Go参考手册
字符串 | strings
字符串 | strings
import "strings"
- 概观
- 索引
- 示例
概观
打包字符串实现简单的函数来操纵 UTF-8 编码的字符串。
有关 Go 中 UTF-8 字符串的信息,请参阅https://blog.golang.org/strings。
索引
- func Compare(a, b string) int
- func Contains(s, substr string) bool
- func ContainsAny(s, chars string) bool
- func ContainsRune(s string, r rune) bool
- func Count(s, substr string) int
- func EqualFold(s, t string) bool
- func Fields(s string) []string
- func FieldsFunc(s string, f func(rune) bool) []string
- func HasPrefix(s, prefix string) bool
- func HasSuffix(s, suffix string) bool
- func Index(s, substr string) int
- func IndexAny(s, chars string) int
- func IndexByte(s string, c byte) int
- func IndexFunc(s string, f func(rune) bool) int
- func IndexRune(s string, r rune) int
- func Join(a []string, sep string) string
- func LastIndex(s, substr string) int
- func LastIndexAny(s, chars string) int
- func LastIndexByte(s string, c byte) int
- func LastIndexFunc(s string, f func(rune) bool) int
- func Map(mapping func(rune) rune, s string) string
- func Repeat(s string, count int) string
- func Replace(s, old, new string, n int) string
- func Split(s, sep string) []string
- func SplitAfter(s, sep string) []string
- func SplitAfterN(s, sep string, n int) []string
- func SplitN(s, sep string, n int) []string
- func Title(s string) string
- func ToLower(s string) string
- func ToLowerSpecial(c unicode.SpecialCase, s string) string
- func ToTitle(s string) string
- func ToTitleSpecial(c unicode.SpecialCase, s string) string
- func ToUpper(s string) string
- func ToUpperSpecial(c unicode.SpecialCase, s string) string
- func Trim(s string, cutset string) string
- func TrimFunc(s string, f func(rune) bool) string
- func TrimLeft(s string, cutset string) string
- func TrimLeftFunc(s string, f func(rune) bool) string
- func TrimPrefix(s, prefix string) string
- func TrimRight(s string, cutset string) string
- func TrimRightFunc(s string, f func(rune) bool) string
- func TrimSpace(s string) string
- func TrimSuffix(s, suffix string) string
- type Reader
- func NewReader(s string) *Reader
- func (r *Reader) Len() int
- func (r *Reader) Read(b []byte) (n int, err error)
- func (r *Reader) ReadAt(b []byte, off int64) (n int, err error)
- func (r *Reader) ReadByte() (byte, error)
- func (r *Reader) ReadRune() (ch rune, size int, err error)
- func (r *Reader) Reset(s string)
- func (r *Reader) Seek(offset int64, whence int) (int64, error)
- func (r *Reader) Size() int64
- func (r *Reader) UnreadByte() error
- func (r *Reader) UnreadRune() error
- func (r *Reader) WriteTo(w io.Writer) (n int64, err error)
- type Replacer
- func NewReplacer(oldnew ...string) *Replacer
- func (r *Replacer) Replace(s string) string
- func (r *Replacer) WriteString(w io.Writer, s string) (n int, err error)
- 错误
示例
Compare Contains ContainsAny ContainsRune Count EqualFold Fields FieldsFunc HasPrefix HasSuffix Index IndexAny IndexByte IndexFunc IndexRune Join LastIndex LastIndexAny Map NewReplacer Repeat Replace Split SplitAfter SplitAfterN SplitN Title ToLower ToTitle ToUpper Trim TrimFunc TrimPrefix TrimSpace TrimSuffix
打包files
compare.go reader.go replace.go search.go strings.go strings_amd64.go strings_decl.go
func CompareSource
func Compare(a, b string) int
Compare 按字典顺序返回一个比较两个字符串的整数。如果 a == b,结果将为0,如果 a <b 则返回-1,如果 a> b 则返回+1。
Compare 仅包含与封装字节的对称性。使用内置的字符串比较运算符 ==,<,> 等通常会更清晰并且速度更快。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.Compare("a", "b"))
fmt.Println(strings.Compare("a", "a"))
fmt.Println(strings.Compare("b", "a"))
}
func ContainsSource
func Contains(s, substr string) bool
包含报告 substr 是否在 s 之内。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.Contains("seafood", "foo"))
fmt.Println(strings.Contains("seafood", "bar"))
fmt.Println(strings.Contains("seafood", ""))
fmt.Println(strings.Contains("", ""))
}
func ContainsAnySource
func ContainsAny(s, chars string) bool
ContainsAny 报告字符中的任何 Unicode 代码点是否在 s 中。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.ContainsAny("team", "i"))
fmt.Println(strings.ContainsAny("failure", "u & i"))
fmt.Println(strings.ContainsAny("foo", ""))
fmt.Println(strings.ContainsAny("", ""))
}
func ContainsRuneSource
func ContainsRune(s string, r rune) bool
ContainsRune 报告 Unicode 代码点 r 是否在 s 内。
示例
package main
import (
"fmt"
"strings"
)
func main() {
// Finds whether a string contains a particular Unicode code point.
