Go参考手册
math/rand
math/rand
import "math/rand"
- 概述
- 索引
- 示例
概述
rand 包 执行伪随机数生成器。
随机数由一个 Source 生成。顶级函数(如 Float64 和 Int )使用默认的共享源,每次运行程序时都会产生确定性的值序列。如果每次运行都需要不同的行为,请使用 Seed 函数初始化默认 Source。默认的 Source 对于多个 goroutine 并发使用是安全的,但由 NewSource 创建的Source不是。
对于适合安全敏感工作的随机数字,请参阅 crypto / rand 软件包。
示例
package main
import (
"fmt"
"math/rand"
)
func main() {
rand.Seed(42) // 试着改变这些数字
answers := []string{
"It is certain",
"It is decidedly so",
"Without a doubt",
"Yes definitely",
"You may rely on it",
"As I see it yes",
"Most likely",
"Outlook good",
"Yes",
"Signs point to yes",
"Reply hazy try again",
"Ask again later",
"Better not tell you now",
"Cannot predict now",
"Concentrate and ask again",
"Don't count on it",
"My reply is no",
"My sources say no",
"Outlook not so good",
"Very doubtful",
}
fmt.Println("Magic 8-Ball says:", answers[rand.Intn(len(answers))])
}
示例(Rand)
此示例显示* Rand上每种方法的使用。全局函数的使用是一样的,没有接收器。
package main
import (
"fmt"
"math/rand"
"os"
"text/tabwriter"
)
func main() {
// 创造并设置生成器.
// 通常应该使用非固定种子, 如time.Now().UnixNano().
// 使用固定的种子挥在每次运行中产生相同的输出
r := rand.New(rand.NewSource(99))
//这里的tabwriter帮助我们生成对齐的输出。
w := tabwriter.NewWriter(os.Stdout, 1, 1, 1, ' ', 0)
defer w.Flush()
show := func(name string, v1, v2, v3 interface{}) {
fmt.Fprintf(w, "%s\t%v\t%v\t%v\n", name, v1, v2, v3)
}
// Float32 和Float64 的值在 [0, 1)之中.
show("Float32", r.Float32(), r.Float32(), r.Float32())
show("Float64", r.Float64(), r.Float64(), r.Float64())
// ExpFloat64值的平均值为1,但是呈指数衰减。
show("ExpFloat64", r.ExpFloat64(), r.ExpFloat64(), r.ExpFloat64())
// NormFloat64值的平均值为0,标准差为1。
show("NormFloat64", r.NormFloat64(), r.NormFloat64(), r.NormFloat64())
// Int31,Int63和Uint32生成给定宽度的值。
// Int方法(未显示)类似于Int31或Int63
//取决于'int'的大小。
show("Int31", r.Int31(), r.Int31(), r.Int31())
show("Int63", r.Int63(), r.Int63(), r.Int63())
show("Uint32", r.Uint32(), r.Uint32(), r.Uint32())
// Intn,Int31n和Int63n将其输出限制为<n。
//他们比使用r.Int()%n更谨慎。
show("Intn(10)", r.Intn(10), r.Intn(10), r.Intn(10))
show("Int31n(10)", r.Int31n(10), r.Int31n(10), r.Int31n(10))
show("Int63n(10)", r.Int63n(10), r.Int63n(10), r.Int63n(10))
// Perm生成数字[0,n]的随机排列。
show("Perm", r.Perm(5), r.Perm(5), r.Perm(5))
}
索引
- func ExpFloat64() float64
- func Float32() float32
- func Float64() float64
- func Int() int
- func Int31() int32
- func Int31n(n int32) int32
- func Int63() int64
- func Int63n(n int64) int64
- func Intn(n int) int
- func NormFloat64() float64
- func Perm(n int) []int
- func Read(p []byte) (n int, err error)
- func Seed(seed int64)
- func Uint32() uint32
- func Uint64() uint64
- type Rand
- func New(src Source) *Rand
- func (r *Rand) ExpFloat64() float64
- func (r *Rand) Float32() float32
- func (r *Rand) Float64() float64
- func (r *Rand) Int() int
- func (r *Rand) Int31() int32
