Ruby 2.4参考手册
路径名 | Pathname
Pathname
父类:对象
路径名表示文件系统上文件或目录的名称,但不表示文件本身。
路径名取决于操作系统:Unix,Windows等。该库适用于本地操作系统的路径名,但实验性地支持非Unix路径名。
路径名可以是相对的或绝对的。直到你试图引用这个文件时,它才会考虑文件是否存在。
路径名是不可变的。它没有破坏性更新的方法。
这个类的目标是以比Ruby标准提供的更简洁的方式处理文件路径信息。下面的例子说明了不同之处。
包括File,FileTest以及一些Dir和FileUtils的所有功能都包含在内,这并不令人惊讶。它基本上是所有这些的外观,等等。
例子
示例1:使用路径名
require 'pathname'
pn = Pathname.new("/usr/bin/ruby")
size = pn.size # 27662
isdir = pn.directory? # false
dir = pn.dirname # Pathname:/usr/bin
base = pn.basename # Pathname:ruby
dir, base = pn.split # [Pathname:/usr/bin, Pathname:ruby]
data = pn.read
pn.open { |f| _ }
pn.each_line { |line| _ }
例2:使用标准的Ruby
pn = "/usr/bin/ruby"
size = File.size(pn) # 27662
isdir = File.directory?(pn) # false
dir = File.dirname(pn) # "/usr/bin"
base = File.basename(pn) # "ruby"
dir, base = File.split(pn) # ["/usr/bin", "ruby"]
data = File.read(pn)
File.open(pn) { |f| _ }
File.foreach(pn) { |line| _ }
示例3:特殊功能
p1 = Pathname.new("/usr/lib") # Pathname:/usr/lib
p2 = p1 + "ruby/1.8" # Pathname:/usr/lib/ruby/1.8
p3 = p1.parent # Pathname:/usr
p4 = p2.relative_path_from(p3) # Pathname:lib/ruby/1.8
pwd = Pathname.pwd # Pathname:/home/gavin
pwd.absolute? # true
p5 = Pathname.new "." # Pathname:.
p5 = p5 + "music/../articles" # Pathname:music/../articles
p5.cleanpath # Pathname:articles
p5.realpath # Pathname:/home/gavin/articles
p5.children # [Pathname:/home/gavin/articles/linux, ...]
功能细分
核心方法
这些方法正在有效地操纵字符串,因为这是一条路径。除了mountpoint?,children,each_child,realdirpath和realpath以外,这些都不能访问文件系统。
- +
- join
- parent
- root?
- absolute?
- relative?
- relative_path_from
- each_filename
- cleanpath
- realpath
- realdirpath
- children
- each_child
- mountpoint?
文件状态谓词方法
这些方法是FileTest的一个外观:
- blockdev?
- chardev?
- directory?
- executable?
- executable_real?
- exist?
- file?
- grpowned?
- owned?
- pipe?
- readable?
- world_readable?
- readable_real?
- setgid?
- setuid?
- size
- size?
- socket?
- sticky?
- symlink?
- writable?
- world_writable?
- writable_real?
- zero?
文件属性和操作方法
这些方法是File的外观:
- atime
- birthtime
- ctime
- mtime
- chmod(mode)
- lchmod(mode)
- chown(owner, group)
- lchown(owner, group)
- fnmatch(pattern, *args)
- fnmatch?(pattern, *args)
- ftype
- make_link(old)
- open(*args, &block)
- readlink
- rename(to)
- stat
- lstat
- make_symlink(old)
- truncate(length)
- utime(atime, mtime)
- basename(*args)
- dirname
- extname
- expand_path(*args)
- splitDirectory methodsThese methods are a facade for Dir:
- ::glob
- ::getwd / ::pwd
- rmdir
- entries
- each_entry(&block)
- mkdir(*args)
- opendir(*args)
IO
这些方法是IO的立面:
- each_line(*args, &block)
- read(*args)
- binread(*args)
- readlines(*args)
- sysopen(* args)Utilities这些方法是Find,FileUtils和其他的混合:
- find(&block)
- mkpath
- rmtree
- unlink / delete
方法文档
正如上面的部分所示,路径名中的大多数方法都是外墙。这些方法的文档通常只是说,例如,“请参阅FileTest#可写?”,因为无论如何您应该熟悉原始方法,其文档(例如通过ri
)将包含更多信息。在某些情况下,将会进行简要说明。
常量
SAME_PATHS SEPARATOR_LIST SEPARATOR_PAT TO_PATH
#to_path被实现,因此路径名对象可用于File.open等。
公共类方法
getwd() Show source
将当前工作目录作为路径名返回。
Pathname.getwd
#=> #<Pathname:/home/zzak/projects/ruby>
请参阅Dir.getwd。
static VALUE
path_s_getwd(VALUE klass)
{
VALUE str;
str = rb_funcall(rb_cDir, rb_intern("getwd"), 0);
return rb_class_new_instance(1, &str, klass);
}
glob(p1, p2 = v2) Show source
返回或产生路径名对象。
Pathname.glob("config/" "*.rb")
#=> [#<Pathname:config/environment.rb>, #<Pathname:config/routes.rb>, ..]
