Ruby 2.4参考手册
过程 | Process
Process::Status
父辈:对象
Process::Status
封装有关正在运行或终止的系统进程状态的信息。内置变量$?
是一个nil
或一个Process::Status
对象。
fork { exit 99 } #=> 26557
Process.wait #=> 26557
$?.class #=> Process::Status
$?.to_i #=> 25344
$? >> 8 #=> 99
$?.stopped? #=> false
$?.exited? #=> true
$?.exitstatus #=> 99
Posix系统使用16位整数记录进程信息。低位记录进程状态(停止,退出,发信号),高位可能包含附加信息(例如程序在退出进程中的返回码)。在Ruby 1.8之前,这些位直接暴露给Ruby程序。Ruby现在将它们封装在一个Process::Status
对象中。然而,为了最大化兼容性,这些对象保留了一个面向比特的接口。在下面的描述中,当我们谈论stat的整数值时,我们指的是这个16位值。
公共实例方法
stat & num → integer Show source
将stat中的位与num进行逻辑与。
fork { exit 0x37 }
Process.wait
sprintf('%04x', $?.to_i) #=> "3700"
sprintf('%04x', $? & 0x1e00) #=> "1600"
static VALUE
pst_bitand(VALUE st1, VALUE st2)
{
int status = PST2INT(st1) & NUM2INT(st2);
return INT2NUM(status);
}
stat == other → true or false Show source
true
如果stat的整数值等于other,则返回。
static VALUE
pst_equal(VALUE st1, VALUE st2)
{
if (st1 == st2) return Qtrue;
return rb_equal(pst_to_i(st1), st2);
}
stat >> num → integer Show source
按住Shift键在比特统计权NUM的地方。
fork { exit 99 } #=> 26563
Process.wait #=> 26563
$?.to_i #=> 25344
$? >> 8 #=> 99
static VALUE
pst_rshift(VALUE st1, VALUE st2)
{
int status = PST2INT(st1) >> NUM2INT(st2);
return INT2NUM(status);
}
coredump? → true or false Show source
true
如果stat在终止时生成了coredump,则返回。不适用于所有平台。
static VALUE
pst_wcoredump(VALUE st)
{
#ifdef WCOREDUMP
int status = PST2INT(st);
if (WCOREDUMP(status))
return Qtrue;
else
return Qfalse;
#else
return Qfalse;
#endif
}
exited? → true or false Show source
返回stattrue
是否正常退出(例如,使用调用或完成程序)。exit()
static VALUE
pst_wifexited(VALUE st)
{
int status = PST2INT(st);
if (WIFEXITED(status))
return Qtrue;
else
return Qfalse;
}
exitstatus → integer or nil Show source
返回stat的返回码的最不重要的八位。只有如果exited?
是true
。
fork { } #=> 26572
Process.wait #=> 26572
$?.exited? #=> true
$?.exitstatus #=> 0
fork { exit 99 } #=> 26573
Process.wait #=> 26573
$?.exited? #=> true
$?.exitstatus #=> 99
static VALUE
pst_wexitstatus(VALUE st)
{
int status = PST2INT(st);
if (WIFEXITED(status))
return INT2NUM(WEXITSTATUS(status));
return Qnil;
}
inspect → string Show source
覆盖检查方法。
system("false")
p $?.inspect #=> "#<Process::Status: pid 12861 exit 1>"
static VALUE
pst_inspect(VALUE st)
{
rb_pid_t pid;
int status;
VALUE vpid, str;
vpid = pst_pid(st);
if (NIL_P(vpid)) {
return rb_sprintf("#<%s: uninitialized>", rb_class2name(CLASS_OF(st)));
}
pid = NUM2PIDT(vpid);
status = PST2INT(st);
str = rb_sprintf("#<%s: ", rb_class2name(CLASS_OF(st)));
pst_message(str, pid, status);
rb_str_cat2(str, ">");
return str;
}
pid → integer Show source
返回此状态对象表示的进程标识。
fork { exit } #=> 26569
Process.wait #=> 26569
$?.pid #=> 26569
static VALUE
pst_pid(VALUE st)
{
return rb_attr_get(st, id_pid);
}
signaled? → true or false Show source
返回true
如果统计终止,因为一个未捕获的信号。
static VALUE
pst_wifsignaled(VALUE st)
{
int status = PST2INT(st);
if (WIFSIGNALED(status))
return Qtrue;
else
return Qfalse;
}
stopped? → true or false Show source
true
如果此进程停止,则返回。只有在相应的wait
呼叫WUNTRACED
设置了标志时才会返回。
static VALUE
pst_wifstopped(VALUE st)
{
int status = PST2INT(st);
if (WIFSTOPPED(status))
return Qtrue;
else
return Qfalse;
}
stopsig → integer or nil Show source
返回导致stat停止的信号的数量(或者nil
如果self没有停止)。
static VALUE
pst_wstopsig(VALUE st)
{
int status = PST2INT(st);
if (WIFSTOPPED(status))
return INT2NUM(WSTOPSIG(status));
return Qnil;
}
success? → true, false or nil Show source
true
如果stat成功false
则返回,如果不成功则返回。nil
如果exited?
不是,则返回true
。
static VALUE
pst_success_p(VALUE st)
{
int status = PST2INT(st);
if (!WIFEXITED(status))
return Qnil;
return WEXITSTATUS(status) == EXIT_SUCCESS ? Qtrue : Qfalse;
}
termsig → integer or nil Show source
返回导致stat终止的信号的编号(或者nil
如果self没有被未被捕获的信号终止)。
static VALUE
pst_wtermsig(VALUE st)
{
int status = PST2INT(st);
if (WIFSIGNALED(status))
return INT2NUM(WTERMSIG(status));
return Qnil;
}
to_i → integer Show source
to_int → integer
将stat中的位返回为a Integer
。在这些位上徘徊依赖于平台。
fork { exit 0xab } #=> 26566
Process.wait #=> 26566
sprintf('%04x', $?.to_i) #=> "ab00"
static VALUE
pst_to_i(VALUE st)
{
return rb_ivar_get(st, id_status);
}
to_s → string Show source
以字符串形式显示pid和退出状态。
system("false")
p $?.to_s #=> "pid 12766 exit 1"
static VALUE
pst_to_s(VALUE st)
{
rb_pid_t pid;
int status;
VALUE str;
pid = NUM2PIDT(pst_pid(st));
status = PST2INT(st);
str = rb_str_buf_new(0);
pst_message(str, pid, status);
return str;
}
过程 | Process相关
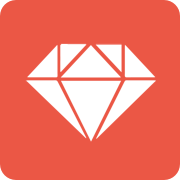
Ruby 是一种面向对象、命令式、函数式、动态的通用编程语言,是世界上最优美而巧妙的语言。
主页 | https://www.ruby-lang.org/ |
源码 | https://github.com/ruby/ruby |
版本 | 2.4 |
发布版本 | 2.4.1 |