- func (r *Rand) Int31n(n int32) int32
- func (r *Rand) Int63() int64
- func (r *Rand) Int63n(n int64) int64
- func (r *Rand) Intn(n int) int
- func (r *Rand) NormFloat64() float64
- func (r *Rand) Perm(n int) []int
- func (r *Rand) Read(p []byte) (n int, err error)
- func (r *Rand) Seed(seed int64)
- func (r *Rand) Uint32() uint32
- func (r *Rand) Uint64() uint64
- type Source
- func NewSource(seed int64) Source
- type Source64
- type Zipf
- func NewZipf(r *Rand, s float64, v float64, imax uint64) *Zipf
- func (z *Zipf) Uint64() uint64
示例
Package Perm Package (Rand)
打包文件
exp.go normal.go rand.go rng.go zipf.go
func ExpFloat64(查看源文件)
func ExpFloat64() float64
ExpFloat64 返回指数分布的 float64 ,其范围为 (0, +math.MaxFloat64],其指数分布的速率参数(lambda)为1,平均值为默认 Source 的 1 / lambda(1)。不同的速率参数,调用者可以使用以下方式调整输出:
sample = ExpFloat64() / desiredRateParameter
func Float32(查看源文件)
func Float32() float32
Float32 以默认 Source 的形式返回一个作为 float32 的 [0.0,1.0) 中的伪随机数。
func Float64(查看源文件)
func Float64() float64
Float64 以默认 Source 的形式返回,作为 float64,一个 [0.0,1.0) 中的伪随机数字。
func Int(查看源文件)
func Int() int
Int 从默认的 Source 返回一个非负的伪随机 int。
func Int31(查看源文件)
func Int31() int32
Int31 从默认 Source 返回一个非负的伪随机31位整数作为 int32。
func Int31n(查看源文件)
func Int31n(n int32) int32
Int31n 以默认 Source 的形式返回 [0,n)中的非负伪随机数,作为 int32。如果n <= 0,它会发生混乱。
func Int63(查看源文件)
func Int63() int64
Int63 从默认的 Source 返回一个非负的伪随机的63位整数作为 int64。
func Int63n(查看源文件)
func Int63n(n int64) int64
Int63n 以 int64 形式返回来自默认 Source 的 [0,n] 中的非负伪随机数。如果n <= 0,它会发生混乱。
func Intn(查看源文件)
func Intn(n int) int
Intn 以 int 形式返回来自默认 Source 的 [0,n] 中的非负伪随机数。如果 n <= 0,它会发生混乱。
func NormFloat64(查看源文件)
func NormFloat64() float64
NormFloat64 从默认的 Source 返回正态分布的 float64,范围为-math.MaxFloat64,+ math.MaxFloat64,标准正态分布(mean = 0,stddev = 1)。要产生不同的正态分布,调用者可以使用以下方式调整输出:
sample = NormFloat64() * desiredStdDev + desiredMean
func Perm(查看源文件)
func Perm(n int) []int
Perm 以默认 Source 的形式返回整数 [0,n) 的伪随机置换。
示例
package main
import (
"fmt"
"math/rand"
)
func main() {
for _, value := range rand.Perm(3) {
fmt.Println(value)
}
}
func Read(查看源文件)
func Read(p []byte) (n int, err error)
Read 从默认 Source 生成 len(p) 个随机字节,并将它们写入 p。它总是返回 len(p) 和一个空的错误。与 Rand.Read 方法不同,读取对于并发使用是安全的。
func Seed(查看源文件)
func Seed(seed int64)
Seed 使用提供的种子值将默认 Source 初始化为确定性状态。如果种子没有被调用,那么生成器的行为就像种子(1)播种一样。具有相同余数的种子值除以2 ^ 31-1会生成相同的伪随机序列。与 Rand.Seed 方法不同,种子对于并发使用是安全的。
func Uint32Source
func Uint32() uint32
Uint32从默认的 Source 返回一个伪随机的32位值作为 uint32。
func Uint64Source
func Uint64() uint64
Uint64 从默认的 Source 返回一个伪随机的64位值作为 uint64。
type RandSource
Rand 是随机数字的来源。
type Rand struct {
// contains filtered or unexported fields
}
func NewSource
func New(src Source) *Rand
New 返回一个新的Rand,它使用 src 中的随机值生成其他随机值。
func (*Rand) ExpFloat64Source
func (r *Rand) ExpFloat64() float64