请参阅Dir.glob。
static VALUE
path_s_glob(int argc, VALUE *argv, VALUE klass)
{
VALUE args[2];
int n;
n = rb_scan_args(argc, argv, "11", &args[0], &args[1]);
if (rb_block_given_p()) {
return rb_block_call(rb_cDir, rb_intern("glob"), n, args, glob_i, klass);
}
else {
VALUE ary;
long i;
ary = rb_funcallv(rb_cDir, rb_intern("glob"), n, args);
ary = rb_convert_type(ary, T_ARRAY, "Array", "to_ary");
for (i = 0; i < RARRAY_LEN(ary); i++) {
VALUE elt = RARRAY_AREF(ary, i);
elt = rb_class_new_instance(1, &elt, klass);
rb_ary_store(ary, i, elt);
}
return ary;
}
}
new(p1) Show source
从给定的String(或String类对象)创建一个Pathname对象。如果path
包含NULL字符(\0
),则会引发ArgumentError。
static VALUE
path_initialize(VALUE self, VALUE arg)
{
VALUE str;
if (RB_TYPE_P(arg, T_STRING)) {
str = arg;
}
else {
str = rb_check_funcall(arg, id_to_path, 0, NULL);
if (str == Qundef)
str = arg;
StringValue(str);
}
if (memchr(RSTRING_PTR(str), '\0', RSTRING_LEN(str)))
rb_raise(rb_eArgError, "pathname contains null byte");
str = rb_obj_dup(str);
set_strpath(self, str);
OBJ_INFECT(self, str);
return self;
}
pwd() Show source
将当前工作目录作为路径名返回。
Pathname.getwd
#=> #<Pathname:/home/zzak/projects/ruby>
请参阅Dir.getwd。
static VALUE
path_s_getwd(VALUE klass)
{
VALUE str;
str = rb_funcall(rb_cDir, rb_intern("getwd"), 0);
return rb_class_new_instance(1, &str, klass);
}
公共实例方法
+(other) Show source
追加一个路径名片段来self
产生一个新的路径名对象。
p1 = Pathname.new("/usr") # Pathname:/usr
p2 = p1 + "bin/ruby" # Pathname:/usr/bin/ruby
p3 = p1 + "/etc/passwd" # Pathname:/etc/passwd
# / is aliased to +.
p4 = p1 / "bin/ruby" # Pathname:/usr/bin/ruby
p5 = p1 / "/etc/passwd" # Pathname:/etc/passwd
此方法不访问文件系统; 它是纯粹的字符串操作。
# File ext/pathname/lib/pathname.rb, line 347
def +(other)
other = Pathname.new(other) unless Pathname === other
Pathname.new(plus(@path, other.to_s))
end
另外别名为:/
/(other)
别名:+
<=>(p1) Show source
为路径名提供区分大小写的比较运算符。
Pathname.new('/usr') <=> Pathname.new('/usr/bin')
#=> -1
Pathname.new('/usr/bin') <=> Pathname.new('/usr/bin')
#=> 0
Pathname.new('/usr/bin') <=> Pathname.new('/USR/BIN')
#=> 1
它将返回-1
,0
或者1
取决于左参数相对于正确参数的值。或者nil
如果参数不具有可比性,它将返回。
static VALUE
path_cmp(VALUE self, VALUE other)
{
VALUE s1, s2;
char *p1, *p2;
char *e1, *e2;
if (!rb_obj_is_kind_of(other, rb_cPathname))
return Qnil;
s1 = get_strpath(self);
s2 = get_strpath(other);
p1 = RSTRING_PTR(s1);
p2 = RSTRING_PTR(s2);
e1 = p1 + RSTRING_LEN(s1);
e2 = p2 + RSTRING_LEN(s2);
while (p1 < e1 && p2 < e2) {
int c1, c2;
c1 = (unsigned char)*p1++;
c2 = (unsigned char)*p2++;
if (c1 == '/') c1 = '\0';
if (c2 == '/') c2 = '\0';
if (c1 != c2) {
if (c1 < c2)
return INT2FIX(-1);
else
return INT2FIX(1);
}
}
if (p1 < e1)
return INT2FIX(1);
if (p2 < e2)
return INT2FIX(-1);
return INT2FIX(0);
}
==(p1) Show source
比较这个路径名other
。该比较是基于字符串的。请注意,两个不同的路径(foo.txt
和./foo.txt
)可以引用同一个文件。
static VALUE
path_eq(VALUE self, VALUE other)
{
if (!rb_obj_is_kind_of(other, rb_cPathname))
return Qfalse;
return rb_str_equal(get_strpath(self), get_strpath(other));
}
===(p1) Show source
比较这个路径名other
。该比较是基于字符串的。请注意,两个不同的路径(foo.txt
和./foo.txt
)可以引用同一个文件。
static VALUE
path_eq(VALUE self, VALUE other)
{
if (!rb_obj_is_kind_of(other, rb_cPathname))
return Qfalse;
return rb_str_equal(get_strpath(self), get_strpath(other));
}
absolute?() Show source
用于测试路径是否绝对的谓词方法。
true
如果路径名以斜杠开始,则返回。
p = Pathname.new('/im/sure')
p.absolute?
#=> true
p = Pathname.new('not/so/sure')
p.absolute?
#=> false
# File ext/pathname/lib/pathname.rb, line 222
def absolute?
!relative?
end
ascend() { |self| ... } Show source
迭代并按照升序为给定路径中的每个元素生成一个新的Pathname对象。
Pathname.new('/path/to/some/file.rb').ascend {|v| p v}
#<Pathname:/path/to/some/file.rb>
#<Pathname:/path/to/some>
#<Pathname:/path/to>
#<Pathname:/path>
#<Pathname:/>
Pathname.new('path/to/some/file.rb').ascend {|v| p v}
#<Pathname:path/to/some/file.rb>
#<Pathname:path/to/some>
#<Pathname:path/to>
#<Pathname:path>
如果没有给出块,则返回枚举器。
enum = Pathname.new("/usr/bin/ruby").ascend
# ... do stuff ...
enum.each { |e| ... }
# yields Pathnames /usr/bin/ruby, /usr/bin, /usr, and /.
它不访问文件系统。
# File ext/pathname/lib/pathname.rb, line 323
def ascend
return to_enum(__method__) unless block_given?
