Go参考手册
数据库 | database
数据库/sql | database/sql
import "database/sql"
- 概述
- 索引
- 示例
- 子目录
概述
Package sql 提供了一个围绕SQL(或类似SQL)数据库的通用接口。
sql 包必须与数据库驱动程序一起使用。查看https://golang.org/s/sqldrivers获取驱动程序列表。
不支持上下文取消的驱动程序直到查询完成后才会返回。
有关使用示例,请参阅https://golang.org/s/sqlwiki上的wiki页面。
索引
- Variables
- func Drivers() []string
- func Register(name string, driver driver.Driver)
- type ColumnType
- func (ci *ColumnType) DatabaseTypeName() string
- func (ci *ColumnType) DecimalSize() (precision, scale int64, ok bool)
- func (ci *ColumnType) Length() (length int64, ok bool)
- func (ci *ColumnType) Name() string
- func (ci *ColumnType) Nullable() (nullable, ok bool)
- func (ci *ColumnType) ScanType() reflect.Type
- type Conn
- func (c *Conn) BeginTx(ctx context.Context, opts *TxOptions) (*Tx, error)
- func (c *Conn) Close() error
- func (c *Conn) ExecContext(ctx context.Context, query string, args ...interface{}) (Result, error)
- func (c *Conn) PingContext(ctx context.Context) error
- func (c *Conn) PrepareContext(ctx context.Context, query string) (*Stmt, error)
- func (c *Conn) QueryContext(ctx context.Context, query string, args ...interface{}) (*Rows, error)
- func (c *Conn) QueryRowContext(ctx context.Context, query string, args ...interface{}) *Row
- type DB
- func Open(driverName, dataSourceName string) (*DB, error)
- func (db *DB) Begin() (*Tx, error)
- func (db *DB) BeginTx(ctx context.Context, opts *TxOptions) (*Tx, error)
- func (db *DB) Close() error
- func (db *DB) Conn(ctx context.Context) (*Conn, error)
- func (db *DB) Driver() driver.Driver
- func (db *DB) Exec(query string, args ...interface{}) (Result, error)
- func (db *DB) ExecContext(ctx context.Context, query string, args ...interface{}) (Result, error)
- func (db *DB) Ping() error
- func (db *DB) PingContext(ctx context.Context) error
- func (db *DB) Prepare(query string) (*Stmt, error)
- func (db *DB) PrepareContext(ctx context.Context, query string) (*Stmt, error)
- func (db *DB) Query(query string, args ...interface{}) (*Rows, error)
- func (db *DB) QueryContext(ctx context.Context, query string, args ...interface{}) (*Rows, error)
- func (db *DB) QueryRow(query string, args ...interface{}) *Row
- func (db *DB) QueryRowContext(ctx context.Context, query string, args ...interface{}) *Row
- func (db *DB) SetConnMaxLifetime(d time.Duration)
- func (db *DB) SetMaxIdleConns(n int)
- func (db *DB) SetMaxOpenConns(n int)
- func (db *DB) Stats() DBStats
- type DBStats
- type IsolationLevel
- type NamedArg
- func Named(name string, value interface{}) NamedArg
- type NullBool
- func (n *NullBool) Scan(value interface{}) error
- func (n NullBool) Value() (driver.Value, error)
- type NullFloat64
- func (n *NullFloat64) Scan(value interface{}) error
- func (n NullFloat64) Value() (driver.Value, error)
- type NullInt64
- func (n *NullInt64) Scan(value interface{}) error
- func (n NullInt64) Value() (driver.Value, error)
- type NullString
- func (ns *NullString) Scan(value interface{}) error
- func (ns NullString) Value() (driver.Value, error)
- type Out
- type RawBytes
- type Result
- type Row
