Go参考手册
测试 | testing
测试 | testing
import "testing"
- 概观
- 索引
- 示例
- 子目录
概观
软件包测试为 Go 软件包的自动化测试提供支持。它旨在与“go test”命令配合使用,该命令可自动执行表单的任何功能
func TestXxx(*testing.T)
其中 Xxx 可以是任何字母数字字符串(但第一个字母不能在 a-z 中)并用于标识测试例程。
在这些功能中,使用错误,失败或相关方法发送失败信号。
要编写一个新的测试套件,请创建一个名称以 _test.go 结尾的文件,其中包含 TestXxx 函数,如此处所述。将该文件放在与正在测试的文件相同的包中。该文件将从常规软件包版本中排除,但会在运行“go test”命令时包含该文件。有关更多详细信息,请运行“go help test”和“go help testflag”。
如果对 * T 和 * B 的 Skip 方法的调用不适用,测试和基准可能会被跳过:
func TestTimeConsuming(t *testing.T) {
if testing.Short() {
t.Skip("skipping test in short mode.")
}
...
}
基准
表单的功能
func BenchmarkXxx(*testing.B)
被认为是基准测试,并且当它的 -bench 标志被提供时由“go test”命令执行。基准按顺序运行。
有关测试标志的说明,请参阅 https://golang.org/cmd/go/#hdr-Description_of_testing_flags。
示例基准函数如下所示:
func BenchmarkHello(b *testing.B) {
for i := 0; i < b.N; i++ {
fmt.Sprintf("hello")
}
}
基准函数必须运行目标代码 b.N 倍。在执行基准测试期间,将调整 b.N,直到基准测试功能持续足够长时间以可靠定时。输出
BenchmarkHello 10000000 282 ns/op
意味着循环以每个循环 282 ns 的速度运行10000000次。
如果基准测试在运行之前需要昂贵的设置,则计时器可能会重置:
func BenchmarkBigLen(b *testing.B) {
big := NewBig()
b.ResetTimer()
for i := 0; i < b.N; i++ {
big.Len()
}
}
如果基准测试需要在并行设置中测试性能,则可以使用 RunParalle l帮助函数; 这样的基准测试旨在与 go 测试 -cpu 标志一起使用:
func BenchmarkTemplateParallel(b *testing.B) {
templ := template.Must(template.New("test").Parse("Hello, {{.}}!"))
b.RunParallel(func(pb *testing.PB) {
var buf bytes.Buffer
for pb.Next() {
buf.Reset()
templ.Execute(&buf, "World")
}
})
}
示例
该软件包还运行并验证示例代码。示例函数可能包含以“Output:”开头的结束语注释,并在运行测试时与函数的标准输出进行比较。(比较忽略前导空间和尾随空间。)以下是一个示例的示例:
func ExampleHello() {
fmt.Println("hello")
// Output: hello
}
func ExampleSalutations() {
fmt.Println("hello, and")
fmt.Println("goodbye")
// Output:
// hello, and
// goodbye
}
注释前缀“Unordered output:”与“Output:”类似,但匹配任何行顺序:
func ExamplePerm() {
for _, value := range Perm(4) {
fmt.Println(value)
}
// Unordered output: 4
// 2
// 1
// 3
// 0
}
没有输出注释的示例函数被编译但不被执行。
声明该包的示例的命名约定,类型 T 上的函数 F,类型 T 和方法 M 是:
func Example() { ... }
func ExampleF() { ... }
func ExampleT() { ... }
func ExampleT_M() { ... }
可以通过为名称添加一个不同的后缀来提供包/类型/函数/方法的多个示例函数。后缀必须以小写字母开头。
func Example_suffix() { ... }
func ExampleF_suffix() { ... }
func ExampleT_suffix() { ... }
func ExampleT_M_suffix() { ... }
当整个测试文件包含单个示例函数,至少一个其他函数,类型,变量或常量声明,并且没有测试或基准测试函数时,将以整个测试文件为例。
Subtests and Sub-benchmarks
T 和 B 的 Run 方法允许定义子测试和子基准,而不必为每个测试定义单独的功能。这使得像表驱动的基准测试和创建分层测试成为可能。它还提供了一种共享通用设置和拆卸代码的方法:
func TestFoo(t *testing.T) {
// <setup code>
t.Run("A=1", func(t *testing.T) { ... })
t.Run("A=2", func(t *testing.T) { ... })
t.Run("B=1", func(t *testing.T) { ... })
// <tear-down code>
}
每个子测试和子基准具有唯一的名称:顶级测试的名称和传递给 Run 的名称序列的组合,用斜杠分隔,并带有可选的尾部序列号以消除歧义。
-run 和 -bench 命令行标志的参数是与测试名称匹配的未锚定正则表达式。对于具有多个斜杠分隔元素(例如子测试)的测试,参数本身是斜线分隔的,表达式依次匹配每个名称元素。由于它是未锚定的,因此一个空的表达式匹配任何字符串。例如,使用“匹配”来表示“其名称包含”:
go test -run '' # Run all tests.
