API 兼容性承诺不涵盖 GODEBUG 变量。请在禁用 HTTP/2 支持之前报告任何问题:https://golang.org/s/http2bug
http包的传输和服务器都自动为简单配置启用 HTTP/2 支持。要为更复杂的配置启用 HTTP/2,使用较低级别的 HTTP/2 功能或使用 Go 的 http2 软件包的较新版本,请直接导入 “golang.org/x/net/http2” 并使用其配置传输和/或 ConfigureServer 功能。通过 golang.org/x/net/http2 软件包手动配置 HTTP/2 优先于net/http 软件包内置的 HTTP/2 支持。
索引
- 常量
- 变量
- func CanonicalHeaderKey(s string) string
- func DetectContentType(data []byte) string
- func Error(w ResponseWriter, error string, code int)
- func Handle(pattern string, handler Handler)
- func HandleFunc(pattern string, handler func(ResponseWriter, *Request))
- func ListenAndServe(addr string, handler Handler) error
- func ListenAndServeTLS(addr, certFile, keyFile string, handler Handler) error
- func MaxBytesReader(w ResponseWriter, r io.ReadCloser, n int64) io.ReadCloser
- func NotFound(w ResponseWriter, r *Request)
- func ParseHTTPVersion(vers string) (major, minor int, ok bool)
- func ParseTime(text string) (t time.Time, err error)
- func ProxyFromEnvironment(req *Request) (*url.URL, error)
- func ProxyURL(fixedURL *url.URL) func(*Request) (*url.URL, error)
- func Redirect(w ResponseWriter, r *Request, url string, code int)
- func Serve(l net.Listener, handler Handler) error
- func ServeContent(w ResponseWriter, req *Request, name string, modtime time.Time, content io.ReadSeeker)
- func ServeFile(w ResponseWriter, r *Request, name string)
- func ServeTLS(l net.Listener, handler Handler, certFile, keyFile string) error
- func SetCookie(w ResponseWriter, cookie *Cookie)
- func StatusText(code int) string
- type Client
- func (c *Client) Do(req *Request) (*Response, error)
- func (c *Client) Get(url string) (resp *Response, err error)
- func (c *Client) Head(url string) (resp *Response, err error)
- func (c *Client) Post(url string, contentType string, body io.Reader) (resp *Response, err error)
- func (c *Client) PostForm(url string, data url.Values) (resp *Response, err error)
- type CloseNotifier
- type ConnState
- func (c ConnState) String() string
- type Cookie
- func (c *Cookie) String() string
- type CookieJar
- type Dir
- func (d Dir) Open(name string) (File, error)
- type File
- type FileSystem
- type Flusher
- type Handler
- func FileServer(root FileSystem) Handler
- func NotFoundHandler() Handler
- func RedirectHandler(url string, code int) Handler
- func StripPrefix(prefix string, h Handler) Handler
- func TimeoutHandler(h Handler, dt time.Duration, msg string) Handler
- type HandlerFunc
- func (f HandlerFunc) ServeHTTP(w ResponseWriter, r *Request)
- type Header
- func (h Header) Add(key, value string)
- func (h Header) Del(key string)
- func (h Header) Get(key string) string
- func (h Header) Set(key, value string)
- func (h Header) Write(w io.Writer) error
- func (h Header) WriteSubset(w io.Writer, exclude map[string]bool) error
- type Hijacker
- type ProtocolError
- func (pe *ProtocolError) Error() string
- type PushOptions
- type Pusher
- type Request
- func NewRequest(method, url string, body io.Reader) (*Request, error)
- func ReadRequest(b *bufio.Reader) (*Request, error)
- func (r *Request) AddCookie(c *Cookie)
- func (r *Request) BasicAuth() (username, password string, ok bool)
- func (r *Request) Context() context.Context
- func (r *Request) Cookie(name string) (*Cookie, error)
- func (r *Request) Cookies() []*Cookie
- func (r *Request) FormFile(key string) (multipart.File, *multipart.FileHeader, error)
- func (r *Request) FormValue(key string) string
- func (r *Request) MultipartReader() (*multipart.Reader, error)
- func (r *Request) ParseForm() error
- func (r *Request) ParseMultipartForm(maxMemory int64) error
- func (r *Request) PostFormValue(key string) string
- func (r *Request) ProtoAtLeast(major, minor int) bool
- func (r *Request) Referer() string
- func (r *Request) SetBasicAuth(username, password string)
- func (r *Request) UserAgent() string
- func (r *Request) WithContext(ctx context.Context) *Request
- func (r *Request) Write(w io.Writer) error
- func (r *Request) WriteProxy(w io.Writer) error
- type Response
- func Get(url string) (resp *Response, err error)
- func Head(url string) (resp *Response, err error)
- func Post(url string, contentType string, body io.Reader) (resp *Response, err error)
- func PostForm(url string, data url.Values) (resp *Response, err error)
- func ReadResponse(r *bufio.Reader, req *Request) (*Response, error)
- func (r *Response) Cookies() []*Cookie
- func (r *Response) Location() (*url.URL, error)
- func (r *Response) ProtoAtLeast(major, minor int) bool
- func (r *Response) Write(w io.Writer) error
- type ResponseWriter
- type RoundTripper
- func NewFileTransport(fs FileSystem) RoundTripper
- type ServeMux
- func NewServeMux() *ServeMux
- func (mux *ServeMux) Handle(pattern string, handler Handler)
- func (mux *ServeMux) HandleFunc(pattern string, handler func(ResponseWriter, *Request))
- func (mux *ServeMux) Handler(r *Request) (h Handler, pattern string)
- func (mux *ServeMux) ServeHTTP(w ResponseWriter, r *Request)
- type Server
- func (srv *Server) Close() error
- func (srv *Server) ListenAndServe() error
- func (srv *Server) ListenAndServeTLS(certFile, keyFile string) error
- func (srv *Server) RegisterOnShutdown(f func())
- func (srv *Server) Serve(l net.Listener) error
- func (srv *Server) ServeTLS(l net.Listener, certFile, keyFile string) error
- func (srv *Server) SetKeepAlivesEnabled(v bool)
- func (srv *Server) Shutdown(ctx context.Context) error
- type Transport
- func (t *Transport) CancelRequest(req *Request)
- func (t *Transport) CloseIdleConnections()
- func (t *Transport) RegisterProtocol(scheme string, rt RoundTripper)
- func (t *Transport) RoundTrip(req *Request) (*Response, error)
示例
FileServer
FileServer (StripPrefix)
Get
Hijacker
ResponseWriter (Trailers)
ServeMux.Handle
StripPrefix
文件包
client.go cookie.go doc.go filetransport.go fs.go h2_bundle.go header.go http.go jar.go method.go request.go response.go server.go sniff.go status.go transfer.go transport.go
常量
常见的HTTP方法。
除非另有说明,否则这些在RFC 7231第4.3节中定义。
const (
MethodGet = "GET"
MethodHead = "HEAD"
MethodPost = "POST"
MethodPut = "PUT"
MethodPatch = "PATCH" // RFC 5789
MethodDelete = "DELETE"
MethodConnect = "CONNECT"
MethodOptions = "OPTIONS"
MethodTrace = "TRACE"
)
向IANA注册的HTTP状态代码。请参阅:http : //www.iana.org/assignments/http-status-codes/http-status-codes.xhtml
const (
StatusContinue = 100 // RFC 7231, 6.2.1
StatusSwitchingProtocols = 101 // RFC 7231, 6.2.2
StatusProcessing = 102 // RFC 2518, 10.1
StatusOK = 200 // RFC 7231, 6.3.1
StatusCreated = 201 // RFC 7231, 6.3.2
StatusAccepted = 202 // RFC 7231, 6.3.3
StatusNonAuthoritativeInfo = 203 // RFC 7231, 6.3.4
StatusNoContent = 204 // RFC 7231, 6.3.5
StatusResetContent = 205 // RFC 7231, 6.3.6
StatusPartialContent = 206 // RFC 7233, 4.1
StatusMultiStatus = 207 // RFC 4918, 11.1
StatusAlreadyReported = 208 // RFC 5842, 7.1
StatusIMUsed = 226 // RFC 3229, 10.4.1
StatusMultipleChoices = 300 // RFC 7231, 6.4.1
StatusMovedPermanently = 301 // RFC 7231, 6.4.2
StatusFound = 302 // RFC 7231, 6.4.3
StatusSeeOther = 303 // RFC 7231, 6.4.4
StatusNotModified = 304 // RFC 7232, 4.1
StatusUseProxy = 305 // RFC 7231, 6.4.5
StatusTemporaryRedirect = 307 // RFC 7231, 6.4.7
StatusPermanentRedirect = 308 // RFC 7538, 3
StatusBadRequest = 400 // RFC 7231, 6.5.1
StatusUnauthorized = 401 // RFC 7235, 3.1
StatusPaymentRequired = 402 // RFC 7231, 6.5.2
StatusForbidden = 403 // RFC 7231, 6.5.3
StatusNotFound = 404 // RFC 7231, 6.5.4
StatusMethodNotAllowed = 405 // RFC 7231, 6.5.5
StatusNotAcceptable = 406 // RFC 7231, 6.5.6
StatusProxyAuthRequired = 407 // RFC 7235, 3.2
StatusRequestTimeout = 408 // RFC 7231, 6.5.7
StatusConflict = 409 // RFC 7231, 6.5.8
StatusGone = 410 // RFC 7231, 6.5.9
StatusLengthRequired = 411 // RFC 7231, 6.5.10
StatusPreconditionFailed = 412 // RFC 7232, 4.2
StatusRequestEntityTooLarge = 413 // RFC 7231, 6.5.11
StatusRequestURITooLong = 414 // RFC 7231, 6.5.12
StatusUnsupportedMediaType = 415 // RFC 7231, 6.5.13
StatusRequestedRangeNotSatisfiable = 416 // RFC 7233, 4.4
StatusExpectationFailed = 417 // RFC 7231, 6.5.14
StatusTeapot = 418 // RFC 7168, 2.3.3
StatusUnprocessableEntity = 422 // RFC 4918, 11.2
StatusLocked = 423 // RFC 4918, 11.3
StatusFailedDependency = 424 // RFC 4918, 11.4
StatusUpgradeRequired = 426 // RFC 7231, 6.5.15
StatusPreconditionRequired = 428 // RFC 6585, 3
StatusTooManyRequests = 429 // RFC 6585, 4
StatusRequestHeaderFieldsTooLarge = 431 // RFC 6585, 5
StatusUnavailableForLegalReasons = 451 // RFC 7725, 3
StatusInternalServerError = 500 // RFC 7231, 6.6.1
StatusNotImplemented = 501 // RFC 7231, 6.6.2
StatusBadGateway = 502 // RFC 7231, 6.6.3
StatusServiceUnavailable = 503 // RFC 7231, 6.6.4
StatusGatewayTimeout = 504 // RFC 7231, 6.6.5
StatusHTTPVersionNotSupported = 505 // RFC 7231, 6.6.6
StatusVariantAlsoNegotiates = 506 // RFC 2295, 8.1
StatusInsufficientStorage = 507 // RFC 4918, 11.5
StatusLoopDetected = 508 // RFC 5842, 7.2
StatusNotExtended = 510 // RFC 2774, 7
StatusNetworkAuthenticationRequired = 511 // RFC 6585, 6
)
DefaultMaxHeaderBytes是HTTP请求中头部允许的最大大小。这可以通过设置Server.MaxHeaderBytes来覆盖。
const DefaultMaxHeaderBytes = 1 << 20 // 1 MB
DefaultMaxIdleConnsPerHost是Transport的MaxIdleConnsPerHost的默认值。
const DefaultMaxIdleConnsPerHost = 2
TimeFormat是在HTTP头中生成时间时使用的时间格式。它就像time.RFC1123一样,但是将GMT编码为时区。格式化的时间必须以UTC格式生成正确的格式。
有关解析此时间格式的信息,请参阅ParseTime。
const TimeFormat = "Mon, 02 Jan 2006 15:04:05 GMT"
TrailerPrefix是ResponseWriter.Header映射键的奇幻的前缀,如果存在,它表示映射条目实际上是用于响应预告片的,而不是响应标头。在ServeHTTP呼叫结束并且值在trailers中发送后,该前缀被剥离。
该机制仅适用于在写入标题之前未知的trailers。如果在写入标题之前,一套trailers是固定的或已知的,则优选正常的trailers机制:
https://golang.org/pkg/net/http/#ResponseWriter
https://golang.org/pkg/net/http/#example_ResponseWriter_trailers
const TrailerPrefix = "Trailer:"
变量
var (
// 推送法返回 ErrNotSupported
// 实现来指示 HTTP\/2 推送支持不是
// 可用。
ErrNotSupported = &ProtocolError{"feature not supported"}
// ErrUnexpectedTrailer 由传输返回, 当服务器
// 答复与拖车头, 但没有块答复。
ErrUnexpectedTrailer = &ProtocolError{"trailer header without chunked transfer encoding"}
// ErrMissingBoundary 是按请求返回的. MultipartReader 当
// request's Content-Type does not include a "boundary" parameter.