- func (r *Row) Scan(dest ...interface{}) error
- type Rows
- func (rs *Rows) Close() error
- func (rs *Rows) ColumnTypes() ([]*ColumnType, error)
- func (rs *Rows) Columns() ([]string, error)
- func (rs *Rows) Err() error
- func (rs *Rows) Next() bool
- func (rs *Rows) NextResultSet() bool
- func (rs *Rows) Scan(dest ...interface{}) error
- type Scanner
- type Stmt
- func (s *Stmt) Close() error
- func (s *Stmt) Exec(args ...interface{}) (Result, error)
- func (s *Stmt) ExecContext(ctx context.Context, args ...interface{}) (Result, error)
- func (s *Stmt) Query(args ...interface{}) (*Rows, error)
- func (s *Stmt) QueryContext(ctx context.Context, args ...interface{}) (*Rows, error)
- func (s *Stmt) QueryRow(args ...interface{}) *Row
- func (s *Stmt) QueryRowContext(ctx context.Context, args ...interface{}) *Row
- type Tx
- func (tx *Tx) Commit() error
- func (tx *Tx) Exec(query string, args ...interface{}) (Result, error)
- func (tx *Tx) ExecContext(ctx context.Context, query string, args ...interface{}) (Result, error)
- func (tx *Tx) Prepare(query string) (*Stmt, error)
- func (tx *Tx) PrepareContext(ctx context.Context, query string) (*Stmt, error)
- func (tx *Tx) Query(query string, args ...interface{}) (*Rows, error)
- func (tx *Tx) QueryContext(ctx context.Context, query string, args ...interface{}) (*Rows, error)
- func (tx *Tx) QueryRow(query string, args ...interface{}) *Row
- func (tx *Tx) QueryRowContext(ctx context.Context, query string, args ...interface{}) *Row
- func (tx *Tx) Rollback() error
- func (tx *Tx) Stmt(stmt *Stmt) *Stmt
- func (tx *Tx) StmtContext(ctx context.Context, stmt *Stmt) *Stmt
- type TxOptions
示例
DB.Query DB.QueryRow DB.Query (MultipleResultSets)
包文件
convert.go ctxutil.go sql.go
变量
在已经提交或回滚的连接上执行的任何操作都会返回 ErrConnDone。
var ErrConnDone = errors.New("database/sql: connection is already closed")
当 QueryRow 没有返回行时,Scan 返回 ErrNoRows。在这种情况下,QueryRow 会返回一个占位符* Row 值,该值将推迟发生此错误直到扫描。
var ErrNoRows = errors.New("sql: no rows in result set")
对已经提交或回滚的事务执行的任何操作都会返回 ErrTxDone。
var ErrTxDone = errors.New("sql: Transaction has already been committed or rolled back")
func Drivers(查看源代码)
func Drivers() []string
驱动程序返回已注册驱动程序名称的排序列表。
func Register(查看源代码)
func Register(name string, driver driver.Driver)
Register 使用提供的名称提供数据库驱动程序。如果使用相同的名称调用 Register 两次,或者驱动程序为零,则会发生混乱。
type ColumnType(查看源代码)
ColumnType 包含列的名称和类型。
type ColumnType struct {
// 包含过滤或未导出的字段
}
func (*ColumnType) DatabaseTypeName(查看源代码)
func (ci *ColumnType) DatabaseTypeName() string
DatabaseTypeName 返回列类型的数据库系统名称。如果返回空字符串,则不支持驱动程序类型名称。请查阅您的驱动程序文档以获取驱动程序数据类型列表 不包括长度说明符。常见类型包括 “VARCHAR”,“TEXT”,“NVARCHAR”,“DECIMAL”,“BOOL”,“INT”,“BIGINT”。
func (*ColumnType) DecimalSize(查看源代码)
func (ci *ColumnType) DecimalSize() (precision, scale int64, ok bool)
DecimalSize 返回小数类型的比例和精度。如果不适用或者不支持,那么ok 是错误的。
func (*ColumnType) Length(查看源代码)
func (ci *ColumnType) Length() (length int64, ok bool)
Length 返回可变长度列类型(如文本和二进制字段类型)的列类型长度。如果类型长度没有限制,则数值为 math.MaxInt64(任何数据库限制仍将适用)。如果列类型不是可变长度,例如 int,或者如果驱动程序不支持,那么 ok 是 false。
func (*ColumnType) Name(查看源代码)
func (ci *ColumnType) Name() string