// The code point for the lowercase letter "a", for example, is 97.
fmt.Println(strings.ContainsRune("aardvark", 97))
fmt.Println(strings.ContainsRune("timeout", 97))
}
func CountSource
func Count(s, substr string) int
Count 计算 s 中不重叠的 substr 实例的数量。如果 substr 是一个空字符串,则 Count 将返回 1 + s 中的 Unicode 代码点数。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.Count("cheese", "e"))
fmt.Println(strings.Count("five", "")) // before & after each rune
}
func EqualFoldSource
func EqualFold(s, t string) bool
EqualFold 报告 s 和 t,解释为 UTF-8 字符串,在 Unicode 大小写折叠下是否相等。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.EqualFold("Go", "go"))
}
func FieldsSource
func Fields(s string) []string
Fields 将字符串 s 分割为一个或多个连续的空格字符的每个实例,由 unicode.IsSpace 定义,如果 s 仅包含空格,则返回 s 的子字符串数组或空列表。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Printf("Fields are: %q", strings.Fields(" foo bar baz "))
}
func FieldsFuncSource
func FieldsFunc(s string, f func(rune) bool) []string
FieldsFunc 在每次运行满足 f(c) 的 Unicode 代码点 c 时分割字符串 s ,并返回一组 s 片段。如果 s 中的所有代码点都满足 f(c) 或者字符串为空,则返回空片。FieldsFunc 不保证它调用 f(c) 的顺序。如果f没有为给定的c返回一致的结果,那么 FieldsFunc 可能会崩溃。
示例
package main
import (
"fmt"
"strings"
"unicode"
)
func main() {
f := func(c rune) bool {
return !unicode.IsLetter(c) && !unicode.IsNumber(c)
}
fmt.Printf("Fields are: %q", strings.FieldsFunc(" foo1;bar2,baz3...", f))
}
func HasPrefixSource
func HasPrefix(s, prefix string) bool
HasPrefix 测试字符串 s 是否以前缀开头。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.HasPrefix("Gopher", "Go"))
fmt.Println(strings.HasPrefix("Gopher", "C"))
fmt.Println(strings.HasPrefix("Gopher", ""))
}
func HasSuffixSource
func HasSuffix(s, suffix string) bool
HasSuffix 测试字符串 s 是否以后缀结尾。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.HasSuffix("Amigo", "go"))
fmt.Println(strings.HasSuffix("Amigo", "O"))
fmt.Println(strings.HasSuffix("Amigo", "Ami"))
fmt.Println(strings.HasSuffix("Amigo", ""))
}
func IndexSource
func Index(s, substr string) int
索引返回 s 中第一个 substr 实例的索引,如果 substr 不存在于 s 中,则返回-1。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.Index("chicken", "ken"))
fmt.Println(strings.Index("chicken", "dmr"))
}
func IndexAnySource
func IndexAny(s, chars string) int
IndexAny 返回 s 中任何 Unicode 代码点的第一个实例的索引,如果没有来自 chars 的 Unicode 代码点,则返回-1。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.IndexAny("chicken", "aeiouy"))
fmt.Println(strings.IndexAny("crwth", "aeiouy"))
}
func IndexByteSource
func IndexByte(s string, c byte) int
IndexByte 返回 s 的第一个实例的索引,如果 c 不存在于 s 中,则返回-1。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.IndexByte("golang", 'g'))
fmt.Println(strings.IndexByte("gophers", 'h'))
fmt.Println(strings.IndexByte("golang", 'x'))
}
func IndexFuncSource
func IndexFunc(s string, f func(rune) bool) int
IndexFunc 将索引返回到满足 f(c) 的第一个 Unicode 代码点的 s 中,否则返回-1。
示例
package main
import (
"fmt"
"strings"
"unicode"
)
func main() {
f := func(c rune) bool {
return unicode.Is(unicode.Han, c)
}
fmt.Println(strings.IndexFunc("Hello, 世界", f))
fmt.Println(strings.IndexFunc("Hello, world", f))
}
func IndexRuneSource
func IndexRune(s string, r rune) int
IndexRune 返回 Unicode 代码点 r 的第一个实例的索引,如果符号不存在于 s 中,则返回-1。如果 r 是 utf8.RuneError ,它将返回任何无效的 UTF-8 字节序列的第一个实例。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.IndexRune("chicken", 'k'))
