Eslint参考手册
规则 | Rules
comma-dangle
在--fix
命令行上的选项可以自动修复一些被这条规则反映的问题。
根据ECMAScript 5(和ECMAScript 3!)规范,对象文字中的尾随逗号是有效的。但是,IE8(当不在IE8文档模式下)和下面的代码在JavaScript中遇到尾随逗号时会引发错误。
var foo = {
bar: "baz",
qux: "quux",
};
尾随逗号可以简化向对象和数组中添加和删除项目的操作,因为只有您正在修改的行必须被触及。支持尾随逗号的另一个论据是,当从对象或数组中添加或删除项目时,它提高了差异的清晰度:
Less clear:
var foo = {
- bar: "baz",
- qux: "quux"
+ bar: "baz"
};
更清晰:
var foo = {
bar: "baz",
- qux: "quux",
};
规则细节
此规则强制在对象和数组文字中一致地使用尾随逗号。
选项
此规则具有字符串选项或对象选项:
{
"comma-dangle": ["error", "never"],
// or
"comma-dangle": ["error", {
"arrays": "never",
"objects": "never",
"imports": "never",
"exports": "never",
"functions": "ignore"
}]
}
-
"never"
(默认)不允许尾随逗号 -
"always"
需要尾随逗号 -
"always-multiline"
需要尾随逗号时的最后一个元素或属性是一个不同的线比所述封闭]
或}
和不允许尾随逗号时的最后一个元素或属性是基于相同行的闭合]
或}
-
"only-multiline"
允许(但不要求)后逗号时的最后一个元素或属性是一个不同的线比所述封闭]
或}
和不允许尾随逗号时的最后一个元素或属性是基于相同行的闭合]
或}
自ECMAScript 2017以来,函数声明和函数调用中的尾随逗号是有效的语法; 但是,字符串选项不检查这些情况的向后兼容性。
您还可以使用对象选项为每种语法类型配置此规则。下列选项每一个都可以被设置为"never"
,"always"
,"always-multiline"
,"only-multiline"
,或"ignore"
。"never"
除非另有说明,每个选项的默认值是。
-
arrays
是数组文字和数组模式的解构。(例如let [a,] = [1,];
) -
objects
是针对解构的对象文字和对象模式。(例如let {a,} = {a: 1};
) -
imports
用于ES模块的进口申报。(例如import {a,} from "foo";
) -
exports
用于ES模块的出口申报。(例如export {a,};
) -
functions
用于函数声明和函数调用。(例如(function(a,){ })(b,);
)-
functions
被设置为"ignore"
默认情况下以字符串选项的一致性。 -
functions
只能在LINTING ECMAScript 2017或更高版本时启用。
-
决不
此规则的默认代码错误代码示例"never"
:
/*eslint comma-dangle: ["error", "never"]*/
var foo = {
bar: "baz",
qux: "quux",
};
var arr = [1,2,];
foo({
bar: "baz",
qux: "quux",
});
具有默认选项的此规则的正确代码示例"never"
:
/*eslint comma-dangle: ["error", "never"]*/
var foo = {
bar: "baz",
qux: "quux"
};
var arr = [1,2];
foo({
bar: "baz",
qux: "quux"
});
总是
此规则的错误代码示例包含以下"always"
选项:
/*eslint comma-dangle: ["error", "always"]*/
var foo = {
bar: "baz",
qux: "quux"
};
var arr = [1,2];
foo({
bar: "baz",
qux: "quux"
});
此规则的正确代码示例包含以下"always"
选项:
/*eslint comma-dangle: ["error", "always"]*/
var foo = {
bar: "baz",
qux: "quux",
};
var arr = [1,2,];
foo({
bar: "baz",
qux: "quux",
});
总是多
此规则的错误代码示例包含以下"always-multiline"
选项:
/*eslint comma-dangle: ["error", "always-multiline"]*/
var foo = {
bar: "baz",
qux: "quux"
};
var foo = { bar: "baz", qux: "quux", };
var arr = [1,2,];
var arr = [1,
2,];
var arr = [
1,
2
];
foo({
bar: "baz",
qux: "quux"
});
此规则的正确代码示例包含以下"always-multiline"
选项:
/*eslint comma-dangle: ["error", "always-multiline"]*/
var foo = {
bar: "baz",
qux: "quux",
};
var foo = {bar: "baz", qux: "quux"};
var arr = [1,2];
var arr = [1,
2];
var arr = [
1,
2,
];
foo({
bar: "baz",
qux: "quux",
});
只有-多
此规则的错误代码示例包含以下"only-multiline"
选项:
/*eslint comma-dangle: ["error", "only-multiline"]*/
var foo = { bar: "baz", qux: "quux", };
var arr = [1,2,];
var arr = [1,
2,];
此规则的正确代码示例包含以下"only-multiline"
选项:
/*eslint comma-dangle: ["error", "only-multiline"]*/
var foo = {
bar: "baz",
qux: "quux",
};
var foo = {
bar: "baz",
qux: "quux"
};
var foo = {bar: "baz", qux: "quux"};
var arr = [1,2];
var arr = [1,
2];
var arr = [
1,
2,
];
var arr = [
1,
2
];
foo({
bar: "baz",
qux: "quux",
});
foo({
bar: "baz",
qux: "quux"
});
功能
此规则的错误代码示例包含以下{"functions": "never"}
选项:
/*eslint comma-dangle: ["error", {"functions": "never"}]*/
function foo(a, b,) {
}
foo(a, b,);
new foo(a, b,);
此规则的正确代码示例包含以下{"functions": "never"}
选项:
/*eslint comma-dangle: ["error", {"functions": "never"}]*/
function foo(a, b) {
}
foo(a, b);
new foo(a, b);
此规则的错误代码示例包含以下{"functions": "always"}
选项:
/*eslint comma-dangle: ["error", {"functions": "always"}]*/
function foo(a, b) {
}
foo(a, b);
new foo(a, b);
此规则的正确代码示例包含以下{"functions": "always"}
选项:
/*eslint comma-dangle: ["error", {"functions": "always"}]*/
function foo(a, b,) {
}
foo(a, b,);
new foo(a, b,);
何时不使用它
如果你不关注悬摆逗号,你可以关闭这个规则。
版
该规则在ESLint 0.16.0中引入。
资源
- 规则来源
- 文档来源
规则 | Rules相关
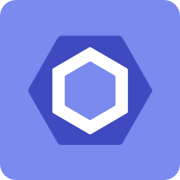
ESLint 是一个代码规范和错误检查工具,有以下几个特性。所有东西都是可以插拔的。你可以调用任意的 rule api 或者 formatter api 去打包或者定义 rule or formatter。任意的 rule 都是独立的。没有特定的 coding style,你可以自己配置。
主页 | https://eslint.org/ |
源码 | https://github.com/eslint/eslint |
发布版本 | 4.12.0 |