go test -run Foo # Run top-level tests matching "Foo", such as "TestFooBar".
go test -run Foo/A= # For top-level tests matching "Foo", run subtests matching "A=".
go test -run /A=1 # For all top-level tests, run subtests matching "A=1".
子测试也可以用来控制并行性。家长测试只有在完成所有分测验后才能完成。在这个例子中,所有的测试都是相互平行的,并且只与对方一起运行,而不管可能定义的其他顶级测试:
func TestGroupedParallel(t *testing.T) {
for _, tc := range tests {
tc := tc // capture range variable
t.Run(tc.Name, func(t *testing.T) {
t.Parallel()
...
})
}
}
运行直到并行子测试完成才会返回,这提供了一种在一组并行测试后进行清理的方法:
func TestTeardownParallel(t *testing.T) {
// This Run will not return until the parallel tests finish.
t.Run("group", func(t *testing.T) {
t.Run("Test1", parallelTest1)
t.Run("Test2", parallelTest2)
t.Run("Test3", parallelTest3)
})
// <tear-down code>
}
Main
测试程序有时需要在测试之前或之后进行额外的设置或拆卸。测试有时还需要控制在主线程上运行哪些代码。为了支持这些和其他情况,如果测试文件包含一个函数:
func TestMain(m *testing.M)
那么生成的测试将调用 TestMain(m) ,而不是直接运行测试。 TestMain 在主要的例程中运行,并且可以在 m.Run 的调用周围进行任何设置和拆卸。然后它应该用 m.Run 的结果调用 os.Exit 。当调用 TestMain 时, flag.Parse 尚未运行。如果 TestMain 依赖于命令行标志,包括那些测试包,它应该明确调用 flag.Parse。
A simple implementation of TestMain is:
func TestMain(m *testing.M) {
// call flag.Parse() here if TestMain uses flags
os.Exit(m.Run())
}
索引
- func AllocsPerRun(runs int, f func()) (avg float64)
- func CoverMode() string
- func Coverage() float64
- func Main(matchString func(pat, str string) (bool, error), tests []InternalTest, benchmarks []InternalBenchmark, examples []InternalExample)
- func RegisterCover(c Cover)
- func RunBenchmarks(matchString func(pat, str string) (bool, error), benchmarks []InternalBenchmark)
- func RunExamples(matchString func(pat, str string) (bool, error), examples []InternalExample) (ok bool)
- func RunTests(matchString func(pat, str string) (bool, error), tests []InternalTest) (ok bool)
- func Short() bool
- func Verbose() bool
- type B
- func (c *B) Error(args ...interface{})
- func (c *B) Errorf(format string, args ...interface{})
- func (c *B) Fail()
- func (c *B) FailNow()
- func (c *B) Failed() bool
- func (c *B) Fatal(args ...interface{})
- func (c *B) Fatalf(format string, args ...interface{})
- func (c *B) Helper()
- func (c *B) Log(args ...interface{})
- func (c *B) Logf(format string, args ...interface{})
- func (c *B) Name() string
- func (b *B) ReportAllocs()
- func (b *B) ResetTimer()
- func (b *B) Run(name string, f func(b *B)) bool
- func (b *B) RunParallel(body func(*PB))
- func (b *B) SetBytes(n int64)
- func (b *B) SetParallelism(p int)
- func (c *B) Skip(args ...interface{})
- func (c *B) SkipNow()
- func (c *B) Skipf(format string, args ...interface{})
- func (c *B) Skipped() bool
- func (b *B) StartTimer()
- func (b *B) StopTimer()
- type BenchmarkResult
- func Benchmark(f func(b *B)) BenchmarkResult