path = @path
yield self
while r = chop_basename(path)
path, = r
break if path.empty?
yield self.class.new(del_trailing_separator(path))
end
end
atime → time Show source
返回文件的上次访问时间。
请参阅File.atime。
static VALUE
path_atime(VALUE self)
{
return rb_funcall(rb_cFile, rb_intern("atime"), 1, get_strpath(self));
}
basename(p1 = v1) Show source
返回路径的最后一个组件。
请参阅File.basename。
static VALUE
path_basename(int argc, VALUE *argv, VALUE self)
{
VALUE str = get_strpath(self);
VALUE fext;
if (rb_scan_args(argc, argv, "01", &fext) == 0)
str = rb_funcall(rb_cFile, rb_intern("basename"), 1, str);
else
str = rb_funcall(rb_cFile, rb_intern("basename"), 2, str, fext);
return rb_class_new_instance(1, &str, rb_obj_class(self));
}
binread([length , offset]) → string Show source
返回文件中的所有字节,N
如果指定则返回第一个字节。
请参阅IO.binread。
static VALUE
path_binread(int argc, VALUE *argv, VALUE self)
{
VALUE args[3];
int n;
args[0] = get_strpath(self);
n = rb_scan_args(argc, argv, "02", &args[1], &args[2]);
return rb_funcallv(rb_cIO, rb_intern("binread"), 1+n, args);
}
binwrite(string, offset ) → fixnum Show source
binwrite(string, offset, open_args ) → fixnum
写入contents
文件,以二进制模式打开它。
请参阅IO.binwrite。
static VALUE
path_binwrite(int argc, VALUE *argv, VALUE self)
{
VALUE args[4];
int n;
args[0] = get_strpath(self);
n = rb_scan_args(argc, argv, "03", &args[1], &args[2], &args[3]);
return rb_funcallv(rb_cIO, rb_intern("binwrite"), 1+n, args);
}
birthtime → time Show source
返回文件的出生时间。如果平台没有生日,则引发NotImplementedError。
请参阅File.birthtime。
static VALUE
path_birthtime(VALUE self)
{
return rb_funcall(rb_cFile, rb_intern("birthtime"), 1, get_strpath(self));
}
blockdev?() Show source
请参阅FileTest#blockdev ?.
static VALUE
path_blockdev_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("blockdev?"), 1, get_strpath(self));
}
chardev?() Show source
请参阅FileTest#chardev ?.
static VALUE
path_chardev_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("chardev?"), 1, get_strpath(self));
}
children(with_directory=true) Show source
将目录的子项(文件和子目录,不递归)作为Pathname对象的数组返回。
默认情况下,返回的路径名将具有足够的信息来访问这些文件。如果您设置with_directory
为false
,则返回的路径名将仅包含文件名。
例如:
pn = Pathname("/usr/lib/ruby/1.8")
pn.children
# -> [ Pathname:/usr/lib/ruby/1.8/English.rb,
Pathname:/usr/lib/ruby/1.8/Env.rb,
Pathname:/usr/lib/ruby/1.8/abbrev.rb, ... ]
pn.children(false)
# -> [ Pathname:English.rb, Pathname:Env.rb, Pathname:abbrev.rb, ... ]
请注意,结果从不包含条目.
和..
目录中,因为它们不是儿童。
# File ext/pathname/lib/pathname.rb, line 438
def children(with_directory=true)
with_directory = false if @path == '.'
result = []
Dir.foreach(@path) {|e|
next if e == '.' || e == '..'
if with_directory
result << self.class.new(File.join(@path, e))
else
result << self.class.new(e)
end
}
result
end
chmod → integer Show source
更改文件权限。
请参阅File.chmod。
static VALUE
path_chmod(VALUE self, VALUE mode)
{
return rb_funcall(rb_cFile, rb_intern("chmod"), 2, mode, get_strpath(self));
}
chown → integer Show source
更改文件的所有者和组。
请参阅File.chown。
static VALUE
path_chown(VALUE self, VALUE owner, VALUE group)
{
return rb_funcall(rb_cFile, rb_intern("chown"), 3, owner, group, get_strpath(self));
}
cleanpath(consider_symlink=false) Show source
self
用连续的斜杠和无用的点去除干净的路径名。文件系统不被访问。
如果consider_symlink
是true
,则使用更保守的算法来避免中断符号链接。这可能会保留..
比绝对必要条目更多的条目,但是如果不访问文件系统,这是无法避免的。
See #realpath.
# File ext/pathname/lib/pathname.rb, line 82
def cleanpath(consider_symlink=false)
if consider_symlink
cleanpath_conservative
else
cleanpath_aggressive
end
end
ctime → time Show source
使用目录信息返回上次更改时间,而不是文件本身。
See File.ctime.
static VALUE
path_ctime(VALUE self)
{
return rb_funcall(rb_cFile, rb_intern("ctime"), 1, get_strpath(self));
}
delete() Show source
如果self
是文件,则使用File.unlink删除文件或目录,或根据需要删除Dir.unlink。
static VALUE
path_unlink(VALUE self)
{
VALUE eENOTDIR = rb_const_get_at(rb_mErrno, rb_intern("ENOTDIR"));
VALUE str = get_strpath(self);
return rb_rescue2(unlink_body, str, unlink_rescue, str, eENOTDIR, (VALUE)0);
}
descend() { |v| ... } Show source
迭代并为给定路径中的每个元素以降序生成新的Pathname对象。
Pathname.new('/path/to/some/file.rb').descend {|v| p v}
#<Pathname:/>
#<Pathname:/path>
#<Pathname:/path/to>
#<Pathname:/path/to/some>
#<Pathname:/path/to/some/file.rb>
Pathname.new('path/to/some/file.rb').descend {|v| p v}
#<Pathname:path>
#<Pathname:path/to>
#<Pathname:path/to/some>
#<Pathname:path/to/some/file.rb>
如果没有给出块,则返回枚举器。
enum = Pathname.new("/usr/bin/ruby").descend
# ... do stuff ...
enum.each { |e| ... }
# yields Pathnames /, /usr, /usr/bin, and /usr/bin/ruby.
它不访问文件系统。
# File ext/pathname/lib/pathname.rb, line 290
def descend
return to_enum(__method__) unless block_given?