ErrMissingBoundary = &ProtocolError{"no multipart boundary param in Content-Type"}
// ErrNotMultipart is returned by Request.MultipartReader when the
// request's Content-Type is not multipart/form-data.
ErrNotMultipart = &ProtocolError{"request Content-Type isn't multipart/form-data"}
// Deprecated: ErrHeaderTooLong is not used.
ErrHeaderTooLong = &ProtocolError{"header too long"}
// Deprecated: ErrShortBody is not used.
ErrShortBody = &ProtocolError{"entity body too short"}
// Deprecated: ErrMissingContentLength is not used.
ErrMissingContentLength = &ProtocolError{"missing ContentLength in HEAD response"}
)
HTTP服务器使用的错误。
var (
// ErrBodyNotAllowed is returned by ResponseWriter.Write calls
// when the HTTP method or response code does not permit a
// body.
ErrBodyNotAllowed = errors.New("http: request method or response status code does not allow body")
// ErrHijacked is returned by ResponseWriter.Write calls when
// the underlying connection has been hijacked using the
// Hijacker interface. A zero-byte write on a hijacked
// connection will return ErrHijacked without any other side
// effects.
ErrHijacked = errors.New("http: connection has been hijacked")
// ErrContentLength is returned by ResponseWriter.Write calls
// when a Handler set a Content-Length response header with a
// declared size and then attempted to write more bytes than
// declared.
ErrContentLength = errors.New("http: wrote more than the declared Content-Length")
// Deprecated: ErrWriteAfterFlush is no longer used.
ErrWriteAfterFlush = errors.New("unused")
)
var (
// ServerContextKey 是一个上下文键。可用于 HTTP
// 具有上下文的处理程序。WithValue 访问服务器,
// 已启动处理程序。关联的值将为
// 类型 * 服务器。
ServerContextKey = &contextKey{"http-server"}
// LocalAddrContextKey 是一个上下文键。可用于
// 带有上下文的 HTTP 处理程序。WithValue 访问地址
// 连接到达的本地地址。
// 关联的值将为类型网。地址.
LocalAddrContextKey = &contextKey{"local-addr"}
)
DefaultClient是默认的客户端,由Get,Head和Post使用。
var DefaultClient = &Client{}
DefaultServeMux是Serve使用的默认ServeMux。
var DefaultServeMux = &defaultServeMux
ErrAbortHandler是中止处理程序的标志性恐慌值。虽然ServeHTTP的任何恐慌都会中止对客户端的响应,但使用ErrAbortHandler进行恐慌也会禁止将堆栈跟踪记录到服务器的错误日志中。
var ErrAbortHandler = errors.New("net/http: abort Handler")
在正文关闭后读取Request或Response Body时返回ErrBodyReadAfterClose。这通常发生在HTTP处理程序在其ResponseWriter上调用WriteHeader或Write之后读取主体时。
var ErrBodyReadAfterClose = errors.New("http: invalid Read on closed Body")
在超时的处理程序中的ResponseWriter Write调用上返回ErrHandlerTimeout。
var ErrHandlerTimeout = errors.New("http: Handler timeout")
当读取格式不正确的分块编码的请求或响应主体时,返回ErrLineTooLong。
var ErrLineTooLong = internal.ErrLineTooLong
当提供的文件字段名称不存在于请求中或文件字段中时,FormFile将返回ErrMissingFile。
var ErrMissingFile = errors.New("http: no such file")
当找不到cookie时,ErrNoCookie通过Request的Cookie方法返回。
var ErrNoCookie = errors.New("http: named cookie not present")
当没有位置标题时,ErrNoLocation由Response's Location方法返回。
var ErrNoLocation = errors.New("http: no Location header in response")
在调用Shutdown或Close之后,服务器的Serve,ServeTLS,ListenAndServe和ListenAndServeTLS方法会返回ErrServerClosed。
var ErrServerClosed = errors.New("http: Server closed")
ErrSkipAltProtocol是由Transport.RegisterProtocol定义的一个sentinel错误值。
var ErrSkipAltProtocol = errors.New("net/http: skip alternate protocol")
Client.CheckRedirect钩子可以返回ErrUseLastResponse来控制重定向的处理方式。如果返回,则不会发送下一个请求,并且返回其最新的响应,并且其主体未关闭。
var ErrUseLastResponse = errors.New("net/http: use last response")
NoBody是一个没有字节的io.ReadCloser。阅读始终返回EOF和关闭总是返回零。它可用于传出的客户端请求中,以明确表示请求具有零字节。但是,另一种方法是简单地将Request.Body设置为零。
var NoBody = noBody{}
func CanonicalHeaderKey(显示源文件)
func CanonicalHeaderKey(s string) string
CanonicalHeaderKey返回标题密钥的规范格式。规范化将第一个字母和连字符后面的任何字母转换为大写;其余的都转换为小写。例如,“accept-encoding”的规范密钥是“Accept-Encoding”。如果s包含空格或无效标题字段字节,则不做任何修改就返回。
func DetectContentType(显示源文件)
func DetectContentType(data []byte) string
DetectContentType实现http://mimesniff.spec.whatwg.org/中描述的算法来确定给定数据的内容类型。它至多会考虑前512个字节的数据。DetectContentType始终返回一个有效的MIME类型:如果无法确定更具体的MIME类型,则返回“application/octet-stream”。
func Error(显示源文件)
func Error(w ResponseWriter, error string, code int)
错误以指定的错误消息和HTTP代码答复请求。它不以其他方式结束请求;调用者应该确保没有进一步写入w。错误信息应该是纯文本。
func Handle(显示源文件)
func Handle(pattern string, handler Handler)
Handle将给定模式的处理程序注册到DefaultServeMux中。ServeMux的文档解释了模式如何匹配。
func HandleFunc(显示源文件)
func HandleFunc(pattern string, handler func(ResponseWriter, *Request))
HandleFunc在DefaultServeMux中为给定模式注册处理函数。ServeMux的文档解释了模式如何匹配。
func ListenAndServe(显示源文件)
func ListenAndServe(addr string, handler Handler) error
ListenAndServe侦听TCP网络地址addr,然后使用处理程序调用Serve来处理传入连接上的请求。接受的连接被配置为启用TCP保持活动。Handler通常为零,在这种情况下使用DefaultServeMux。
一个简单的示例服务器是:
package main
import (
"io"
"net/http"
"log"
)
// hello world, the web server
func HelloServer(w http.ResponseWriter, req *http.Request) {
io.WriteString(w, "hello, world!\n")
}
func main() {
http.HandleFunc("/hello", HelloServer)
log.Fatal(http.ListenAndServe(":12345", nil))
}
ListenAndServe总是返回一个非零错误。
func ListenAndServeTLS(显示源文件)
func ListenAndServeTLS(addr, certFile, keyFile string, handler Handler) error
ListenAndServeTLS的作用与ListenAndServe完全相同,只不过它需要HTTPS连接。此外,必须提供包含服务器证书和匹配私钥的文件。如果证书由证书颁发机构签署,则certFile应该是服务器证书,任何中间器和CA证书的串联。
一个简单的示例服务器是:
import (
"log"
"net/http"
)
func handler(w http.ResponseWriter, req *http.Request) {
w.Header().Set("Content-Type", "text/plain")
w.Write([]byte("This is an example server.\n"))
}
func main() {
http.HandleFunc("/", handler)
log.Printf("About to listen on 10443. Go to https://127.0.0.1:10443/")
err := http.ListenAndServeTLS(":10443", "cert.pem", "key.pem", nil)
log.Fatal(err)
}
可以使用crypto/tls中的generate_cert.go来生成cert.pem和key.pem。
ListenAndServeTLS总是返回一个非零错误。
func MaxBytesReader(显示源文件)
func MaxBytesReader(w ResponseWriter, r io.ReadCloser, n int64) io.ReadCloser
MaxBytesReader与io.LimitReader类似,但是用于限制传入请求体的大小。与io.LimitReader相反,MaxBytesReader的结果是一个ReadCloser,它为超出限制的Read返回一个非EOF错误,并在其Close方法被调用时关闭底层的阅读器。
MaxBytesReader可防止客户端意外或恶意发送大量请求并浪费服务器资源。
func NotFound(显示源文件)
func NotFound(w ResponseWriter, r *Request)
NotFound使用HTTP 404找不到错误来回复请求。
func ParseHTTPVersion(显示源文件)
func ParseHTTPVersion(vers string) (major, minor int, ok bool)
ParseHTTPVersion分析HTTP版本字符串。“HTTP/1.0”返回(1, 0, true)。
func ParseTime(显示源文件)
func ParseTime(text string) (t time.Time, err error)
ParseTime分析时间标题(例如Date: header),尝试HTTP/1.1允许的三种格式:TimeFormat,time.RFC850和time.ANSIC。
func ProxyFromEnvironment(显示源文件)
func ProxyFromEnvironment(req *Request) (*url.URL, error)
ProxyFromEnvironment返回用于给定请求的代理的URL,如环境变量HTTP_PROXY,HTTPS_PROXY和NO_PROXY(或其小写版本)所示。对于https请求,HTTPS_PROXY优先于HTTP_PROXY。
环境值可以是完整的URL或“host:port”,在这种情况下,假设“http”方案。如果值是不同的形式,则返回错误。
如果没有在环境中定义代理,或者代理不应该用于给定请求(如NO_PROXY所定义),则返回零URL和零错误。
作为一种特殊情况,如果req.URL.Host是“localhost”(带或不带端口号),则会返回一个零URL和零错误。
func ProxyURL(显示源文件)
func ProxyURL(fixedURL *url.URL) func(*Request) (*url.URL, error)
ProxyURL返回一个总是返回相同URL的代理函数(用于传输)。
func Redirect(显示源文件)
func Redirect(w ResponseWriter, r *Request, url string, code int)
将重定向到url的请求重定向到请求,url可能是相对于请求路径的路径。
提供的代码应该位于3xx范围内,通常是StatusMovedPermanently,StatusFound或StatusSeeOther。
func Serve(显示源文件)
func Serve(l net.Listener, handler Handler) error
Serve接受侦听器l上的传入HTTP连接,为每个服务器创建一个新的服务程序。服务程序读取请求,然后调用处理程序来回复它们。Handler通常为零,在这种情况下使用DefaultServeMux。
func ServeContent(显示源文件)
func ServeContent(w ResponseWriter, req *Request, name string, modtime time.Time, content io.ReadSeeker)
ServeContent使用提供的ReadSeeker中的内容答复请求。ServeContent优于io.Copy的主要好处是它正确处理Range请求,设置MIME类型,并处理If-Match,If-Unmodified-Since,If-None-Match,If-Modified-Since和If-Range要求。
如果未设置响应的Content-Type标头,则ServeContent首先尝试从名称的文件扩展名中推导出该类型,如果失败,则返回读取内容的第一个块并将其传递给DetectContentType。这个名字是没有用的;特别是它可以是空的,并且不会在响应中发送。