- func (r BenchmarkResult) AllocedBytesPerOp() int64
- func (r BenchmarkResult) AllocsPerOp() int64
- func (r BenchmarkResult) MemString() string
- func (r BenchmarkResult) NsPerOp() int64
- func (r BenchmarkResult) String() string
- type Cover
- type CoverBlock
- type InternalBenchmark
- type InternalExample
- type InternalTest
- type M
- func MainStart(deps testDeps, tests []InternalTest, benchmarks []InternalBenchmark, examples []InternalExample) *M
- func (m *M) Run() int
- type PB
- func (pb *PB) Next() bool
- type T
- func (c *T) Error(args ...interface{})
- func (c *T) Errorf(format string, args ...interface{})
- func (c *T) Fail()
- func (c *T) FailNow()
- func (c *T) Failed() bool
- func (c *T) Fatal(args ...interface{})
- func (c *T) Fatalf(format string, args ...interface{})
- func (c *T) Helper()
- func (c *T) Log(args ...interface{})
- func (c *T) Logf(format string, args ...interface{})
- func (c *T) Name() string
- func (t *T) Parallel()
- func (t *T) Run(name string, f func(t *T)) bool
- func (c *T) Skip(args ...interface{})
- func (c *T) SkipNow()
- func (c *T) Skipf(format string, args ...interface{})
- func (c *T) Skipped() bool
- type TB
示例
B.RunParallel
打包文件
allocs.go benchmark.go cover.go example.go match.go testing.go
func AllocsPerRunSource
func AllocsPerRun(runs int, f func()) (avg float64)
AllocsPerRun 返回f期间的平均分配数量。尽管返回值的类型为 float64 ,但它始终是一个整数值。
要计算分配数量,函数将首先作为热身运行一次。然后测量并返回指定运行次数内的平均分配数量。
AllocsPerRun 在测量过程中将 GOMAXPROCS 设置为1,并在返回之前将其恢复。
func CoverModeSource
func CoverMode() string
CoverMode 报告测试覆盖模式的设置。值为“set”,“count”或“atomic”。如果测试覆盖未启用,返回值将为空。
func CoverageSource
func Coverage() float64
Coverage 将当前代码覆盖范围报告为范围0,1中的一个分数。如果未启用 Coverage ,则 Coverage 返回0。
当运行大量顺序测试用例时,在每个测试用例之后检查 Coverage 对于识别哪些测试用例可以使用新代码路径很有用。它不会替代 'go test -cover' 和 'go tool cover' 生成的报告。
func MainSource
func Main(matchString func(pat, str string) (bool, error), tests []InternalTest, benchmarks []InternalBenchmark, examples []InternalExample)
Main 是一个内部函数,是执行“go test”命令的一部分。它被出口,因为它是交叉包装并且早于“内部”包装。它不再被“去测试”使用,而是尽可能地保留在模拟使用Main进行“测试”的其他系统上,但是由于新功能被添加到测试包中,Main有时无法更新。模拟“go test”的系统应该更新为使用 MainStart。
func RegisterCoverSource
func RegisterCover(c Cover)
RegisterCover 记录测试的覆盖率数据累加器。注意:此功能是测试基础架构的内部功能,可能会更改。Go 1 兼容性指南并未涵盖此内容。
func RunBenchmarksSource
func RunBenchmarks(matchString func(pat, str string) (bool, error), benchmarks []InternalBenchmark)
内部函数,因为它是交叉包装而被导出;部分执行“go test”命令。
func RunExamplesSource
func RunExamples(matchString func(pat, str string) (bool, error), examples []InternalExample) (ok bool)
内部函数,因为它是交叉包装而被导出;部分执行“go test”命令。
func RunTestsSource
func RunTests(matchString func(pat, str string) (bool, error), tests []InternalTest) (ok bool)
内部函数,因为它是交叉包装而被导出;部分执行“go test”命令。
func ShortSource
