Go参考手册
net/rpc
net/rpc
import "net/rpc"
- 概况
- 索引
- 子目录
概况
软件包 rpc 通过网络或其他 I/O 连接提供对对象的导出方法的访问。服务器注册一个对象,使其作为一个服务显示为对象类型的名称。注册后,对象的导出方法将可以远程访问。服务器可以注册多个不同类型的对象(服务),但注册多个相同类型的对象是错误的。
只有符合这些标准的方法才能用于远程访问; 其他方法将被忽略:
- the method's type is exported.
- the method is exported.
- the method has two arguments, both exported (or builtin) types.
- the method's second argument is a pointer.
- the method has return type error.
实际上,该方法必须看起来像
func (t *T) MethodName(argType T1, replyType *T2) error
T1 和 T2 可以通过 encoding/gob 进行编组。即使使用不同的编解码器,这些要求也适用。(将来,这些要求可能会因为自定义编解码器而变差。)
该方法的第一个参数表示由调用者提供的参数; 第二个参数表示要返回给调用者的结果参数。如果非零,方法的返回值作为客户端看到的字符串传回,就像由 errors.New 创建的那样。如果返回错误,答复参数将不会被发送回客户端。
服务器可以通过调用 ServeConn 来处理单个连接上的请求。更典型的是,它将创建一个网络监听器并调用 Accept,或者对于 HTTP 监听器 HandleHTTP 和 http.Serve 。
客户希望使用该服务建立连接,然后在连接上调用 NewClient 。便捷功能 Dial(DialHTTP)执行原始网络连接(HTTP 连接)的两个步骤。生成的 Client 对象具有 Call 和 Go 两个方法,它们指定要调用的服务和方法,包含参数的指针以及用于接收结果参数的指针。
Call 方法等待远程调用完成,而 Go 方法异步启动调用,并使用 Call 结构的完成通道发送完成信号。
除非设置了明确的编解码器,否则使用包 encoding / gob 传输数据。
这是一个简单的例子。服务器希望导出 Arith 类型的对象:
package server
import "errors"
type Args struct {
A, B int
}
type Quotient struct {
Quo, Rem int
}
type Arith int
func (t *Arith) Multiply(args *Args, reply *int) error {
*reply = args.A * args.B
return nil
}
func (t *Arith) Divide(args *Args, quo *Quotient) error {
if args.B == 0 {
return errors.New("divide by zero")
}
quo.Quo = args.A / args.B
quo.Rem = args.A % args.B
return nil
}
服务器调用(对于 HTTP 服务):
arith := new(Arith)
rpc.Register(arith)
rpc.HandleHTTP()
l, e := net.Listen("tcp", ":1234")
if e != nil {
log.Fatal("listen error:", e)
}
go http.Serve(l, nil)
此时,客户可以使用“Arith.Multiply”和“Arith.Divide”方法查看“Arith”服务。要调用它,客户端首先拨打服务器:
client, err := rpc.DialHTTP("tcp", serverAddress + ":1234")
if err != nil {
log.Fatal("dialing:", err)
}
然后它可以进行远程调用:
// Synchronous call
args := &server.Args{7,8}
var reply int
err = client.Call("Arith.Multiply", args, &reply)
if err != nil {
log.Fatal("arith error:", err)
}
fmt.Printf("Arith: %d*%d=%d", args.A, args.B, reply)
或
// Asynchronous call
quotient := new(Quotient)
divCall := client.Go("Arith.Divide", args, quotient, nil)
replyCall := <-divCall.Done // will be equal to divCall
// check errors, print, etc.
