Ruby 2.4参考手册
GC
GC::Profiler
GC分析器提供对GC运行信息的访问,包括时间,长度和对象空间大小。
示例:
GC::Profiler.enable
require 'rdoc/rdoc'
GC::Profiler.report
GC::Profiler.disable
另请参阅GC.count,GC.malloc_allocated_size和GC.malloc_allocations
Public Class Methods
GC::Profiler.clear → nil Show source
清除GC分析器数据。
static VALUE
gc_profile_clear(void)
{
rb_objspace_t *objspace = &rb_objspace;
if (GC_PROFILE_RECORD_DEFAULT_SIZE * 2 < objspace->profile.size) {
objspace->profile.size = GC_PROFILE_RECORD_DEFAULT_SIZE * 2;
objspace->profile.records = realloc(objspace->profile.records, sizeof(gc_profile_record) * objspace->profile.size);
if (!objspace->profile.records) {
rb_memerror();
}
}
MEMZERO(objspace->profile.records, gc_profile_record, objspace->profile.size);
objspace->profile.next_index = 0;
objspace->profile.current_record = 0;
return Qnil;
}
GC::Profiler.disable → nil Show source
停止GC分析器。
static VALUE
gc_profile_disable(void)
{
rb_objspace_t *objspace = &rb_objspace;
objspace->profile.run = FALSE;
objspace->profile.current_record = 0;
return Qnil;
}
GC::Profiler.enable → nil Show source
启动GC分析器。
static VALUE
gc_profile_enable(void)
{
rb_objspace_t *objspace = &rb_objspace;
objspace->profile.run = TRUE;
objspace->profile.current_record = 0;
return Qnil;
}
GC::Profiler.enabled? → true or false Show source
GC配置文件模式的当前状态。
static VALUE
gc_profile_enable_get(VALUE self)
{
rb_objspace_t *objspace = &rb_objspace;
return objspace->profile.run ? Qtrue : Qfalse;
}
GC::Profiler.raw_data → Hash, ...()
返回从最早到最后一个排序的各个原始配置文件数据哈希的数组:GC_INVOKE_TIME
。
例如:
[
{
:GC_TIME=>1.3000000000000858e-05,
:GC_INVOKE_TIME=>0.010634999999999999,
:HEAP_USE_SIZE=>289640,
:HEAP_TOTAL_SIZE=>588960,
:HEAP_TOTAL_OBJECTS=>14724,
:GC_IS_MARKED=>false
},
# ...
]
key的意思是:
:GC_TIME
此GC运行所用的时间,以秒为单位
:GC_INVOKE_TIME
从启动到调用GC所经历的时间(以秒为单位)
:HEAP_USE_SIZE
使用堆的总字节数
:HEAP_TOTAL_SIZE
以字节为单位的堆的总大小
:HEAP_TOTAL_OBJECTS
对象的总数
:GC_IS_MARKED
如果GC处于标记阶段,则返回true
如果使用ruby构建GC_PROFILE_MORE_DETAIL
,您还可以访问以下散列键:
:GC_MARK_TIME
:GC_SWEEP_TIME
:ALLOCATE_INCREASE
:ALLOCATE_LIMIT
:HEAP_USE_PAGES
:HEAP_LIVE_OBJECTS
:HEAP_FREE_OBJECTS
:HAVE_FINALIZE
static VALUE
gc_profile_record_get(void)
{
VALUE prof;
VALUE gc_profile = rb_ary_new();
size_t i;
rb_objspace_t *objspace = (&rb_objspace);
if (!objspace->profile.run) {
return Qnil;
}
for (i =0; i < objspace->profile.next_index; i++) {
gc_profile_record *record = &objspace->profile.records[i];
prof = rb_hash_new();
rb_hash_aset(prof, ID2SYM(rb_intern("GC_FLAGS")), gc_info_decode(0, rb_hash_new(), record->flags));
rb_hash_aset(prof, ID2SYM(rb_intern("GC_TIME")), DBL2NUM(record->gc_time));
rb_hash_aset(prof, ID2SYM(rb_intern("GC_INVOKE_TIME")), DBL2NUM(record->gc_invoke_time));
rb_hash_aset(prof, ID2SYM(rb_intern("HEAP_USE_SIZE")), SIZET2NUM(record->heap_use_size));