func Short() bool
Short 报告是否设置了 -test.short 标志。
func VerboseSource
func Verbose() bool
详细报告是否设置了 -test.v 标志。
type BSource
B 是一种传递给 Benchmark 函数的类型,用于管理基准计时并指定要运行的迭代次数。
当 Benchmark 函数返回或调用 FailNow,Fatal,Fatalf,SkipNow,Skip 或 Skipf 方法时,基准结束。这些方法只能从运行 Benchmark 函数的 goroutine 中调用。其他报告方法(如 Log 和 Error 的变体)可以从多个 goroutines 同时调用。
就像在测试中一样,基准测试日志在执行过程中会累积并在完成时转储到标准错误。与测试不同,基准测试日志始终打印出来,以免隐藏存在可能影响基准测试结果的输出。
type B struct {
N int
// contains filtered or unexported fields
}
func (*B) ErrorSource
func (c *B) Error(args ...interface{})
错误等同于 Log ,然后是 Fail 。
func (*B) ErrorfSource
func (c *B) Errorf(format string, args ...interface{})
Errorf 等同于 Logf ,然后是 Fail 。
func (*B) FailSource
func (c *B) Fail()
Fail 会将该功能标记为失败,但会继续执行。
func (*B) FailNowSource
func (c *B) FailNow()
FailNow 将该函数标记为失败并停止其执行。执行将继续在下一个测试或基准。必须从运行测试或基准测试函数的 goroutine 调用 FailNow ,而不是在测试期间创建的其他 goutoutine 调用。调用 FailNow 不会停止那些其他的 goroutine 。
func (*B) FailedSource
func (c *B) Failed() bool
Failed 报告功能是否失败。
func (*B) FatalSource
func (c *B) Fatal(args ...interface{})
Fatal 相当于 Log ,然后是 FailNow 。
func (*B) FatalfSource
func (c *B) Fatalf(format string, args ...interface{})
Fatalf 等同于 Logf,然后是 FailNow。
func (*B) HelperSource
func (c *B) Helper()
助手将调用函数标记为测试帮助函数。打印文件和行信息时,该功能将被跳过。可以从多个 goroutines 同时调用助手。如果 Heller 从 TestXxx / BenchmarkXxx 函数或子测试/次级基准测试函数直接调用,则 Helper 不起作用。
func (*B) LogSource
func (c *B) Log(args ...interface{})
日志使用默认格式化格式化其参数,类似于 Println ,并将文本记录在错误日志中。对于测试,仅当测试失败或设置了 -test.v 标志时才会打印文本。对于基准测试,总是打印文本以避免性能取决于 -test.v 标志的值。
func (*B) LogfSource
func (c *B) Logf(format string, args ...interface{})
Logf 根据格式格式化其参数,类似于 Printf ,并将文本记录在错误日志中。如果没有提供,最后换行符会被添加。对于测试,仅当测试失败或设置了 -test.v 标志时才会打印文本。对于基准测试,总是打印文本以避免性能取决于 -test.v 标志的值。
func (*B) NameSource
func (c *B) Name() string
Name 返回正在运行的测试或基准的名称。
func (*B) ReportAllocsSource
func (b *B) ReportAllocs()
ReportAllocs 为此基准启用 malloc 统计信息。它相当于设置 -test.benchmem,但它只影响调用 ReportAllocs 的基准函数。
func (*B) ResetTimerSource
func (b *B) ResetTimer()
ResetTimer 将经过的基准时间和内存分配计数器清零。它不会影响计时器是否正在运行。
func (*B) RunSource
func (b *B) Run(name string, f func(b *B)) bool
运行基准f作为具有给定名称的子基准。它报告是否有任何失败。
子基准与其他基准相似。调用 Run 至少一次的基准测试本身不会被测量,并且会在 N = 1 时被调用一次。
运行可以从多个 goroutine 同时调用,但所有此类调用都必须在 b 返回的外部基准测试函数之前返回。
func (*B) RunParallelSource
func (b *B) RunParallel(body func(*PB))
RunParallel 并行运行一个基准。它创建了多个 goroutine 并在它们之间分配 b.N 迭代。goroutines 的数量默认为 GOMAXPROCS 。为了增加非 CPU 绑定基准的并行性,请在RunParallel 之前调用 SetParallelism。RunParalle l通常与 go-test 标志一起使用。
body 函数将在每个 goroutine 中运行。它应该设置任何 goroutine-local 状态,然后迭代直到 pb.Next 返回 false。它不应该使用 StartTimer,StopTimer 或 ResetTimer 函数,因为它们具有全局效果。它也不应该叫 Run。
示例
package main
import (
"bytes"
"testing"
"text/template"
)
func main() {
// Parallel benchmark for text/template.Template.Execute on a single object.