Name 返回列的名称或别名。
func (*ColumnType) Nullable(查看源代码)
func (ci *ColumnType) Nullable() (nullable, ok bool)
Nullable 返回列是否可以为 null。如果一个驱动程序不支持这个属性,ok 将会是 false。
func (*ColumnType) ScanType(查看源代码)
func (ci *ColumnType) ScanType() reflect.Type
ScanType 使用 Rows.Scan 返回适合扫描的 Go 类型。如果驱动程序不支持此属性,ScanType 将返回空接口的类型。
type Conn(查看源代码)
Conn 代表一个数据库会话而不是一个数据库会话池。除非特别需要连续的单个数据库会话,否则首选运行 DB 查询。
Conn 必须调用 Close 来将连接返回到数据库池,并且可以与正在运行的查询同时进行。
在调用 Close 之后,连接上的所有操作都会因 ErrConnDone 而失败。
type Conn struct {
// 包含过滤或未导出的字段
}
func (*Conn) BeginTx(查看源代码)
func (c *Conn) BeginTx(ctx context.Context, opts *TxOptions) (*Tx, error)
BeginTx 开始一个交易。
提供的上下文用于事务提交或回滚之前。如果上下文被取消,sql包将回滚事务。如果提供给 BeginTx 的上下文被取消,则Tx.Commit 将返回错误。
提供的 TxOptions 是可选的,如果应该使用默认值,则可能为零。如果使用驱动程序不支持的非默认隔离级别,则会返回错误。
func (*Conn) Close(查看源代码)
func (c *Conn) Close() error
Close 将连接返回到连接池。Close 后的所有操作都将返回ErrConnDone。Close 是安全地与其他操作同时进行,并会阻止,直到所有其他操作完成。首先取消使用的上下文,然后直接调用 close 可能会很有用。
func (*Conn) ExecContext(查看源代码)
func (c *Conn) ExecContext(ctx context.Context, query string, args ...interface{}) (Result, error)
ExecContext 执行查询而不返回任何行。该参数适用于查询中的任何占位符参数。
func (*Conn) PingContext(查看源代码)
func (c *Conn) PingContext(ctx context.Context) error
PingContext 验证到数据库的连接是否仍然存在。
func (*Conn) PrepareContext(查看源代码)
func (c *Conn) PrepareContext(ctx context.Context, query string) (*Stmt, error)
PrepareContext 为以后的查询或执行创建一个准备好的语句。可以从返回的语句中同时运行多个查询或执行。当语句不再需要时,调用者必须调用语句的 Close 方法。
提供的上下文用于编写声明,而不用于执行声明。
func (*Conn) QueryContext(查看源代码)
func (c *Conn) QueryContext(ctx context.Context, query string, args ...interface{}) (*Rows, error)
QueryContext 执行一个返回行的查询,通常是一个 SELECT。该参数适用于查询中的任何占位符参数。
func (*Conn) QueryRowContext(查看源代码)
func (c *Conn) QueryRowContext(ctx context.Context, query string, args ...interface{}) *Row
QueryRowContext 执行一个预计最多只返回一行的查询。QueryRowContext 总是返回一个非零值。错误被推迟到行的扫描方法被调用。如果查询未选择行,则*Row 的扫描将返回错误号。否则,*Row 的扫描将扫描第一个选定的行并丢弃其余行。
type DB(查看源代码)
DB是一个数据库句柄,表示一个包含零个或多个底层连接的池。多个 goroutine 并发使用是安全的。
sql 包自动创建并释放连接;它也保持空闲连接的空闲池。如果数据库具有每个连接状态的概念,则只能在事务内可靠地观察到这种状态。一旦 DB.Begin 被调用,返回的 Tx 就绑定到单个连接。在事务上调用 Commit 或 Rollback 后,该事务的连接将返回到数据库的空闲连接池。池的大小可以通过 SetMaxIdleConns 进行控制。
type DB struct {
// 包含过滤或未导出的字段
}
func Open(查看源代码)
func Open(driverName, dataSourceName string) (*DB, error)
Open 打开一个由其数据库驱动程序名称和驱动程序特定的数据源名称指定的数据库,通常由至少一个数据库名称和连接信息组成。
大多数用户将通过驱动程序特定的连接帮助程序函数打开数据库,该函数返回一个 *DB。Go标准库中不包含数据库驱动程序。有关第三方驱动程序的列表,请参阅https://golang.org/s/sqldrivers。
Open 可能只是验证其参数而不创建与数据库的连接。要验证数据源名称是否有效,请调用 Ping。
返回的数据库对于多个 goroutine 的并发使用是安全的,并保持其自己的空闲连接池。因此,Open 函数应该只调用一次。很少有必要关闭数据库。
func (*DB) Begin(查看源代码)
func (db *DB) Begin() (*Tx, error)
Begin 开始一个交易。默认隔离级别取决于驱动程序。
func (*DB) BeginTx(查看源代码)
func (db *DB) BeginTx(ctx context.Context, opts *TxOptions) (*Tx, error)
BeginTx 开始一个交易。
提供的上下文用于事务提交或回滚之前。如果上下文被取消,sql包将回滚事务。如果提供给 BeginTx 的上下文被取消,则Tx.Commit 将返回错误。
提供的 TxOptions 是可选的,如果应该使用默认值,则可能为零。如果使用驱动程序不支持的非默认隔离级别,则会返回错误。
func (*DB) Close(查看源代码)
func (db *DB) Close() error
Close 关闭数据库,释放所有打开的资源。
关闭数据库是很少见的,因为数据库处理意味着很长寿命并且在许多 goroutine 之间共享。
func (*DB) Conn(查看源代码)
func (db *DB) Conn(ctx context.Context) (*Conn, error)