vs = []
ascend {|v| vs << v }
vs.reverse_each {|v| yield v }
nil
end
directory?() Show source
See FileTest#directory?.
static VALUE
path_directory_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("directory?"), 1, get_strpath(self));
}
dirname() Show source
返回除路径最后一个组件外的所有内容。
请参阅File.dirname。
static VALUE
path_dirname(VALUE self)
{
VALUE str = get_strpath(self);
str = rb_funcall(rb_cFile, rb_intern("dirname"), 1, str);
return rb_class_new_instance(1, &str, rb_obj_class(self));
}
each_child(with_directory=true, &b) Show source
迭代目录的子目录(文件和子目录,而不是递归)。
它产生每个Pathname子对象。
默认情况下,产生的路径名将具有足够的信息来访问这些文件。
如果您设置with_directory
为false
,则返回的路径名将仅包含文件名。
Pathname("/usr/local").each_child {|f| p f }
#=> #<Pathname:/usr/local/share>
# #<Pathname:/usr/local/bin>
# #<Pathname:/usr/local/games>
# #<Pathname:/usr/local/lib>
# #<Pathname:/usr/local/include>
# #<Pathname:/usr/local/sbin>
# #<Pathname:/usr/local/src>
# #<Pathname:/usr/local/man>
Pathname("/usr/local").each_child(false) {|f| p f }
#=> #<Pathname:share>
# #<Pathname:bin>
# #<Pathname:games>
# #<Pathname:lib>
# #<Pathname:include>
# #<Pathname:sbin>
# #<Pathname:src>
# #<Pathname:man>
请注意,结果从不包含条目.
和..
目录中,因为它们不是子对象。
See #children
# File ext/pathname/lib/pathname.rb, line 488
def each_child(with_directory=true, &b)
children(with_directory).each(&b)
end
each_entry() Show source
遍历目录中的条目(文件和子目录),为每个条目生成一个Pathname对象。
static VALUE
path_each_entry(VALUE self)
{
VALUE args[1];
args[0] = get_strpath(self);
return rb_block_call(rb_cDir, rb_intern("foreach"), 1, args, each_entry_i, rb_obj_class(self));
}
each_filename() { |filename| ... } Show source
迭代路径的每个组件。
Pathname.new("/usr/bin/ruby").each_filename {|filename| ... }
# yields "usr", "bin", and "ruby".
如果没有给出块,则返回枚举器。
enum = Pathname.new("/usr/bin/ruby").each_filename
# ... do stuff ...
enum.each { |e| ... }
# yields "usr", "bin", and "ruby".
# File ext/pathname/lib/pathname.rb, line 258
def each_filename # :yield: filename
return to_enum(__method__) unless block_given?
_, names = split_names(@path)
names.each {|filename| yield filename }
nil
end
each_line {|line| ... } Show source
each_line(sep=$/ , open_args) {|line| block } → nil
each_line(limit , open_args) {|line| block } → nil
each_line(sep, limit , open_args) {|line| block } → nil
each_line(...) → an_enumerator
遍历文件中的每一行,并为每个行生成一个String对象。
static VALUE
path_each_line(int argc, VALUE *argv, VALUE self)
{
VALUE args[4];
int n;
args[0] = get_strpath(self);
n = rb_scan_args(argc, argv, "03", &args[1], &args[2], &args[3]);
if (rb_block_given_p()) {
return rb_block_call(rb_cIO, rb_intern("foreach"), 1+n, args, 0, 0);
}
else {
return rb_funcallv(rb_cIO, rb_intern("foreach"), 1+n, args);
}
}
empty?() Show source
测试文件是空的。
请参见Dir#空?和FileTest#空?
static VALUE
path_empty_p(VALUE self)
{
VALUE path = get_strpath(self);
if (RTEST(rb_funcall(rb_mFileTest, rb_intern("directory?"), 1, path)))
return rb_funcall(rb_cDir, rb_intern("empty?"), 1, path);
else
return rb_funcall(rb_mFileTest, rb_intern("empty?"), 1, path);
}
entries() Show source
返回目录中的条目(文件和子目录),每个条目都作为Pathname对象。
结果只包含目录中的名称,没有任何尾随斜杠或递归查找。
pp Pathname.new('/usr/local').entries
#=> [#<Pathname:share>,
# #<Pathname:lib>,
# #<Pathname:..>,
# #<Pathname:include>,
# #<Pathname:etc>,
# #<Pathname:bin>,
# #<Pathname:man>,
# #<Pathname:games>,
# #<Pathname:.>,
# #<Pathname:sbin>,
# #<Pathname:src>]
结果可能包含当前目录#<Pathname:.>
和父目录#<Pathname:..>
。
如果你不想要.
和..
