Ruby 2.4参考手册
Shell
Shell
Parent:ObjectIncluded modules:Shell::Error
Shell为常见的UNIX shell命令实现了一个惯用的Ruby界面。
它为用户提供了使用过滤器和管道执行命令的能力,例如通过使用Ruby的本地设施执行sh / csh。
例子
创建临时文件
在这个例子中,我们将在/ tmp目录下的三个不同文件夹中创建三个tmpFile。
sh = Shell.cd("/tmp") # Change to the /tmp directory
sh.mkdir "shell-test-1" unless sh.exists?("shell-test-1")
# make the 'shell-test-1' directory if it doesn't already exist
sh.cd("shell-test-1") # Change to the /tmp/shell-test-1 directory
for dir in ["dir1", "dir3", "dir5"]
if !sh.exists?(dir)
sh.mkdir dir # make dir if it doesn't already exist
sh.cd(dir) do
# change to the `dir` directory
f = sh.open("tmpFile", "w") # open a new file in write mode
f.print "TEST\n" # write to the file
f.close # close the file handler
end
print sh.pwd # output the process working directory
end
end
用自身创建临时文件
这个例子和第一个例子是一样的,只不过我们使用了Shell :: CommandProcessor#transact。
Shell :: CommandProcessor#transact对自己执行给定的块,在本例中为sh; 我们的Shell对象。 在块内我们可以将sh.cd替换为cd,因为块内的范围已经使用了sh。
sh = Shell.cd("/tmp")
sh.transact do
mkdir "shell-test-1" unless exists?("shell-test-1")
cd("shell-test-1")
for dir in ["dir1", "dir3", "dir5"]
if !exists?(dir)
mkdir dir
cd(dir) do
f = open("tmpFile", "w")
f.print "TEST\n"
f.close
end
print pwd
end
end
end
Pipe /etc/printcap into a file
在这个例子中,我们将读取由cupsd生成的操作系统文件/ etc / printcap,然后将其输出到相对于sh的pwd的新文件。
sh = Shell.new
sh.cat("/etc/printcap") | sh.tee("tee1") > "tee2"
(sh.cat < "/etc/printcap") | sh.tee("tee11") > "tee12"
sh.cat("/etc/printcap") | sh.tee("tee1") >> "tee2"
(sh.cat < "/etc/printcap") | sh.tee("tee11") >> "tee12"
属性
cascadeRW
debugR
debug?R
verboseRW
verbose?RW
command_processorR
cwdR
返回当前的工作目录。
debugR
debug?R
dirR
返回当前的工作目录。
dir_stackR
dirsR
getwdR
返回当前的工作目录。
process_controllerR
pwdR
返回当前的工作目录。
record_separatorRW
system_pathR
返回数组中的命令搜索路径
umaskRW
返回umask
verboseRW
verbose?RW
公共类方法
alias_command(alias, command, *opts, &block) Show source
Shell :: CommandProcessor.alias_command的便捷方法。 定义一个将使用另一个名称执行命令的实例方法。
Shell.def_system_command('date')
Shell.alias_command('date_in_utc', 'date', '-u')
Shell.new.date_in_utc # => Sat Jan 25 16:59:57 UTC 2014
# File lib/shell.rb, line 393
def Shell.alias_command(ali, command, *opts, &block)
CommandProcessor.alias_command(ali, command, *opts, &block)
end
cd(path) Show source
用当前工作目录设置为路径创建一个新的Shell实例。
# File lib/shell.rb, line 125
def cd(path)
new(path)
end
debug=(val) Show source
# File lib/shell.rb, line 114
def debug=(val)
@debug = val
@verbose = val if val
end
def_system_command(command, path = command) Show source
Shell :: CommandProcessor.def_system_command的便捷方法。 定义将执行给定shell命令的实例方法。 如果可执行文件不在:: default_system_path中,则必须提供它的路径。
Shell.def_system_command('hostname')
Shell.new.hostname # => localhost
# How to use an executable that's not in the default path
Shell.def_system_command('run_my_program', "~/hello")
Shell.new.run_my_program # prints "Hello from a C program!"
# File lib/shell.rb, line 373
def Shell.def_system_command(command, path = command)
CommandProcessor.def_system_command(command, path)
end
default_record_separator() Show source
# File lib/shell.rb, line 158
def default_record_separator
if @default_record_separator
@default_record_separator
else
$/
end
end
default_record_separator=(rs) Show source
# File lib/shell.rb, line 166
def default_record_separator=(rs)
@default_record_separator = rs
end
default_system_path() Show source
以当前名称的数组形式返回当前shell的PATH环境变量中的目录。这为Shell的所有实例设置#system_path。
例如:如果在你当前的shell中,且执行了以下操作:
$ echo $PATH
/usr/bin:/bin:/usr/local/bin
然后在上面的shell中运行此方法将返回:
["/usr/bin", "/bin", "/usr/local/bin"]
# File lib/shell.rb, line 142
def default_system_path
if @default_system_path
@default_system_path
else
ENV["PATH"].split(":")
end
end
default_system_path=(path) Show source
设置Shell的新实例应该具有#system_path作为其初始system_path。
path
应该是一组目录名字符串。
# File lib/shell.rb, line 154
def default_system_path=(path)
@default_system_path = path
end
install_system_commands(pre = "sys_") Show source
Shell :: CommandProcessor.install_system_commands的便捷方法。定义表示在:: default_system_path中找到的所有可执行文件的实例方法,并在其名称前面加上给定的前缀。
Shell.install_system_commands
Shell.new.sys_echo("hello") # => hello
# File lib/shell.rb, line 413
def Shell.install_system_commands(pre = "sys_")
CommandProcessor.install_system_commands(pre)
end
new(pwd, umask) → obj Show source
创建一个当前目录设置为当前进程目录的Shell对象,除非pwd
参数另有指定。
# File lib/shell.rb, line 183
def initialize(pwd = Dir.pwd, umask = nil)
@cwd = File.expand_path(pwd)
@dir_stack = []
@umask = umask
@system_path = Shell.default_system_path
@record_separator = Shell.default_record_separator
@command_processor = CommandProcessor.new(self)
@process_controller = ProcessController.new(self)
@verbose = Shell.verbose
@debug = Shell.debug
end
notify(*opts) { |mes| ... } Show source
# File lib/shell.rb, line 426
def self.notify(*opts)
Shell::debug_output_synchronize do
if opts[-1].kind_of?(String)
yorn = verbose?
