Ruby 2.4参考手册
Ripper
Ripper
Parent:Object
Ripper是一个Ruby脚本解析器。
您可以使用基于事件的样式从解析器中获取信息。诸如抽象语法树或Ruby程序的简单词法分析等信息。
用法
Ripper提供了一个简单的界面,用于将程序解析为符号表达式树(或S表达式)。
理解解析器的输出可能会成为一个挑战,建议您使用PP来设置输出的格式以便易读。
require 'ripper'
require 'pp'
pp Ripper.sexp('def hello(world) "Hello, #{world}!"; end')
#=> [:program,
[[:def,
[:@ident, "hello", [1, 4]],
[:paren,
[:params, [[:@ident, "world", [1, 10]]], nil, nil, nil, nil, nil, nil]],
[:bodystmt,
[[:string_literal,
[:string_content,
[:@tstring_content, "Hello, ", [1, 18]],
[:string_embexpr, [[:var_ref, [:@ident, "world", [1, 27]]]]],
[:@tstring_content, "!", [1, 33]]]]],
nil,
nil,
nil]]]]
你可以在上面的例子中看到,表达式以:program开始。
从这里,方法定义在:def,后面跟着方法的标识符:@ident。 方法的标识符出现在括号:paren和方法参数下:params。
接下来是方法主体,从bodystmt(stmt含义语句)开始,它包含方法的完整定义。
在我们的例子中,我们只是返回一个String,所以接下来我们有:string_literal表达式。
在我们的:string_literal中,您会注意到两个@tstring_content,这是Hello和!的文字部分。 在两个@tstring_content语句之间是一个:string_embexpr,其中embexpr是嵌入式表达式。 我们的表达式由一个局部变量或var_ref组成,其中包含世界的标识符(@ident)。
常量
EVENTS
这个数组包含所有ripper事件的名称。
PARSER_EVENTS
该数组包含parser事件的名称。
SCANNER_EVENTS
该数组包含scanner事件的名称。
版本
Ripper的版本
公共类方法
dedent_string(p1, p2) Show source
static VALUE
parser_dedent_string(VALUE self, VALUE input, VALUE width)
{
int wid, col;
StringValue(input);
wid = NUM2UINT(width);
rb_str_modify(input);
col = dedent_string(input, wid);
return INT2NUM(col);
}
lex(src, filename = '-', lineno = 1) Show source
标记Ruby程序并返回一个数组的数组,格式如[[lineno,column],type,token]。
require 'ripper'
require 'pp'
pp Ripper.lex("def m(a) nil end")
#=> [[[1, 0], :on_kw, "def"],
[[1, 3], :on_sp, " " ],
[[1, 4], :on_ident, "m" ],
[[1, 5], :on_lparen, "(" ],
[[1, 6], :on_ident, "a" ],
[[1, 7], :on_rparen, ")" ],
[[1, 8], :on_sp, " " ],
[[1, 9], :on_kw, "nil"],
[[1, 12], :on_sp, " " ],
[[1, 13], :on_kw, "end"]]
# File ext/ripper/lib/ripper/lexer.rb, line 42
def Ripper.lex(src, filename = '-', lineno = 1)
Lexer.new(src, filename, lineno).lex
end
new(src, filename="(ripper)", lineno=1) → ripper Show source
创建一个新的Ripper对象。src 必须是一个String,一个IO或一个已经获得方法的对象。
此方法不会开始分析。另见#parse和:: parse。
static VALUE
ripper_initialize(int argc, VALUE *argv, VALUE self)
{
struct parser_params *parser;
VALUE src, fname, lineno;
TypedData_Get_Struct(self, struct parser_params, &parser_data_type, parser);
rb_scan_args(argc, argv, "12", &src, &fname, &lineno);
if (RB_TYPE_P(src, T_FILE)) {
lex_gets = ripper_lex_io_get;
}
else if (rb_respond_to(src, id_gets)) {
lex_gets = ripper_lex_get_generic;
}
else {
StringValue(src);
lex_gets = lex_get_str;
}
lex_input = src;
parser->eofp = 0;
if (NIL_P(fname)) {
fname = STR_NEW2("(ripper)");
OBJ_FREEZE(fname);
}
else {
StringValue(fname);
fname = rb_str_new_frozen(fname);
}
parser_initialize(parser);
ruby_sourcefile_string = fname;
ruby_sourcefile = RSTRING_PTR(fname);
ruby_sourceline = NIL_P(lineno) ? 0 : NUM2INT(lineno) - 1;
return Qnil;
}
parse(src, filename = '(ripper)', lineno = 1) Show source
解析从src读取的给定的Ruby程序。 src必须是一个String或一个IO或具有gets方法的对象。
# File ext/ripper/lib/ripper/core.rb, line 17
def Ripper.parse(src, filename = '(ripper)', lineno = 1)
new(src, filename, lineno).parse
end
sexp(src, filename = '-', lineno = 1) Show source
实验
解析src并创建S-exp树。 返回更可读的树,而不是:: sexp_raw。 此方法主要供开发人员使用。
require 'ripper'
require 'pp'
pp Ripper.sexp("def m(a) nil end")
#=> [:program,
[[:def,
[:@ident, "m", [1, 4]],
[:paren, [:params, [[:@ident, "a", [1, 6]]], nil, nil, nil, nil]],
[:bodystmt, [[:var_ref, [:@kw, "nil", [1, 9]]]], nil, nil, nil]]]]
# File ext/ripper/lib/ripper/sexp.rb, line 30
def Ripper.sexp(src, filename = '-', lineno = 1)
builder = SexpBuilderPP.new(src, filename, lineno)
sexp = builder.parse
sexp unless builder.error?