testing.Benchmark(func(b *testing.B) {
templ := template.Must(template.New("test").Parse("Hello, {{.}}!"))
// RunParallel will create GOMAXPROCS goroutines
// and distribute work among them.
b.RunParallel(func(pb *testing.PB) {
// Each goroutine has its own bytes.Buffer.
var buf bytes.Buffer
for pb.Next() {
// The loop body is executed b.N times total across all goroutines.
buf.Reset()
templ.Execute(&buf, "World")
}
})
})
}
func (*B) SetBytesSource
func (b *B) SetBytes(n int64)
SetBytes 记录单个操作中处理的字节数。如果这被调用,基准将报告 ns / op和MB / s 。
func (*B) SetParallelismSource
func (b *B) SetParallelism(p int)
SetParallelism 将 RunParallel 使用的 goroutines 的数量设置为 p * GOMAXPROCS。通常不需要为 CPU 绑定的基准调用 SetParallelism。如果 p 小于1,该调用将不起作用。
func (*B) SkipSource
func (c *B) Skip(args ...interface{})
跳过相当于 Log,然后是 SkipNow。
func (*B) SkipNowSource
func (c *B) SkipNow()
SkipNow 将测试标记为已被跳过并停止执行。如果测试失败(请参阅错误,Errorf,失败),然后跳过,它仍然被认为失败。执行将继续在下一个测试或基准。另请参阅 FailNow。必须从运行测试的 goroutine 调用 SkipNow,而不是从测试期间创建的其他 goutoutine 调用。调用 SkipNow 不会停止那些其他的 goroutines。
func (*B) SkipfSource
func (c *B) Skipf(format string, args ...interface{})
Skipf 相当于 Logf,后跟 SkipNow。
func (*B) SkippedSource
func (c *B) Skipped() bool
Skipped 报告是否跳过测试。
func (*B) StartTimerSource
func (b *B) StartTimer()
StartTimer 开始计时测试。该功能在基准测试开始之前自动调用,但它也可用于在调用 StopTimer 之后恢复计时。
func (*B) StopTimerSource
func (b *B) StopTimer()
StopTimer 停止计时测试。这可用于在执行复杂的初始化时暂停计时器,而不需要进行测量。
type BenchmarkResultSource
基准测试的结果。
type BenchmarkResult struct {
N int // The number of iterations.
T time.Duration // The total time taken.
Bytes int64 // Bytes processed in one iteration.
MemAllocs uint64 // The total number of memory allocations.
MemBytes uint64 // The total number of bytes allocated.