如果modtime不是零时间或Unix纪元,则ServeContent将其包含在响应中的Last-Modified标头中。如果请求包含If-Modified-Since标头,则ServeContent使用modtime决定是否需要发送内容。
内容的Seek方法必须工作:ServeContent使用搜索到内容的结尾来确定其大小。
如果调用者已经根据RFC 7232第2.3节设置了w的ETag头,则ServeContent使用它来处理使用If-Match,If-None-Match或If-Range的请求。
请注意* os.File实现了io.ReadSeeker接口。
func ServeFile(显示源文件)
func ServeFile(w ResponseWriter, r *Request, name string)
ServeFile使用指定文件或目录的内容答复请求。
如果提供的文件或目录名称是相对路径,则相对于当前目录进行解释并可能上升到父目录。如果提供的名称是从用户输入构建的,则应在调用ServeFile之前对其进行消毒。作为预防措施,ServeFile将拒绝r.URL.Path包含“..”路径元素的请求。
作为一种特殊情况,ServeFile将r.URL.Path以“/index.html”结尾的任何请求重定向到相同的路径,而没有最终的“index.html”。要避免这种重定向,请修改路径或使用ServeContent。
func ServeTLS(显示源文件)
func ServeTLS(l net.Listener, handler Handler, certFile, keyFile string) error
Serve接受listener l上的传入HTTPS连接,为每个服务器创建一个新的服务程序。服务程序读取请求,然后调用处理程序来回复它们。
Handler通常为零,在这种情况下使用DefaultServeMux。
此外,必须提供包含服务器证书和匹配私钥的文件。如果证书由证书颁发机构签署,则certFile应该是服务器证书,任何中间器和CA证书的串联。
func SetCookie(显示源文件)
func SetCookie(w ResponseWriter, cookie *Cookie)
SetCookie将一个Set-Cookie头添加到提供的ResponseWriter的头文件中。提供的cookie必须具有有效的名称。无效的Cookie可能会悄悄丢弃。
func StatusText(显示源文件)
func StatusText(code int) string
StatusText返回HTTP状态码的文本。如果代码未知,它将返回空字符串。
type Client(显示源文件)
客户端是一个HTTP客户端。其零值(DefaultClient)是使用DefaultTransport的可用客户端。
客户端的传输通常具有内部状态(缓存的TCP连接),因此客户端应该被重用,而不是根据需要创建。客户端可以安全地由多个goroutine并发使用。
客户端比RoundTripper(比如Transport)更高级,并且还处理诸如cookie和重定向之类的HTTP细节。
在重定向之后,客户端将转发在初始请求中设置的所有标头,除了:
•将“Authorization”,“WWW-Authenticate”和“Cookie”等敏感标头转发给不受信任的目标时。在重定向到不是子域匹配的域或初始域的完全匹配时,这些标头将被忽略。例如,从“foo.com”到“foo.com”或“sub.foo.com”的重定向将转发敏感标题,但重定向到“bar.com”则不会。
•转发“Cookie”标头时使用非零 cookie Jar。由于每个重定向可能会改变cookie jar的状态,重定向可能会改变初始请求中设置的cookie。转发“Cookie”标头时,任何突变的Cookie都将被忽略,并期望Jar将插入这些带有更新值的突变cookie(假设该匹配的来源)。如果Jar为零,则最初的cookie将不发送更改。
type Client struct {
// Transport specifies the mechanism by which individual
// HTTP requests are made.
// If nil, DefaultTransport is used.
Transport RoundTripper
// CheckRedirect specifies the policy for handling redirects.
// If CheckRedirect is not nil, the client calls it before
// following an HTTP redirect. The arguments req and via are
// the upcoming request and the requests made already, oldest
// first. If CheckRedirect returns an error, the Client's Get
// method returns both the previous Response (with its Body
// closed) and CheckRedirect's error (wrapped in a url.Error)
// instead of issuing the Request req.
// As a special case, if CheckRedirect returns ErrUseLastResponse,
// then the most recent response is returned with its body
// unclosed, along with a nil error.
//
// If CheckRedirect is nil, the Client uses its default policy,
// which is to stop after 10 consecutive requests.
CheckRedirect func(req *Request, via []*Request) error
// Jar specifies the cookie jar.
//
// The Jar is used to insert relevant cookies into every
// outbound Request and is updated with the cookie values
// of every inbound Response. The Jar is consulted for every
// redirect that the Client follows.
//
// If Jar is nil, cookies are only sent if they are explicitly
// set on the Request.
Jar CookieJar
// Timeout specifies a time limit for requests made by this
// Client. The timeout includes connection time, any
// redirects, and reading the response body. The timer remains
// running after Get, Head, Post, or Do return and will
// interrupt reading of the Response.Body.
//
// A Timeout of zero means no timeout.
//
// The Client cancels requests to the underlying Transport
// using the Request.Cancel mechanism. Requests passed
// to Client.Do may still set Request.Cancel; both will
// cancel the request.
//
// For compatibility, the Client will also use the deprecated
// CancelRequest method on Transport if found. New
// RoundTripper implementations should use Request.Cancel
// instead of implementing CancelRequest.
Timeout time.Duration
}
func (*Client) Do(显示源文件)
func (c *Client) Do(req *Request) (*Response, error)
不要发送HTTP请求,并按照客户端上配置的策略(例如redirects, cookies,auth)返回HTTP响应。
如果由客户端策略(如CheckRedirect)引起,或者无法说出HTTP(如网络连接问题),则返回错误。非2xx状态码不会导致错误。
如果返回的错误为零,则Response将包含一个非零体,用户需要关闭。如果Body尚未关闭,则客户端的底层RoundTripper(通常是Transport)可能无法重新使用持久性TCP连接到服务器以进行后续“保持活动”请求。
如果非零,请求主体将被底层传输关闭,即使出现错误。
出错时,可以忽略任何响应。非零错误的非零响应仅在CheckRedirect失败时发生,即使返回的Response.Body已经关闭。
通常会使用Get,Post或PostForm来代替Do.
如果服务器回复重定向,则客户端首先使用CheckRedirect函数确定是否应该遵循重定向。如果允许,301,302或303重定向会导致后续请求使用HTTP方法GET(如果原始请求是HEAD,则为HEAD),但不包含主体。只要定义了Request.GetBody函数,307或308重定向就会保留原始的HTTP方法和主体。NewRequest函数自动为通用标准库体类型设置GetBody。
func (*Client) Get(显示源文件)
func (c *Client) Get(url string) (resp *Response, err error)
将问题GET获取到指定的URL。如果响应是以下重定向代码之一,则在调用客户端的CheckRedirect函数后,Get遵循重定向:
301 (Moved Permanently)
302 (Found)
303 (See Other)
307 (Temporary Redirect)
308 (Permanent Redirect)
如果客户端的CheckRedirect功能失败或者出现HTTP协议错误,则返回错误。非2xx响应不会导致错误。
当err为零时,resp总是包含非零的resp.Body。来电者在完成阅读后应关闭resp.Body。
要使用自定义标题发出请求,请使用NewRequest和Client.Do。
func (*Client) Head(显示源文件)
func (c *Client) Head(url string) (resp *Response, err error)
Head向指定的URL发出HEAD。如果响应是以下重定向代码之一,则Head在调用客户端的CheckRedirect函数后遵循重定向:
301 (Moved Permanently)
302 (Found)
303 (See Other)
307 (Temporary Redirect)
308 (Permanent Redirect)
func (*Client) Post(显示源文件)
func (c *Client) Post(url string, contentType string, body io.Reader) (resp *Response, err error)
发布POST到指定的URL。
调用者在完成阅读后应关闭resp.Body。
如果提供的主体是io.Closer,则在请求后关闭。
要设置自定义标题,请使用NewRequest和Client.Do。
有关如何处理重定向的详细信息,请参阅Client.Do方法文档。
func (*Client) PostForm(显示源文件)
func (c *Client) PostForm(url string, data url.Values) (resp *Response, err error)
PostForm向指定的URL发布POST,并将数据的键和值作为请求主体进行URL编码。
Content-Type头部设置为application/x-www-form-urlencoded。要设置其他标题,请使用NewRequest和DefaultClient.Do。
当err为零时,resp总是包含非零的resp.Body。调用者在完成阅读后应关闭resp.Body。
有关如何处理重定向的详细信息,请参阅Client.Do方法文档。
type CloseNotifier(显示源文件)
CloseNotifier接口由ResponseWriters实现,它允许检测基础连接何时消失。
如果客户端在响应准备好之前断开连接,则可以使用此机制取消服务器上的长时间操作。
type CloseNotifier interface {
// CloseNotify returns a channel that receives at most a
// single value (true) when the client connection has gone
// away.
//
// CloseNotify may wait to notify until Request.Body has been
// fully read.
//
// After the Handler has returned, there is no guarantee
// that the channel receives a value.
//
// If the protocol is HTTP/1.1 and CloseNotify is called while
// processing an idempotent request (such a GET) while
// HTTP/1.1 pipelining is in use, the arrival of a subsequent
// pipelined request may cause a value to be sent on the
// returned channel. In practice HTTP/1.1 pipelining is not
// enabled in browsers and not seen often in the wild. If this
// is a problem, use HTTP/2 or only use CloseNotify on methods
// such as POST.
CloseNotify() <-chan bool
}
type ConnState(显示源文件)
ConnState表示客户端连接到服务器的状态。它由可选的Server.ConnState挂钩使用。
type ConnState int
const (
// StateNew represents a new connection that is expected to
// send a request immediately. Connections begin at this
// state and then transition to either StateActive or
// StateClosed.
StateNew ConnState = iota
// StateActive represents a connection that has read 1 or more
// bytes of a request. The Server.ConnState hook for
// StateActive fires before the request has entered a handler
// and doesn't fire again until the request has been
// handled. After the request is handled, the state
// transitions to StateClosed, StateHijacked, or StateIdle.
// For HTTP/2, StateActive fires on the transition from zero
// to one active request, and only transitions away once all
// active requests are complete. That means that ConnState
// cannot be used to do per-request work; ConnState only notes
// the overall state of the connection.