end
sexp_raw(src, filename = '-', lineno = 1) Show source
实验
解析src并创建S-exp树。 此方法主要供开发人员使用。
require 'ripper'
require 'pp'
pp Ripper.sexp_raw("def m(a) nil end")
#=> [:program,
[:stmts_add,
[:stmts_new],
[:def,
[:@ident, "m", [1, 4]],
[:paren, [:params, [[:@ident, "a", [1, 6]]], nil, nil, nil]],
[:bodystmt,
[:stmts_add, [:stmts_new], [:var_ref, [:@kw, "nil", [1, 9]]]],
nil,
nil,
nil]]]]
# File ext/ripper/lib/ripper/sexp.rb, line 56
def Ripper.sexp_raw(src, filename = '-', lineno = 1)
builder = SexpBuilder.new(src, filename, lineno)
sexp = builder.parse
sexp unless builder.error?
end
slice(src, pattern, n = 0) Show source
实验
解析src并返回一个与pattern匹配的字符串。 模式应该被描述为正则表达式。
require 'ripper'
p Ripper.slice('def m(a) nil end', 'ident') #=> "m"
p Ripper.slice('def m(a) nil end', '[ident lparen rparen]+') #=> "m(a)"
p Ripper.slice("<<EOS\nstring\nEOS",
'heredoc_beg nl $(tstring_content*) heredoc_end', 1)
#=> "string\n"
# File ext/ripper/lib/ripper/lexer.rb, line 112
def Ripper.slice(src, pattern, n = 0)
if m = token_match(src, pattern)
then m.string(n)
else nil
end
end
tokenize(src, filename = '-', lineno = 1) Show source
标记Ruby程序并返回一个字符串数组。
p Ripper.tokenize("def m(a) nil end")
# => ["def", " ", "m", "(", "a", ")", " ", "nil", " ", "end"]
# File ext/ripper/lib/ripper/lexer.rb, line 20
def Ripper.tokenize(src, filename = '-', lineno = 1)
Lexer.new(src, filename, lineno).tokenize
end
公共实例方法
ripper#column → Integer Show source
返回当前解析行的列号。该数字从0开始。
static VALUE
ripper_column(VALUE self)
{
struct parser_params *parser;
long col;
TypedData_Get_Struct(self, struct parser_params, &parser_data_type, parser);
if (!ripper_initialized_p(parser)) {
rb_raise(rb_eArgError, "method called for uninitialized object");
}
if (NIL_P(parser->parsing_thread)) return Qnil;
col = parser->tokp - lex_pbeg;
return LONG2NUM(col);
}
ripper#encoding → encoding Show source
返回源的编码。
VALUE
rb_parser_encoding(VALUE vparser)
{
struct parser_params *parser;
TypedData_Get_Struct(vparser, struct parser_params, &parser_data_type, parser);
return rb_enc_from_encoding(current_enc);
}
ripper#end_seen? → Boolean Show source
如果解析源以+ _ END _ + 结尾,则返回true 。
VALUE
rb_parser_end_seen_p(VALUE vparser)
{
struct parser_params *parser;
TypedData_Get_Struct(vparser, struct parser_params, &parser_data_type, parser);
return ruby__end__seen ? Qtrue : Qfalse;
}
ripper#error? → Boolean Show source
如果解析的源有错误,则返回true。
static VALUE
ripper_error_p(VALUE vparser)
{
struct parser_params *parser;
TypedData_Get_Struct(vparser, struct parser_params, &parser_data_type, parser);
return parser->error_p ? Qtrue : Qfalse;
}
ripper#filename → String Show source
返回当前解析文件名。
static VALUE
ripper_filename(VALUE self)
{
struct parser_params *parser;
TypedData_Get_Struct(self, struct parser_params, &parser_data_type, parser);
if (!ripper_initialized_p(parser)) {
rb_raise(rb_eArgError, "method called for uninitialized object");
}
return ruby_sourcefile_string;
}
ripper#lineno → Integer Show source
返回当前解析行的行号。该数字从1开始。
static VALUE
ripper_lineno(VALUE self)
{
struct parser_params *parser;
TypedData_Get_Struct(self, struct parser_params, &parser_data_type, parser);