fmt.Println(strings.IndexRune("chicken", 'd'))
}
func JoinSource
func Join(a []string, sep string) string
Join 连接 a 的元素以创建单个字符串。分隔符字符串 sep 放置在结果字符串中的元素之间。
示例
package main
import (
"fmt"
"strings"
)
func main() {
s := []string{"foo", "bar", "baz"}
fmt.Println(strings.Join(s, ", "))
}
func LastIndexSource
func LastIndex(s, substr string) int
LastIndex 返回 s 中最后一个 substr 实例的索引,如果 substr 不存在于 s 中,则返回-1。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.Index("go gopher", "go"))
fmt.Println(strings.LastIndex("go gopher", "go"))
fmt.Println(strings.LastIndex("go gopher", "rodent"))
}
func LastIndexAnySource
func LastIndexAny(s, chars string) int
LastIndexAny 返回来自 s 中字符的任何 Unicode 代码点的最后一个实例的索引,如果没有来自 chars 的 Unicode 代码点存在于 s 中,则返回-1。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.LastIndexAny("go gopher", "go"))
fmt.Println(strings.LastIndexAny("go gopher", "rodent"))
fmt.Println(strings.LastIndexAny("go gopher", "fail"))
}
func LastIndexByteSource
func LastIndexByte(s string, c byte) int
LastIndexByte 返回 s 的最后一个实例的索引,如果 c 不存在于 s 中,则返回-1。
func LastIndexFuncSource
func LastIndexFunc(s string, f func(rune) bool) int
LastIndexFunc 将索引返回到满足 f(c) 的最后一个 Unicode 代码点的 s 中,否则返回-1。
func MapSource
func Map(mapping func(rune) rune, s string) string
Map 根据映射函数返回字符串 s 的一个副本,并修改其所有字符。如果映射返回负值,则字符将从字符串中删除而不会被替换。
示例
package main
import (
"fmt"
"strings"
)
func main() {
rot13 := func(r rune) rune {
switch {
case r >= 'A' && r <= 'Z':
return 'A' + (r-'A'+13)%26
case r >= 'a' && r <= 'z':
return 'a' + (r-'a'+13)%26
}
return r
}
fmt.Println(strings.Map(rot13, "'Twas brillig and the slithy gopher..."))
}
func RepeatSource
func Repeat(s string, count int) string
Repeat 返回一个由字符串 s 的计数副本组成的新字符串。
如果count为负数或(len(s)* count)的结果溢出,它会发生混乱。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println("ba" + strings.Repeat("na", 2))
}
func ReplaceSource
func Replace(s, old, new string, n int) string
Replace 返回字符串s的一个副本,其中前 n 个不重叠的旧实例由 new 替换。如果 old 为空,则它在字符串的开始处和每个 UTF-8 序列之后进行匹配,最多生成一个 k-1 字符串的 k+1 替换。如果 n <0,则替换次数没有限制。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.Replace("oink oink oink", "k", "ky", 2))
fmt.Println(strings.Replace("oink oink oink", "oink", "moo", -1))
}
func SplitSource
func Split(s, sep string) []string
将切片分割成由 sep 分隔的所有子字符串,并在这些分隔符之间返回一个子切片片段。
如果 s 不包含 sep 且 sep 不为空,则 Split 将返回长度为1的片段,其唯一元素为 s。
如果 sep 为空,Split 会在每个 UTF-8 序列之后分裂。如果 s 和 sep 都为空,Split 将返回一个空片。
它相当于 SplitN,计数为-1。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Printf("%q\n", strings.Split("a,b,c", ","))
fmt.Printf("%q\n", strings.Split("a man a plan a canal panama", "a "))
fmt.Printf("%q\n", strings.Split(" xyz ", ""))
fmt.Printf("%q\n", strings.Split("", "Bernardo O'Higgins"))
}
func SplitAfterSource
func SplitAfter(s, sep string) []string
SplitAfter 在每个 sep 实例之后切片到所有子字符串中,并返回这些子字符串的一部分。
如果 s 不包含 sep 且 sep 不为空,则 SplitAfter 将返回长度为1的片段,其唯一元素为 s。
如果 sep 为空,则 SplitAfter 会在每个 UTF-8 序列后分割。如果 s 和 sep 均为空,则 SplitAfter 将返回空片。
它相当于 SplitAfterN,计数为-1。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Printf("%q\n", strings.SplitAfter("a,b,c", ","))
}
func SplitAfterNSource
func SplitAfterN(s, sep string, n int) []string
在每个 sep 实例之后,SplitAfterN 将 s 切成子字符串,并返回这些子字符串的一部分。
计数决定要返回的子字符串的数量:
n > 0: at most n substrings; the last substring will be the unsplit remainder.