想要目录,请考虑#children。
static VALUE
path_entries(VALUE self)
{
VALUE klass, str, ary;
long i;
klass = rb_obj_class(self);
str = get_strpath(self);
ary = rb_funcall(rb_cDir, rb_intern("entries"), 1, str);
ary = rb_convert_type(ary, T_ARRAY, "Array", "to_ary");
for (i = 0; i < RARRAY_LEN(ary); i++) {
VALUE elt = RARRAY_AREF(ary, i);
elt = rb_class_new_instance(1, &elt, klass);
rb_ary_store(ary, i, elt);
}
return ary;
}
eql?(p1) Show source
比较这个路径名other
。该比较是基于字符串的。请注意,两个不同的路径(foo.txt
和./foo.txt
)可以引用同一个文件。
static VALUE
path_eq(VALUE self, VALUE other)
{
if (!rb_obj_is_kind_of(other, rb_cPathname))
return Qfalse;
return rb_str_equal(get_strpath(self), get_strpath(other));
}
executable?() Show source
请参阅FileTest#可执行文件?
static VALUE
path_executable_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("executable?"), 1, get_strpath(self));
}
executable_real?() Show source
请参阅FileTest#executable_real ?.
static VALUE
path_executable_real_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("executable_real?"), 1, get_strpath(self));
}
exist?() Show source
请参阅FileTest#exists ?.
static VALUE
path_exist_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("exist?"), 1, get_strpath(self));
}
expand_path(p1 = v1) Show source
返回文件的绝对路径。
请参阅File.expand_path。
static VALUE
path_expand_path(int argc, VALUE *argv, VALUE self)
{
VALUE str = get_strpath(self);
VALUE dname;
if (rb_scan_args(argc, argv, "01", &dname) == 0)
str = rb_funcall(rb_cFile, rb_intern("expand_path"), 1, str);
else
str = rb_funcall(rb_cFile, rb_intern("expand_path"), 2, str, dname);
return rb_class_new_instance(1, &str, rb_obj_class(self));
}
extname() Show source
返回文件的扩展名。
请参阅File.extname。
static VALUE
path_extname(VALUE self)
{
VALUE str = get_strpath(self);
return rb_funcall(rb_cFile, rb_intern("extname"), 1, str);
}
file?() Show source
请参阅FileTest#文件?
static VALUE
path_file_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("file?"), 1, get_strpath(self));
}
find(ignore_error: true) { |pathname| ... } Show source
以深度优先方式遍历目录树,为“this”目录下的每个文件生成一个路径名。
如果没有给出块,则返回枚举器。
由于它是由标准库模块Find实现的,因此可以使用Find#prune来控制遍历。
如果self
是.
,产生的路径名以当前目录中的文件名开头,而不是./
。
请参阅查找#查找
# File ext/pathname/lib/pathname.rb, line 556
def find(ignore_error: true) # :yield: pathname
return to_enum(__method__, ignore_error: ignore_error) unless block_given?
require 'find'
if @path == '.'
Find.find(@path, ignore_error: ignore_error) {|f| yield self.class.new(f.sub(%r{\A\./}, '')) }
else
Find.find(@path, ignore_error: ignore_error) {|f| yield self.class.new(f) }
end
end
fnmatch(pattern, flags) → string Show source
fnmatch?(pattern, flags) → string
如果接收者匹配给定的模式,则返回true
。
请参阅File.fnmatch。
static VALUE
path_fnmatch(int argc, VALUE *argv, VALUE self)
{
VALUE str = get_strpath(self);
VALUE pattern, flags;
if (rb_scan_args(argc, argv, "11", &pattern, &flags) == 1)
return rb_funcall(rb_cFile, rb_intern("fnmatch"), 2, pattern, str);
else
return rb_funcall(rb_cFile, rb_intern("fnmatch"), 3, pattern, str, flags);
}
fnmatch?(pattern, flags) → string Show source
如果接收者匹配给定的模式,则返回true
。
请参阅File.fnmatch。
static VALUE
path_fnmatch(int argc, VALUE *argv, VALUE self)
{
VALUE str = get_strpath(self);
VALUE pattern, flags;
if (rb_scan_args(argc, argv, "11", &pattern, &flags) == 1)
return rb_funcall(rb_cFile, rb_intern("fnmatch"), 2, pattern, str);
else
return rb_funcall(rb_cFile, rb_intern("fnmatch"), 3, pattern, str, flags);
}
freeze → obj Show source
冻结此路径名。
请参阅对象#冻结。
static VALUE
path_freeze(VALUE self)
{
rb_call_super(0, 0);
rb_str_freeze(get_strpath(self));
return self;
}
ftype → string Show source
返回文件的“类型”(“文件”,“目录”等)。
请参阅File.ftype。
static VALUE
path_ftype(VALUE self)
{
return rb_funcall(rb_cFile, rb_intern("ftype"), 1, get_strpath(self));
}
grpowned?() Show source
请参阅FileTest#grpowned ?.
static VALUE
path_grpowned_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("grpowned?"), 1, get_strpath(self));
}
join(*args) Show source
将指定的路径名加入self
以创建新的路径名对象。
path0 = Pathname.new("/usr") # Pathname:/usr
path0 = path0.join("bin/ruby") # Pathname:/usr/bin/ruby
# is the same as
path1 = Pathname.new("/usr") + "bin/ruby" # Pathname:/usr/bin/ruby
path0 == path1
#=> true
# File ext/pathname/lib/pathname.rb, line 405
def join(*args)
return self if args.empty?
result = args.pop
result = Pathname.new(result) unless Pathname === result
return result if result.absolute?
args.reverse_each {|arg|
arg = Pathname.new(arg) unless Pathname === arg
result = arg + result
return result if result.absolute?
