Ruby 2.4参考手册
摘要 | Digest
Digest::Instance
该模块为摘要实现对象提供实例方法来计算消息摘要值。
公共实例方法
digest_obj << string → digest_obj Show source
使用给定的字符串 更新摘要并返回self。
update()方法和左移运算符被每个实现子类覆盖。(一个应该是另一个的别名)
static VALUE
rb_digest_instance_update(VALUE self, VALUE str)
{
rb_digest_instance_method_unimpl(self, "update");
UNREACHABLE;
}
digest_obj == another_digest_obj → boolean Show source
digest_obj == string → boolean
如果给出一个字符串,检查它是否等于摘要对象的十六进制编码的散列值。如果给出另一个摘要实例,则检查它们是否具有相同的散列值。否则返回false。
static VALUE
rb_digest_instance_equal(VALUE self, VALUE other)
{
VALUE str1, str2;
if (rb_obj_is_kind_of(other, rb_mDigest_Instance) == Qtrue) {
str1 = rb_digest_instance_digest(0, 0, self);
str2 = rb_digest_instance_digest(0, 0, other);
} else {
str1 = rb_digest_instance_to_s(self);
str2 = rb_check_string_type(other);
if (NIL_P(str2)) return Qfalse;
}
/* never blindly assume that subclass methods return strings */
StringValue(str1);
StringValue(str2);
if (RSTRING_LEN(str1) == RSTRING_LEN(str2) &&
rb_str_cmp(str1, str2) == 0) {
return Qtrue;
}
return Qfalse;
}
base64digest(str = nil) Show source
如果没有提供,则以base64编码形式返回摘要的结果哈希值,并保持摘要的状态。
如果给出a,则以base64编码形式返回给定的散列值,并在process.stringstring之前和之后将摘要重置为初始状态
在任何一种情况下,返回值都适当填充'=',并且不包含换行符。
# File ext/digest/lib/digest.rb, line 67
def base64digest(str = nil)
[str ? digest(str) : digest].pack('m0')
end
base64digest!() Show source
返回结果散列值并将摘要重置为初始状态。
# File ext/digest/lib/digest.rb, line 73
def base64digest!
[digest!].pack('m0')
end
block_length → integer Show source
返回摘要的块长度。
这个方法被每个实现子类覆盖。
static VALUE
rb_digest_instance_block_length(VALUE self)
{
rb_digest_instance_method_unimpl(self, "block_length");
UNREACHABLE;
}
bubblebabble → hash_string Show source
以Bubblebabble编码形式返回结果散列值。
static VALUE
rb_digest_instance_bubblebabble(VALUE self)
{
return bubblebabble_str_new(rb_funcall(self, id_digest, 0));
}
digest → string Show source
digest(string) → string
如果没有提供,则返回摘要的结果哈希值,保持摘要的状态。
如果给出了一个字符串,则返回给定字符串的散列值,并在该过程之前和之后将摘要重置为初始状态。
static VALUE
rb_digest_instance_digest(int argc, VALUE *argv, VALUE self)
{
VALUE str, value;
if (rb_scan_args(argc, argv, "01", &str) > 0) {
rb_funcall(self, id_reset, 0);
rb_funcall(self, id_update, 1, str);
value = rb_funcall(self, id_finish, 0);
rb_funcall(self, id_reset, 0);
} else {
value = rb_funcall(rb_obj_clone(self), id_finish, 0);
}
return value;
}
digest! → string Show source
返回结果散列值并将摘要重置为初始状态。
static VALUE
rb_digest_instance_digest_bang(VALUE self)
{
VALUE value = rb_funcall(self, id_finish, 0);
rb_funcall(self, id_reset, 0);
return value;
}
digest_length → integer Show source
返回摘要的哈希值的长度。
这个方法应该被每个实现子类覆盖。如果不是,则返回digest_obj.digest().length()。
static VALUE
rb_digest_instance_digest_length(VALUE self)
{
/* subclasses really should redefine this method */
VALUE digest = rb_digest_instance_digest(0, 0, self);
/* never blindly assume that #digest() returns a string */
StringValue(digest);
return INT2NUM(RSTRING_LEN(digest));
}
file(name) Show source
使用给定文件名的内容更新摘要并返回self。