StateActive
// StateIdle represents a connection that has finished
// handling a request and is in the keep-alive state, waiting
// for a new request. Connections transition from StateIdle
// to either StateActive or StateClosed.
StateIdle
// StateHijacked represents a hijacked connection.
// This is a terminal state. It does not transition to StateClosed.
StateHijacked
// StateClosed represents a closed connection.
// This is a terminal state. Hijacked connections do not
// transition to StateClosed.
StateClosed
)
func (ConnState) String(显示源文件)
func (c ConnState) String() string
type Cookie(显示源文件)
Cookie表示HTTP响应的Set-Cookie头或HTTP请求的Cookie头中发送的HTTP cookie。
See http://tools.ietf.org/html/rfc6265 for details.
type Cookie struct {
Name string
Value string
Path string // optional
Domain string // optional
Expires time.Time // optional
RawExpires string // for reading cookies only
// MaxAge=0 means no 'Max-Age' attribute specified.
// MaxAge<0 means delete cookie now, equivalently 'Max-Age: 0'
// MaxAge>0 means Max-Age attribute present and given in seconds
MaxAge int
Secure bool
HttpOnly bool
Raw string
Unparsed []string // Raw text of unparsed attribute-value pairs
}
func (*Cookie) String(显示源文件)
func (c *Cookie) String() string
字符串返回用于Cookie标头(如果仅设置了Name和Value)或Set-Cookie响应标头(如果设置了其他字段)的Cookie的序列化。如果c为零或c.Name无效,则返回空字符串。
type CookieJar(显示源文件)
CookieJar管理HTTP请求中cookie的存储和使用。
CookieJar的实现必须安全,以供多个goroutine同时使用。
net/http/cookiejar包提供了一个CookieJar实现。
type CookieJar interface {
// SetCookies handles the receipt of the cookies in a reply for the
// given URL. It may or may not choose to save the cookies, depending
// on the jar's policy and implementation.
SetCookies(u *url.URL, cookies []*Cookie)
// Cookies returns the cookies to send in a request for the given URL.
// It is up to the implementation to honor the standard cookie use
// restrictions such as in RFC 6265.
Cookies(u *url.URL) []*Cookie
}
type Dir(显示源文件)
Dir使用限于特定目录树的本机文件系统实现FileSystem。
虽然FileSystem.Open方法采用'/' - 分隔的路径,但Dir的字符串值是本地文件系统上的文件名,而不是URL,因此它由filepath.Separator分隔,而不一定是'/'。
请注意,Dir将允许访问以句点开头的文件和目录,这可能会暴露敏感目录(如.git目录)或敏感文件(如.htpasswd)。要排除具有前导期的文件,请从服务器中删除文件/目录或创建自定义FileSystem实现。
空的Dir被视为“。”。
type Dir string
func (Dir) Open(显示源文件)
func (d Dir) Open(name string) (File, error)
type File(显示源文件)
File由FileSystem的Open方法返回,并可由FileServer实现提供。
这些方法的行为应该与* os.File中的方法相同。
type File interface {
io.Closer
io.Reader
io.Seeker
Readdir(count int) ([]os.FileInfo, error)
Stat() (os.FileInfo, error)
}
type FileSystem(显示源文件)
FileSystem实现对指定文件集合的访问。无论主机操作系统惯例如何,文件路径中的元素都用斜线('/', U+002F)字符分隔。
type FileSystem interface {
Open(name string) (File, error)
}
type Flusher(显示源文件)
Flusher接口由ResponseWriters实现,它允许HTTP处理器将缓冲数据刷新到客户端。
默认的HTTP/1.x和HTTP/2 ResponseWriter实现支持Flusher,但ResponseWriter包装可能不支持。处理程序应始终在运行时测试此功能。
请注意,即使对于支持Flush的ResponseWriters,如果客户端通过HTTP代理连接,缓存的数据可能无法到达客户端,直到响应完成。
type Flusher interface {
// Flush sends any buffered data to the client.
Flush()
}
type Handler(显示源文件)
Handler响应HTTP请求。
ServeHTTP应该将回复头文件和数据写入ResponseWriter,然后返回。返回请求完成的信号;在完成ServeHTTP调用之后或同时完成使用ResponseWriter或从Request.Body中读取是无效的。
根据HTTP客户端软件,HTTP协议版本以及客户端与Go服务器之间的任何中介,在写入ResponseWriter后,可能无法从Request.Body中读取数据。谨慎的处理程序应先读取Request.Body,然后回复。
除阅读主体外,处理程序不应修改提供的请求。
如果ServeHTTP发生混乱,则服务器(ServeHTTP的调用者)认为恐慌的影响与活动请求分离。它恢复恐慌,将堆栈跟踪记录到服务器错误日志中,并根据HTTP协议关闭网络连接或发送HTTP/2 RST_STREAM。要终止处理程序,以便客户端看到中断的响应,但服务器不记录错误,请使用值ErrAbortHandler恐慌。
type Handler interface {
ServeHTTP(ResponseWriter, *Request)
}
func FileServer(显示源文件)
func FileServer(root FileSystem) Handler
FileServer返回一个处理程序,该处理程序为root用户提供文件系统内容的HTTP请求。
要使用操作系统的文件系统实现,请使用http.Dir:
http.Handle("/", http.FileServer(http.Dir("/tmp")))
作为一种特殊情况,返回的文件服务器将以“/index.html”结尾的任何请求重定向到相同的路径,而没有最终的“index.html”。
示例
package main
import (
"log"
"net/http"
)
func main() {
// Simple static webserver:
log.Fatal(http.ListenAndServe(":8080", http.FileServer(http.Dir("/usr/share/doc"))))
}
示例(StripPrefix)
package main
import (
"net/http"
)
func main() {
// To serve a directory on disk (/tmp) under an alternate URL
// path (/tmpfiles/), use StripPrefix to modify the request
// URL's path before the FileServer sees it:
http.Handle("/tmpfiles/", http.StripPrefix("/tmpfiles/", http.FileServer(http.Dir("/tmp"))))
}
func NotFoundHandler(显示源文件)
func NotFoundHandler() Handler
NotFoundHandler返回一个简单的请求处理程序,用于处理每个请求的“404页未找到”答复。
func RedirectHandler(显示源文件)
func RedirectHandler(url string, code int) Handler
RedirectHandler返回一个请求处理程序,它使用给定的状态码将其收到的每个请求重定向到给定的url。
提供的代码应该位于3xx范围内,通常是StatusMovedPermanently,StatusFound或StatusSeeOther。
func StripPrefix(显示源文件)
func StripPrefix(prefix string, h Handler) Handler
StripPrefix通过从请求URL的Path中移除给定的前缀并调用处理程序h来返回一个处理程序,该处理程序提供HTTP请求。StripPrefix通过回答HTTP 404找不到错误来处理对不以前缀开头的路径的请求。
示例
package main
import (
"net/http"
)
func main() {
// To serve a directory on disk (/tmp) under an alternate URL
// path (/tmpfiles/), use StripPrefix to modify the request
// URL's path before the FileServer sees it:
http.Handle("/tmpfiles/", http.StripPrefix("/tmpfiles/", http.FileServer(http.Dir("/tmp"))))
}
func TimeoutHandler(显示源文件)
func TimeoutHandler(h Handler, dt time.Duration, msg string) Handler
TimeoutHandler返回一个处理器,它在给定的时间限制内运行h。
新的Handler调用h.ServeHTTP来处理每个请求,但是如果一个调用的运行时间超过其时间限制,处理程序会响应503服务不可用错误和其正文中的给定消息。(如果msg为空,将发送一个合适的默认消息。)在这样的超时之后,h写入其ResponseWriter将返回ErrHandlerTimeout。
TimeoutHandler将所有Handler缓冲区写入内存,并且不支持Hijacker或Flusher接口。
type HandlerFunc(显示源文件)
HandlerFunc类型是一个适配器,允许使用普通函数作为HTTP处理程序。如果f是具有适当签名的函数,则HandlerFunc(f)是调用f的Handler。
type HandlerFunc func(ResponseWriter, *Request)
func (HandlerFunc) ServeHTTP(显示源文件)
func (f HandlerFunc) ServeHTTP(w ResponseWriter, r *Request)
ServeHTTP calls f(w, r).
type Header(显示源文件)
标题表示HTTP标题中的键值对。
type Header map[string][]string
func (Header) Add(显示源文件)
func (h Header) Add(key, value string)
添加将关键字值对添加到标题。它附加到与键相关的任何现有值。
func (Header) Del(显示源文件)
func (h Header) Del(key string)
Del删除与键关联的值。
func (Header) Get(显示源文件)
func (h Header) Get(key string) string
获取与给定键相关的第一个值。它不区分大小写; textproto.CanonicalMIMEHeaderKey用于规范提供的密钥。如果没有与该键关联的值,Get返回“”。要访问密钥的多个值或使用非规范密钥,请直接访问地图。
func (Header) Set(显示源文件)
func (h Header) Set(key, value string)
Set将与键关联的标题条目设置为单个元素值。它取代了任何与键相关的现有值。
func (Header) Write(显示源文件)
func (h Header) Write(w io.Writer) error
Write以格式写入标题。
func (Header) WriteSubset(显示源文件)
func (h Header) WriteSubset(w io.Writer, exclude map[string]bool) error
WriteSubset以连线格式写入标题。如果exclude不为零,则不会写入excludekey == true的键。
type Hijacker(显示源文件)
Hijacker接口由ResponseWriters实现,它允许HTTP处理程序接管连接。
HTTP/1.x连接的默认ResponseWriter支持劫持程序,但HTTP/2连接故意不支持。ResponseWriter包装器也可能不支持劫持程序。处理程序应始终在运行时测试此功能。
type Hijacker interface {
// Hijack lets the caller take over the connection.
// After a call to Hijack the HTTP server library
// will not do anything else with the connection.
//
// It becomes the caller's responsibility to manage
// and close the connection.
//
// The returned net.Conn may have read or write deadlines
// already set, depending on the configuration of the
// Server. It is the caller's responsibility to set
// or clear those deadlines as needed.
//
// The returned bufio.Reader may contain unprocessed buffered
// data from the client.
//
// After a call to Hijack, the original Request.Body should
// not be used.
Hijack() (net.Conn, *bufio.ReadWriter, error)
}
示例
package main
import (
"fmt"
"log"
"net/http"
)
func main() {
http.HandleFunc("/hijack", func(w http.ResponseWriter, r *http.Request) {
hj, ok := w.(http.Hijacker)
if !ok {
http.Error(w, "webserver doesn't support hijacking", http.StatusInternalServerError)
return
}
conn, bufrw, err := hj.Hijack()
if err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
// Don't forget to close the connection:
defer conn.Close()
bufrw.WriteString("Now we're speaking raw TCP. Say hi: ")
bufrw.Flush()
s, err := bufrw.ReadString('\n')
if err != nil {
log.Printf("error reading string: %v", err)
return
}
fmt.Fprintf(bufrw, "You said: %q\nBye.\n", s)
bufrw.Flush()
})
}
type ProtocolError(显示源文件)
ProtocolError表示HTTP协议错误。
Deprecated:并非与协议错误有关的http包中的所有错误都是ProtocolError 类型。
type ProtocolError struct {
ErrorString string
}
func (*ProtocolError) Error(显示源文件)
func (pe *ProtocolError) Error() string
type PushOptions(显示源文件)
PushOptions描述了Pusher.Push的选项。
type PushOptions struct {
// Method specifies the HTTP method for the promised request.