rb_hash_aset(prof, ID2SYM(rb_intern("HEAP_TOTAL_SIZE")), SIZET2NUM(record->heap_total_size));
rb_hash_aset(prof, ID2SYM(rb_intern("HEAP_TOTAL_OBJECTS")), SIZET2NUM(record->heap_total_objects));
rb_hash_aset(prof, ID2SYM(rb_intern("GC_IS_MARKED")), Qtrue);
#if GC_PROFILE_MORE_DETAIL
rb_hash_aset(prof, ID2SYM(rb_intern("GC_MARK_TIME")), DBL2NUM(record->gc_mark_time));
rb_hash_aset(prof, ID2SYM(rb_intern("GC_SWEEP_TIME")), DBL2NUM(record->gc_sweep_time));
rb_hash_aset(prof, ID2SYM(rb_intern("ALLOCATE_INCREASE")), SIZET2NUM(record->allocate_increase));
rb_hash_aset(prof, ID2SYM(rb_intern("ALLOCATE_LIMIT")), SIZET2NUM(record->allocate_limit));
rb_hash_aset(prof, ID2SYM(rb_intern("HEAP_USE_PAGES")), SIZET2NUM(record->heap_use_pages));
rb_hash_aset(prof, ID2SYM(rb_intern("HEAP_LIVE_OBJECTS")), SIZET2NUM(record->heap_live_objects));
rb_hash_aset(prof, ID2SYM(rb_intern("HEAP_FREE_OBJECTS")), SIZET2NUM(record->heap_free_objects));
rb_hash_aset(prof, ID2SYM(rb_intern("REMOVING_OBJECTS")), SIZET2NUM(record->removing_objects));
rb_hash_aset(prof, ID2SYM(rb_intern("EMPTY_OBJECTS")), SIZET2NUM(record->empty_objects));
rb_hash_aset(prof, ID2SYM(rb_intern("HAVE_FINALIZE")), (record->flags & GPR_FLAG_HAVE_FINALIZE) ? Qtrue : Qfalse);
#endif
#if RGENGC_PROFILE > 0
rb_hash_aset(prof, ID2SYM(rb_intern("OLD_OBJECTS")), SIZET2NUM(record->old_objects));
rb_hash_aset(prof, ID2SYM(rb_intern("REMEMBERED_NORMAL_OBJECTS")), SIZET2NUM(record->remembered_normal_objects));
rb_hash_aset(prof, ID2SYM(rb_intern("REMEMBERED_SHADY_OBJECTS")), SIZET2NUM(record->remembered_shady_objects));
#endif
rb_ary_push(gc_profile, prof);
}
return gc_profile;
}
GC::Profiler.report Show source
GC::Profiler.report(io)
将:: result写入$stdout
给定的IO对象。
static VALUE
gc_profile_report(int argc, VALUE *argv, VALUE self)
{
VALUE out;
if (argc == 0) {
out = rb_stdout;
}
else {
rb_scan_args(argc, argv, "01", &out);
}
gc_profile_dump_on(out, rb_io_write);
return Qnil;
}
GC::Profiler.result → String Show source
返回配置文件数据报告,例如:
GC 1 invokes.
Index Invoke Time(sec) Use Size(byte) Total Size(byte) Total Object GC time(ms)
1 0.012 159240 212940 10647 0.00000000000001530000
static VALUE
gc_profile_result(void)
{
VALUE str = rb_str_buf_new(0);
gc_profile_dump_on(str, rb_str_buf_append);
return str;
}
GC::Profiler.total_time → float Show source
在几秒钟内用于垃圾收集的总时间
static VALUE
gc_profile_total_time(VALUE self)
{
double time = 0;
rb_objspace_t *objspace = &rb_objspace;
if (objspace->profile.run && objspace->profile.next_index > 0) {
size_t i;
size_t count = objspace->profile.next_index;
for (i = 0; i < count; i++) {
time += objspace->profile.records[i].gc_time;
}
}
return DBL2NUM(time);
}
GC相关
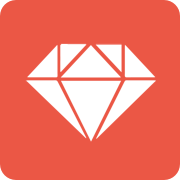
Ruby 是一种面向对象、命令式、函数式、动态的通用编程语言,是世界上最优美而巧妙的语言。
主页 | https://www.ruby-lang.org/ |
源码 | https://github.com/ruby/ruby |
版本 | 2.4 |
发布版本 | 2.4.1 |