Eslint参考手册
规则 | Rules
no-useless-call
函数调用可以由Function.prototype.call()
和写入Function.prototype.apply()
。但是Function.prototype.call()
并且Function.prototype.apply()
比正常的函数调用慢。
规则细节
此规则旨在标记使用情况,Function.prototype.call()
并且Function.prototype.apply()
可以用正常的函数调用来替代。
此规则的错误代码示例:
/*eslint no-useless-call: "error"*/
// These are same as `foo(1, 2, 3);`
foo.call(undefined, 1, 2, 3);
foo.apply(undefined, [1, 2, 3]);
foo.call(null, 1, 2, 3);
foo.apply(null, [1, 2, 3]);
// These are same as `obj.foo(1, 2, 3);`
obj.foo.call(obj, 1, 2, 3);
obj.foo.apply(obj, [1, 2, 3]);
此规则的正确代码示例:
/*eslint no-useless-call: "error"*/
// The `this` binding is different.
foo.call(obj, 1, 2, 3);
foo.apply(obj, [1, 2, 3]);
obj.foo.call(null, 1, 2, 3);
obj.foo.apply(null, [1, 2, 3]);
obj.foo.call(otherObj, 1, 2, 3);
obj.foo.apply(otherObj, [1, 2, 3]);
// The argument list is variadic.
foo.apply(undefined, args);
foo.apply(null, args);
obj.foo.apply(obj, args);
已知限制
此规则静态比较代码以检查是否thisArg
更改。所以如果关于代码thisArg
是一个动态表达式,这个规则不能正确判断。
此规则的错误代码示例:
/*eslint no-useless-call: "error"*/
a[i++].foo.call(a[i++], 1, 2, 3);
此规则的正确代码示例:
/*eslint no-useless-call: "error"*/
a[++i].foo.call(a[i], 1, 2, 3);
何时不使用它
如果你不想被通知不需要做.call()
和.apply()
,你可以放心禁用此规则。
版本
该规则在 ESLint 1.0.0-rc-1 中引入。
资源
- Rule source
- Documentation source
规则 | Rules相关
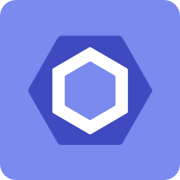
ESLint 是一个代码规范和错误检查工具,有以下几个特性。所有东西都是可以插拔的。你可以调用任意的 rule api 或者 formatter api 去打包或者定义 rule or formatter。任意的 rule 都是独立的。没有特定的 coding style,你可以自己配置。
主页 | https://eslint.org/ |
源码 | https://github.com/eslint/eslint |
发布版本 | 4.12.0 |