Ruby 2.4参考手册
数学 | Math
CMath
包含的模块:Math
CMath是一个为复数提供三角函数和超越函数的函数库。该模块中的函数接受整数,浮点数或复数作为参数。
请注意,功能的选择与模块数学中的功能类似,但不完全相同。有两个模块的原因是一些用户对复数不感兴趣,甚至不知道它们是什么。他们宁愿让Math#sqrt引发异常,而不是返回一个复数。
欲了解更多信息,你可以参考Complex class。
用法
要开始使用这个库,只需要cmath库:
require "cmath"
公共类方法
acos(z) Show source
返回z的反余弦
CMath.acos(1 + 1i) #=> (0.9045568943023813-1.0612750619050357i)
# File lib/cmath.rb, line 280
def acos(z)
begin
if z.real? and z >= -1 and z <= 1
RealMath.acos(z)
else
(-1.0).i * log(z + 1.0.i * sqrt(1.0 - z * z))
end
rescue NoMethodError
handle_no_method_error
end
end
acosh(z) Show source
返回z的反双曲余弦
CMath.acosh(1 + 1i) #=> (1.0612750619050357+0.9045568943023813i)
# File lib/cmath.rb, line 345
def acosh(z)
begin
if z.real? and z >= 1
RealMath.acosh(z)
else
log(z + sqrt(z * z - 1.0))
end
rescue NoMethodError
handle_no_method_error
end
end
asin(z) Show source
返回z的反正弦
CMath.asin(1 + 1i) #=> (0.6662394324925153+1.0612750619050355i)
# File lib/cmath.rb, line 264
def asin(z)
begin
if z.real? and z >= -1 and z <= 1
RealMath.asin(z)
else
(-1.0).i * log(1.0.i * z + sqrt(1.0 - z * z))
end
rescue NoMethodError
handle_no_method_error
end
end
asinh(z) Show source
返回z的反双曲正弦
CMath.asinh(1 + 1i) #=> (1.0612750619050357+0.6662394324925153i)
# File lib/cmath.rb, line 329
def asinh(z)
begin
if z.real?
RealMath.asinh(z)
else
log(z + sqrt(1.0 + z * z))
end
rescue NoMethodError
handle_no_method_error
end
end
atan(z) Show source
返回z的反正切
CMath.atan(1 + 1i) #=> (1.0172219678978514+0.4023594781085251i)
# File lib/cmath.rb, line 296
def atan(z)
begin
if z.real?
RealMath.atan(z)
else
1.0.i * log((1.0.i + z) / (1.0.i - z)) / 2.0
end
rescue NoMethodError
handle_no_method_error
end
end
atan2(y,x) Show source
使用y和x的符号返回y除以x的反正切,以确定象限
CMath.atan2(1 + 1i, 0) #=> (1.5707963267948966+0.0i)
# File lib/cmath.rb, line 313
def atan2(y,x)
begin
if y.real? and x.real?
RealMath.atan2(y,x)
else
(-1.0).i * log((x + 1.0.i * y) / sqrt(x * x + y * y))
end
rescue NoMethodError
handle_no_method_error
end
end
atanh(z) Show source
返回z的反双曲正切
CMath.atanh(1 + 1i) #=> (0.4023594781085251+1.0172219678978514i)
# File lib/cmath.rb, line 361
def atanh(z)
begin
if z.real? and z >= -1 and z <= 1
RealMath.atanh(z)
else
log((1.0 + z) / (1.0 - z)) / 2.0
end
rescue NoMethodError
handle_no_method_error
end
end
cbrt(z) Show source
返回z的立方根的主值
CMath.cbrt(1 + 4i) #=> (1.449461632813119+0.6858152562177092i)
# File lib/cmath.rb, line 156
def cbrt(z)
z ** (1.0/3)
end
cos(z) Show source
返回z的余弦,其中z以弧度形式给出
CMath.cos(1 + 1i) #=> (0.8337300251311491-0.9888977057628651i)
# File lib/cmath.rb, line 181
def cos(z)
begin
if z.real?
RealMath.cos(z)
else
Complex(RealMath.cos(z.real) * RealMath.cosh(z.imag),
-RealMath.sin(z.real) * RealMath.sinh(z.imag))
end
rescue NoMethodError
handle_no_method_error
end
end
cosh(z) Show source
返回z的双曲余弦,其中z以弧度形式给出
CMath.cosh(1 + 1i) #=> (0.8337300251311491+0.9888977057628651i)
# File lib/cmath.rb, line 231
def cosh(z)
begin
if z.real?