}
self + result
end
lchmod → integer Show source
与#chmod相同,但不符合符号链接。
请参阅File.lchmod。
static VALUE
path_lchmod(VALUE self, VALUE mode)
{
return rb_funcall(rb_cFile, rb_intern("lchmod"), 2, mode, get_strpath(self));
}
lchown → integer Show source
与#chown相同,但不符合符号链接。
请参阅File.lchown。
static VALUE
path_lchown(VALUE self, VALUE owner, VALUE group)
{
return rb_funcall(rb_cFile, rb_intern("lchown"), 3, owner, group, get_strpath(self));
}
lstat() Show source
请参阅File.lstat。
static VALUE
path_lstat(VALUE self)
{
return rb_funcall(rb_cFile, rb_intern("lstat"), 1, get_strpath(self));
}
make_link(old) Show source
在路径名中创建硬链接。
请参阅File.link。
static VALUE
path_make_link(VALUE self, VALUE old)
{
return rb_funcall(rb_cFile, rb_intern("link"), 2, old, get_strpath(self));
}
make_symlink(old) Show source
创建一个符号链接。
请参阅File.symlink。
static VALUE
path_make_symlink(VALUE self, VALUE old)
{
return rb_funcall(rb_cFile, rb_intern("symlink"), 2, old, get_strpath(self));
}
mkdir(p1 = v1) Show source
创建引用的目录。
请参阅Dir.mkdir。
static VALUE
path_mkdir(int argc, VALUE *argv, VALUE self)
{
VALUE str = get_strpath(self);
VALUE vmode;
if (rb_scan_args(argc, argv, "01", &vmode) == 0)
return rb_funcall(rb_cDir, rb_intern("mkdir"), 1, str);
else
return rb_funcall(rb_cDir, rb_intern("mkdir"), 2, str, vmode);
}
mkpath() Show source
创建完整路径,包括任何尚不存在的中间目录。
请参阅FileUtils#mkpath和FileUtils#mkdir_p
# File ext/pathname/lib/pathname.rb, line 573
def mkpath
require 'fileutils'
FileUtils.mkpath(@path)
nil
end
mountpoint?() Show source
如果self
指向一个安装点,则返回true
。
# File ext/pathname/lib/pathname.rb, line 189
def mountpoint?
begin
stat1 = self.lstat
stat2 = self.parent.lstat
stat1.dev == stat2.dev && stat1.ino == stat2.ino ||
stat1.dev != stat2.dev
rescue Errno::ENOENT
false
end
end
mtime → time Show source
返回文件的上次修改时间。
请参阅File.mtime。
static VALUE
path_mtime(VALUE self)
{
return rb_funcall(rb_cFile, rb_intern("mtime"), 1, get_strpath(self));
}
open(p1 = v1, p2 = v2, p3 = v3) Show source
打开文件进行阅读或写作。
请参阅File.open。
static VALUE
path_open(int argc, VALUE *argv, VALUE self)
{
VALUE args[4];
int n;
args[0] = get_strpath(self);
n = rb_scan_args(argc, argv, "03", &args[1], &args[2], &args[3]);
if (rb_block_given_p()) {
return rb_block_call(rb_cFile, rb_intern("open"), 1+n, args, 0, 0);
}
else {
return rb_funcallv(rb_cFile, rb_intern("open"), 1+n, args);
}
}
opendir() Show source
打开引用的目录。
请参阅Dir.open。
static VALUE
path_opendir(VALUE self)
{
VALUE args[1];
args[0] = get_strpath(self);
return rb_block_call(rb_cDir, rb_intern("open"), 1, args, 0, 0);
}
owned?() Show source
请参阅FileTest#拥有么?
static VALUE
path_owned_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("owned?"), 1, get_strpath(self));
}
parent() Show source
返回父目录。
这与之相同self + '..'
。
# File ext/pathname/lib/pathname.rb, line 184
def parent
self + '..'
end
pipe?() Show source
请参阅FileTest#pipe ?.
static VALUE
path_pipe_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("pipe?"), 1, get_strpath(self));
}
read([length , offset]) → string Show source
read([length , offset], open_args) → string
返回文件中的所有数据,N
如果指定了第一个字节。
请参阅IO.read。
static VALUE
path_read(int argc, VALUE *argv, VALUE self)
{
VALUE args[4];
int n;
args[0] = get_strpath(self);
n = rb_scan_args(argc, argv, "03", &args[1], &args[2], &args[3]);
return rb_funcallv(rb_cIO, rb_intern("read"), 1+n, args);
}
readable?() Show source
请参阅FileTest#可读?
static VALUE
path_readable_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("readable?"), 1, get_strpath(self));
}
readable_real?() Show source
请参阅FileTest#readable_real ?.
static VALUE
path_readable_real_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("readable_real?"), 1, get_strpath(self));
}
readlines(sep=$/ , open_args) → array Show source
readlines(limit , open_args) → array
readlines(sep, limit , open_args) → array
返回文件中的所有行。
参见IO.readlines。
static VALUE
path_readlines(int argc, VALUE *argv, VALUE self)
{
VALUE args[4];
int n;
args[0] = get_strpath(self);
n = rb_scan_args(argc, argv, "03", &args[1], &args[2], &args[3]);
return rb_funcallv(rb_cIO, rb_intern("readlines"), 1+n, args);
}
readlink() Show source
阅读符号链接。
请参阅File.readlink。
static VALUE
path_readlink(VALUE self)
{
VALUE str;
str = rb_funcall(rb_cFile, rb_intern("readlink"), 1, get_strpath(self));
return rb_class_new_instance(1, &str, rb_obj_class(self));
}
realdirpath(p1 = v1) Show source
返回self
实际文件系统中的真实(绝对)路径名。
不包含符号链接或无用的点..
和.
