Go参考手册
net/http
net/http/httptrace
import "net/http/httptrace"
- 概述
- 索引
- 示例
概述
httptrace包提供跟踪HTTP客户端请求中的事件的机制。
示例
package main
import (
"fmt"
"log"
"net/http"
"net/http/httptrace"
)
func main() {
req, _ := http.NewRequest("GET", "http://example.com", nil)
trace := &httptrace.ClientTrace{
GotConn: func(connInfo httptrace.GotConnInfo) {
fmt.Printf("Got Conn: %+v\n", connInfo)
},
DNSDone: func(dnsInfo httptrace.DNSDoneInfo) {
fmt.Printf("DNS Info: %+v\n", dnsInfo)
},
}
req = req.WithContext(httptrace.WithClientTrace(req.Context(), trace))
_, err := http.DefaultTransport.RoundTrip(req)
if err != nil {
log.Fatal(err)
}
}
索引
- func WithClientTrace(ctx context.Context, trace *ClientTrace) context.Context
- type ClientTrace
- func ContextClientTrace(ctx context.Context) *ClientTrace
- type DNSDoneInfo
- type DNSStartInfo
- type GotConnInfo
- type WroteRequestInfo
示例
包
文件包
trace.go
func WithClientTrace(显示源文件)
func WithClientTrace(ctx context.Context, trace *ClientTrace) context.Context
WithClientTrace根据提供的父ctx返回一个新的上下文。除了使用ctx注册的任何以前的挂钩外,使用返回的上下文创建的HTTP客户端请求将使用提供的跟踪挂钩。在提供的轨迹中定义的任何钩子将首先被调用。
type ClientTrace(显示源文件)
ClientTrace是一组挂钩,可以在传出的HTTP请求的各个阶段运行。任何特定的钩子可能是零。函数可以从不同的goroutine同时调用,有些可能在请求完成或失败后调用。
ClientTrace当前在单次往返过程中跟踪单个HTTP请求和响应,并且没有跨越一系列重定向请求的挂钩。
有关更多信息,请参阅https://blog.golang.org/http-tracing。
type ClientTrace struct {
// GetConn is called before a connection is created or
// retrieved from an idle pool. The hostPort is the
// "host:port" of the target or proxy. GetConn is called even
// if there's already an idle cached connection available.
GetConn func(hostPort string)
// GotConn is called after a successful connection is
// obtained. There is no hook for failure to obtain a
// connection; instead, use the error from
// Transport.RoundTrip.
GotConn func(GotConnInfo)
// PutIdleConn is called when the connection is returned to
// the idle pool. If err is nil, the connection was
// successfully returned to the idle pool. If err is non-nil,
// it describes why not. PutIdleConn is not called if
// connection reuse is disabled via Transport.DisableKeepAlives.
// PutIdleConn is called before the caller's Response.Body.Close
// call returns.
// For HTTP/2, this hook is not currently used.
PutIdleConn func(err error)
// GotFirstResponseByte is called when the first byte of the response
// headers is available.
GotFirstResponseByte func()
// Got100Continue is called if the server replies with a "100
// Continue" response.
Got100Continue func()
// DNSStart is called when a DNS lookup begins.
DNSStart func(DNSStartInfo)
// DNSDone is called when a DNS lookup ends.
DNSDone func(DNSDoneInfo)
// ConnectStart is called when a new connection's Dial begins.
// If net.Dialer.DualStack (IPv6 "Happy Eyeballs") support is
// enabled, this may be called multiple times.
ConnectStart func(network, addr string)
// ConnectDone is called when a new connection's Dial
// completes. The provided err indicates whether the
// connection completedly successfully.
// If net.Dialer.DualStack ("Happy Eyeballs") support is
// enabled, this may be called multiple times.
ConnectDone func(network, addr string, err error)
// TLSHandshakeStart is called when the TLS handshake is started. When
// connecting to a HTTPS site via a HTTP proxy, the handshake happens after
// the CONNECT request is processed by the proxy.
TLSHandshakeStart func()
// TLSHandshakeDone is called after the TLS handshake with either the
// successful handshake's connection state, or a non-nil error on handshake
// failure.
TLSHandshakeDone func(tls.ConnectionState, error)
// WroteHeaders is called after the Transport has written
// the request headers.
WroteHeaders func()
// Wait100Continue is called if the Request specified
// "Expected: 100-continue" and the Transport has written the
// request headers but is waiting for "100 Continue" from the
// server before writing the request body.
Wait100Continue func()
// WroteRequest is called with the result of writing the
// request and any body. It may be called multiple times
// in the case of retried requests.
WroteRequest func(WroteRequestInfo)
}
func ContextClientTrace(显示源文件)
func ContextClientTrace(ctx context.Context) *ClientTrace
ContextClientTrace返回与提供的上下文关联的ClientTrace。如果没有,则返回零。
type DNSDoneInfo(显示源文件)
DNSDoneInfo包含有关DNS查找结果的信息。
type DNSDoneInfo struct {
// Addrs are the IPv4 and/or IPv6 addresses found in the DNS
// lookup. The contents of the slice should not be mutated.
Addrs []net.IPAddr
// Err is any error that occurred during the DNS lookup.
Err error
// Coalesced is whether the Addrs were shared with another
// caller who was doing the same DNS lookup concurrently.
Coalesced bool
}
type DNSStartInfo(显示源文件)
DNSStartInfo包含有关DNS请求的信息。
type DNSStartInfo struct {
Host string
}
type GotConnInfo(显示源文件)
GotConnInfo是ClientTrace.GotConn函数的参数,并包含有关获取的连接的信息。
type GotConnInfo struct {
// Conn is the connection that was obtained. It is owned by
// the http.Transport and should not be read, written or
// closed by users of ClientTrace.
Conn net.Conn
// Reused is whether this connection has been previously
// used for another HTTP request.
Reused bool
// WasIdle is whether this connection was obtained from an
// idle pool.
WasIdle bool
// IdleTime reports how long the connection was previously
// idle, if WasIdle is true.
IdleTime time.Duration
}
type WroteRequestInfo(显示源文件)
WroteRequestInfo包含提供给WroteRequest钩子的信息。
type WroteRequestInfo struct {
// Err is any error encountered while writing the Request.
Err error
}
net/http相关
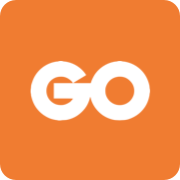
Go 是一种编译型语言,它结合了解释型语言的游刃有余,动态类型语言的开发效率,以及静态类型的安全性。它也打算成为现代的,支持网络与多核计算的语言。要满足这些目标,需要解决一些语言上的问题:一个富有表达能力但轻量级的类型系统,并发与垃圾回收机制,严格的依赖规范等等。这些无法通过库或工具解决好,因此Go也就应运而生了。
主页 | https://golang.org/ |
源码 | https://go.googlesource.com/go |
发布版本 | 1.9.2 |