RealMath.cosh(z)
else
Complex(RealMath.cosh(z.real) * RealMath.cos(z.imag),
RealMath.sinh(z.real) * RealMath.sin(z.imag))
end
rescue NoMethodError
handle_no_method_error
end
end
exp(z) Show source
Math :: E提升到 z 的平方
CMath.exp(1.i * Math::PI) #=> (-1.0+1.2246467991473532e-16i)
# File lib/cmath.rb, line 61
def exp(z)
begin
if z.real?
RealMath.exp(z)
else
ere = RealMath.exp(z.real)
Complex(ere * RealMath.cos(z.imag),
ere * RealMath.sin(z.imag))
end
rescue NoMethodError
handle_no_method_error
end
end
log(z, b=::Math::E) Show source
返回Complex的自然对数。如果给出第二个参数,它将是对数的基础。
CMath.log(1 + 4i) #=> (1.416606672028108+1.3258176636680326i)
CMath.log(1 + 4i, 10) #=> (0.6152244606891369+0.5757952953408879i)
# File lib/cmath.rb, line 81
def log(z, b=::Math::E)
begin
if z.real? && z >= 0 && b >= 0
RealMath.log(z, b)
else
Complex(RealMath.log(z.abs), z.arg) / log(b)
end
rescue NoMethodError
handle_no_method_error
end
end
log10(z) Show source
返回z的基数10的对数
CMath.log10(-1) #=> (0.0+1.3643763538418412i)
# File lib/cmath.rb, line 113
def log10(z)
begin
if z.real? and z >= 0
RealMath.log10(z)
else
log(z) / RealMath.log(10)
end
rescue NoMethodError
handle_no_method_error
end
end
log2(z) Show source
返回z的基数2的对数
CMath.log2(-1) => (0.0+4.532360141827194i)
# File lib/cmath.rb, line 97
def log2(z)
begin
if z.real? and z >= 0
RealMath.log2(z)
else
log(z) / RealMath.log(2)
end
rescue NoMethodError
handle_no_method_error
end
end
sin(z) Show source
返回z的正弦值,其中z以弧度形式给出
CMath.sin(1 + 1i) #=> (1.2984575814159773+0.6349639147847361i)
# File lib/cmath.rb, line 164
def sin(z)
begin
if z.real?
RealMath.sin(z)
else
Complex(RealMath.sin(z.real) * RealMath.cosh(z.imag),
RealMath.cos(z.real) * RealMath.sinh(z.imag))
end
rescue NoMethodError
handle_no_method_error
end
end
sinh(z) Show source
返回z的双曲正弦,其中z以弧度形式给出
CMath.sinh(1 + 1i) #=> (0.6349639147847361+1.2984575814159773i)
# File lib/cmath.rb, line 214
def sinh(z)
begin
if z.real?
RealMath.sinh(z)
else
Complex(RealMath.sinh(z.real) * RealMath.cos(z.imag),
RealMath.cosh(z.real) * RealMath.sin(z.imag))
end
rescue NoMethodError
handle_no_method_error
end
end
sqrt(z) Show source
返回Complex的非负平方根。
CMath.sqrt(-1 + 0i) #=> 0.0+1.0i
# File lib/cmath.rb, line 129
def sqrt(z)
begin
if z.real?
if z < 0
Complex(0, RealMath.sqrt(-z))
else
RealMath.sqrt(z)
end
else
if z.imag < 0 ||
(z.imag == 0 && z.imag.to_s[0] == '-')
sqrt(z.conjugate).conjugate
else
r = z.abs
x = z.real
Complex(RealMath.sqrt((r + x) / 2.0), RealMath.sqrt((r - x) / 2.0))
end
end
rescue NoMethodError
handle_no_method_error
end
end
tan(z) Show source
返回z的正切值,其中z以弧度形式给出
CMath.tan(1 + 1i) #=> (0.27175258531951174+1.0839233273386943i)
# File lib/cmath.rb, line 198
def tan(z)
begin
if z.real?
RealMath.tan(z)
else
sin(z) / cos(z)
end
rescue NoMethodError
handle_no_method_error
end
end
tanh(z) Show source
返回z的双曲正切,其中z以弧度形式给出
CMath.tanh(1 + 1i) #=> (1.0839233273386943+0.27175258531951174i)
# File lib/cmath.rb, line 248
def tanh(z)
begin
if z.real?
