JavaScript参考手册
错误 | Errors
Error
通过 Error 的构造器可以创建一个错误对象。当运行时错误产生时,Error的实例对象会被抛出。Error对象 也 可用于用户自定义的异常的基础对象。下面列出了各种内建的标准错误类型。
语法
new Error([message[, fileName[, lineNumber]]])
参数
message
可选的。人类可读的错误描述。
fileName
可选的。fileName
创建的Error
对象上属性的值。缺省为包含调用Error()
构造函数的代码的文件的名称。
lineNumber
可选的。lineNumber
创建的Error
对象上属性的值。默认为包含Error()
构造函数调用的行号。
描述
当代码运行时的发生错误,会创建新的Error对象,并将其抛出。
该页面描述了Error对象自身的使用,以及其构造函数的使用. 关于Error实例的内部属性和方法,请看 Error.prototype.
Error 类型
除了通用的Error构造函数外,JavaScript还有6个其他类型的错误构造函数。更多客户端异常,详见Exception Handling Statements。
EvalError
创建一个error实例,表示错误的原因:与 eval()
有关。
InternalError
创建一个代表Javascript引擎内部错误的异常抛出的实例。 如: "递归太多".RangeError
创建一个error实例,表示错误的原因:数值变量或参数超出其有效范围。
ReferenceError
创建一个error实例,表示错误的原因:无效引用。
SyntaxError
创建一个error实例,表示错误的原因:eval()
在解析代码的过程中发生的语法错误。
TypeError
创建一个error实例,表示错误的原因:变量或参数不属于有效类型。
URIError
创建一个error实例,表示错误的原因:给 encodeURI()
或 decodeURl()
传递的参数无效。
属性
Error.prototype允许添加属性到Error实例.
方法
全局Error对象自身不包含任何方法,但从原型链中继承了一些方法.
Error
实例
Error
非泛型错误的所有实例和实例都从中继承Error.prototype
。和所有的构造函数一样,你可以使用构造函数的原型来为使用该构造函数创建的所有实例添加属性或方法。
属性
标准属性
Error.prototype.constructor
指定创建实例原型的函数。
Error.prototype.message
错误信息。
Error.prototype.name
错误名称。
供应商特定的扩展
非标准
这个功能是非标准的,不在标准计划中。不要在面向Web的站点上使用它:它不适用于每个用户。实现之间也可能存在不兼容,并且在未来可能会改变。
微软
Error.prototype.description
错误描述。类似于message
。
Error.prototype.number
错误编号。
Mozilla
Error.prototype.fileName
引发此错误的文件路径。
Error.prototype.lineNumber
引发此错误的文件中的行号。
Error.prototype.columnNumber
引发此错误的列号。
Error.prototype.stack
堆栈跟踪。
方法
Error.prototype.toSource()
返回包含指定Error
对象的源的字符串; 你可以使用这个值来创建一个新的对象。重写该Object.prototype.toSource()
方法。
Error.prototype.toString()
返回表示指定对象的字符串。重写该Object.prototype.toString()
方法。
示例
例1: 抛出一个基本错误
通常你会使用throw关键字来抛出你创建的Error对象。可以使用 try...catch 结构来处理异常:
try {
throw new Error('Whoops!');
} catch (e) {
console.log(e.name + ': ' + e.message);
}
例2: 处理一个特定错误
你可以通过判断异常的类型来特定处理某一类的异常,即判断 constructor 属性,当使用现代Javascript引擎时,可使用instanceof 关键字:
try {
foo.bar();
} catch (e) {
if (e instanceof EvalError) {
console.log(e.name + ': ' + e.message);
} else if (e instanceof RangeError) {
console.log(e.name + ': ' + e.message);
}
// ... etc
}
示例: 自定义异常类型
你可能希望自定义基于Error的异常类型,使得你能够 throw new MyError() 并可以使用 instanceof MyError 来检查某个异常的类型. 这种需求的通用解决方法如下.