// If set, it must be "GET" or "HEAD". Empty means "GET".
Method string
// Header specifies additional promised request headers. This cannot
// include HTTP/2 pseudo header fields like ":path" and ":scheme",
// which will be added automatically.
Header Header
}
type Pusher(显示源文件)
Pusher是ResponseWriters实现的接口,支持HTTP/2服务器推送。有关更多背景信息,请参阅https://tools.ietf.org/html/rfc7540#section-8.2。
type Pusher interface {
// Push initiates an HTTP/2 server push. This constructs a synthetic
// request using the given target and options, serializes that request
// into a PUSH_PROMISE frame, then dispatches that request using the
// server's request handler. If opts is nil, default options are used.
//
// The target must either be an absolute path (like "/path") or an absolute
// URL that contains a valid host and the same scheme as the parent request.
// If the target is a path, it will inherit the scheme and host of the
// parent request.
//
// The HTTP/2 spec disallows recursive pushes and cross-authority pushes.
// Push may or may not detect these invalid pushes; however, invalid
// pushes will be detected and canceled by conforming clients.
//
// Handlers that wish to push URL X should call Push before sending any
// data that may trigger a request for URL X. This avoids a race where the
// client issues requests for X before receiving the PUSH_PROMISE for X.
//
// Push returns ErrNotSupported if the client has disabled push or if push
// is not supported on the underlying connection.
Push(target string, opts *PushOptions) error
}
type Request(显示源文件)
请求表示由服务器接收或由客户端发送的HTTP请求。
客户端和服务器使用情况的字段语义略有不同。除了下面字段的注释之外,请参阅Request.Write和RoundTripper的文档。
type Request struct {
// Method specifies the HTTP method (GET, POST, PUT, etc.).
// For client requests an empty string means GET.
Method string
// URL specifies either the URI being requested (for server
// requests) or the URL to access (for client requests).
//
// For server requests the URL is parsed from the URI
// supplied on the Request-Line as stored in RequestURI. For
// most requests, fields other than Path and RawQuery will be
// empty. (See RFC 2616, Section 5.1.2)
//
// For client requests, the URL's Host specifies the server to
// connect to, while the Request's Host field optionally
// specifies the Host header value to send in the HTTP
// request.
URL *url.URL
// The protocol version for incoming server requests.
//
// For client requests these fields are ignored. The HTTP
// client code always uses either HTTP/1.1 or HTTP/2.
// See the docs on Transport for details.
Proto string // "HTTP/1.0"
ProtoMajor int // 1
ProtoMinor int // 0
// Header contains the request header fields either received
// by the server or to be sent by the client.
//
// If a server received a request with header lines,
//
// Host: example.com
// accept-encoding: gzip, deflate
// Accept-Language: en-us
// fOO: Bar
// foo: two
//
// then
//
// Header = map[string][]string{
// "Accept-Encoding": {"gzip, deflate"},
// "Accept-Language": {"en-us"},
// "Foo": {"Bar", "two"},
// }
//
// For incoming requests, the Host header is promoted to the
// Request.Host field and removed from the Header map.
//
// HTTP defines that header names are case-insensitive. The
// request parser implements this by using CanonicalHeaderKey,
// making the first character and any characters following a
// hyphen uppercase and the rest lowercase.
//
// For client requests, certain headers such as Content-Length
// and Connection are automatically written when needed and
// values in Header may be ignored. See the documentation
// for the Request.Write method.
Header Header
// Body is the request's body.
//
// For client requests a nil body means the request has no
// body, such as a GET request. The HTTP Client's Transport
// is responsible for calling the Close method.
//
// For server requests the Request Body is always non-nil
// but will return EOF immediately when no body is present.
// The Server will close the request body. The ServeHTTP
// Handler does not need to.
Body io.ReadCloser
// GetBody defines an optional func to return a new copy of
// Body. It is used for client requests when a redirect requires
// reading the body more than once. Use of GetBody still
// requires setting Body.
//
// For server requests it is unused.
GetBody func() (io.ReadCloser, error)
// ContentLength records the length of the associated content.
// The value -1 indicates that the length is unknown.
// Values >= 0 indicate that the given number of bytes may
// be read from Body.
// For client requests, a value of 0 with a non-nil Body is
// also treated as unknown.
ContentLength int64
// TransferEncoding lists the transfer encodings from outermost to
// innermost. An empty list denotes the "identity" encoding.
// TransferEncoding can usually be ignored; chunked encoding is
// automatically added and removed as necessary when sending and
// receiving requests.
TransferEncoding []string
// Close indicates whether to close the connection after
// replying to this request (for servers) or after sending this
// request and reading its response (for clients).
//
// For server requests, the HTTP server handles this automatically
// and this field is not needed by Handlers.
//
// For client requests, setting this field prevents re-use of
// TCP connections between requests to the same hosts, as if
// Transport.DisableKeepAlives were set.
Close bool
// For server requests Host specifies the host on which the
// URL is sought. Per RFC 2616, this is either the value of
// the "Host" header or the host name given in the URL itself.
// It may be of the form "host:port". For international domain
// names, Host may be in Punycode or Unicode form. Use
// golang.org/x/net/idna to convert it to either format if
// needed.
//
// For client requests Host optionally overrides the Host
// header to send. If empty, the Request.Write method uses
// the value of URL.Host. Host may contain an international
// domain name.
Host string
// Form contains the parsed form data, including both the URL
// field's query parameters and the POST or PUT form data.
// This field is only available after ParseForm is called.
// The HTTP client ignores Form and uses Body instead.
Form url.Values
// PostForm contains the parsed form data from POST, PATCH,
// or PUT body parameters.
//
// This field is only available after ParseForm is called.
// The HTTP client ignores PostForm and uses Body instead.
PostForm url.Values
// MultipartForm is the parsed multipart form, including file uploads.
// This field is only available after ParseMultipartForm is called.
// The HTTP client ignores MultipartForm and uses Body instead.
MultipartForm *multipart.Form
// Trailer specifies additional headers that are sent after the request
// body.
//
// For server requests the Trailer map initially contains only the
// trailer keys, with nil values. (The client declares which trailers it
// will later send.) While the handler is reading from Body, it must
// not reference Trailer. After reading from Body returns EOF, Trailer
// can be read again and will contain non-nil values, if they were sent
// by the client.
//
// For client requests Trailer must be initialized to a map containing
// the trailer keys to later send. The values may be nil or their final
// values. The ContentLength must be 0 or -1, to send a chunked request.
// After the HTTP request is sent the map values can be updated while
// the request body is read. Once the body returns EOF, the caller must
// not mutate Trailer.
//
// Few HTTP clients, servers, or proxies support HTTP trailers.
Trailer Header
// RemoteAddr allows HTTP servers and other software to record
// the network address that sent the request, usually for
// logging. This field is not filled in by ReadRequest and
// has no defined format. The HTTP server in this package
// sets RemoteAddr to an "IP:port" address before invoking a
// handler.
// This field is ignored by the HTTP client.
RemoteAddr string
// RequestURI is the unmodified Request-URI of the
// Request-Line (RFC 2616, Section 5.1) as sent by the client
// to a server. Usually the URL field should be used instead.
// It is an error to set this field in an HTTP client request.
RequestURI string
// TLS allows HTTP servers and other software to record
// information about the TLS connection on which the request
// was received. This field is not filled in by ReadRequest.
// The HTTP server in this package sets the field for
// TLS-enabled connections before invoking a handler;
// otherwise it leaves the field nil.
// This field is ignored by the HTTP client.
TLS *tls.ConnectionState
// Cancel is an optional channel whose closure indicates that the client
// request should be regarded as canceled. Not all implementations of
// RoundTripper may support Cancel.
//
// For server requests, this field is not applicable.
//
// Deprecated: Use the Context and WithContext methods
// instead. If a Request's Cancel field and context are both
// set, it is undefined whether Cancel is respected.
Cancel <-chan struct{}
// Response is the redirect response which caused this request
// to be created. This field is only populated during client
// redirects.
Response *Response
// contains filtered or unexported fields
}
func NewRequest(显示源文件)
func NewRequest(method, url string, body io.Reader) (*Request, error)
NewRequest返回给定方法,URL和可选主体的新请求。
如果提供的主体也是io.Closer,则返回的Request.Body将设置为body,并且将由客户端方法Do,Post和PostForm以及Transport.RoundTrip关闭。
NewRequest返回适用于Client.Do或Transport.RoundTrip的请求。要创建与测试服务器处理程序一起使用的请求,请使用net/http/httptest软件包中的NewRequest函数,使用ReadRequest或手动更新请求字段。有关入站和出站请求字段之间的差异,请参阅请求类型的文档。
如果body是* bytes.Buffer,* bytes.Reader或* strings.Reader类型,则返回的请求的ContentLength被设置为它的精确值(而不是-1),GetBody被填充(所以307和308重定向可以重放body),如果ContentLength为0,则Body设置为NoBody。
func ReadRequest(显示源文件)
func ReadRequest(b *bufio.Reader) (*Request, error)
ReadRequest读取并解析来自b的传入请求。
func (*Request) AddCookie(显示源文件)
func (r *Request) AddCookie(c *Cookie)
AddCookie向请求添加一个cookie。根据RFC 6265第5.4节的规定,AddCookie不会附加多个Cookie标题字段。这意味着所有的cookies(如果有的话)被写入同一行,并以分号分隔。
func (*Request) BasicAuth(显示源文件)
func (r *Request) BasicAuth() (username, password string, ok bool)
如果请求使用HTTP基本认证,BasicAuth将返回请求的授权标头中提供的用户名和密码。请参阅RFC 2617,第2部分。
func (*Request) Context(显示源文件)
func (r *Request) Context() context.Context