RealMath.tanh(z)
else
sinh(z) / cosh(z)
end
rescue NoMethodError
handle_no_method_error
end
end
私有实例方法
acos(z) Show source
返回z的反余弦
CMath.acos(1 + 1i) #=> (0.9045568943023813-1.0612750619050357i)
# File lib/cmath.rb, line 280
def acos(z)
begin
if z.real? and z >= -1 and z <= 1
RealMath.acos(z)
else
(-1.0).i * log(z + 1.0.i * sqrt(1.0 - z * z))
end
rescue NoMethodError
handle_no_method_error
end
end
acosh(z) Show source
返回z的反双曲余弦
CMath.acosh(1 + 1i) #=> (1.0612750619050357+0.9045568943023813i)
# File lib/cmath.rb, line 345
def acosh(z)
begin
if z.real? and z >= 1
RealMath.acosh(z)
else
log(z + sqrt(z * z - 1.0))
end
rescue NoMethodError
handle_no_method_error
end
end
asin(z) Show source
返回z的反正弦
CMath.asin(1 + 1i) #=> (0.6662394324925153+1.0612750619050355i)
# File lib/cmath.rb, line 264
def asin(z)
begin
if z.real? and z >= -1 and z <= 1
RealMath.asin(z)
else
(-1.0).i * log(1.0.i * z + sqrt(1.0 - z * z))
end
rescue NoMethodError
handle_no_method_error
end
end
asinh(z) Show source
返回z的反双曲正弦
CMath.asinh(1 + 1i) #=> (1.0612750619050357+0.6662394324925153i)
# File lib/cmath.rb, line 329
def asinh(z)
begin
if z.real?
RealMath.asinh(z)
else
log(z + sqrt(1.0 + z * z))
end
rescue NoMethodError
handle_no_method_error
end
end
atan(z) Show source
返回z的反正切
CMath.atan(1 + 1i) #=> (1.0172219678978514+0.4023594781085251i)
# File lib/cmath.rb, line 296
def atan(z)
begin
if z.real?
RealMath.atan(z)
else
1.0.i * log((1.0.i + z) / (1.0.i - z)) / 2.0
end
rescue NoMethodError
handle_no_method_error
end
end
atan2(y,x) Show source
使用y和x的符号返回y除以x的反正切,以确定象限
CMath.atan2(1 + 1i, 0) #=> (1.5707963267948966+0.0i)
# File lib/cmath.rb, line 313
def atan2(y,x)
begin
if y.real? and x.real?
RealMath.atan2(y,x)
else
(-1.0).i * log((x + 1.0.i * y) / sqrt(x * x + y * y))
end
rescue NoMethodError
handle_no_method_error
end
end
atanh(z) Show source
返回z的反双曲正切
CMath.atanh(1 + 1i) #=> (0.4023594781085251+1.0172219678978514i)
# File lib/cmath.rb, line 361
def atanh(z)
begin
if z.real? and z >= -1 and z <= 1
RealMath.atanh(z)
else
log((1.0 + z) / (1.0 - z)) / 2.0
end
rescue NoMethodError
handle_no_method_error
end
end
cbrt(z) Show source
返回z的立方根的主值
CMath.cbrt(1 + 4i) #=> (1.449461632813119+0.6858152562177092i)
# File lib/cmath.rb, line 156
def cbrt(z)
z ** (1.0/3)
end
cos(z) Show source
返回z的余弦,其中z以弧度给出
CMath.cos(1 + 1i) #=> (0.8337300251311491-0.9888977057628651i)
# File lib/cmath.rb, line 181
def cos(z)
begin
if z.real?
RealMath.cos(z)
else
Complex(RealMath.cos(z.real) * RealMath.cosh(z.imag),
-RealMath.sin(z.real) * RealMath.sinh(z.imag))
end
rescue NoMethodError
handle_no_method_error
end
end
cosh(z) Show source
返回z的双曲余弦,其中z以弧度给出
CMath.cosh(1 + 1i) #=> (0.8337300251311491+0.9888977057628651i)
# File lib/cmath.rb, line 231
def cosh(z)
begin
if z.real?
RealMath.cosh(z)
else
Complex(RealMath.cosh(z.real) * RealMath.cos(z.imag),
RealMath.sinh(z.real) * RealMath.sin(z.imag))
end
rescue NoMethodError
handle_no_method_error
end
end
exp(z) Show source
Math :: E提升到z的平方
CMath.exp(1.i * Math::PI) #=> (-1.0+1.2246467991473532e-16i)
# File lib/cmath.rb, line 61
def exp(z)
begin
if z.real?