。
真实路径名的最后一个组件可能不存在。
static VALUE
path_realdirpath(int argc, VALUE *argv, VALUE self)
{
VALUE basedir, str;
rb_scan_args(argc, argv, "01", &basedir);
str = rb_funcall(rb_cFile, rb_intern("realdirpath"), 2, get_strpath(self), basedir);
return rb_class_new_instance(1, &str, rb_obj_class(self));
}
realpath(p1 = v1) Show source
返回self
实际文件系统中的真实(绝对)路径名。
不包含符号链接或无用的点..
和.
。
调用此方法时,路径名的所有组件都必须存在。
static VALUE
path_realpath(int argc, VALUE *argv, VALUE self)
{
VALUE basedir, str;
rb_scan_args(argc, argv, "01", &basedir);
str = rb_funcall(rb_cFile, rb_intern("realpath"), 2, get_strpath(self), basedir);
return rb_class_new_instance(1, &str, rb_obj_class(self));
}
relative?() Show source
与#absolute相反?
如果路径名以斜杠开始,则返回false
。
p = Pathname.new('/im/sure')
p.relative?
#=> false
p = Pathname.new('not/so/sure')
p.relative?
#=> true
# File ext/pathname/lib/pathname.rb, line 237
def relative?
path = @path
while r = chop_basename(path)
path, = r
end
path == ''
end
relative_path_from(base_directory) Show source
返回给定base_directory
与接收器之间的相对路径。
如果self
是绝对的,那么也base_directory
一定是绝对的。
如果self
是相对的,那么base_directory
一定是相对的。
此方法不访问文件系统。它假定没有符号链接。
当找不到相对路径时引发ArgumentError。
# File ext/pathname/lib/pathname.rb, line 503
def relative_path_from(base_directory)
dest_directory = self.cleanpath.to_s
base_directory = base_directory.cleanpath.to_s
dest_prefix = dest_directory
dest_names = []
while r = chop_basename(dest_prefix)
dest_prefix, basename = r
dest_names.unshift basename if basename != '.'
end
base_prefix = base_directory
base_names = []
while r = chop_basename(base_prefix)
base_prefix, basename = r
base_names.unshift basename if basename != '.'
end
unless SAME_PATHS[dest_prefix, base_prefix]
raise ArgumentError, "different prefix: #{dest_prefix.inspect} and #{base_directory.inspect}"
end
while !dest_names.empty? &&
!base_names.empty? &&
SAME_PATHS[dest_names.first, base_names.first]
dest_names.shift
base_names.shift
end
if base_names.include? '..'
raise ArgumentError, "base_directory has ..: #{base_directory.inspect}"
end
base_names.fill('..')
relpath_names = base_names + dest_names
if relpath_names.empty?
Pathname.new('.')
else
Pathname.new(File.join(*relpath_names))
end
end
rename(p1) Show source
重命名文件。
请参阅File.rename。
static VALUE
path_rename(VALUE self, VALUE to)
{
return rb_funcall(rb_cFile, rb_intern("rename"), 2, get_strpath(self), to);
}
rmdir() Show source
删除引用的目录。
请参阅Dir.rmdir。
static VALUE
path_rmdir(VALUE self)
{
return rb_funcall(rb_cDir, rb_intern("rmdir"), 1, get_strpath(self));
}
rmtree() Show source
递归删除一个目录,包括它下面的所有目录。
请参阅FileUtils#rm_r
# File ext/pathname/lib/pathname.rb, line 582
def rmtree
# The name "rmtree" is borrowed from File::Path of Perl.
# File::Path provides "mkpath" and "rmtree".
require 'fileutils'
FileUtils.rm_r(@path)
nil
end
root?() Show source
根目录的谓词方法。true
如果路径名由连续的斜杠组成,则返回。
它不访问文件系统。所以它可能会返回false
一些指向根的路径名,例如/usr/..
。
# File ext/pathname/lib/pathname.rb, line 207
def root?
!!(chop_basename(@path) == nil && /#{SEPARATOR_PAT}/o =~ @path)
end
setgid?() Show source
请参阅FileTest#setgid ?.
static VALUE
path_setgid_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("setgid?"), 1, get_strpath(self));
}
setuid?() Show source
请参阅FileTest#setuid ?.
static VALUE
path_setuid_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("setuid?"), 1, get_strpath(self));
}
size() Show source
请参阅FileTest#大小。
static VALUE
path_size(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("size"), 1, get_strpath(self));
}
size?() Show source
请参阅FileTest#size ?.
static VALUE
path_size_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("size?"), 1, get_strpath(self));
}
socket?() Show source
请参阅FileTest#socket ?.
static VALUE
path_socket_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("socket?"), 1, get_strpath(self));
}
split() Show source
返回数组中的dirname和basename。
请参阅File.split。
static VALUE
path_split(VALUE self)
{
VALUE str = get_strpath(self);
VALUE ary, dirname, basename;
ary = rb_funcall(rb_cFile, rb_intern("split"), 1, str);
ary = rb_check_array_type(ary);
dirname = rb_ary_entry(ary, 0);
basename = rb_ary_entry(ary, 1);
dirname = rb_class_new_instance(1, &dirname, rb_obj_class(self));
basename = rb_class_new_instance(1, &basename, rb_obj_class(self));
return rb_ary_new3(2, dirname, basename);
}
stat() Show source
返回一个File :: Stat对象。
请参阅File.stat。
static VALUE
path_stat(VALUE self)
{
return rb_funcall(rb_cFile, rb_intern("stat"), 1, get_strpath(self));
}
sticky?() Show source
请参阅FileTest#sticky ?.