上下文返回请求的上下文。要更改上下文,请使用WithContext。
返回的上下文总是非零;它默认为后台上下文。
对于传出的客户端请求,上下文控制取消。
对于传入的服务器请求,当客户端连接关闭,取消请求(使用HTTP/2)或ServeHTTP方法返回时,上下文将被取消。
func (*Request) Cookie(显示源文件)
func (r *Request) Cookie(name string) (*Cookie, error)
Cookie返回请求中提供的命名cookie或ErrNoCookie,如果未找到。如果多个cookie匹配给定名称,则只会返回一个cookie。
func (*Request) Cookies(显示源文件)
func (r *Request) Cookies() []*Cookie
Cookies解析并返回随请求发送的HTTP Cookie。
func (*Request) FormFile(显示源文件)
func (r *Request) FormFile(key string) (multipart.File, *multipart.FileHeader, error)
FormFile返回提供的表单键的第一个文件。如有必要,FormFile调用ParseMultipartForm和ParseForm。
func (*Request) FormValue(显示源文件)
func (r *Request) FormValue(key string) string
FormValue返回查询命名组件的第一个值。POST和PUT正文参数优先于URL查询字符串值。如有必要,FormValue调用ParseMultipartForm和ParseForm,并忽略这些函数返回的任何错误。如果键不存在,FormValue返回空字符串。要访问同一个键的多个值,请调用ParseForm,然后直接检查Request.Form。
func (*Request) MultipartReader(显示源文件)
func (r *Request) MultipartReader() (*multipart.Reader, error)
如果这是multipart/form-data POST请求,则MultipartReader会返回MIME多部分阅读器,否则返回nil并显示错误。使用此函数而不是ParseMultipartForm将请求主体作为流处理。
func (*Request) ParseForm(显示源文件)
func (r *Request) ParseForm() error
ParseForm填充r.Form和r.PostForm。
对于所有请求,ParseForm解析来自URL的原始查询并更新r.Form。
对于POST,PUT和PATCH请求,它还将请求主体解析为表单并将结果放入r.PostForm和r.Form中。请求主体参数优先于r.Form中的URL查询字符串值。
对于其他HTTP方法,或者当Content-Type不是application/x-www-form-urlencoded时,请求体不会被读取,并且r.PostForm被初始化为非零空值。
如果请求体的大小尚未被MaxBytesReader限制,则大小限制为10MB。
ParseMultipartForm自动调用ParseForm。ParseForm是幂等的。
func (*Request) ParseMultipartForm(显示源文件)
func (r *Request) ParseMultipartForm(maxMemory int64) error
ParseMultipartForm将请求主体解析为multipart/form-data。对整个请求体进行解析,并将其文件部分的总共maxMemory字节存储在内存中,其余部分存储在磁盘中的临时文件中。如有必要,ParseMultipartForm会调用ParseForm。在一次调用ParseMultipartForm后,后续调用不起作用。
func (*Request) PostFormValue(显示源文件)
func (r *Request) PostFormValue(key string) string
PostFormValue返回POST或PUT请求主体的命名组件的第一个值。网址查询参数被忽略。如有必要,PostFormValue调用ParseMultipartForm和ParseForm,并忽略这些函数返回的任何错误。如果键不存在,PostFormValue返回空字符串。
func (*Request) ProtoAtLeast(显示源文件)
func (r *Request) ProtoAtLeast(major, minor int) bool
ProtoAtLeast报告请求中使用的HTTP协议是否至少major.minor。
func (*Request) Referer(显示源文件)
func (r *Request) Referer() string
如果在请求中发送,Referer返回引用URL。
引用者在请求本身中拼写错误,这是HTTP早期的错误。这个值也可以从Header映射中作为Header“Referer”获取; 将其作为一种方法提供的好处是,编译器可以诊断使用备用(正确的英文)拼写req.Referrer()的程序,但无法诊断使用Header“Referrer”的程序。
func (*Request) SetBasicAuth(显示源文件)
func (r *Request) SetBasicAuth(username, password string)
SetBasicAuth将请求的授权标头设置为使用带有所提供的用户名和密码的HTTP基本认证。
使用HTTP基本身份验证时,提供的用户名和密码未加密。
func (*Request) UserAgent(显示源文件)
func (r *Request) UserAgent() string
如果在请求中发送,UserAgent会返回客户端的用户代理。
func (*Request) WithContext(显示源文件)
func (r *Request) WithContext(ctx context.Context) *Request
WithContext返回r的浅表副本,其上下文已更改为ctx。提供的ctx必须是非零。
func (*Request) Write(显示源文件)
func (r *Request) Write(w io.Writer) error
写入有线格式的HTTP/1.1请求,这是标头和正文。该方法会查询请求的以下字段:
Host
URL
Method (defaults to "GET")
Header
ContentLength
TransferEncoding
Body
如果Body存在,则Content-Length <= 0且TransferEncoding尚未设置为“identity”,Write将“Transfer-Encoding: chunked”添加到标头。身体在发送后关闭。
func (*Request) WriteProxy(显示源文件)
func (r *Request) WriteProxy(w io.Writer) error
WriteProxy就像Write,但以HTTP代理的预期形式写入请求。特别是,WriteProxy按照RFC 2616的5.1.2节(包括方案和主机)以绝对URI写入请求的初始Request-URI行。无论哪种情况,WriteProxy还会使用r.Host或r.URL.Host写入一个主机头。
type Response(显示源文件)
响应表示来自HTTP请求的响应。
type Response struct {
Status string // e.g. "200 OK"
StatusCode int // e.g. 200
Proto string // e.g. "HTTP/1.0"
ProtoMajor int // e.g. 1
ProtoMinor int // e.g. 0
// Header maps header keys to values. If the response had multiple
// headers with the same key, they may be concatenated, with comma
// delimiters. (Section 4.2 of RFC 2616 requires that multiple headers
// be semantically equivalent to a comma-delimited sequence.) When
// Header values are duplicated by other fields in this struct (e.g.,
// ContentLength, TransferEncoding, Trailer), the field values are
// authoritative.
//
// Keys in the map are canonicalized (see CanonicalHeaderKey).
Header Header
// Body represents the response body.
//
// The http Client and Transport guarantee that Body is always
// non-nil, even on responses without a body or responses with
// a zero-length body. It is the caller's responsibility to
// close Body. The default HTTP client's Transport does not
// attempt to reuse HTTP/1.0 or HTTP/1.1 TCP connections
// ("keep-alive") unless the Body is read to completion and is
// closed.
//
// The Body is automatically dechunked if the server replied
// with a "chunked" Transfer-Encoding.
Body io.ReadCloser
// ContentLength records the length of the associated content. The
// value -1 indicates that the length is unknown. Unless Request.Method
// is "HEAD", values >= 0 indicate that the given number of bytes may
// be read from Body.
ContentLength int64
// Contains transfer encodings from outer-most to inner-most. Value is
// nil, means that "identity" encoding is used.
TransferEncoding []string
// Close records whether the header directed that the connection be
// closed after reading Body. The value is advice for clients: neither
// ReadResponse nor Response.Write ever closes a connection.
Close bool
// Uncompressed reports whether the response was sent compressed but
// was decompressed by the http package. When true, reading from
// Body yields the uncompressed content instead of the compressed
// content actually set from the server, ContentLength is set to -1,
// and the "Content-Length" and "Content-Encoding" fields are deleted
// from the responseHeader. To get the original response from
// the server, set Transport.DisableCompression to true.
Uncompressed bool
// Trailer maps trailer keys to values in the same
// format as Header.
//
// The Trailer initially contains only nil values, one for
// each key specified in the server's "Trailer" header
// value. Those values are not added to Header.
//
// Trailer must not be accessed concurrently with Read calls
// on the Body.
//
// After Body.Read has returned io.EOF, Trailer will contain
// any trailer values sent by the server.
Trailer Header
// Request is the request that was sent to obtain this Response.
// Request's Body is nil (having already been consumed).
// This is only populated for Client requests.
Request *Request
// TLS contains information about the TLS connection on which the
// response was received. It is nil for unencrypted responses.
// The pointer is shared between responses and should not be
// modified.
TLS *tls.ConnectionState
}
func GetSource
func Get(url string) (resp *Response, err error)
将问题GET获取到指定的URL。如果响应是以下重定向代码之一,则Get遵循重定向,最多可重定向10次:
301 (Moved Permanently)
302 (Found)
303 (See Other)
307 (Temporary Redirect)
308 (Permanent Redirect)
如果重定向太多或出现HTTP协议错误,则会返回错误。非2xx响应不会导致错误。
当err为零时,resp总是包含非零的resp.Body。来电者在完成阅读后应关闭resp.Body。
Get是一个DefaultClient.Get的包装器。
要使用自定义标题发出请求,请使用NewRequest和DefaultClient.Do。
示例
package main
import (
"fmt"
"io/ioutil"
"log"
"net/http"
)
func main() {
res, err := http.Get("http://www.google.com/robots.txt")
if err != nil {
log.Fatal(err)
}
robots, err := ioutil.ReadAll(res.Body)
res.Body.Close()
if err != nil {
log.Fatal(err)
}
fmt.Printf("%s", robots)
}
func Head(显示源文件)
func Head(url string) (resp *Response, err error)
Head向指定的URL发出HEAD。如果响应是以下重定向代码之一,Head会遵循重定向,最多可重定向10次:
301 (Moved Permanently)
302 (Found)
303 (See Other)
307 (Temporary Redirect)
308 (Permanent Redirect)
Head是DefaultClient.Head的包装
func Post(显示源文件)
func Post(url string, contentType string, body io.Reader) (resp *Response, err error)
发布POST到指定的URL。
调用者在完成阅读后应关闭resp.Body。
如果提供的主体是io.Closer,则在请求后关闭。
Post是DefaultClient.Post的一个包装。
要设置自定义标题,请使用NewRequest和DefaultClient.Do。
有关如何处理重定向的详细信息,请参阅Client.Do方法文档。
func PostForm(显示源文件)
func PostForm(url string, data url.Values) (resp *Response, err error)
PostForm向指定的URL发布POST,并将数据的键和值作为请求主体进行URL编码。
Content-Type头部设置为application/x-www-form-urlencoded。要设置其他标题,请使用NewRequest和DefaultClient.Do。
当err为零时,resp总是包含非零的resp.Body。调用者在完成阅读后应关闭resp.Body。
PostForm是DefaultClient.PostForm的封装。
有关如何处理重定向的详细信息,请参阅Client.Do方法文档。
func ReadResponse(显示源文件)
func ReadResponse(r *bufio.Reader, req *Request) (*Response, error)
ReadResponse读取并返回来自r的HTTP响应。req参数可选地指定对应于此响应的请求。如果为零,则假定有GET请求。读完resp.Body后,客户必须调用resp.Body.Close。通话结束后,客户可以检查resp.Trailer以查找响应预告片中包含的key/value pairs。
func (*Response) Cookies(显示源文件)
func (r *Response) Cookies() []*Cookie
Cookies分析并返回Set-Cookie标题中设置的Cookie。
func (*Response) Location(显示源文件)
func (r *Response) Location() (*url.URL, error)
位置返回响应的“位置”标题的URL(如果存在)。相对重定向相对于响应的请求被解析。如果不存在位置标题,则返回ErrNoLocation。
func (*Response) ProtoAtLeast(显示源文件)
func (r *Response) ProtoAtLeast(major, minor int) bool
ProtoAtLeast报告响应中使用的HTTP协议是否至少是major.minor。
func (*Response) Write(显示源文件)
func (r *Response) Write(w io.Writer) error
Write r以HTTP/1.x服务器响应格式写入w,包括状态行,标题,正文和可选的trailer。
该方法参考响应r的以下字段:
StatusCode
ProtoMajor
ProtoMinor
Request.Method
TransferEncoding
Trailer
Body
ContentLength
Header, values for non-canonical keys will have unpredictable behavior
响应主体在发送后关闭。
type ResponseWriter(显示源文件)
HTTP处理程序使用ResponseWriter接口来构造HTTP响应。
在Handler.ServeHTTP方法返回后,可能不会使用ResponseWriter。
type ResponseWriter interface {
// Header returns the header map that will be sent by
// WriteHeader. The Header map also is the mechanism with which
// Handlers can set HTTP trailers.
//
// Changing the header map after a call to WriteHeader (or
// Write) has no effect unless the modified headers are
// trailers.
//
// There are two ways to set Trailers. The preferred way is to
// predeclare in the headers which trailers you will later
// send by setting the "Trailer" header to the names of the
// trailer keys which will come later. In this case, those
// keys of the Header map are treated as if they were
// trailers. See the example. The second way, for trailer
// keys not known to the Handler until after the first Write,
// is to prefix the Header map keys with the TrailerPrefix
// constant value. See TrailerPrefix.
//
// To suppress implicit response headers (such as "Date"), set
// their value to nil.
Header() Header
// Write writes the data to the connection as part of an HTTP reply.
//
// If WriteHeader has not yet been called, Write calls
// WriteHeader(http.StatusOK) before writing the data. If the Header
// does not contain a Content-Type line, Write adds a Content-Type set
// to the result of passing the initial 512 bytes of written data to
// DetectContentType.