Conn 通过打开新连接或从连接池返回现有连接返回单个连接。Conn 将阻塞,直到连接返回或 ctx 被取消。在同一个 Conn 上运行的查询将在同一个数据库会话中运行。
通过调用 Conn.Close,每个 Conn 必须在使用后返回到数据库池。
func (*DB) Driver(查看源代码)
func (db *DB) Driver() driver.Driver
Driver 返回数据库的底层驱动程序。
func (*DB) Exec(查看源代码)
func (db *DB) Exec(query string, args ...interface{}) (Result, error)
Exec 执行查询而不返回任何行。该参数适用于查询中的任何占位符参数。
func (*DB) ExecContext(查看源代码)
func (db *DB) ExecContext(ctx context.Context, query string, args ...interface{}) (Result, error)
ExecContext 执行查询而不返回任何行。该参数适用于查询中的任何占位符参数。
func (*DB) Ping(查看源代码)
func (db *DB) Ping() error
Ping 验证与数据库的连接仍然存在,并在必要时建立连接。
func (*DB) PingContext(查看源代码)
func (db *DB) PingContext(ctx context.Context) error
PingContext 验证与数据库的连接是否仍然存在,如果需要的话建立连接。
func (*DB) Prepare(查看源代码)
func (db *DB) Prepare(query string) (*Stmt, error)
Prepare 为以后的查询或执行创建一个准备好的语句。可以从返回的语句中同时运行多个查询或执行。当语句不再需要时,调用者必须调用语句的 Close 方法。
func (*DB) PrepareContext(查看源代码)
func (db *DB) PrepareContext(ctx context.Context, query string) (*Stmt, error)
PrepareContext 为以后的查询或执行创建一个准备好的语句。可以从返回的语句中同时运行多个查询或执行。当语句不再需要时,调用者必须调用语句的 Close 方法。
提供的上下文用于编写声明,而不用于执行声明。
func (*DB) Query(查看源代码)
func (db *DB) Query(query string, args ...interface{}) (*Rows, error)
查询执行一个返回行的查询,通常是一个 SELECT。该参数适用于查询中的任何占位符参数。
示例
代码:
age := 27
rows, err := db.Query("SELECT name FROM users WHERE age=?", age)
if err != nil {
log.Fatal(err)
}
defer rows.Close()
for rows.Next() {
var name string
if err := rows.Scan(&name); err != nil {
log.Fatal(err)
}
fmt.Printf("%s is %d\n", name, age)
}
if err := rows.Err(); err != nil {
log.Fatal(err)
}
示例(MultipleResultSets)
代码:
age := 27
q := `
create temp table uid (id bigint); -- Create temp table for queries.
insert into uid
select id from users where age < ?; -- Populate temp table.
-- First result set.
select
users.id, name
from
users
join uid on users.id = uid.id
;
-- Second result set.
select
ur.user, ur.role
from
user_roles as ur
join uid on uid.id = ur.user
;
`
rows, err := db.Query(q, age)
if err != nil {
log.Fatal(err)
}
defer rows.Close()
for rows.Next() {
var (
id int64
name string
)
if err := rows.Scan(&id, &name); err != nil {
log.Fatal(err)
}
fmt.Printf("id %d name is %s\n", id, name)
}
if !rows.NextResultSet() {
log.Fatal("expected more result sets", rows.Err())
}
var roleMap = map[int64]string{
1: "user",
2: "admin",
3: "gopher",
}
for rows.Next() {
var (
id int64
role int64
)
if err := rows.Scan(&id, &role); err != nil {
log.Fatal(err)
}
fmt.Printf("id %d has role %s\n", id, roleMap[role])
}
if err := rows.Err(); err != nil {
log.Fatal(err)
}
func (*DB) QueryContext(查看源代码)
func (db *DB) QueryContext(ctx context.Context, query string, args ...interface{}) (*Rows, error)
QueryContext 执行一个返回行的查询,通常是一个 SELECT。该参数适用于查询中的任何占位符参数。
func (*DB) QueryRow(查看源代码)
func (db *DB) QueryRow(query string, args ...interface{}) *Row
QueryRow 执行一个查询,该查询最多只返回一行。QueryRow总是返回一个非零值。错误被推迟到行的扫描方法被调用。如果查询未选择行,则*Row 的扫描将返回错误号。否则,*Row 的扫描将扫描第一个选定的行并丢弃其余行。
示例
代码:
id := 123
var username string
err := db.QueryRow("SELECT username FROM users WHERE id=?", id).Scan(&username)
switch {
case err == sql.ErrNoRows:
log.Printf("No user with that ID.")