}
func BenchmarkSource
func Benchmark(f func(b *B)) BenchmarkResult
基准测试标准的单一功能。用于创建不使用“go test”命令的自定义基准。
如果 f 调用 Run,则结果将是运行其所有不调用单个基准测试中顺序运行的子基准的估计值。
func (BenchmarkResult) AllocedBytesPerOpSource
func (r BenchmarkResult) AllocedBytesPerOp() int64
AllocedBytesPerOp 返回 r.MemBytes / rN
func (BenchmarkResult) AllocsPerOpSource
func (r BenchmarkResult) AllocsPerOp() int64
AllocsPerOp 返回 r.MemAllocs / rN
func (BenchmarkResult) MemStringSource
func (r BenchmarkResult) MemString() string
MemString 以' go test '的格式返回 r.AllocedBytesPerOp 和 r.AllocsPerOp。
func (BenchmarkResult) NsPerOpSource
func (r BenchmarkResult) NsPerOp() int64
func (BenchmarkResult) StringSource
func (r BenchmarkResult) String() string
type CoverSource
Cover 记录了测试覆盖率检查的信息。注意:此结构是测试基础架构的内部结构,可能会更改。Go 1 兼容性指南并未涵盖此内容。
type Cover struct {
Mode string
Counters map[string][]uint32
Blocks map[string][]CoverBlock
CoveredPackages string
}
type CoverBlockSource
CoverBlock 记录单个基本块的覆盖率数据。注意:此结构是测试基础架构的内部结构,可能会更改。Go 1 兼容性指南并未涵盖此内容。
type CoverBlock struct {
Line0 uint32
Col0 uint16
Line1 uint32
Col1 uint16
Stmts uint16
}
type InternalBenchmarkSource
内部类型,但因为是交叉包装而被导出; 部分执行“go test”命令。
type InternalBenchmark struct {
Name string
F func(b *B)
}
type InternalExampleSource
type InternalExample struct {
Name string
F func()
Output string
Unordered bool
}
type InternalTestSource
内部类型,但因为是交叉包装而被导出; 部分执行“go test”命令。
type InternalTest struct {
Name string
F func(*T)
}
键入MSource
M 是传递给 TestMain 函数来运行实际测试的类型。
type M struct {
// contains filtered or unexported fields
}
func MainStartSource
func MainStart(deps testDeps, tests []InternalTest, benchmarks []InternalBenchmark, examples []InternalExample) *M
MainStart 旨在供'go test'生成的测试使用。这并不意味着直接被调用,并且不受 Go 1 兼容性文档的约束。它可能会改变从发布到发布的签名。
func (*M) RunSource
func (m *M) Run() int
Run 运行测试。它返回一个退出代码传递给 os.Exit。
键入PBSource
RunParallel 使用 PB 来运行平行基准。
type PB struct {
// contains filtered or unexported fields
}
func (*PB) NextSource
func (pb *PB) Next() bool
Next 报告是否有更多的迭代要执行。
键入TSource
T 是传递给测试功能以管理测试状态并支持格式化测试日志的类型。日志在执行过程中累计,完成后转储到标准输出。
当测试函数返回或调用任何方法 FailNow,Fatal,Fatalf,SkipNow,Skip 或 Skip f时,测试结束。这些方法以及 Parallel 方法只能从运行 Test 函数的 goroutine 中调用。
其他报告方法(如 Log 和 Error 的变体)可以从多个 goroutines 同时调用。
type T struct {
// contains filtered or unexported fields
}
func (*T) ErrorSource
func (c *T) Error(args ...interface{})
Error 等同于 Log,然后是 Fail。
func (*T) ErrorfSource
func (c *T) Errorf(format string, args ...interface{})
Errorf 等同于 Logf,然后是 Fail。
func (*T) FailSource
func (c *T) Fail()
Fail 会将该功能标记为失败,但会继续执行。
func (*T) FailNowSource
func (c *T) FailNow()
FailNow 将该函数标记为失败并停止其执行。执行将继续在下一个测试或基准。必须从运行测试或基准测试函数的 goroutine 调用 FailNow,而不是在测试期间创建的其他 goutoutine 调用。调用 FailNow 不会停止那些其他的 goroutine。