//
// Depending on the HTTP protocol version and the client, calling
// Write or WriteHeader may prevent future reads on the
// Request.Body. For HTTP/1.x requests, handlers should read any
// needed request body data before writing the response. Once the
// headers have been flushed (due to either an explicit Flusher.Flush
// call or writing enough data to trigger a flush), the request body
// may be unavailable. For HTTP/2 requests, the Go HTTP server permits
// handlers to continue to read the request body while concurrently
// writing the response. However, such behavior may not be supported
// by all HTTP/2 clients. Handlers should read before writing if
// possible to maximize compatibility.
Write([]byte) (int, error)
// WriteHeader sends an HTTP response header with status code.
// If WriteHeader is not called explicitly, the first call to Write
// will trigger an implicit WriteHeader(http.StatusOK).
// Thus explicit calls to WriteHeader are mainly used to
// send error codes.
WriteHeader(int)
}
示例(Trailers)
HTTP Trailers是一组key/value pairs,如HTTP响应之后的headers,而不是之前。
package main
import (
"io"
"net/http"
)
func main() {
mux := http.NewServeMux()
mux.HandleFunc("/sendstrailers", func(w http.ResponseWriter, req *http.Request) {
// Before any call to WriteHeader or Write, declare
// the trailers you will set during the HTTP
// response. These three headers are actually sent in
// the trailer.
w.Header().Set("Trailer", "AtEnd1, AtEnd2")
w.Header().Add("Trailer", "AtEnd3")
w.Header().Set("Content-Type", "text/plain; charset=utf-8") // normal header
w.WriteHeader(http.StatusOK)
w.Header().Set("AtEnd1", "value 1")
io.WriteString(w, "This HTTP response has both headers before this text and trailers at the end.\n")
w.Header().Set("AtEnd2", "value 2")
w.Header().Set("AtEnd3", "value 3") // These will appear as trailers.
})
}
type RoundTripper(显示源文件)
RoundTripper是一个接口,表示执行单个HTTP事务的能力,获得给定请求的响应。
RoundTripper必须是安全的,以供多个goroutine同时使用。
type RoundTripper interface {
// RoundTrip executes a single HTTP transaction, returning
// a Response for the provided Request.
//
// RoundTrip should not attempt to interpret the response. In
// particular, RoundTrip must return err == nil if it obtained
// a response, regardless of the response's HTTP status code.
// A non-nil err should be reserved for failure to obtain a
// response. Similarly, RoundTrip should not attempt to
// handle higher-level protocol details such as redirects,
// authentication, or cookies.
//
// RoundTrip should not modify the request, except for
// consuming and closing the Request's Body.
//
// RoundTrip must always close the body, including on errors,
// but depending on the implementation may do so in a separate
// goroutine even after RoundTrip returns. This means that
// callers wanting to reuse the body for subsequent requests
// must arrange to wait for the Close call before doing so.
//
// The Request's URL and Header fields must be initialized.
RoundTrip(*Request) (*Response, error)
}
DefaultTransport是Transport的默认实现,由DefaultClient使用。它根据需要建立网络连接,并将它们缓存以供随后的调用重用。它按照$ HTTP_PROXY和$ NO_PROXY(或$ http_proxy和$ no_proxy)环境变量的指示使用HTTP代理。
var DefaultTransport RoundTripper = &Transport{
Proxy: ProxyFromEnvironment,
DialContext: (&net.Dialer{
Timeout: 30 * time.Second,
KeepAlive: 30 * time.Second,
DualStack: true,
}).DialContext,
MaxIdleConns: 100,
IdleConnTimeout: 90 * time.Second,
TLSHandshakeTimeout: 10 * time.Second,
ExpectContinueTimeout: 1 * time.Second,
}
func NewFileTransport(显示源文件)
func NewFileTransport(fs FileSystem) RoundTripper
NewFileTransport返回一个新的RoundTripper,为所提供的FileSystem提供服务。返回的RoundTripper会忽略其传入请求中的URL主机以及请求的大多数其他属性。
NewFileTransport的典型用例是使用Transport注册“文件”协议,如下所示:
t := &http.Transport{}
t.RegisterProtocol("file", http.NewFileTransport(http.Dir("/")))
c := &http.Client{Transport: t}
res, err := c.Get("file:///etc/passwd")
...
type ServeMux(显示源文件)
ServeMux是一个HTTP请求多路复用器。它将每个传入请求的URL与注册模式列表进行匹配,并调用与该URL最匹配的模式的处理程序。
模式命名固定的,根源化的路径,如“/favicon.ico”,或根源子树,如“/images/”(注意尾部斜线)。较长的模式优先于较短的模式,因此,如果有处理程序注册了“/images/”和“/images / thumbnails/”,后一个处理程序将被调用以“/images/thumbnails/”开头的路径,前者将接收“/images/”子树中任何其他路径的请求。
请注意,由于以斜杠结尾的模式命名了一个有根的子树,因此模式“/”会匹配所有未被其他已注册模式匹配的路径,而不仅仅是具有Path ==“/”的URL。
如果一个子树已经注册并且接收到一个请求,并且命名了子树根而没有结尾的斜杠,ServeMux会将该请求重定向到子树根(添加尾部斜线)。此行为可以通过单独注册路径而不使用结尾斜杠来覆盖。例如,注册“/images/”会导致ServeMux将“/images”的请求重定向到“/images/”,除非单独注册了“/images”。
模式可以有选择地以主机名开头,只限制与主机上的URL匹配。特定于主机的模式优先于一般模式,因此处理程序可能会注册两种模式“/codesearch”和“codesearch.google.com/”,而不会接管“ http://www.google.com/”的请求”。
ServeMux还负责清理URL请求路径,重定向任何包含的请求。或..元素或重复的斜杠到一个等效的,更干净的URL。
type ServeMux struct {
// contains filtered or unexported fields
}
func NewServeMux(显示源文件)
func NewServeMux() *ServeMux
NewServeMux分配并返回一个新的ServeMux。
func (*ServeMux) Handle(显示源文件)
func (mux *ServeMux) Handle(pattern string, handler Handler)
Handle为给定模式注册处理程序。如果处理程序已经存在模式,则处理恐慌。
示例
代码:
mux := http.NewServeMux()
mux.Handle("/api/", apiHandler{})
mux.HandleFunc("/", func(w http.ResponseWriter, req *http.Request) {
// The "/" pattern matches everything, so we need to check
// that we're at the root here.
if req.URL.Path != "/" {
http.NotFound(w, req)
return
}
fmt.Fprintf(w, "Welcome to the home page!")
})
func (*ServeMux) HandleFunc(显示源文件)
func (mux *ServeMux) HandleFunc(pattern string, handler func(ResponseWriter, *Request))
HandleFunc为给定模式注册处理函数。
func (*ServeMux) Handler(显示源文件)
func (mux *ServeMux) Handler(r *Request) (h Handler, pattern string)
Handler返回用于给定请求的处理程序,请参阅r.Method,r.Host和r.URL.Path。它总是返回一个非零处理程序。如果路径不是其规范形式,则处理程序将是一个内部生成的处理程序,该处理程序将重定向到规范路径。如果主机包含端口,则在匹配处理程序时将忽略该端口。
CONNECT请求的路径和主机未改变。
处理程序还返回匹配请求的注册模式,或者在内部生成的重定向的情况下,返回跟随重定向后匹配的模式。
如果没有适用于请求的注册处理程序,则Handler返回“未找到页面”处理程序和空白模式。
func (*ServeMux) ServeHTTP(显示源文件)
func (mux *ServeMux) ServeHTTP(w ResponseWriter, r *Request)
ServeHTTP将请求分派给其模式与请求URL最匹配的处理程序。
type Server(显示源文件)
服务器定义运行HTTP服务器的参数。服务器的零值是有效的配置。
type Server struct {
Addr string // TCP address to listen on, ":http" if empty
Handler Handler // handler to invoke, http.DefaultServeMux if nil
TLSConfig *tls.Config // optional TLS config, used by ServeTLS and ListenAndServeTLS
// ReadTimeout is the maximum duration for reading the entire
// request, including the body.
//
// Because ReadTimeout does not let Handlers make per-request
// decisions on each request body's acceptable deadline or
// upload rate, most users will prefer to use
// ReadHeaderTimeout. It is valid to use them both.
ReadTimeout time.Duration
// ReadHeaderTimeout is the amount of time allowed to read
// request headers. The connection's read deadline is reset
// after reading the headers and the Handler can decide what
// is considered too slow for the body.
ReadHeaderTimeout time.Duration
// WriteTimeout is the maximum duration before timing out
// writes of the response. It is reset whenever a new
// request's header is read. Like ReadTimeout, it does not
// let Handlers make decisions on a per-request basis.
WriteTimeout time.Duration
// IdleTimeout is the maximum amount of time to wait for the
// next request when keep-alives are enabled. If IdleTimeout
// is zero, the value of ReadTimeout is used. If both are
// zero, ReadHeaderTimeout is used.
IdleTimeout time.Duration
// MaxHeaderBytes controls the maximum number of bytes the
// server will read parsing the request header's keys and
// values, including the request line. It does not limit the
// size of the request body.
// If zero, DefaultMaxHeaderBytes is used.
MaxHeaderBytes int
// TLSNextProto optionally specifies a function to take over
// ownership of the provided TLS connection when an NPN/ALPN
// protocol upgrade has occurred. The map key is the protocol
// name negotiated. The Handler argument should be used to
// handle HTTP requests and will initialize the Request's TLS
// and RemoteAddr if not already set. The connection is
// automatically closed when the function returns.
// If TLSNextProto is not nil, HTTP/2 support is not enabled
// automatically.
TLSNextProto map[string]func(*Server, *tls.Conn, Handler)
// ConnState specifies an optional callback function that is
// called when a client connection changes state. See the
// ConnState type and associated constants for details.
ConnState func(net.Conn, ConnState)
// ErrorLog specifies an optional logger for errors accepting
// connections and unexpected behavior from handlers.
// If nil, logging goes to os.Stderr via the log package's
// standard logger.