case err != nil:
log.Fatal(err)
default:
fmt.Printf("Username is %s\n", username)
}
func (*DB) QueryRowContext(查看源代码)
func (db *DB) QueryRowContext(ctx context.Context, query string, args ...interface{}) *Row
QueryRowContext 执行一个预计最多只返回一行的查询。QueryRowContext 总是返回一个非零值。错误被推迟到行的扫描方法被调用。如果查询未选择行,则*Row 的扫描将返回错误号。否则,*Row 的扫描将扫描第一个选定的行并丢弃其余行。
func (*DB) SetConnMaxLifetime(查看源代码)
func (db *DB) SetConnMaxLifetime(d time.Duration)
SetConnMaxLifetime 设置连接可以被重用的最大时间量。
过期的连接可能会在重用之前被缓慢地关闭。
如果d <= 0,连接将永久重用。
func (*DB) SetMaxIdleConns(查看源代码)
func (db *DB) SetMaxIdleConns(n int)
SetMaxIdleConns 设置空闲连接池中的最大连接数。
如果 MaxOpenConns 大于0但小于新的 MaxIdleConns,那么新的 MaxIdleConns 将减少以匹配 MaxOpenConns 限制
如果n <= 0,则不保留空闲连接。
func (*DB) SetMaxOpenConns(查看源代码)
func (db *DB) SetMaxOpenConns(n int)
SetMaxOpenConns 设置打开到数据库的最大连接数。
如果 MaxIdleConns 大于0并且新的 MaxOpenConns 小于MaxIdleConns,则 MaxIdleConns 将减少以匹配新的MaxOpenConns 限制
如果n <= 0,那么打开连接的数量没有限制。默认值是0(无限制)。
func (*DB) Stats(查看源代码)
func (db *DB) Stats() DBStats
Stats 返回数据库统计信息
type DBStats(查看源代码)
DBStats 包含数据库统计信息。
type DBStats struct {
// OpenConnections是打开连接到数据库的数量。
OpenConnections int
}
type IsolationLevel(查看源代码)
IsolationLevel 是 TxOptions 中使用的事务隔离级别。
type IsolationLevel int
BeginTx 中驱动程序可能支持的各种隔离级别。如果驱动程序不支持给定的隔离级别,则可能会返回错误。
参见https://en.wikipedia.org/wiki/Isolation_(database_systems)#Isolation_levels(https://en.wikipedia.org/wiki/Isolation_(database_systems%29个#Isolation_levels)。
const (
LevelDefault IsolationLevel = iota
LevelReadUncommitted
LevelReadCommitted
LevelWriteCommitted
LevelRepeatableRead
LevelSnapshot
LevelSerializable
LevelLinearizable
)
type NamedArg(查看源代码)
NamedArg 是一个命名参数。NamedArg 值可以用作 Query 或Exec 的参数,并绑定到 SQL 语句中的相应命名参数。
有关创建 NamedArg 值的更简洁的方法,请参阅 Named 函数。
type NamedArg struct {
// Name是参数占位符的名称。
//
// 如果为空,参数列表中的序号位置将为
// 被使用。
//
// 名称必须省略任何符号前缀。
Name string
// 值是参数的值。
// 它可以被分配与查询相同的值类型
// 参数。
Value interface{}
// 包含过滤或未导出的字段
}
func Named(查看源代码)
func Named(name string, value interface{}) NamedArg
Named 提供了一种更简洁的方式来创建 NamedArg 值。
用法示例:
db.ExecContext(ctx, `
delete from Invoice
where
TimeCreated < @end
and TimeCreated >= @start;`,
sql.Named("start", startTime),
sql.Named("end", endTime),
)
type NullBool(查看源代码)
NullBool 表示一个可能为 null 的布尔值。NullBool 实现了Scanner 接口,因此它可以用作扫描目标,类似于 NullString。
type NullBool struct {
Bool bool
Valid bool // 如果Bool不为NULL,则有效为真
}
func (*NullBool) Scan(查看源代码)
func (n *NullBool) Scan(value interface{}) error
Scan 实现扫描仪界面。
func (NullBool) Value(查看源代码)
func (n NullBool) Value() (driver.Value, error)
Value 实现了驱动 Valuer 接口。
type NullFloat64(查看源代码)
NullFloat64 表示可能为 null 的 float64。NullFloat64 实现了扫描器接口,因此它可以用作扫描目标,类似于 NullString。
type NullFloat64 struct {
Float64 float64
Valid bool //如果Float64不为NULL,则有效
}
func (*NullFloat64) Scan(查看源代码)
func (n *NullFloat64) Scan(value interface{}) error
Scan 实现扫描仪界面。
func (NullFloat64) Value(查看源代码)
func (n NullFloat64) Value() (driver.Value, error)
Value 实现了驱动 Valuer 接口。
type NullInt64(查看源代码)
NullInt64 代表可能为 null 的 int64。NullInt64 实现了扫描器接口,因此它可以用作扫描目标,类似于 NullString。
type NullInt64 struct {
Int64 int64
Valid bool // 如果Int64不为NULL,则有效为真
}
func (*NullInt64) Scan(查看源代码)
func (n *NullInt64) Scan(value interface{}) error
Scan 实现扫描仪界面。
func (NullInt64) Value(查看源代码)
func (n NullInt64) Value() (driver.Value, error)
Value 实现了驱动 Valuer 接口。
type NullString(查看源代码)
NullString 表示一个可能为空的字符串。NullString 实现了扫描器接口,因此它可以用作扫描目标:
var s NullString
err := db.QueryRow("SELECT name FROM foo WHERE id=?", id).Scan(&s)
...