if (!ripper_initialized_p(parser)) {
rb_raise(rb_eArgError, "method called for uninitialized object");
}
if (NIL_P(parser->parsing_thread)) return Qnil;
return INT2NUM(ruby_sourceline);
}
ripper#parse Show source
开始解析并返回根操作的值。
static VALUE
ripper_parse(VALUE self)
{
struct parser_params *parser;
TypedData_Get_Struct(self, struct parser_params, &parser_data_type, parser);
if (!ripper_initialized_p(parser)) {
rb_raise(rb_eArgError, "method called for uninitialized object");
}
if (!NIL_P(parser->parsing_thread)) {
if (parser->parsing_thread == rb_thread_current())
rb_raise(rb_eArgError, "Ripper#parse is not reentrant");
else
rb_raise(rb_eArgError, "Ripper#parse is not multithread-safe");
}
parser->parsing_thread = rb_thread_current();
rb_ensure(ripper_parse0, self, ripper_ensure, self);
return parser->result;
}
yydebug → true or false Show source
得到yydebug。
VALUE
rb_parser_get_yydebug(VALUE self)
{
struct parser_params *parser;
TypedData_Get_Struct(self, struct parser_params, &parser_data_type, parser);
return yydebug ? Qtrue : Qfalse;
}
yydebug = flag Show source
设置yydebug。
VALUE
rb_parser_set_yydebug(VALUE self, VALUE flag)
{
struct parser_params *parser;
TypedData_Get_Struct(self, struct parser_params, &parser_data_type, parser);
yydebug = RTEST(flag);
return flag;
}
私有实例方法
_dispatch_0() Show source
# File ext/ripper/lib/ripper/core.rb, line 32
def _dispatch_0() nil end
_dispatch_1(a) Show source
# File ext/ripper/lib/ripper/core.rb, line 33
def _dispatch_1(a) a end
_dispatch_2(a, b) Show source
# File ext/ripper/lib/ripper/core.rb, line 34
def _dispatch_2(a, b) a end
_dispatch_3(a, b, c) Show source
# File ext/ripper/lib/ripper/core.rb, line 35
def _dispatch_3(a, b, c) a end
_dispatch_4(a, b, c, d) Show source
# File ext/ripper/lib/ripper/core.rb, line 36
def _dispatch_4(a, b, c, d) a end
_dispatch_5(a, b, c, d, e) Show source
# File ext/ripper/lib/ripper/core.rb, line 37
def _dispatch_5(a, b, c, d, e) a end
_dispatch_6(a, b, c, d, e, f) Show source
# File ext/ripper/lib/ripper/core.rb, line 38
def _dispatch_6(a, b, c, d, e, f) a end
_dispatch_7(a, b, c, d, e, f, g) Show source
# File ext/ripper/lib/ripper/core.rb, line 39
def _dispatch_7(a, b, c, d, e, f, g) a end
compile_error(msg) Show source
解析器发现语法错误时调用此方法。
# File ext/ripper/lib/ripper/core.rb, line 60
def compile_error(msg)
end
dedent_string(p1, p2) Show source
static VALUE
parser_dedent_string(VALUE self, VALUE input, VALUE width)
{
int wid, col;
StringValue(input);
wid = NUM2UINT(width);
rb_str_modify(input);
col = dedent_string(input, wid);
return INT2NUM(col);
}
warn(fmt, *args) Show source
解析器生成弱警告时调用此方法。 fmt和args是printf样式。
# File ext/ripper/lib/ripper/core.rb, line 51
def warn(fmt, *args)
end
warning(fmt, *args) Show source
解析器生成强烈警告时会调用此方法。 fmt和args是printf样式。
# File ext/ripper/lib/ripper/core.rb, line 56
def warning(fmt, *args)
end
Ripper | ||
---|---|---|
Ripper::Filter | 详细 |
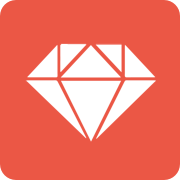
Ruby 是一种面向对象、命令式、函数式、动态的通用编程语言,是世界上最优美而巧妙的语言。
主页 | https://www.ruby-lang.org/ |
源码 | https://github.com/ruby/ruby |
版本 | 2.4 |
发布版本 | 2.4.1 |