RealMath.exp(z)
else
ere = RealMath.exp(z.real)
Complex(ere * RealMath.cos(z.imag),
ere * RealMath.sin(z.imag))
end
rescue NoMethodError
handle_no_method_error
end
end
log(z, b=::Math::E) Show source
返回Complex的自然对数。如果给出第二个参数,它将是对数的基础。
CMath.log(1 + 4i) #=> (1.416606672028108+1.3258176636680326i)
CMath.log(1 + 4i, 10) #=> (0.6152244606891369+0.5757952953408879i)
# File lib/cmath.rb, line 81
def log(z, b=::Math::E)
begin
if z.real? && z >= 0 && b >= 0
RealMath.log(z, b)
else
Complex(RealMath.log(z.abs), z.arg) / log(b)
end
rescue NoMethodError
handle_no_method_error
end
end
log10(z) Show source
返回z的基数10的对数
CMath.log10(-1) #=> (0.0+1.3643763538418412i)
# File lib/cmath.rb, line 113
def log10(z)
begin
if z.real? and z >= 0
RealMath.log10(z)
else
log(z) / RealMath.log(10)
end
rescue NoMethodError
handle_no_method_error
end
end
log2(z) Show source
返回z的基数2的对数
CMath.log2(-1) => (0.0+4.532360141827194i)
# File lib/cmath.rb, line 97
def log2(z)
begin
if z.real? and z >= 0
RealMath.log2(z)
else
log(z) / RealMath.log(2)
end
rescue NoMethodError
handle_no_method_error
end
end
sin(z) Show source
返回z的正弦值,其中z以弧度给出
CMath.sin(1 + 1i) #=> (1.2984575814159773+0.6349639147847361i)
# File lib/cmath.rb, line 164
def sin(z)
begin
if z.real?
RealMath.sin(z)
else
Complex(RealMath.sin(z.real) * RealMath.cosh(z.imag),
RealMath.cos(z.real) * RealMath.sinh(z.imag))
end
rescue NoMethodError
handle_no_method_error
end
end
sinh(z) Show source
返回z的双曲正弦,其中z以弧度给出
CMath.sinh(1 + 1i) #=> (0.6349639147847361+1.2984575814159773i)
# File lib/cmath.rb, line 214
def sinh(z)
begin
if z.real?
RealMath.sinh(z)
else
Complex(RealMath.sinh(z.real) * RealMath.cos(z.imag),
RealMath.cosh(z.real) * RealMath.sin(z.imag))
end
rescue NoMethodError
handle_no_method_error
end
end
sqrt(z) Show source
返回Complex的非负平方根。
CMath.sqrt(-1 + 0i) #=> 0.0+1.0i
# File lib/cmath.rb, line 129
def sqrt(z)
begin
if z.real?
if z < 0
Complex(0, RealMath.sqrt(-z))
else
RealMath.sqrt(z)
end
else
if z.imag < 0 ||
(z.imag == 0 && z.imag.to_s[0] == '-')
sqrt(z.conjugate).conjugate
else
r = z.abs
x = z.real
Complex(RealMath.sqrt((r + x) / 2.0), RealMath.sqrt((r - x) / 2.0))
end
end
rescue NoMethodError
handle_no_method_error
end
end
tan(z) Show source
返回z的正切值,其中z以弧度给出
CMath.tan(1 + 1i) #=> (0.27175258531951174+1.0839233273386943i)
# File lib/cmath.rb, line 198
def tan(z)
begin
if z.real?
RealMath.tan(z)
else
sin(z) / cos(z)
end
rescue NoMethodError
handle_no_method_error
end
end
tanh(z) Show source
返回z的双曲正切,其中z以弧度给出
CMath.tanh(1 + 1i) #=> (1.0839233273386943+0.27175258531951174i)
# File lib/cmath.rb, line 248
def tanh(z)
begin
if z.real?
RealMath.tanh(z)
else
sinh(z) / cosh(z)
end
rescue NoMethodError
handle_no_method_error
end
end
数学 | Math相关
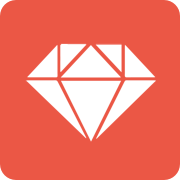
Ruby 是一种面向对象、命令式、函数式、动态的通用编程语言,是世界上最优美而巧妙的语言。
主页 | https://www.ruby-lang.org/ |
源码 | https://github.com/ruby/ruby |
版本 | 2.4 |
发布版本 | 2.4.1 |