if s.Valid {
// 使用 s.String
} else {
// NULL值(空值)
}
type NullString struct {
String string
Valid bool // 如果String不为NULL,则有效值为true
}
func (*NullString) Scan(查看源代码)
func (ns *NullString) Scan(value interface{}) error
Scan 实现扫描仪界面。
func (NullString) Value(查看源代码)
func (ns NullString) Value() (driver.Value, error)
Value 实现了驱动 Valuer 接口。
type Out(查看源代码)
Out 可用于从存储过程中检索 OUTPUT 值参数。
并非所有驱动程序和数据库都支持 OUTPUT 值参数。
用法示例:
var outArg string
_, err := db.ExecContext(ctx, "ProcName", sql.Named("Arg1", Out{Dest: &outArg}))
type Out struct {
// Dest是指向将被设置为结果的值的指针
// 存储过程OUTPUT参数。
Dest interface{}
// In是参数是否是INOUT参数。 如果是这样,输入值存储
// 过程是Dest指针的取消引用值,然后将其替换为
// 输出值。
In bool
// 包含过滤或未导出的字段
}
type RawBytes(查看源代码)
RawBytes 是一个字节片段,用于保存对数据库本身拥有的内存的引用。在扫描到 RawBytes 之后,切片只有在下一次调用 Next,Scan 或 Close 时才有效。
type RawBytes []byte
type Result(查看源代码)
Result 汇总了执行的 SQL 命令。
type Result interface {
// LastInsertId返回数据库生成的整数
// 以响应命令。 通常这将来自
// 插入新行时的“自动增量(auto increment)”列。 并非全部
// 数据库支持这个功能,以及这样的语法
// 声明各不相同
LastInsertId() (int64, error)
// RowsAffected返回受影响的行数
// 更新,插入或删除。 不是每个数据库或数据库
// 驱动可能会支持这个。
RowsAffected() (int64, error)
}
type Row(查看源代码)
Row 是调用 QueryRow 选择单个行的结果。
type Row struct {
// 包含过滤或未导出的字段
}
func (*Row) Scan(查看源代码)
func (r *Row) Scan(dest ...interface{}) error
Scan 将匹配行中的列复制到 dest 指向的值中。有关详细信息,请参阅 Rows.Scan 上的文档。如果多个行与查询匹配,则 Scan 使用第一行并丢弃其余行。如果没有行与查询匹配,则 Scan 会返回 ErrNoRows。
type Rows(查看源代码)
Rows 是查询的结果。它的游标在结果集的第一行之前开始。使用下一步在行中前进:
rows, err := db.Query("SELECT ...")
...
defer rows.Close()
for rows.Next() {
var id int
var name string
err = rows.Scan(&id, &name)
...
}
err = rows.Err() // 获取迭代期间遇到的任何错误
type Rows struct {
// 包含过滤或未导出的字段
}
func (*Rows) Close(查看源代码)
func (rs *Rows) Close() error
Close 关闭行,防止进一步枚举。如果 Next 被调用并返回false,并且没有其他结果集,则行自动关闭,并且检查 Err 的结果就足够了。关闭是幂等的,不会影响 Err 的结果。
func (*Rows) ColumnTypes(查看源代码)
func (rs *Rows) ColumnTypes() ([]*ColumnType, error)
ColumnTypes 返回列信息,如列类型,长度和空值。某些驱动程序可能无法提供某些信息。
func (*Rows) Columns(查看源代码)
func (rs *Rows) Columns() ([]string, error)
列将返回列名称。如果行关闭,或者行来自 QueryRow 并且存在延迟错误,则列将返回错误。
func (*Rows) Err(查看源代码)
func (rs *Rows) Err() error
Err 会返回在迭代期间遇到的错误(如果有的话)。Err 可能会在显式或隐式关闭后调用。
func (*Rows) Next(查看源代码)
func (rs *Rows) Next() bool
接下来准备下一个结果行以便用 Scan 方法读取。它在成功时返回 true,如果没有下一个结果行或在准备过程中发生错误,则返回 false。应该咨询 Err 以区分这两种情况。
每次调用 Scan,即使是第一次,都必须先调用 Next。
func (*Rows) NextResultSet(查看源代码)
func (rs *Rows) NextResultSet() bool
NextResultSet 准备下一个读取结果集。如果有进一步的结果集,则返回 true;如果没有进一步的结果集或者有错误前进,则返回 false。应该参考 Err 方法来区分这两种情况。
在调用 NextResultSet 之后,应始终在扫描之前调用 Next 方法。如果有更多的结果集,它们在结果集中可能没有行。
func (*Rows) Scan(查看源代码)
func (rs *Rows) Scan(dest ...interface{}) error
Scan 将当前行中的列复制到dest指向的值中。dest 中的值数量必须与行中的列数相同。
Scan 将从数据库读取的列转换为以下常见的 Go 类型和 sql 包提供的特殊类型:
*string
*[]byte
*int, *int8, *int16, *int32, *int64