static VALUE
path_sticky_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("sticky?"), 1, get_strpath(self));
}
sub(*args) Show source
返回一个由String#sub替代的路径名。
path1 = Pathname.new('/usr/bin/perl')
path1.sub('perl', 'ruby')
#=> #<Pathname:/usr/bin/ruby>
static VALUE
path_sub(int argc, VALUE *argv, VALUE self)
{
VALUE str = get_strpath(self);
if (rb_block_given_p()) {
str = rb_block_call(str, rb_intern("sub"), argc, argv, 0, 0);
}
else {
str = rb_funcallv(str, rb_intern("sub"), argc, argv);
}
return rb_class_new_instance(1, &str, rb_obj_class(self));
}
sub_ext(p1) Show source
返回一个repl
作为后缀添加到基本名称的路径名。
如果自己没有扩展部分,repl
则附加。
Pathname.new('/usr/bin/shutdown').sub_ext('.rb')
#=> #<Pathname:/usr/bin/shutdown.rb>
static VALUE
path_sub_ext(VALUE self, VALUE repl)
{
VALUE str = get_strpath(self);
VALUE str2;
long extlen;
const char *ext;
const char *p;
StringValue(repl);
p = RSTRING_PTR(str);
extlen = RSTRING_LEN(str);
ext = ruby_enc_find_extname(p, &extlen, rb_enc_get(str));
if (ext == NULL) {
ext = p + RSTRING_LEN(str);
}
else if (extlen <= 1) {
ext += extlen;
}
str2 = rb_str_subseq(str, 0, ext-p);
rb_str_append(str2, repl);
OBJ_INFECT(str2, str);
return rb_class_new_instance(1, &str2, rb_obj_class(self));
}
symlink?() Show source
请参阅FileTest#符号链接?.
static VALUE
path_symlink_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("symlink?"), 1, get_strpath(self));
}
sysopen([mode, perm]) → fixnum Show source
请参阅IO.sysopen。
static VALUE
path_sysopen(int argc, VALUE *argv, VALUE self)
{
VALUE args[3];
int n;
args[0] = get_strpath(self);
n = rb_scan_args(argc, argv, "02", &args[1], &args[2]);
return rb_funcallv(rb_cIO, rb_intern("sysopen"), 1+n, args);
}
taint → obj Show source
玷污这个路径名。
请参阅对象#污点。
static VALUE
path_taint(VALUE self)
{
rb_call_super(0, 0);
rb_obj_taint(get_strpath(self));
return self;
}
to_path → string Show source
以String形式返回路径。
#to_path被实现,因此路径名对象可用于File.open等。
static VALUE
path_to_s(VALUE self)
{
return rb_obj_dup(get_strpath(self));
}
to_s → string Show source
以String形式返回路径。
#to_path被实现,因此路径名对象可用于File.open等。
static VALUE
path_to_s(VALUE self)
{
return rb_obj_dup(get_strpath(self));
}
truncate(p1) Show source
将文件截断为length
字节。
请参阅File.truncate。
static VALUE
path_truncate(VALUE self, VALUE length)
{
return rb_funcall(rb_cFile, rb_intern("truncate"), 2, get_strpath(self), length);
}
unlink() Show source
如果self
是文件,则使用File.unlink删除文件或目录,或根据需要删除Dir.unlink。
static VALUE
path_unlink(VALUE self)
{
VALUE eENOTDIR = rb_const_get_at(rb_mErrno, rb_intern("ENOTDIR"));
VALUE str = get_strpath(self);
return rb_rescue2(unlink_body, str, unlink_rescue, str, eENOTDIR, (VALUE)0);
}
untaint → obj Show source
取消此路径名称。
请参阅Object#untaint。
static VALUE
path_untaint(VALUE self)
{
rb_call_super(0, 0);
rb_obj_untaint(get_strpath(self));
return self;
}
utime(p1, p2) Show source
更新文件的访问和修改时间。
请参阅File.utime。
static VALUE
path_utime(VALUE self, VALUE atime, VALUE mtime)
{
return rb_funcall(rb_cFile, rb_intern("utime"), 3, atime, mtime, get_strpath(self));
}
world_readable?() Show source
请参阅FileTest#world_readable ?.
static VALUE
path_world_readable_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("world_readable?"), 1, get_strpath(self));
}
world_writable?() Show source
请参阅FileTest#world_writable ?.
static VALUE
path_world_writable_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("world_writable?"), 1, get_strpath(self));
}
writable?() Show source
请参阅FileTest#可写吗?
static VALUE
path_writable_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("writable?"), 1, get_strpath(self));
}
writable_real?() Show source
请参阅FileTest#writable_real ?.
static VALUE
path_writable_real_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("writable_real?"), 1, get_strpath(self));
}
write(string, offset ) → fixnum Show source
write(string, offset, open_args ) → fixnum
写入contents
文件。
请参阅IO.write。
static VALUE
path_write(int argc, VALUE *argv, VALUE self)
{
VALUE args[4];
int n;
args[0] = get_strpath(self);
n = rb_scan_args(argc, argv, "03", &args[1], &args[2], &args[3]);
return rb_funcallv(rb_cIO, rb_intern("write"), 1+n, args);
}
zero?() Show source
请参阅FileTest#zero ?.
static VALUE
path_zero_p(VALUE self)
{
return rb_funcall(rb_mFileTest, rb_intern("zero?"), 1, get_strpath(self));
}
路径名 | Pathname相关
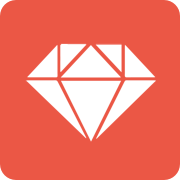
Ruby 是一种面向对象、命令式、函数式、动态的通用编程语言,是世界上最优美而巧妙的语言。
主页 | https://www.ruby-lang.org/ |
源码 | https://github.com/ruby/ruby |
版本 | 2.4 |
发布版本 | 2.4.1 |