func (*T) FailedSource
func (c *T) Failed() bool
Failed 的报告功能是否失败。
func (*T) FatalSource
func (c *T) Fatal(args ...interface{})
Fatal 相当于 Log,然后是 FailNow。
func (*T) FatalfSource
func (c *T) Fatalf(format string, args ...interface{})
Fatalf 等同于 Logf,然后是 FailNow。
func (*T) HelperSource
func (c *T) Helper()
Helper 将调用函数标记为测试帮助函数。打印文件和行信息时,该功能将被跳过。可以从多个 goroutines 同时调用 Helper 。如果 Heller 从 TestXxx / BenchmarkXxx 函数或子测试/次级基准测试函数直接调用,则 Helper 不起作用。
func (*T) LogSource
func (c *T) Log(args ...interface{})
日志使用默认格式化格式化其参数,类似于 Println,并将文本记录在错误日志中。对于测试,仅当测试失败或设置了 -test.v 标志时才会打印文本。对于基准测试,总是打印文本以避免性能取决于 -test.v 标志的值。
func (*T) LogfSource
func (c *T) Logf(format string, args ...interface{})
Logf 根据格式格式化其参数,类似于 Printf,并将文本记录在错误日志中。如果没有提供,最后换行符会被添加。对于测试,仅当测试失败或设置了 -test.v 标志时才会打印文本。对于基准测试,总是打印文本以避免性能取决于 -test.v 标志的值。
func (*T) NameSource
func (c *T) Name() string
Name 返回正在运行的测试或基准的名称。
func (*T) ParallelSource
func (t *T) Parallel()
并行信号表示该测试将与其他并行测试并行运行(并且仅与其他测试并行)。当由于使用 -test.count 或 -test.cpu 而多次运行测试时,单个测试的多个实例永远不会彼此并行运行。
func (*T) RunSource
func (t *T) Run(name string, f func(t *T)) bool
Run 运行 f 作为名为 t 的子测试。它报告f是否成功。运行 f 在一个单独的 goroutine 中运行 f 并阻塞,直到所有并行子测试完成。
Run 可以从多个 goroutine 同时调用,但所有这些调用必须在外部测试函数返回之前返回。
func (*T) SkipSource
func (c *T) Skip(args ...interface{})
Skip 相当于 Log,然后是 SkipNow。
func (*T) SkipNowSource
func (c *T) SkipNow()
SkipNow 将测试标记为已被跳过并停止执行。如果测试失败(请参阅错误,Errorf,失败),然后跳过,它仍然被认为失败。执行将继续在下一个测试或基准。另请参阅 FailNow。必须从运行测试的 goroutine 调用 SkipNow,而不是从测试期间创建的其他 goutoutine 调用。调用 SkipNow 不会停止那些其他的 goroutines。
func (*T) SkipfSource
func (c *T) Skipf(format string, args ...interface{})
Skipf 相当于 Logf,后跟 SkipNow。
func (*T) SkippedSource
func (c *T) Skipped() bool
Skipped 报告是否跳过测试。
键入TBSource
TB 是 T 和 B 共同的界面。
type TB interface {
Error(args ...interface{})
Errorf(format string, args ...interface{})
Fail()
FailNow()
Failed() bool
Fatal(args ...interface{})
Fatalf(format string, args ...interface{})
Log(args ...interface{})
Logf(format string, args ...interface{})
Name() string
Skip(args ...interface{})
SkipNow()
Skipf(format string, args ...interface{})
Skipped() bool
Helper()
// contains filtered or unexported methods
}
子目录
名称 |
概要 |
---|
| .. |
| iotest | 打包iotest 实现主要用于测试的读者和写入者。|
| quick | 打包quick 实现实用功能,以帮助进行黑匣子测试。|
测试 | testing | ||
---|---|---|
测试 | testing | 详细 | |
testing/iotest | 详细 | |
testing/quick | 详细 |
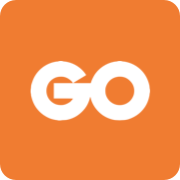
Go 是一种编译型语言,它结合了解释型语言的游刃有余,动态类型语言的开发效率,以及静态类型的安全性。它也打算成为现代的,支持网络与多核计算的语言。要满足这些目标,需要解决一些语言上的问题:一个富有表达能力但轻量级的类型系统,并发与垃圾回收机制,严格的依赖规范等等。这些无法通过库或工具解决好,因此Go也就应运而生了。
主页 | https://golang.org/ |
源码 | https://go.googlesource.com/go |
发布版本 | 1.9.2 |