*uint, *uint8, *uint16, *uint32, *uint64
*bool
*float32, *float64
*interface{}
*RawBytes
any type implementing Scanner (see Scanner docs)
在最简单的情况下,如果源列中的值的类型是整数,bool 或字符串类型 T 和 dest 的类型是 *T,则 Scan 只是通过指针分配值。
Scan 也可以在字符串和数字类型之间进行转换,只要不会丢失任何信息。当Scan 将从数字数据库列扫描的所有数字串化为*字符串时,将扫描为数字类型进行溢出检查。例如,值为300的float64 或值为“300”的字符串可以扫描到 uint16,但不能扫描到uint8,但 float64(255)或“255”可扫描到uint8。一个例外是某些 float64 数字字符串的扫描可能会在字符串化时丢失信息。通常,将浮点列扫描到 *float64。
如果 dest 参数的类型为 *[]byte,则 Scan 会在该参数中保存相应数据的副本。副本由调用者拥有,可以无限期修改和保存。可以通过使用类型 *RawBytes 的参数来避免该副本;请参阅 RawBytes 的文档以了解其使用限制。
如果参数具有类型 *interface{},则扫描会复制底层驱动程序提供的值而不进行转换。从类型 []byte 的源值扫描到 *interface{}时,会创建片的副本,并且调用者拥有结果。
时间类型的源值可以被扫描为类型 *time.Time, *interface {},*string 或 *[]byte 的值。当转换到后两者时,使用 time.Format3339Nano。
可以将bool类型的源值扫描到*bool,*interface {},*string,*[]字节或 *RawBytes 类型中。
对于扫描到 *bool,源可能是true,false,1,0或 strconv.ParseBool 可解析的字符串输入。
type Scanner(查看源代码)
Scanner 是 Scan 使用的接口。
type Scanner interface {
// 扫描从数据库驱动程序分配一个值。
//
// src值将是以下类型之一:
//
// int64
// float64
// bool
// []byte
// string
// time.Time
// nil - for NULL values
//
// 如果该值无法存储,则应返回错误
// 而不会丢失信息。
Scan(src interface{}) error
}
type Stmt(查看源代码)
Stmt 是一个准备好的声明。Stmt 对于多个 goroutines 并发使用是安全的。
type Stmt struct {
// 包含过滤或未导出的字段
}
func (*Stmt) Close(查看源代码)
func (s *Stmt) Close() error
Close 关闭声明。
func (*Stmt) Exec(查看源代码)
func (s *Stmt) Exec(args ...interface{}) (Result, error)
Exec 使用给定的参数执行一个准备好的语句,并返回一个总结语句效果的 Result 。
func (*Stmt) ExecContext(查看源代码)
func (s *Stmt) ExecContext(ctx context.Context, args ...interface{}) (Result, error)
ExecContext 使用给定的参数执行一个准备好的语句,并返回一个总结语句效果的 Result 。
func (*Stmt) Query(查看源代码)
func (s *Stmt) Query(args ...interface{}) (*Rows, error)
查询使用给定的参数执行准备好的查询语句,并将查询结果作为 *Row 返回。
func (*Stmt) QueryContext(查看源代码)
func (s *Stmt) QueryContext(ctx context.Context, args ...interface{}) (*Rows, error)
QueryContext 使用给定的参数执行准备好的查询语句,并将查询结果作为 *Row 返回。
func (*Stmt) QueryRow(查看源代码)
func (s *Stmt) QueryRow(args ...interface{}) *Row
QueryRow 使用给定的参数执行一个准备好的查询语句。如果在执行语句期间发生错误,则在返回的 *Row 上调用 Scan 以返回该错误,该行总是非零。如果查询未选择行,则*Row 的扫描将返回错误号。否则,*Row 的扫描将扫描第一个选定的行并丢弃其余行。
用法示例:
var name string
err := nameByUseridStmt.QueryRow(id).Scan(&name)
func (*Stmt) QueryRowContext(查看源代码)
func (s *Stmt) QueryRowContext(ctx context.Context, args ...interface{}) *Row
QueryRowContext 使用给定的参数执行准备好的查询语句。如果在执行语句期间发生错误,则在返回的 *Row 上调用 Scan 以返回该错误,该行总是非零。如果查询未选择行,则 *Row 的扫描将返回错误号。否则,*Row 的扫描将扫描第一个选定的行并丢弃其余行。
用法示例:
var name string
err := nameByUseridStmt.QueryRowContext(ctx, id).Scan(&name)
type Tx(查看源代码)
Tx 是正在进行的数据库事务。
Transaction 必须以对 Commit 或 Rollback 的调用结束。
在调用 Commit 或 Rollback 之后,事务上的所有操作都会因ErrTxDone 而失败。
通过调用事务的 Prepare 或 Stmt 方法为事务准备的语句通过对Commit 或 Rollback 的调用关闭。
type Tx struct {
// 包含过滤或未导出的字段
}
func (*Tx) Commit(查看源代码)
