Go参考手册
math/cmplx
math/cmplx
import "math/cmplx"
- 概述
- Index
- 示例
概述
cmplx 包为复数提供基本的常量和数学函数。
索引
- func Abs(x complex128) float64
- func Acos(x complex128) complex128
- func Acosh(x complex128) complex128
- func Asin(x complex128) complex128
- func Asinh(x complex128) complex128
- func Atan(x complex128) complex128
- func Atanh(x complex128) complex128
- func Conj(x complex128) complex128
- func Cos(x complex128) complex128
- func Cosh(x complex128) complex128
- func Cot(x complex128) complex128
- func Exp(x complex128) complex128
- func Inf() complex128
- func IsInf(x complex128) bool
- func IsNaN(x complex128) bool
- func Log(x complex128) complex128
- func Log10(x complex128) complex128
- func NaN() complex128
- func Phase(x complex128) float64
- func Polar(x complex128) (r, θ float64)
- func Pow(x, y complex128) complex128
- func Rect(r, θ float64) complex128
- func Sin(x complex128) complex128
- func Sinh(x complex128) complex128
- func Sqrt(x complex128) complex128
- func Tan(x complex128) complex128
- func Tanh(x complex128) complex128
示例
Abs Exp Polar
打包文件
abs.go asin.go conj.go exp.go isinf.go isnan.go log.go phase.go polar.go pow.go rect.go sin.go sqrt.go tan.go
func Abs(显示源文件)
func Abs(x complex128) float64
Abs 返回 x 的绝对值(也称为模数)。
示例
package main
import (
"fmt"
"math/cmplx"
)
func main() {
fmt.Printf("%.1f", cmplx.Abs(3+4i))
}
func Acos (显示源文件)
func Acos(x complex128) complex128
Acos 返回 x 的反余弦。
func Acosh(显示源文件)
func Acosh(x complex128) complex128
Acosh 返回 x 的反双曲余弦。
func Asin(显示源文件)
func Asin(x complex128) complex128
Asin 返回 x 的反正弦。
func Asinh(显示源文件)
func Asinh(x complex128) complex128
Asinh 返回 x 的反双曲正弦。
func Atan(显示源文件)
func Atan(x complex128) complex128
Atan 返回 x 的反正切值。
func Atanh(显示源文件)
func Atanh(x complex128) complex128
Atanh 返回 x 的反双曲正切。
func Conj(显示源文件)
func Conj(x complex128) complex128
Conj 返回 x 的复共轭。
func Cos(显示源文件)
func Cos(x complex128) complex128
Cos 返回 x 的余弦。
func Cosh(显示源文件)
func Cosh(x complex128) complex128
Cosh 返回 x 的双曲余弦。
func Cot(显示源文件)
func Cot(x complex128) complex128
Cot 返回 x 的余切值。
func Exp(显示源文件)
func Exp(x complex128) complex128
Exp 返回 e ** x,即 x 的基数 e 指数。
示例
ExampleExp 计算欧拉的特征。
package main
import (
"fmt"
"math"
"math/cmplx"
)
func main() {
fmt.Printf("%.1f", cmplx.Exp(1i*math.Pi)+1)
}
func Inf(显示源文件)
func Inf() complex128
Inf 返回复数无穷大复数 (+Inf, +Inf) 。
func IsInf(显示源文件)
func IsInf(x complex128) bool
如果 real(x)或 imag(x) 是无穷大,IsInf 返回 true。
func IsNaN(查看源文件)
func IsNaN(x complex128) bool
如果 real(x) 或 imag(x) 是 NaN 且既不是无穷大, IsNaN 也会返回 true。
func Log(查看源文件)
func Log(x complex128) complex128
Log 返回 x 的自然对数。
func Log10(查看源文件)
func Log10(x complex128) complex128
Log10 返回 x 的小数对数。
func NaN(查看源文件)
func NaN() complex128
NaN 返回一个复杂的“非数字”值。
func Phase(查看源文件)
func Phase(x complex128) float64
阶段返回x的阶段(也称为参数)。返回的值在-Pi,Pi范围内。
func PolarSource
func Polar(x complex128) (r, θ float64)
极性返回x的绝对值r和相位θ,使得x = r * e **θi。相位在-Pi,Pi范围内。
示例
package main
import (
"fmt"
"math"
"math/cmplx"
)
func main() {
r, theta := cmplx.Polar(2i)
fmt.Printf("r: %.1f, θ: %.1f*π", r, theta/math.Pi)
}
func PowSource
func Pow(x, y complex128) complex128
Pow返回x ** y,y的基x指数。为了与 math.Pow 的通用兼容性:
Pow(0, ±0) returns 1+0i
Pow(0, c) for real(c)<0 returns Inf+0i if imag(c) is zero, otherwise Inf+Inf i.
func RectSource
func Rect(r, θ float64) complex128
Rect以极坐标r,θ返回复数x。
func SinSource
func Sin(x complex128) complex128
Sin返回x的正弦值。
func SinhSource
func Sinh(x complex128) complex128
Sinh返回x的双曲正弦。
func SqrtSource
func Sqrt(x complex128) complex128
Sqrt返回x的平方根。结果r被选择为使得real(r)≥0且imag(r)与imag(x)具有相同的符号。
func TanSource
func Tan(x complex128) complex128
Tan返回x的正切值。
func TanhSource
func Tanh(x complex128) complex128
Tanh返回x的双曲正切。
math/cmplx | ||
---|---|---|
math/cmplx | 详细 |
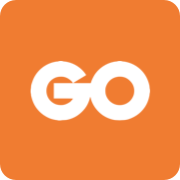
Go 是一种编译型语言,它结合了解释型语言的游刃有余,动态类型语言的开发效率,以及静态类型的安全性。它也打算成为现代的,支持网络与多核计算的语言。要满足这些目标,需要解决一些语言上的问题:一个富有表达能力但轻量级的类型系统,并发与垃圾回收机制,严格的依赖规范等等。这些无法通过库或工具解决好,因此Go也就应运而生了。
主页 | https://golang.org/ |
源码 | https://go.googlesource.com/go |
发布版本 | 1.9.2 |