ExpFloat64 返回指数分布的 float64,其范围为 (0, +math.MaxFloat64] ,其指数分布的速率参数(lambda)为1,平均值为 1 / lambda(1)。为了生成具有不同速率参数的分布,呼叫者可以使用以下方式调整输出:
sample = ExpFloat64() / desiredRateParameter
func (*Rand) Float32Source
func (r *Rand) Float32() float32
Float32 作为 float32 返回 [0.0,1.0) 中的伪随机数。
func (*Rand) Float64Source
func (r *Rand) Float64() float64
Float64 作为 float64 返回 [0.0,1.0) 中的伪随机数。
func (*Rand) IntSource
func (r *Rand) Int() int
Int 返回一个非负的伪随机 int。
func (*Rand) Int31Source
func (r *Rand) Int31() int32
Int31 以 int32 形式返回一个非负的伪随机31位整数。
func (*Rand) Int31nSource
func (r *Rand) Int31n(n int32) int32
Int31n 以 int32 形式返回 [0,n)中的非负伪随机数。如果 n <= 0,它会发生混乱。
func (*Rand) Int63Source
func (r *Rand) Int63() int64
Int63 以 int64 的形式返回一个非负的伪随机63位整数。
func (*Rand) Int63nSource
func (r *Rand) Int63n(n int64) int64
Int63n 以 int64 的形式返回 [0,n)中的非负伪随机数。如果 n <= 0,它会发生混乱。
func (*Rand) IntnSource
func (r *Rand) Intn(n int) int
Intn 返回 int [0,n] 中的非负伪随机数。如果 n <= 0,它会发生混乱。
func (*Rand) NormFloat64Source
func (r *Rand) NormFloat64() float64
NormFloat64 返回正态分布的 float64,范围为-math.MaxFloat64,+ math.MaxFloat64,标准正态分布(mean = 0,stddev = 1)。要产生不同的正态分布,呼叫者可以使用以下方式调整输出:
sample = NormFloat64() * desiredStdDev + desiredMean
func (*Rand) PermSource
func (r *Rand) Perm(n int) []int
Perm作为一个 n 分片返回一个整数 [0,n)的伪随机置换。
func (*Rand) ReadSource
func (r *Rand) Read(p []byte) (n int, err error)
Read 生成 len(p)个随机字节并将它们写入p。它总是返回 len(p)和一个零错误。阅读不应与任何其他 Rand 方法同时调用。
func (*Rand) SeedSource
func (r *Rand) Seed(seed int64)
Seed 使用提供的种子值将发生器初始化为确定性状态。Seed 不应该与任何其他 Rand 方法同时调用。
func (*Rand) Uint32Source
func (r *Rand) Uint32() uint32
Uint32 返回一个伪随机的32位值作为 uint32。
func (*Rand) Uint64Source
func (r *Rand) Uint64() uint64
Uint64 返回一个伪随机的64位值作为 uint64。
type SourceSource
源表示均匀分布的伪随机 int64 值的来源,范围 [0,1 << 63)。
type Source interface {
Int63() int64
Seed(seed int64)
}
func NewSourceSource
func NewSource(seed int64) Source
NewSource 返回一个新的伪随机源,并给定值。与顶级函数使用的默认源不同,此源对于多个 goroutine 并发使用并不安全。
type Source64Source
Source64是一个Source,它也可以直接在[0,1 << 64)范围内生成均匀分布的伪随机uint64值。如果 Rand r 的底层 Source 实现Source64,则 r.Uint64 将一次调用的结果返回给 s.Uint64,而不是对 s.Int63进行两次调用。
type Source64 interface {
Source
Uint64() uint64
}
type ZipfSource
Zipf 生成 Zipf 分布式变量。
type Zipf struct {
// contains filtered or unexported fields
}
func NewZipfSource
func NewZipf(r *Rand, s float64, v float64, imax uint64) *Zipf
NewZipf 返回一个 Zipf 变量生成器。生成器生成值 k∈0,imax,使得P(k)与(v + k)**(-s)成比例。要求:s> 1且v> = 1。
func (*Zipf) Uint64Source
func (z *Zipf) Uint64() uint64
Uint64 返回从 Zipf 对象描述的 Zipf 分布中绘制的值。
math/rand | ||
---|---|---|
math/rand | 详细 |
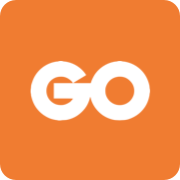
Go 是一种编译型语言,它结合了解释型语言的游刃有余,动态类型语言的开发效率,以及静态类型的安全性。它也打算成为现代的,支持网络与多核计算的语言。要满足这些目标,需要解决一些语言上的问题:一个富有表达能力但轻量级的类型系统,并发与垃圾回收机制,严格的依赖规范等等。这些无法通过库或工具解决好,因此Go也就应运而生了。
主页 | https://golang.org/ |
源码 | https://go.googlesource.com/go |
发布版本 | 1.9.2 |