func (tx *Tx) Commit() error
Commit 提交事务(transaction)。
func (*Tx) Exec(查看源代码)
func (tx *Tx) Exec(query string, args ...interface{}) (Result, error)
Exec 执行不返回行的查询。例如:INSERT 和 UPDATE。
func (*Tx) ExecContext(查看源代码)
func (tx *Tx) ExecContext(ctx context.Context, query string, args ...interface{}) (Result, error)
ExecContext 执行不返回行的查询。例如:INSERT 和 UPDATE。
func (*Tx) Prepare(查看源代码)
func (tx *Tx) Prepare(query string) (*Stmt, error)
Prepare 创建一个准备好的语句,以便在事务中使用。
返回的语句在事务内运行,并且一旦事务被提交或回滚就不能再使用。
要在此事务中使用现有的准备语句,请参阅 Tx.Stmt。
func (*Tx) PrepareContext(查看源代码)
func (tx *Tx) PrepareContext(ctx context.Context, query string) (*Stmt, error)
PrepareContext 在事务中创建一个准备好的语句。
返回的语句在事务内运行,并在事务提交或回滚时关闭。
要在此事务中使用现有的准备语句,请参阅 Tx.Stmt。
提供的上下文将用于准备上下文,而不用于执行返回的语句。返回的语句将在事务上下文中运行。
func (*Tx) Query(查看源代码)
func (tx *Tx) Query(query string, args ...interface{}) (*Rows, error)
查询执行一个返回行的查询,通常是一个 SELECT。
func (*Tx) QueryContext(查看源代码)
func (tx *Tx) QueryContext(ctx context.Context, query string, args ...interface{}) (*Rows, error)
QueryContext 执行一个返回行的查询,通常是一个 SELECT。
func (*Tx) QueryRow(查看源代码)
func (tx *Tx) QueryRow(query string, args ...interface{}) *Row
QueryRow 执行一个查询,该查询最多只返回一行。QueryRow 总是返回一个非零值。错误被推迟到行的扫描方法被调用。如果查询未选择行,则*Row 的扫描将返回错误号。否则,*Row 的扫描将扫描第一个选定的行并丢弃其余行。
func (*Tx) QueryRowContext(查看源代码)
func (tx *Tx) QueryRowContext(ctx context.Context, query string, args ...interface{}) *Row
QueryRowContext 执行一个预计最多只返回一行的查询。QueryRowContext 总是返回一个非零值。错误被推迟到行的扫描方法被调用。如果查询未选择行,则*Row 的扫描将返回错误号。否则,*Row 的扫描将扫描第一个选定的行并丢弃其余行。
func (*Tx) Rollback(查看源代码)
func (tx *Tx) Rollback() error
Rollback 将中止事务。
func (*Tx) Stmt(查看源代码)
func (tx *Tx) Stmt(stmt *Stmt) *Stmt
Stmt 从现有的语句中返回特定于事务的预处理语句。
例子:
updateMoney, err := db.Prepare("UPDATE balance SET money=money+? WHERE id=?")
...
tx, err := db.Begin()
...
res, err := tx.Stmt(updateMoney).Exec(123.45, 98293203)
返回的语句在事务内运行,并在事务提交或回滚时关闭。
func (*Tx) StmtContext(查看源代码)
func (tx *Tx) StmtContext(ctx context.Context, stmt *Stmt) *Stmt
StmtContext 从现有语句中返回特定于事务的预处理语句。
示例:
updateMoney, err := db.Prepare("UPDATE balance SET money=money+? WHERE id=?")
...
tx, err := db.Begin()
...
res, err := tx.StmtContext(ctx, updateMoney).Exec(123.45, 98293203)
返回的语句在事务内运行,并在事务提交或回滚时关闭。
type TxOptions(查看源代码)
TxOptions 保存要在 DB.BeginTx 中使用的事务选项。
type TxOptions struct {
// 隔离(Isolation)是事务隔离级别。
// 如果为零,则使用驱动程序或数据库的默认级别。
Isolation IsolationLevel
ReadOnly bool
}
子目录
名称 |
概要 |
---|---|
| 驱动程序| |
软件包驱动程序定义由 sql 包使用的数据库驱动程序实现的接口。 |
数据库 | database相关
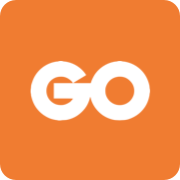
Go 是一种编译型语言,它结合了解释型语言的游刃有余,动态类型语言的开发效率,以及静态类型的安全性。它也打算成为现代的,支持网络与多核计算的语言。要满足这些目标,需要解决一些语言上的问题:一个富有表达能力但轻量级的类型系统,并发与垃圾回收机制,严格的依赖规范等等。这些无法通过库或工具解决好,因此Go也就应运而生了。
主页 | https://golang.org/ |
源码 | https://go.googlesource.com/go |
发布版本 | 1.9.2 |