ErrorLog *log.Logger
// contains filtered or unexported fields
}
func (*Server) Close(显示源文件)
func (srv *Server) Close() error
立即关闭关闭状态StateNew,StateActive或StateIdle中的所有活动net.Listeners和任何连接。要正常关机,请使用关机。
关闭不会尝试关闭(甚至不知道)任何被劫持的连接,例如WebSockets。
Close返回关闭服务器底层侦听器返回的任何错误。
func (*Server) ListenAndServe(显示源文件)
func (srv *Server) ListenAndServe() error
ListenAndServe监听TCP网络地址srv.Addr,然后调用Serve处理传入连接上的请求。接受的连接被配置为启用TCP保持活动。如果srv.Addr为空,则使用“:http”。ListenAndServe总是返回一个非零错误。
func (*Server) ListenAndServeTLS(显示源文件)
func (srv *Server) ListenAndServeTLS(certFile, keyFile string) error
ListenAndServeTLS侦听TCP网络地址srv.Addr,然后调用Serve处理传入TLS连接上的请求。接受的连接被配置为启用TCP保持活动。
如果服务器的TLSConfig.Certificates和TLSConfig.GetCertificate都不填充,则必须提供包含服务器的证书和匹配私钥的文件名。如果证书由证书颁发机构签署,则certFile应该是服务器证书,任何中间器和CA证书的串联。
如果srv.Addr为空,则使用“:https”。
ListenAndServeTLS总是返回一个非零错误。
func (*Server) RegisterOnShutdown(显示源文件)
func (srv *Server) RegisterOnShutdown(f func())
RegisterOnShutdown注册一个函数来调用Shutdown。这可以用于正常关闭经过NPN/ALPN协议升级或已被劫持的连接。此功能应启动特定于协议的正常关机,但不应等待关机完成。
func (*Server) Serve(显示源文件)
func (srv *Server) Serve(l net.Listener) error
Serve接受Listener l上的传入连接,为每个服务器创建一个新的服务程序。服务程序读取请求,然后调用srv.Handler来回复它们。
对于HTTP/2支持,在调用Serve之前,应将srv.TLSConfig初始化为提供的侦听器的TLS配置。如果srv.TLSConfig非零,并且在Config.NextProtos中不包含字符串“h2”,则不启用HTTP/2支持。
始终返回非零错误。关机或关闭后,返回的错误是ErrServerClosed。
func (*Server) ServeTLS(显示源文件)
func (srv *Server) ServeTLS(l net.Listener, certFile, keyFile string) error
ServeTLS接受Listener l上的传入连接,为每个连接创建一个新的服务例程。服务程序读取请求,然后调用srv.Handler来回复它们。
此外,如果服务器的TLSConfig.Certificates和TLSConfig.GetCertificate都未被填充,则必须提供包含服务器的证书和匹配私钥的文件。如果证书由证书颁发机构签署,则certFile应该是服务器的串联证书,任何中间件和CA的证书。
对于HTTP/2支持,在调用Serve之前,应将srv.TLSConfig初始化为提供的侦听器的TLS配置。如果srv.TLSConfig非零,并且在Config.NextProtos中不包含字符串“h2”,则不启用HTTP/2支持。
ServeTLS总是返回一个非零错误。关机或关闭后,返回的错误是ErrServerClosed。
func (*Server) SetKeepAlivesEnabled(显示源文件)
func (srv *Server) SetKeepAlivesEnabled(v bool)
SetKeepAlivesEnabled控制是否启用HTTP保持活动。默认情况下,保持活动状态始终处于启用状态。在关闭过程中,只有非常资源受限的环境或服务器才能禁用它们。
func (*Server) Shutdown(显示源文件)
func (srv *Server) Shutdown(ctx context.Context) error
关机正常关闭服务器而不中断任何活动连接。首先关闭所有打开的监听程序,然后关闭所有空闲连接,然后无限期地等待连接返回到空闲状态然后关闭。如果提供的上下文在关闭完成之前到期,Shutdown将返回上下文的错误,否则返回关闭服务器的底层侦听器返回的任何错误。
当调用Shutdown时,Serve,ListenAndServe和ListenAndServeTLS立即返回ErrServerClosed。确保程序不会退出,而是等待Shutdown返回。
关机不会尝试关闭或等待被劫持的连接,如WebSockets。如果需要,Shutdown的调用者应该分别通知关闭的这种长期连接并等待它们关闭。
type Transport(显示源文件)
Transport是RoundTripper的一个实现,它支持HTTP,HTTPS和HTTP代理(对于使用CONNECT的HTTP或HTTPS)。
默认情况下,传输缓存连接以供将来重新使用。访问多台主机时可能会留下许多开放连接。可以使用传输的CloseIdleConnections方法和MaxIdleConnsPerHost和DisableKeepAlives字段管理此行为。
运输应该重用,而不是根据需要创建。运输对于多个goroutines并发使用是安全的。
传输是用于发出HTTP和HTTPS请求的低级原语。对于高级功能(如Cookie和重定向),请参阅客户端。
Transport对于HTTP URL使用HTTP/1.1,对于HTTPS URL使用HTTP/1.1或HTTP/2,具体取决于服务器是否支持HTTP/2以及如何配置Transport。DefaultTransport支持HTTP/2。要在传输上明确启用HTTP/2,请使用golang.org/x/net/http2并调用ConfigureTransport。有关HTTP/2的更多信息,请参阅软件包文档。
type Transport struct {
// Proxy specifies a function to return a proxy for a given
// Request. If the function returns a non-nil error, the
// request is aborted with the provided error.
//
// The proxy type is determined by the URL scheme. "http"
// and "socks5" are supported. If the scheme is empty,
// "http" is assumed.
//
// If Proxy is nil or returns a nil *URL, no proxy is used.
Proxy func(*Request) (*url.URL, error)
// DialContext specifies the dial function for creating unencrypted TCP connections.
// If DialContext is nil (and the deprecated Dial below is also nil),
// then the transport dials using package net.
DialContext func(ctx context.Context, network, addr string) (net.Conn, error)
// Dial specifies the dial function for creating unencrypted TCP connections.
//
// Deprecated: Use DialContext instead, which allows the transport
// to cancel dials as soon as they are no longer needed.
// If both are set, DialContext takes priority.
Dial func(network, addr string) (net.Conn, error)
// DialTLS specifies an optional dial function for creating
// TLS connections for non-proxied HTTPS requests.
//
// If DialTLS is nil, Dial and TLSClientConfig are used.
//
// If DialTLS is set, the Dial hook is not used for HTTPS
// requests and the TLSClientConfig and TLSHandshakeTimeout
// are ignored. The returned net.Conn is assumed to already be
// past the TLS handshake.
DialTLS func(network, addr string) (net.Conn, error)
// TLSClientConfig specifies the TLS configuration to use with
// tls.Client.
// If nil, the default configuration is used.
// If non-nil, HTTP/2 support may not be enabled by default.
TLSClientConfig *tls.Config
// TLSHandshakeTimeout specifies the maximum amount of time waiting to
// wait for a TLS handshake. Zero means no timeout.
TLSHandshakeTimeout time.Duration
// DisableKeepAlives, if true, prevents re-use of TCP connections
// between different HTTP requests.
DisableKeepAlives bool
// DisableCompression, if true, prevents the Transport from
// requesting compression with an "Accept-Encoding: gzip"
// request header when the Request contains no existing
// Accept-Encoding value. If the Transport requests gzip on
// its own and gets a gzipped response, it's transparently
// decoded in the Response.Body. However, if the user
// explicitly requested gzip it is not automatically
// uncompressed.
DisableCompression bool
// MaxIdleConns controls the maximum number of idle (keep-alive)
// connections across all hosts. Zero means no limit.
MaxIdleConns int
// MaxIdleConnsPerHost, if non-zero, controls the maximum idle
// (keep-alive) connections to keep per-host. If zero,
// DefaultMaxIdleConnsPerHost is used.
MaxIdleConnsPerHost int
// IdleConnTimeout is the maximum amount of time an idle
// (keep-alive) connection will remain idle before closing
// itself.
// Zero means no limit.
IdleConnTimeout time.Duration
// ResponseHeaderTimeout, if non-zero, specifies the amount of
// time to wait for a server's response headers after fully
// writing the request (including its body, if any). This
// time does not include the time to read the response body.
ResponseHeaderTimeout time.Duration
// ExpectContinueTimeout, if non-zero, specifies the amount of
// time to wait for a server's first response headers after fully
// writing the request headers if the request has an
// "Expect: 100-continue" header. Zero means no timeout and
// causes the body to be sent immediately, without
// waiting for the server to approve.
// This time does not include the time to send the request header.
ExpectContinueTimeout time.Duration
// TLSNextProto specifies how the Transport switches to an
// alternate protocol (such as HTTP/2) after a TLS NPN/ALPN
// protocol negotiation. If Transport dials an TLS connection
// with a non-empty protocol name and TLSNextProto contains a
// map entry for that key (such as "h2"), then the func is
// called with the request's authority (such as "example.com"
// or "example.com:1234") and the TLS connection. The function
// must return a RoundTripper that then handles the request.
// If TLSNextProto is not nil, HTTP/2 support is not enabled
// automatically.
TLSNextProto map[string]func(authority string, c *tls.Conn) RoundTripper
// ProxyConnectHeader optionally specifies headers to send to
// proxies during CONNECT requests.
ProxyConnectHeader Header
// MaxResponseHeaderBytes specifies a limit on how many
// response bytes are allowed in the server's response
// header.
//
// Zero means to use a default limit.
MaxResponseHeaderBytes int64
// contains filtered or unexported fields
}
func (*Transport) CancelRequest(显示源文件)
func (t *Transport) CancelRequest(req *Request)
CancelRequest通过关闭其连接来取消正在进行的请求。只有在RoundTrip返回后才能调用CancelRequest。
已弃用:使用Request.WithContext来创建具有可取消上下文的请求。CancelRequest无法取消HTTP/2请求。
func (*Transport) CloseIdleConnections(显示源文件)
func (t *Transport) CloseIdleConnections()
CloseIdleConnections关闭以前连接的所有连接,但是现在处于“保持活动”状态。它不会中断当前正在使用的任何连接。
func (*Transport) RegisterProtocol(显示源文件)
func (t *Transport) RegisterProtocol(scheme string, rt RoundTripper)
RegisterProtocol用方案注册一个新的协议。运输将使用给定的方案将请求传递给rt。模拟HTTP请求语义是rt的责任。
其他软件包可以使用RegisterProtocol来提供“ftp”或“file”等协议方案的实现。
如果rt.RoundTrip返回ErrSkipAltProtocol,Transport将为该请求处理RoundTrip本身,就好像该协议未注册一样。
func (*Transport) RoundTrip(显示源文件)
func (t *Transport) RoundTrip(req *Request) (*Response, error)
RoundTrip实现RoundTripper接口。
对于更高级别的HTTP客户端支持(如处理Cookie和重定向),请参阅获取,发布和客户端类型。
子目录
Name |
Synopsis |
---|---|
cgi |
cgi包实现了RFC 3875中规定的CGI(通用网关接口) |
cookiejar |
包cookiejar实现了符合内存RFC 6265的http.CookieJar。 |
fcgi |
fcgi包实现FastCGI协议。 |
httptest |
httptest包提供了用于HTTP测试的实用程序。 |
httptrace |
包httptrace提供跟踪HTTP客户端请求中的事件的机制。 |
httputil |
软件包httputil提供HTTP实用程序功能,补充了net/http软件包中较常见的功能。 |
pprof |
软件包pprof通过其HTTP服务器运行时分析数据以pprof可视化工具预期的格式提供服务。 |
net/http | ||
---|---|---|
net/http | 详细 | |
net/http/cgi | 详细 | |
net/http/cookiejar | 详细 | |
net/http/fcgi | 详细 | |
net/http/httptest | 详细 | |
net/http/httptrace | 详细 | |
net/http/httputil | 详细 | |
net/http/internal | 详细 | |
net/http/pprof | 详细 |
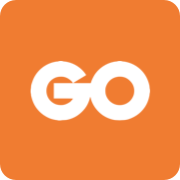
Go 是一种编译型语言,它结合了解释型语言的游刃有余,动态类型语言的开发效率,以及静态类型的安全性。它也打算成为现代的,支持网络与多核计算的语言。要满足这些目标,需要解决一些语言上的问题:一个富有表达能力但轻量级的类型系统,并发与垃圾回收机制,严格的依赖规范等等。这些无法通过库或工具解决好,因此Go也就应运而生了。
主页 | https://golang.org/ |
源码 | https://go.googlesource.com/go |
发布版本 | 1.9.2 |