# File ext/digest/lib/digest.rb, line 48
def file(name)
File.open(name, "rb") {|f|
buf = ""
while f.read(16384, buf)
update buf
end
}
self
end
hexdigest → string Show source
hexdigest(string) → string
如果没有提供,则以十六进制编码形式返回摘要的结果散列值,并保持摘要的状态。
如果给出一个字符串,则以十六进制编码形式返回给定字符串的散列值,并在该过程之前和之后将摘要重置为初始状态。
static VALUE
rb_digest_instance_hexdigest(int argc, VALUE *argv, VALUE self)
{
VALUE str, value;
if (rb_scan_args(argc, argv, "01", &str) > 0) {
rb_funcall(self, id_reset, 0);
rb_funcall(self, id_update, 1, str);
value = rb_funcall(self, id_finish, 0);
rb_funcall(self, id_reset, 0);
} else {
value = rb_funcall(rb_obj_clone(self), id_finish, 0);
}
return hexencode_str_new(value);
}
hexdigest! → string Show source
以十六进制编码形式返回结果散列值,并将摘要重置为初始状态。
static VALUE
rb_digest_instance_hexdigest_bang(VALUE self)
{
VALUE value = rb_funcall(self, id_finish, 0);
rb_funcall(self, id_reset, 0);
return hexencode_str_new(value);
}
inspect → string Show source
创建摘要对象的可输出版本。
static VALUE
rb_digest_instance_inspect(VALUE self)
{
VALUE str;
size_t digest_len = 32; /* about this size at least */
const char *cname;
cname = rb_obj_classname(self);
/* #<Digest::ClassName: xxxxx...xxxx> */
str = rb_str_buf_new(2 + strlen(cname) + 2 + digest_len * 2 + 1);
rb_str_buf_cat2(str, "#<");
rb_str_buf_cat2(str, cname);
rb_str_buf_cat2(str, ": ");
rb_str_buf_append(str, rb_digest_instance_hexdigest(0, 0, self));
rb_str_buf_cat2(str, ">");
return str;
}
length → integer Show source
返回digest_obj.digest_length()。
static VALUE
rb_digest_instance_length(VALUE self)
{
return rb_funcall(self, id_digest_length, 0);
}
new → another_digest_obj Show source
返回摘要对象的新的初始化副本。相当于digest_obj.clone()。reset()。
static VALUE
rb_digest_instance_new(VALUE self)
{
VALUE clone = rb_obj_clone(self);
rb_funcall(clone, id_reset, 0);
return clone;
}
reset → digest_obj Show source
将摘要重置为初始状态并返回自身。
这个方法被每个实现子类覆盖。
static VALUE
rb_digest_instance_reset(VALUE self)
{
rb_digest_instance_method_unimpl(self, "reset");
UNREACHABLE;
}
size → integer Show source
返回digest_obj.digest_length()。
static VALUE
rb_digest_instance_length(VALUE self)
{
return rb_funcall(self, id_digest_length, 0);
}
to_s → string Show source
返回digest_obj.hexdigest()。
static VALUE
rb_digest_instance_to_s(VALUE self)
{
return rb_funcall(self, id_hexdigest, 0);
}
update(string) → digest_obj Show source
使用给定的字符串更新摘要并返回自身。
update()方法和左移运算符被每个实现子类覆盖。(一个应该是另一个的别名)
static VALUE
rb_digest_instance_update(VALUE self, VALUE str)
{
rb_digest_instance_method_unimpl(self, "update");
UNREACHABLE;
}
私有实例方法
instance_eval { finish } → digest_obj Show source
完成摘要并返回结果散列值。
每个实现子类都会覆盖此方法,并且通常会被私有化,因为其中一些子类可能会使内部数据未初始化。不要从外面调用这个方法。使用digest!()代替,这样可确保内部数据因安全原因被重置。
static VALUE
rb_digest_instance_finish(VALUE self)
{
rb_digest_instance_method_unimpl(self, "finish");
UNREACHABLE;
}
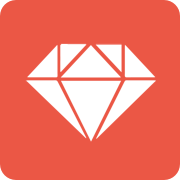
Ruby 是一种面向对象、命令式、函数式、动态的通用编程语言,是世界上最优美而巧妙的语言。
主页 | https://www.ruby-lang.org/ |
源码 | https://github.com/ruby/ruby |
版本 | 2.4 |
发布版本 | 2.4.1 |