Ruby 2.4参考手册
URI
URI::MailTo
Parent:URI::GenericIncluded modules:URI::REGEXP
RFC6068,mailto URL方案
常量
COMPONENT
URI::MailTo的可用组件的数组
DEFAULT_PORT
URI::MailTo的默认端口为nil
属性
headersR
由URL设置的电子邮件标题,作为数组阵列
toR
作为字符串的URL的主要电子邮件地址
公共类方法
build(args) Show source
描述
从组件创建一个新的URI::MailTo对象,并进行语法检查。
组件可以作为数组或散列提供。如果使用数组,则必须提供组件的标题。
如果使用散列,则键是冒号前面的组件名称。
头文件可以作为预先编码的字符串提供,例如“subject = subscribe&cc = address”,或者是一个Array of Arrays
'subject', 'subscribe', 'cc', 'address'
示例:
require 'uri'
m1 = URI::MailTo.build(['joe@example.com', 'subject=Ruby'])
puts m1.to_s -> mailto:joe@example.com?subject=Ruby
m2 = URI::MailTo.build(['john@example.com', [['Subject', 'Ruby'], ['Cc', 'jack@example.com']]])
puts m2.to_s -> mailto:john@example.com?Subject=Ruby&Cc=jack@example.com
m3 = URI::MailTo.build({:to => 'listman@example.com', :headers => [['subject', 'subscribe']]})
puts m3.to_s -> mailto:listman@example.com?subject=subscribe
调用超类方法URI::Generic.build
# File lib/uri/mailto.rb, line 85
def self.build(args)
tmp = Util.make_components_hash(self, args)
case tmp[:to]
when Array
tmp[:opaque] = tmp[:to].join(',')
when String
tmp[:opaque] = tmp[:to].dup
else
tmp[:opaque] = ''
end
if tmp[:headers]
query =
case tmp[:headers]
when Array
tmp[:headers].collect { |x|
if x.kind_of?(Array)
x[0] + '=' + x[1..-1].join
else
x.to_s
end
}.join('&')
when Hash
tmp[:headers].collect { |h,v|
h + '=' + v
}.join('&')
else
tmp[:headers].to_s
end
unless query.empty?
tmp[:opaque] << '?' << query
end
end
super(tmp)
end
new(*arg) Show source
描述
从通用URL组件创建新的URI::MailTo对象,不进行语法检查。
这个方法通常从URI.parse中调用,它检查每个组件的有效性。
调用超类方法URI::Generic.new
# File lib/uri/mailto.rb, line 132
def initialize(*arg)
super(*arg)
@to = nil
@headers = []
# The RFC3986 parser does not normally populate opaque
@opaque = "?#{@query}" if @query && !@opaque
unless @opaque
raise InvalidComponentError,
"missing opaque part for mailto URL"
end
to, header = @opaque.split('?', 2)
# allow semicolon as a addr-spec separator
# http://support.microsoft.com/kb/820868
unless /\A(?:[^@,;]+@[^@,;]+(?:\z|[,;]))*\z/ =~ to
raise InvalidComponentError,
"unrecognised opaque part for mailtoURL: #{@opaque}"
end
if arg[10] # arg_check
self.to = to
self.headers = header
else
set_to(to)
set_headers(header)
end
end
公共实例方法
headers=(v) Show source
二进制头文件 v
# File lib/uri/mailto.rb, line 232
def headers=(v)
check_headers(v)
set_headers(v)
v
end
to=(v) Show source
二传手 v
# File lib/uri/mailto.rb, line 200
def to=(v)
check_to(v)
set_to(v)
v
end
to_mailtext() Show source
以字符串的形式返回URL的RFC822电子邮件文本。
示例:
require 'uri'
uri = URI.parse("mailto:ruby-list@ruby-lang.org?Subject=subscribe&cc=myaddr")
uri.to_mailtext
# => "To: ruby-list@ruby-lang.org\nSubject: subscribe\nCc: myaddr\n\n\n"
# File lib/uri/mailto.rb, line 268
def to_mailtext
to = URI.decode_www_form_component(@to)
head = ''
body = ''
@headers.each do |x|
case x[0]
when 'body'
body = URI.decode_www_form_component(x[1])
when 'to'
to << ', ' + URI.decode_www_form_component(x[1])
else
head << URI.decode_www_form_component(x[0]).capitalize + ': ' +
URI.decode_www_form_component(x[1]) + "\n"
end
end
"To: #{to}
#{head}
#{body}
"
end
另外别名为:to_rfc822text
to_rfc822text()
别名为:to_mailtext
to_s() Show source
从URI构造字符串
# File lib/uri/mailto.rb, line 239
def to_s
@scheme + ':' +
if @to
@to
else
''
end +
if @headers.size > 0
'?' + @headers.collect{|x| x.join('=')}.join('&')
else
''
end +
if @fragment
'#' + @fragment
else
''
end
end
受保护的实例方法
set_headers(v) Show source
标题的私人二传手 v
# File lib/uri/mailto.rb, line 221
def set_headers(v)
@headers = []
if v
v.split('&').each do |x|
@headers << x.split(/=/, 2)
end
end
end
set_to(v) Show source
私人二传手 v
# File lib/uri/mailto.rb, line 194
def set_to(v)
@to = v
end
私有实例方法
check_headers(v) Show source
检查标头v
组件
- HEADER_REGEXP
# File lib/uri/mailto.rb, line 208
def check_headers(v)
return true unless v
return true if v.size == 0
if HEADER_REGEXP !~ v
raise InvalidComponentError,
"bad component(expected opaque component): #{v}"
end
true
end
check_to(v) Show source
检查v
组件
# File lib/uri/mailto.rb, line 169
def check_to(v)
return true unless v
return true if v.size == 0
v.split(/[,;]/).each do |addr|
# check url safety as path-rootless
if /\A(?:%\h\h|[!$&-.0-;=@-Z_a-z~])*\z/ !~ addr
raise InvalidComponentError,
"an address in 'to' is invalid as URI #{addr.dump}"
end
# check addr-spec
# don't s/\+/ /g
addr.gsub!(/%\h\h/, URI::TBLDECWWWCOMP_)
if EMAIL_REGEXP !~ addr
raise InvalidComponentError,
"an address in 'to' is invalid as uri-escaped addr-spec #{addr.dump}"
end
end
true
end
URI相关
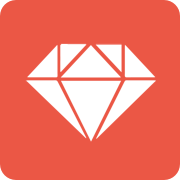
Ruby 是一种面向对象、命令式、函数式、动态的通用编程语言,是世界上最优美而巧妙的语言。
主页 | https://www.ruby-lang.org/ |
源码 | https://github.com/ruby/ruby |
版本 | 2.4 |
发布版本 | 2.4.1 |