ES6自定义错误类
如果没有额外的配置, Babel和其他transpilers将不能正确处理下面的代码。
有些浏览器在使用ES6类时在堆栈跟踪中包含CustomError构造函数。
class CustomError extends Error {
constructor(foo = 'bar', ...params) {
//Pass remaining arguments (including vendor specific ones) to parent constructor
super(...params);
//Custom debugging information
this.foo = foo;
this.date = new Date();
}
}
try {
throw new CustomError('baz', 'bazMessage');
} catch(e){
console.log(e.foo); //baz
console.log(e.message); //bazMessage
console.log(e.stack); //stacktrace
}
ES5自定义错误对象
使用原型声明时,所有浏览器都在堆栈跟踪中包含CustomError构造函数。
function CustomError(foo, message, fileName, lineNumber) {
var instance = new Error(message, fileName, lineNumber);
instance.foo = foo;
Object.setPrototypeOf(instance, Object.getPrototypeOf(this));
return instance;
}
CustomError.prototype = Object.create(Error.prototype, {
constructor: {
value: Error,
enumerable: false,
writable: true,
configurable: true
}
});
if (Object.setPrototypeOf){
Object.setPrototypeOf(CustomError, Error);
} else {
CustomError.__proto__ = Error;
}
try {
throw new CustomError('baz', 'bazMessage');
} catch(e){
console.log(e.foo); //baz
console.log(e.message) ;//bazMessage
}
规范
Specification |
Status |
Comment |
---|---|---|
ECMAScript 1st Edition (ECMA-262) |
Standard |
Initial definition. Implemented in JavaScript 1.1. |
ECMAScript 5.1 (ECMA-262)The definition of 'Error' in that specification. |
Standard |
|
ECMAScript 2015 (6th Edition, ECMA-262)The definition of 'Error' in that specification. |
Standard |
|
ECMAScript Latest Draft (ECMA-262)The definition of 'Error' in that specification. |
Living Standard |
|
浏览器兼容性
Feature |
Chrome |
Edge |
Firefox |
Internet Explorer |
Opera |
Safari |
---|---|---|---|---|---|---|
Basic Support |
(Yes) |
(Yes) |
(Yes) |
6 |
(Yes) |
(Yes) |
prototype |
(Yes) |
(Yes) |
(Yes) |
6 |
(Yes) |
(Yes) |
columnNumber |
No |
No |
(Yes) |
No |
No |
No |
fileName |
No |
No |
(Yes) |
No |
No |
No |
lineNumber |
No |
No |
(Yes) |
No |
No |
No |
message |
(Yes) |
(Yes) |
(Yes) |
6 |
(Yes) |
(Yes) |
name |
(Yes) |
(Yes) |
(Yes) |
6 |
(Yes) |
(Yes) |
stack |
(Yes) |
(Yes) |
(Yes) |
10 |
(Yes) |
6 |
toSource |
No |
No |
(Yes) |
No |
No |
No |
toString |
(Yes) |
(Yes) |
(Yes) |
6 |
(Yes) |
(Yes) |
Feature |
Android |
Chrome for Android |
Edge mobile |
Firefox for Android |
IE mobile |
Opera Android |
iOS Safari |
---|---|---|---|---|---|---|---|
Basic Support |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
8.1 |
(Yes) |
(Yes) |
prototype |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
8.1 |
(Yes) |
(Yes) |
columnNumber |
No |
No |
No |
(Yes) |
No |
No |
No |
fileName |
No |
No |
No |
(Yes) |
No |
No |
No |
lineNumber |
No |
No |
No |
(Yes) |
No |
No |
No |
message |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
8.1 |
(Yes) |
(Yes) |
name |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
8.1 |
(Yes) |
(Yes) |
stack |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
? |
(Yes) |
6 |
toSource |
No |
No |
No |
(Yes) |
No |
No |
No |
toString |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
8.1 |
(Yes) |
(Yes) |
错误 | Errors相关
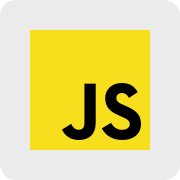
JavaScript 是一种高级编程语言,通过解释执行,是一门动态类型,面向对象(基于原型)的解释型语言。它已经由ECMA(欧洲电脑制造商协会)通过 ECMAScript 实现语言的标准化。它被世界上的绝大多数网站所使用,也被世界主流浏览器( Chrome、IE、FireFox、Safari、Opera )支持。JavaScript 是一门基于原型、函数先行的语言,是一门多范式的语言,它支持面向对象编程,命令式编程,以及函数式编程。它提供语法来操控文本、数组、日期以及正则表达式等,不支持 I/O,比如网络