Go参考手册
net/smtp
net/smtp
import "net/smtp"
- 概观
- 索引
- 示例
概观
smtp 包实现了RFC 5321中定义的简单邮件传输协议。它还实现了以下扩展:
8BITMIME RFC 1652
AUTH RFC 2554
STARTTLS RFC 3207
其他扩展可能由客户端处理。
smtp 软件包被冻结,不接受新的功能。一些外部软件包提供更多功能。看到:
https://godoc.org/?q=smtp
示例
编码:
// Connect to the remote SMTP server.
c, err := smtp.Dial("mail.example.com:25")
if err != nil {
log.Fatal(err)
}
// Set the sender and recipient first
if err := c.Mail("sender@example.org"); err != nil {
log.Fatal(err)
}
if err := c.Rcpt("recipient@example.net"); err != nil {
log.Fatal(err)
}
// Send the email body.
wc, err := c.Data()
if err != nil {
log.Fatal(err)
}
_, err = fmt.Fprintf(wc, "This is the email body")
if err != nil {
log.Fatal(err)
}
err = wc.Close()
if err != nil {
log.Fatal(err)
}
// Send the QUIT command and close the connection.
err = c.Quit()
if err != nil {
log.Fatal(err)
}
索引
- func SendMail(addr string, a Auth, from string, to []string, msg []byte) error
- type Auth
- func CRAMMD5Auth(username, secret string) Auth
- func PlainAuth(identity, username, password, host string) Auth
- type Client
- func Dial(addr string) (*Client, error)
- func NewClient(conn net.Conn, host string) (*Client, error)
- func (c *Client) Auth(a Auth) error
- func (c *Client) Close() error
- func (c *Client) Data() (io.WriteCloser, error)
- func (c *Client) Extension(ext string) (bool, string)
- func (c *Client) Hello(localName string) error
- func (c *Client) Mail(from string) error
- func (c *Client) Quit() error
- func (c *Client) Rcpt(to string) error
- func (c *Client) Reset() error
- func (c *Client) StartTLS(config *tls.Config) error
- func (c *Client) TLSConnectionState() (state tls.ConnectionState, ok bool)
- func (c *Client) Verify(addr string) error
- type ServerInfo
示例
Package
PlainAuth
SendMail
打包文件
auth.go smtp.go
func SendMail(显示源文件)
func SendMail(addr string, a Auth, from string, to []string, msg []byte) error
SendMail 在 addr 连接到服务器,如果可能的话,切换到 TLS,如果可能,使用可选机制进行身份验证,然后使用消息 msg 从地址发送到地址的电子邮件。addr 必须包含一个端口,如“mail.example.com:smtp”中所示。
to 参数中的地址是 SMTP RCPT 地址。
msg 参数应该是一个 RFC 822 风格的电子邮件,首先包含标题,空白行,然后是邮件正文。msg 的行应该是 CRLF 终止的。 msg 标题通常应包含“From”,“To”,“Subject”和“Cc”等字段。发送“密件抄送”邮件是通过在 to 参数中包含一个电子邮件地址来完成的,但不包括在msg头文件中。
SendMail 函数和 net / smtp 包是低级机制,不支持 DKIM 签名,MIME 附件(请参阅mime / multipart包)或其他邮件功能。更高级别的软件包存在于标准库之外。
示例
package main
import (
"log"
"net/smtp"
)
func main() {
// Set up authentication information.
auth := smtp.PlainAuth("", "user@example.com", "password", "mail.example.com")
// Connect to the server, authenticate, set the sender and recipient,
// and send the email all in one step.
to := []string{"recipient@example.net"}
msg := []byte("To: recipient@example.net\r\n" +
"Subject: discount Gophers!\r\n" +
"\r\n" +
"This is the email body.\r\n")
err := smtp.SendMail("mail.example.com:25", auth, "sender@example.org", to, msg)
if err != nil {
log.Fatal(err)
}
}
type Auth(显示源文件)
身份验证通过 SMTP 身份验证机制实施。
type Auth interface {
// Start begins an authentication with a server.
// It returns the name of the authentication protocol
// and optionally data to include in the initial AUTH message
// sent to the server. It can return proto == "" to indicate
// that the authentication should be skipped.
// If it returns a non-nil error, the SMTP client aborts
// the authentication attempt and closes the connection.
Start(server *ServerInfo) (proto string, toServer []byte, err error)
// Next continues the authentication. The server has just sent
// the fromServer data. If more is true, the server expects a
// response, which Next should return as toServer; otherwise
// Next should return toServer == nil.
// If Next returns a non-nil error, the SMTP client aborts
// the authentication attempt and closes the connection.