服务器实现通常会为客户端提供一个简单的,类型安全的包装器。
net / rpc 软件包被冻结,不接受新的功能。
索引
- 常量
- 变量
- func Accept(lis net.Listener)
- func HandleHTTP()
- func Register(rcvr interface{}) error
- func RegisterName(name string, rcvr interface{}) error
- func ServeCodec(codec ServerCodec)
- func ServeConn(conn io.ReadWriteCloser)
- func ServeRequest(codec ServerCodec) error
- type Call
- type Client
- func Dial(network, address string) (*Client, error)
- func DialHTTP(network, address string) (*Client, error)
- func DialHTTPPath(network, address, path string) (*Client, error)
- func NewClient(conn io.ReadWriteCloser) *Client
- func NewClientWithCodec(codec ClientCodec) *Client
- func (client *Client) Call(serviceMethod string, args interface{}, reply interface{}) error
- func (client *Client) Close() error
- func (client *Client) Go(serviceMethod string, args interface{}, reply interface{}, done chan *Call) *Call
- type ClientCodec
- type Request
- type Response
- type Server
- func NewServer() *Server
- func (server *Server) Accept(lis net.Listener)
- func (server *Server) HandleHTTP(rpcPath, debugPath string)
- func (server *Server) Register(rcvr interface{}) error
- func (server *Server) RegisterName(name string, rcvr interface{}) error
- func (server *Server) ServeCodec(codec ServerCodec)
- func (server *Server) ServeConn(conn io.ReadWriteCloser)
- func (server *Server) ServeHTTP(w http.ResponseWriter, req *http.Request)
- func (server *Server) ServeRequest(codec ServerCodec) error
- type ServerCodec
- type ServerError
- func (e ServerError) Error() string
包文件
client.go debug.go server.go
常量
const (
// Defaults used by HandleHTTP
DefaultRPCPath = "/_goRPC_"
DefaultDebugPath = "/debug/rpc"
)
变量
DefaultServer 是 * Server 的默认实例。
var DefaultServer = NewServer()
var ErrShutdown = errors.New("connection is shut down")
func Accept(显示源文件)
func Accept(lis net.Listener)
Accept 接受监听器上的连接,并为每个传入连接向 DefaultServer 提供请求。接受块; 调用者通常在 go 语句中调用它。
func HandleHTTP(显示源文件)
func HandleHTTP()
HandleHTTP 在 DefaultRPCPath 上向 DefaultServer 注册 RPC 消息的 HTTP 处理程序,并在 DefaultDebugPath 上注册一个调试处理程序。仍然需要调用 http.Serve(),通常在 go 语句中。
func Register(显示源文件)
func Register(rcvr interface{}) error
Register 在 DefaultServer 中发布接收者的方法。
func RegisterName(显示源文件)
func RegisterName(name string, rcvr interface{}) error
RegisterName 与 Register 类似,但使用提供的名称来代替接收者的具体类型。
func ServeCodec(显示源文件)
func ServeCodec(codec ServerCodec)
ServeCodec 就像 ServeConn,但使用指定的编解码器来解码请求并对响应进行编码。
func ServeConn(显示源文件)
func ServeConn(conn io.ReadWriteCloser)
ServeConn 在一个连接上运行 DefaultServer。ServeConn 块,服务于连接,直到客户端挂断。调用者通常在 go 语句中调用 ServeConn 。ServeConn 在连接上使用 gob wire 格式(请参阅软件包 gob)。要使用备用编解码器,请使用 ServeCodec 。
func ServeRequest(显示源文件)
func ServeRequest(codec ServerCodec) error
ServeRequest 与 ServeCodec 类似,但同步提供单个请求。完成后它不会关闭编解码器。
type Call(显示源文件)
Call 表示一个活动的 RPC 。
type Call struct {
ServiceMethod string // The name of the service and method to call.
Args interface{} // The argument to the function (*struct).
Reply interface{} // The reply from the function (*struct).
Error error // After completion, the error status.
Done chan *Call // Strobes when call is complete.
}
type Client(显示源文件)
Client 代表一个 RPC 客户端。可能有多个与一个客户端相关的未决呼叫,并且一个客户端可能会被多个 goroutine 同时使用。
type Client struct {
// contains filtered or unexported fields
}
func Dial(显示源文件)
func Dial(network, address string) (*Client, error)
Dial 连接到指定网络地址的 RPC 服务器。
func DialHTTP(显示源文件)
func DialHTTP(network, address string) (*Client, error)
DialHTTP 连接到指定网络地址的 HTTP RPC 服务器,该地址监听默认的 HTTP RPC 路径。
func DialHTTPPath(显示源文件)
func DialHTTPPath(network, address, path string) (*Client, error)
DialHTTPPath 通过指定的网络地址和路径连接到 HTTP RPC 服务器。
func NewClient(显示源文件)
func NewClient(conn io.ReadWriteCloser) *Client
NewClient 返回一个新的客户端来处理对连接另一端的一组服务的请求。它向连接的写入端添加一个缓冲区,以便将 header 和 payload 作为一个单元发送。
func NewClientWithCodec(显示源文件)
func NewClientWithCodec(codec ClientCodec) *Client
NewClientWithCodec 与 NewClient 类似,但使用指定的编解码器对请求进行编码和解码响应。
func (*Client) Call(显示源文件)
func (client *Client) Call(serviceMethod string, args interface{}, reply interface{}) error
Call 调用指定的函数,等待它完成,并返回其错误状态。
func (*Client) Close(显示源文件)
func (client *Client) Close() error
Close 调用底层编解码器的 Close 方法。如果连接已关闭,则返回 ErrShutdown 。
func (*Client) Go(显示源文件)
func (client *Client) Go(serviceMethod string, args interface{}, reply interface{}, done chan *Call) *Call
Go 异步调用该函数。它返回表示调用的 Call 结构。完成通道将通过返回相同的呼叫对象在呼叫完成时发出信号。如果完成,则 Go 将分配一个新频道。如果非零,则必须进行缓冲或 Go 会故意崩溃。
type ClientCodec(显示源文件)
ClientCodec 实现 RPC 请求的写入和读取 RPC 会话客户端的 RPC 响应。客户端调用 WriteRequest 向连接写入请求,并成对调用 ReadResponseHeader 和 ReadResponseBody 以读取响应。客户在完成连接后调用 Close 。可以使用零参数调用 ReadResponseBody,以强制读取响应主体并丢弃。
type ClientCodec interface {
// WriteRequest must be safe for concurrent use by multiple goroutines.