else
yorn = opts.pop
end
return unless yorn
if @debug_display_thread_id
if @debug_display_process_id
prefix = "shell(##{Process.pid}:#{Thread.current.to_s.sub("Thread", "Th")}): "
else
prefix = "shell(#{Thread.current.to_s.sub("Thread", "Th")}): "
end
else
prefix = "shell: "
end
_head = true
STDERR.print opts.collect{|mes|
mes = mes.dup
yield mes if iterator?
if _head
_head = false
prefix + mes
else
" "* prefix.size + mes
end
}.join("\n")+"\n"
end
end
unalias_command(ali) Show source
Shell :: CommandProcessor.unalias_command的便捷方法
# File lib/shell.rb, line 398
def Shell.unalias_command(ali)
CommandProcessor.unalias_command(ali)
end
undef_system_command(command) Show source
Shell :: CommandProcessor.undef_system_command的便捷方法
# File lib/shell.rb, line 378
def Shell.undef_system_command(command)
CommandProcessor.undef_system_command(command)
end
公共实例方法
cd(path = nil, verbose = @verbose)
别名为:chdir
chdir(path) Show source
创建当前目录设置为路径的Shell对象。
如果给出了一个块,它将在块结束时恢复当前目录。
如果调用为迭代器,则在块结束时恢复当前目录。
# File lib/shell.rb, line 261
def chdir(path = nil, verbose = @verbose)
check_point
if iterator?
notify("chdir(with block) #{path}") if verbose
cwd_old = @cwd
begin
chdir(path, nil)
yield
ensure
chdir(cwd_old, nil)
end
else
notify("chdir #{path}") if verbose
path = "~" unless path
@cwd = expand_path(path)
notify "current dir: #{@cwd}"
rehash
Void.new(self)
end
end
另外别名为:cd
debug=(val) Show source
# File lib/shell.rb, line 216
def debug=(val)
@debug = val
@verbose = val if val
end
expand_path(path) Show source
# File lib/shell.rb, line 227
def expand_path(path)
File.expand_path(path, @cwd)
end
inspect() Show source
调用超类方法Object#inspect
# File lib/shell.rb, line 418
def inspect
if debug.kind_of?(Integer) && debug > 2
super
else
to_s
end
end
jobs() Show source
返回预定任务的列表。
# File lib/shell.rb, line 345
def jobs
@process_controller.jobs
end
kill(signal, job) Show source
将给定的signal发送给给定的job
# File lib/shell.rb, line 353
def kill(sig, command)
@process_controller.kill_job(sig, command)
end
popd()
别名为:popdir
popdir() Show source
从目录栈中弹出一个目录,并将当前目录设置为它。
# File lib/shell.rb, line 329
def popdir
check_point
notify("popdir")
if pop = @dir_stack.pop
chdir pop
notify "dir stack: [#{@dir_stack.join ', '}]"
self
else
Shell.Fail DirStackEmpty
end
Void.new(self)
end
另外别名为:popd
pushd(path = nil, verbose = @verbose)
别名为:pushdir
pushdir(path) Show source
pushdir(path) { &block }
将当前目录推送到目录堆栈,将当前目录更改为路径。
如果省略了路径,它将交换其当前目录和其目录堆栈的顶部。
如果给出了一个块,它将在块结束时恢复当前目录。
# File lib/shell.rb, line 295
def pushdir(path = nil, verbose = @verbose)
check_point
if iterator?
notify("pushdir(with block) #{path}") if verbose
pushdir(path, nil)
begin
yield
ensure
popdir
end
elsif path
notify("pushdir #{path}") if verbose
@dir_stack.push @cwd
chdir(path, nil)
notify "dir stack: [#{@dir_stack.join ', '}]"
self
else
notify("pushdir") if verbose
if pop = @dir_stack.pop
@dir_stack.push @cwd
chdir pop
notify "dir stack: [#{@dir_stack.join ', '}]"
self
else
Shell.Fail DirStackEmpty
end
end
Void.new(self)
end
另外别名为:pushd
system_path=(path) Show source
设置系统路径(Shell实例的PATH环境变量)。
路径应该是一个目录名称字符串数组。
# File lib/shell.rb, line 204
def system_path=(path)
@system_path = path
rehash
end
Shell | ||
---|---|---|
Shellwords | 详细 |
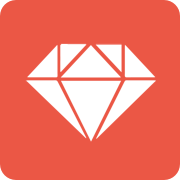
Ruby 是一种面向对象、命令式、函数式、动态的通用编程语言,是世界上最优美而巧妙的语言。
主页 | https://www.ruby-lang.org/ |
源码 | https://github.com/ruby/ruby |
版本 | 2.4 |
发布版本 | 2.4.1 |