Ruby 2.4参考手册
Prime
Prime
Parent:ObjectIncluded modules:Enumerable, Singleton
The set of all prime numbers.
Example
Prime.each(100) do |prime|
p prime #=> 2, 3, 5, 7, 11, ...., 97
end
Prime is Enumerable:
Prime.first 5 # => [2, 3, 5, 7, 11]
Retrieving the instance
For convenience, each instance method of Prime
.instance can be accessed as a class method of Prime
.
e.g.
Prime.instance.prime?(2) #=> true
Prime.prime?(2) #=> true
Generators
A “generator” provides an implementation of enumerating pseudo-prime numbers and it remembers the position of enumeration and upper bound. Furthermore, it is an external iterator of prime enumeration which is compatible with an Enumerator.
Prime
::PseudoPrimeGenerator
is the base class for generators. There are few implementations of generator.
Prime
::EratosthenesGenerator
Uses eratosthenes' sieve.
Prime
::TrialDivisionGenerator
Uses the trial division method.
Prime
::Generator23
Generates all positive integers which are not divisible by either 2 or 3. This sequence is very bad as a pseudo-prime sequence. But this is faster and uses much less memory than the other generators. So, it is suitable for factorizing an integer which is not large but has many prime factors. e.g. for #prime? .
Public Instance Methods
each(ubound = nil, generator = EratosthenesGenerator.new, &block) Show source
Iterates the given block over all prime numbers.
Parameters
ubound
Optional. An arbitrary positive number. The upper bound of enumeration. The method enumerates prime numbers infinitely if ubound
is nil.
generator
Optional. An implementation of pseudo-prime generator.
Return value
An evaluated value of the given block at the last time. Or an enumerator which is compatible to an Enumerator
if no block given.
Description
Calls block
once for each prime number, passing the prime as a parameter.
ubound
Upper bound of prime numbers. The iterator stops after it yields all prime numbers p <= ubound
.
# File lib/prime.rb, line 135
def each(ubound = nil, generator = EratosthenesGenerator.new, &block)
generator.upper_bound = ubound
generator.each(&block)
end
int_from_prime_division(pd) Show source
Re-composes a prime factorization and returns the product.
Parameters
pd
Array of pairs of integers. The each internal pair consists of a prime number – a prime factor – and a natural number – an exponent.
Example
For [[p_1, e_1], [p_2, e_2], ...., [p_n, e_n]]
, it returns:
p_1**e_1 * p_2**e_2 * .... * p_n**e_n.
Prime.int_from_prime_division([[2,2], [3,1]]) #=> 12
# File lib/prime.rb, line 171
def int_from_prime_division(pd)
pd.inject(1){|value, (prime, index)|
value * prime**index
}
end
prime?(value, generator = Prime::Generator23.new) Show source
Returns true if value
is a prime number, else returns false.
Parameters
value
an arbitrary integer to be checked.
generator
optional. A pseudo-prime generator.
# File lib/prime.rb, line 147
def prime?(value, generator = Prime::Generator23.new)
raise ArgumentError, "Expected a prime generator, got #{generator}" unless generator.respond_to? :each
raise ArgumentError, "Expected an integer, got #{value}" unless value.respond_to?(:integer?) && value.integer?
return false if value < 2
generator.each do |num|
q,r = value.divmod num
return true if q < num
return false if r == 0
end
end
prime_division(value, generator = Prime::Generator23.new) Show source
Returns the factorization of value
.
Parameters
value
An arbitrary integer.
generator
Optional. A pseudo-prime generator. generator
.succ must return the next pseudo-prime number in the ascending order. It must generate all prime numbers, but may also generate non prime numbers too.
Exceptions
ZeroDivisionError
when value
is zero.
Example
For an arbitrary integer:
n = p_1**e_1 * p_2**e_2 * .... * p_n**e_n,
#prime_division(n) returns:
[[p_1, e_1], [p_2, e_2], ...., [p_n, e_n]].
Prime.prime_division(12) #=> [[2,2], [3,1]]
# File lib/prime.rb, line 201
def prime_division(value, generator = Prime::Generator23.new)
raise ZeroDivisionError if value == 0
if value < 0
value = -value
pv = [[-1, 1]]
else
pv = []
end
generator.each do |prime|
count = 0
while (value1, mod = value.divmod(prime)
mod) == 0
value = value1
count += 1
end
if count != 0
pv.push [prime, count]
end
break if value1 <= prime
end
if value > 1
pv.push [value, 1]
end
pv
end
Ruby Core © 1993–2017 Yukihiro Matsumoto
Licensed under the Ruby License.
Ruby Standard Library © contributors
Licensed under their own licenses.
Prime | ||
---|---|---|
Prime::EratosthenesGenerator | 详细 | |
Prime::EratosthenesSieve | 详细 | |
Prime::Generator23 | 详细 | |
Prime::PseudoPrimeGenerator | 详细 | |
Prime::TrialDivision | 详细 | |
Prime::TrialDivisionGenerator | 详细 |
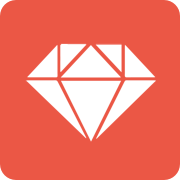
Ruby 是一种面向对象、命令式、函数式、动态的通用编程语言,是世界上最优美而巧妙的语言。
主页 | https://www.ruby-lang.org/ |
源码 | https://github.com/ruby/ruby |
版本 | 2.4 |
发布版本 | 2.4.1 |