WriteRequest(*Request, interface{}) error
ReadResponseHeader(*Response) error
ReadResponseBody(interface{}) error
Close() error
}
type Request(显示源文件)
Request 是每个 RPC 调用之前写入的头文件。它在内部使用,但在此处记录为对调试的帮助,例如分析网络流量时。
type Request struct {
ServiceMethod string // format: "Service.Method"
Seq uint64 // sequence number chosen by client
// contains filtered or unexported fields
}
type Response(显示源文件)
Response 是每个 RPC 返回之前写入的头文件。它在内部使用,但在此处记录为对调试的帮助,例如分析网络流量时。
type Response struct {
ServiceMethod string // echoes that of the Request
Seq uint64 // echoes that of the request
Error string // error, if any.
// contains filtered or unexported fields
}
type Server(显示源文件)
Server 代表一个 RPC 服务器。
type Server struct {
// contains filtered or unexported fields
}
func NewServer(显示源文件)
func NewServer() *Server
NewServer 返回一个新的服务器。
func (*Server) Accept(显示源文件)
func (server *Server) Accept(lis net.Listener)
Accept 接受侦听器上的连接并为每个传入连接提供请求。接受阻塞,直到侦听器返回非零错误。调用者通常在 go 语句中调用 Accept 。
func (*Server) HandleHTTP(显示源文件)
func (server *Server) HandleHTTP(rpcPath, debugPath string)
HandleHTTP 为 rpcPath 上的 RPC 消息注册一个 HTTP 处理程序,并在 debugPath 上注册一个调试处理程序。仍然需要调用 http.Serve(),通常在 go 语句中。
func (*Server) Register(显示源文件)
func (server *Server) Register(rcvr interface{}) error
Register 在服务器中发布满足以下条件的接收器值的方法集合:
- exported method of exported type
- two arguments, both of exported type
- the second argument is a pointer
- one return value, of type error
如果接收方不是导出类型或没有合适的方法,它将返回一个错误。它还使用包日志记录错误。客户端使用“Type.Method”形式的字符串访问每个方法,其中 Type 是接收者的具体类型。
func (*Server) RegisterName(显示源文件)
func (server *Server) RegisterName(name string, rcvr interface{}) error
RegisterName 与 Register 类似,但使用提供的名称来代替接收者的具体类型。
func (*Server) ServeCodec(显示源文件)
func (server *Server) ServeCodec(codec ServerCodec)
ServeCodec 就像 ServeConn,但使用指定的编解码器来解码请求并对响应进行编码。
func (*Server) ServeConn(显示源文件)
func (server *Server) ServeConn(conn io.ReadWriteCloser)
ServeConn 在单个连接上运行服务器。ServeConn 块,服务于连接,直到客户端挂断。调用者通常在 go 语句中调用 ServeConn 。ServeConn 在连接上使用 gob wire 格式(请参阅软件包 gob)。要使用备用编解码器,请使用 ServeCodec 。
func (*Server) ServeHTTP(显示源文件)
func (server *Server) ServeHTTP(w http.ResponseWriter, req *http.Request)
ServeHTTP 实现了一个应答 RPC 请求的 http.Handler 。
func (*Server) ServeRequest(显示源文件)
func (server *Server) ServeRequest(codec ServerCodec) error
ServeRequest 与 ServeCodec 类似,但同步提供单个请求。完成后它不会关闭编解码器。
type ServerCodec(显示源文件)
ServerCodec 实现读取 RPC 请求并为 RPC 会话的服务器端写入 RPC 响应。服务器成对调用 ReadRequestHeader 和 ReadRequestBody 来读取来自连接的请求,并且它调用 WriteResponse 来写回响应。服务器在连接完成后调用 Close 。可以使用零参数调用 ReadRequestBody 来强制请求的主体被读取和丢弃。
type ServerCodec interface {
ReadRequestHeader(*Request) error
ReadRequestBody(interface{}) error
// WriteResponse must be safe for concurrent use by multiple goroutines.
WriteResponse(*Response, interface{}) error
Close() error
}
type ServerError(显示源文件)
ServerError 表示从 RPC 连接的远程端返回的错误。
type ServerError string
func (ServerError) Error(显示源文件)
func (e ServerError) Error() string
子目录
Name |
Synopsis |
---|---|
jsonrpc |
包 jsonrpc 为 rpc 包实现了一个 JSON-RPC 1.0 ClientCodec 和 ServerCodec |
net/rpc相关
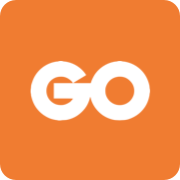
Go 是一种编译型语言,它结合了解释型语言的游刃有余,动态类型语言的开发效率,以及静态类型的安全性。它也打算成为现代的,支持网络与多核计算的语言。要满足这些目标,需要解决一些语言上的问题:一个富有表达能力但轻量级的类型系统,并发与垃圾回收机制,严格的依赖规范等等。这些无法通过库或工具解决好,因此Go也就应运而生了。
主页 | https://golang.org/ |
源码 | https://go.googlesource.com/go |
发布版本 | 1.9.2 |