n == 0: the result is nil (zero substrings)
n < 0: all substrings
s 和 sep 的边缘情况(例如,空字符串)按照 SplitAfter 文档中的描述进行处理。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Printf("%q\n", strings.SplitAfterN("a,b,c", ",", 2))
}
func SplitNSource
func SplitN(s, sep string, n int) []string
将 SplitN 切片分割成由 sep 分隔的子字符串,并返回这些分隔符之间的一个子字符串片段。
计数决定要返回的子字符串的数量:
n > 0: at most n substrings; the last substring will be the unsplit remainder.
n == 0: the result is nil (zero substrings)
n < 0: all substrings
s 和 sep 的边缘情况(例如,空字符串)按照 Split 的文档中的描述进行处理。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Printf("%q\n", strings.SplitN("a,b,c", ",", 2))
z := strings.SplitN("a,b,c", ",", 0)
fmt.Printf("%q (nil = %v)\n", z, z == nil)
}
func TitleSource
func Title(s string) string
标题返回字符串 s 的一个副本,其中所有的 Unicode 字母开始将单词映射到其标题大小写。
BUG(rsc):规则标题用于单词边界的规则不能正确处理 Unicode 标点符号。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.Title("her royal highness"))
}
func ToLowerSource
func ToLower(s string) string
ToLower 返回字符串 s 的一个副本,其中所有 Unicode 字母映射为小写字母。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.ToLower("Gopher"))
}
func ToLowerSpecialSource
func ToLowerSpecial(c unicode.SpecialCase, s string) string
ToLowerSpecial 返回字符串 s 的一个副本,其中所有的 Unicode 字母都映射到它们的小写字母,优先考虑特殊的外壳规则。
func ToTitleSource
func ToTitle(s string) string
ToTitle 返回字符串 s 的一个副本,其中所有 Unicode 字母映射到它们的标题大小写。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.ToTitle("loud noises"))
fmt.Println(strings.ToTitle("хлеб"))
}
func ToTitleSpecialSource
func ToTitleSpecial(c unicode.SpecialCase, s string) string
ToTitleSpecial 返回字符串 s 的副本,其中所有的 Unicode 字母映射到它们的标题大小写,优先考虑特殊的外壳规则。
func ToUpperSource
func ToUpper(s string) string
ToUpper 返回字符串 s 的副本,其中所有的 Unicode 字母都映射为大写字母。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.ToUpper("Gopher"))
}
func ToUpperSpecialSource
func ToUpperSpecial(c unicode.SpecialCase, s string) string
ToUpperSpecial 返回字符串 s 的副本,其中所有的 Unicode 字母映射为大写字母,优先考虑特殊的外壳规则。
func TrimSource
func Trim(s string, cutset string) string
Trim 将返回字符串 s 的一部分,并将 cutset 中包含的所有前导和尾部 Unicode 代码点删除。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Printf("[%q]", strings.Trim(" !!! Achtung! Achtung! !!! ", "! "))
}
func TrimFuncSource
func TrimFunc(s string, f func(rune) bool) string
TrimFunc 返回字符串 s 的一个片段,其中所有前导和尾随 Unicode 代码点 c 都满足 f(c)。
示例
package main
import (
"fmt"
"strings"
"unicode"
)
func main() {
f := func(c rune) bool {
return !unicode.IsLetter(c) && !unicode.IsNumber(c)
}
fmt.Printf("[%q]", strings.TrimFunc(" Achtung1! Achtung2,...", f))
}
func TrimLeftSource
func TrimLeft(s string, cutset string) string
TrimLeft 返回 cutset 中包含的所有前导 Unicode 代码点被删除的字符串 s 的一部分。
func TrimLeftFuncSource
func TrimLeftFunc(s string, f func(rune) bool) string
TrimLeftFunc 返回字符串 s 的一部分,其中所有前导 Unicode 代码点 c 满足 f(c)。
func TrimPrefixSource
func TrimPrefix(s, prefix string) string
TrimPrefix 在没有提供的前导前缀字符串的情况下返回 s。如果 s 不是以前缀开始,则 s 不变。
示例
package main
import (
"fmt"
"strings"
)
func main() {
var s = "Goodbye,, world!"