Next(fromServer []byte, more bool) (toServer []byte, err error)
}
func CRAMMD5Auth(显示源文件)
func CRAMMD5Auth(username, secret string) Auth
CRAMMD5Auth 返回一个Auth,它实现 RFC 2195 中定义的 CRAM-MD5 认证机制。返回的 Auth 使用给定的用户名和密码使用质询 - 响应机制向服务器进行认证。
func PlainAuth(显示源文件)
func PlainAuth(identity, username, password, host string) Auth
PlainAuth 返回一个 Auth,它实现 RFC 4616 中定义的 PLAIN 身份验证机制。返回的 Auth 使用给定的用户名和密码对主机进行身份验证,并充当身份。通常身份应该是空字符串,充当用户名。
如果连接使用 TLS 或连接到本地主机,PlainAuth 将仅发送凭据。否则,身份验证将失败并出现错误,而不会发送凭据。
示例
编码:
// hostname is used by PlainAuth to validate the TLS certificate.
hostname := "mail.example.com"
auth := smtp.PlainAuth("", "user@example.com", "password", hostname)
err := smtp.SendMail(hostname+":25", auth, from, recipients, msg)
if err != nil {
log.Fatal(err)
}
type Client(显示源文件)
客户端代表到 SMTP 服务器的客户端连接。
type Client struct {
// Text is the textproto.Conn used by the Client. It is exported to allow for
// clients to add extensions.
Text *textproto.Conn
// contains filtered or unexported fields
}
func Dial(显示源文件)
func Dial(addr string) (*Client, error)
Dial 返回一个新的连接到 SMTP 服务器的客户端。 addr 必须包含一个端口,如“mail.example.com:smtp”中所示。
func NewClient(显示源文件)
func NewClient(conn net.Conn, host string) (*Client, error)
NewClient 使用现有连接和主机作为服务器名称返回一个新的客户端,以便在进行身份验证时使用。
func (*Client) Auth(显示源文件)
func (c *Client) Auth(a Auth) error
Auth 使用提供的认证机制来认证客户端。认证失败将关闭连接。只有通告 AUTH 扩展的服务器才支持此功能。
func (*Client) Close(显示源文件)
func (c *Client) Close() error
Close 关闭连接。
func (*Client) Data(显示源文件)
func (c *Client) Data() (io.WriteCloser, error)
数据向服务器发出 DATA 命令并返回可用于写入邮件标题和正文的编写器。调用者应该在调用c上的更多方法之前关闭作者。对数据的调用必须在一个或多个对 Rcpt 的调用之前进行。
func (*Client) Extension(显示源文件)
func (c *Client) Extension(ext string) (bool, string)
Extension 报告扩展是否受服务器支持。扩展名不区分大小写。如果支持扩展,Extension 还会返回一个字符串,其中包含服务器为扩展指定的任何参数。
func (*Client) Hello(显示源文件)
func (c *Client) Hello(localName string) error
Hello 将 HELO 或 EHLO 作为给定的主机名称发送到服务器。仅当客户端需要控制所使用的主机名称时才需要调用此方法。否则客户端会自动将自身介绍为“本地主机”。如果调用 Hello,则必须在任何其他方法之前调用它。
func (*Client) Mail(显示源文件)
func (c *Client) Mail(from string) error
邮件使用提供的电子邮件地址向服务器发出MAIL 命令。如果服务器支持 8BITMIME 扩展,则 Mail 会添加 BODY = 8BITMIME 参数。这将启动一个邮件事务,然后是一个或多个 Rcpt 调用。
func (*Client) Quit(显示源文件)
func (c *Client) Quit() error
退出发送 QUIT 命令并关闭连接到服务器。
func (*Client) Rcpt(显示源文件)
func (c *Client) Rcpt(to string) error
Rcpt 使用提供的电子邮件地址向服务器发出 RCPT 命令。对 Rcpt 的调用必须在对 Mail 的调用之后进行,然后可以进行数据调用或其他 Rcpt 调用。
func (*Client) Reset(显示源文件)
func (c *Client) Reset() error
重置将 RSET 命令发送到服务器,中止当前的邮件事务。
func (*Client) StartTLS(显示源文件)
func (c *Client) StartTLS(config *tls.Config) error
StartTLS 发送 STARTTLS 命令并加密所有进一步的通信。只有通告 STARTTLS 扩展的服务器才支持此功能。
func (*Client) TLSConnectionState(显示源文件)
func (c *Client) TLSConnectionState() (state tls.ConnectionState, ok bool)
TLSConnectionState 返回客户端的 TLS 连接状态。如果 StartTLS 不成功,则返回值为零值。
func (*Client) Verify(显示源文件)
func (c *Client) Verify(addr string) error
验证检查服务器上电子邮件地址的有效性。如果验证返回 nil ,则地址有效。非零返回不一定表示无效地址。出于安全原因,许多服务器不会验证地址。
type ServerInfo(显示源文件)
ServerInfo 记录有关 SMTP 服务器的信息。
type ServerInfo struct {
Name string // SMTP server name
TLS bool // using TLS, with valid certificate for Name
Auth []string // advertised authentication mechanisms
}
net/smtp | ||
---|---|---|
net/smtp | 详细 |
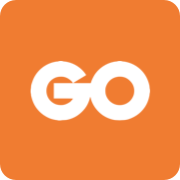
Go 是一种编译型语言,它结合了解释型语言的游刃有余,动态类型语言的开发效率,以及静态类型的安全性。它也打算成为现代的,支持网络与多核计算的语言。要满足这些目标,需要解决一些语言上的问题:一个富有表达能力但轻量级的类型系统,并发与垃圾回收机制,严格的依赖规范等等。这些无法通过库或工具解决好,因此Go也就应运而生了。
主页 | https://golang.org/ |
源码 | https://go.googlesource.com/go |
发布版本 | 1.9.2 |