Eslint参考手册
规则 | Rules
comma-style
The --fix
option on the command line can automatically fix some of the problems reported by this rule.
The Comma Style rule enforces styles for comma-separated lists. There are two comma styles primarily used in JavaScript:
- The standard style, in which commas are placed at the end of the current line
- Comma First style, in which commas are placed at the start of the next line
One of the justifications for using Comma First style is that it can help track missing and trailing commas. These are problematic because missing commas in variable declarations can lead to the leakage of global variables and trailing commas can lead to errors in older versions of IE.
Rule Details
This rule enforce consistent comma style in array literals, object literals, and variable declarations.
This rule does not apply in either of the following cases:
- comma preceded and followed by linebreak (lone comma)
- single-line array literals, object literals, and variable declarations
Options
This rule has a string option:
-
"last"
(default) requires a comma after and on the same line as an array element, object property, or variable declaration -
"first"
requires a comma before and on the same line as an array element, object property, or variable declaration
This rule also accepts an additional exceptions
object:
-
"exceptions"
has properties whose names correspond to node types in the abstract syntax tree (AST) of JavaScript code:
- `"ArrayExpression": true` ignores comma style in array literals
- `"ArrayPattern": true` ignores comma style in array patterns of destructuring
- `"ArrowFunctionExpression": true` ignores comma style in the parameters of arrow function expressions
- `"CallExpression": true` ignores comma style in the arguments of function calls
- `"FunctionDeclaration": true` ignores comma style in the parameters of function declarations
- `"FunctionExpression": true` ignores comma style in the parameters of function expressions
- `"ImportDeclaration": true` ignores comma style in the specifiers of import declarations
- `"ObjectExpression": true` ignores comma style in object literals
- `"ObjectPattern": true` ignores comma style in object patterns of destructuring
- `"VariableDeclaration": true` ignores comma style in variable declarations
A way to determine the node types as defined by ESTree is to use the online demo.
last
Examples of incorrect code for this rule with the default "last"
option:
/*eslint comma-style: ["error", "last"]*/
var foo = 1
,
bar = 2;
var foo = 1
, bar = 2;
var foo = ["apples"
, "oranges"];
function bar() {
return {
"a": 1
,"b:": 2
};
}
Examples of correct code for this rule with the default "last"
option:
/*eslint comma-style: ["error", "last"]*/
var foo = 1, bar = 2;
var foo = 1,
bar = 2;
var foo = ["apples",
"oranges"];
function bar() {
return {
"a": 1,
"b:": 2
};
}
first
Examples of incorrect code for this rule with the "first"
option:
/*eslint comma-style: ["error", "first"]*/
var foo = 1,
bar = 2;
var foo = ["apples",
"oranges"];
function bar() {
return {
"a": 1,
"b:": 2
};
}
Examples of correct code for this rule with the "first"
option:
/*eslint comma-style: ["error", "first"]*/
var foo = 1, bar = 2;
var foo = 1
,bar = 2;
var foo = ["apples"
,"oranges"];
function bar() {
return {
"a": 1
,"b:": 2
};
}
exceptions
An example use case is to enforce comma style only in var statements.
Examples of incorrect code for this rule with sample "first", { "exceptions": { … } }
options:
/*eslint comma-style: ["error", "first", { "exceptions": { "ArrayExpression": true, "ObjectExpression": true } }]*/
var o = {},
a = [];
Examples of correct code for this rule with sample "first", { "exceptions": { … } }
options:
/*eslint comma-style: ["error", "first", { "exceptions": { "ArrayExpression": true, "ObjectExpression": true } }]*/
var o = {fst:1,
snd: [1,
2]}
, a = [];
When Not To Use It
This rule can safely be turned off if your project does not care about enforcing a consistent comma style.
Further Reading
For more information on the Comma First style:
- A better coding convention for lists and object literals in JavaScript by isaacs
- npm coding style guideline
Related Rules
- operator-linebreak
Version
This rule was introduced in ESLint 0.9.0.
Resources
- Rule source
- Documentation source
© JS Foundation and other contributors
Licensed under the MIT License.
https://eslint.org/docs/rules/comma-style
规则 | Rules相关
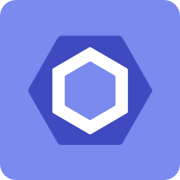
ESLint 是一个代码规范和错误检查工具,有以下几个特性。所有东西都是可以插拔的。你可以调用任意的 rule api 或者 formatter api 去打包或者定义 rule or formatter。任意的 rule 都是独立的。没有特定的 coding style,你可以自己配置。
主页 | https://eslint.org/ |
源码 | https://github.com/eslint/eslint |
发布版本 | 4.12.0 |