s = strings.TrimPrefix(s, "Goodbye,")
s = strings.TrimPrefix(s, "Howdy,")
fmt.Print("Hello" + s)
}
func TrimRightSource
func TrimRight(s string, cutset string) string
TrimRight 返回字符串 s 的一部分,删除 cutset 中包含的所有尾部 Unicode 代码点。
func TrimRightFuncSource
func TrimRightFunc(s string, f func(rune) bool) string
TrimRightFunc 返回字符串 s 的一个片段,其中所有的尾随 Unicode 代码点 c 满足 f(c) 。
func TrimSpaceSource
func TrimSpace(s string) string
TrimSpace 返回字符串 s 的一部分,删除所有前导和尾部空白,如 Unicode 定义的。
示例
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(strings.TrimSpace(" \t\n a lone gopher \n\t\r\n"))
}
func TrimSuffixSource
func TrimSuffix(s, suffix string) string
TrimSuffix 在没有提供尾随后缀字符串的情况下返回 s。如果 s 不以后缀结尾,则 s 不变。
示例
package main
import (
"fmt"
"strings"
)
func main() {
var s = "Hello, goodbye, etc!"
s = strings.TrimSuffix(s, "goodbye, etc!")
s = strings.TrimSuffix(s, "planet")
fmt.Print(s, "world!")
}
键入ReaderSource
Reader 通过读取字符串来实现 io.Reader,io.ReaderAt,io.Seeker,io.WriterTo,io.ByteScanner 和 io.RuneScanner 接口。
type Reader struct {
// contains filtered or unexported fields
}
func NewReaderSource
func NewReader(s string) *Reader
NewReader 从 s 中返回一个新的 Reader 读数。它与 bytes.NewBufferString 类似,但更高效且只读。
func (*Reader) LenSource
func (r *Reader) Len() int
Len 返回字符串未读部分的字节数。
func (*Reader) ReadSource
func (r *Reader) Read(b []byte) (n int, err error)
func (*Reader) ReadAtSource
func (r *Reader) ReadAt(b []byte, off int64) (n int, err error)
func (*Reader) ReadByteSource
func (r *Reader) ReadByte() (byte, error)
func (*Reader) ReadRuneSource
func (r *Reader) ReadRune() (ch rune, size int, err error)
func (*Reader) ResetSource
func (r *Reader) Reset(s string)
重置将读取器重置为从 s 读取。
func (*Reader) SeekSource
func (r *Reader) Seek(offset int64, whence int) (int64, error)
Seek 实现了 io.Seeker 接口。
func (*Reader) SizeSource
func (r *Reader) Size() int64
Size 返回基础字符串的原始长度。Size 是可通过 ReadAt 读取的字节数。返回的值总是相同的,不受调用任何其他方法的影响。
func (*Reader) UnreadByteSource
func (r *Reader) UnreadByte() error
func (*Reader) UnreadRuneSource
func (r *Reader) UnreadRune() error
func (*Reader) WriteToSource
func (r *Reader) WriteTo(w io.Writer) (n int64, err error)
WriteTo 实现 io.WriterTo 接口。
键入ReplacerSource
Replacer 用替换替换字符串列表。由多个 goroutines 并行使用是安全的。
type Replacer struct {
// contains filtered or unexported fields
}
func NewReplacerSource
func NewReplacer(oldnew ...string) *Replacer
NewReplacer 从旧的新的字符串对列表中返回一个新的 Replacer。按顺序执行替换,不会有重叠匹配。
示例
package main
import (
"fmt"
"strings"
)
func main() {
r := strings.NewReplacer("<", "<", ">", ">")
fmt.Println(r.Replace("This is <b>HTML</b>!"))
}
func (*Replacer) ReplaceSource
func (r *Replacer) Replace(s string) string
Replace 返回执行所有替换的s的副本。
func (*Replacer) WriteStringSource
func (r *Replacer) WriteString(w io.Writer, s string) (n int, err error)
WriteString 将 s 写入 w 并执行所有替换。
错误
- ☞ 标题用于单词边界的规则不能正确处理 Unicode 标点符号。
字符串 | strings相关
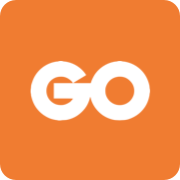
Go 是一种编译型语言,它结合了解释型语言的游刃有余,动态类型语言的开发效率,以及静态类型的安全性。它也打算成为现代的,支持网络与多核计算的语言。要满足这些目标,需要解决一些语言上的问题:一个富有表达能力但轻量级的类型系统,并发与垃圾回收机制,严格的依赖规范等等。这些无法通过库或工具解决好,因此Go也就应运而生了。
主页 | https://golang.org/ |
源码 | https://go.googlesource.com/go |
发布版本 | 1.9.2 |