Ruby 2.4参考手册
CSV
CSV::Row
Parent:ObjectIncluded modules:Enumerable
CSV :: Row是数组的一部分,也是散列的一部分。它保留字段的顺序并允许重复项像Array一样,但也允许您按名称访问字段,就像它们在哈希中一样。
如果激活了标题行处理,则由CSV返回的所有行都将从此类构建。
属性
rowR
用于比较相等的内部数据格式。
公共类方法
new(headers, fields, header_row = false) Show source
从构造一个新的CSV ::行headers
和fields
,其预计将阵列。如果一个数组比另一个数组短,它将被填充nil
物体。
可选header_row
参数可以设置为true
通过#header_row指示?和#field_row ?,这是一个标题行。否则,该行被假定为一个字段行。
CSV :: Row对象通过委托支持以下Array方法:
- empty?()
- length()
- size()
# File lib/csv.rb, line 235
def initialize(headers, fields, header_row = false)
@header_row = header_row
headers.each { |h| h.freeze if h.is_a? String }
# handle extra headers or fields
@row = if headers.size >= fields.size
headers.zip(fields)
else
fields.zip(headers).map { |pair| pair.reverse! }
end
end
公共实例方法
<<( field ) Show source
<<( header_and_field_array )
<<( header_and_field_hash )
如果提供了双元素数组,则假定它是一个标题和字段,并且该元素被添加。哈希以相同的方式工作,其中键为标题,值为字段。其他任何东西都被认为是一个单独的字段,并附有一个nil
标题。
此方法返回用于链接的行。
# File lib/csv.rb, line 380
def <<(arg)
if arg.is_a?(Array) and arg.size == 2 # appending a header and name
@row << arg
elsif arg.is_a?(Hash) # append header and name pairs
arg.each { |pair| @row << pair }
else # append field value
@row << [nil, arg]
end
self # for chaining
end
==(other) Show source
如果此行包含与<相同的顺序的标题和字段>返回true
。
# File lib/csv.rb, line 523
def ==(other)
return @row == other.row if other.is_a? CSV::Row
@row == other
end
Alias for: field
[]=( header, value ) Show source
[]=( header, offset, value )
[]=( index, value )
用#field中描述的语义查找字段并指定value
。
使用索引分配行的末尾将设置所有对之间的对[nil, nil]
。分配给未使用的标题会附加新对。
# File lib/csv.rb, line 347
def []=(*args)
value = args.pop
if args.first.is_a? Integer
if @row[args.first].nil? # extending past the end with index
@row[args.first] = [nil, value]
@row.map! { |pair| pair.nil? ? [nil, nil] : pair }
else # normal index assignment
@row[args.first][1] = value
end
else
index = index(*args)
if index.nil? # appending a field
self << [args.first, value]
else # normal header assignment
@row[index][1] = value
end
end
end
delete( header ) 显示源文件
delete( header, offset )
delete( index )
用于从header
或行中删除行中的一对index
。该对位于#field中。删除的一对会被返回,或者nil
如果找不到一对。
# File lib/csv.rb, line 415
def delete(header_or_index, minimum_index = 0)
if header_or_index.is_a? Integer # by index
@row.delete_at(header_or_index)
elsif i = index(header_or_index, minimum_index) # by header
@row.delete_at(i)
else
[ ]
end
end
delete_if(&block) 显示源文件
提供的内容block
是为行中的每一对传递一个标题和字段,并且预期会返回,true
或者false
取决于是否应删除该对。
此方法返回用于链接的行。
如果没有给出块,则返回枚举器。
# File lib/csv.rb, line 434
def delete_if(&block)
block or return enum_for(__method__) { size }
@row.delete_if(&block)
self # for chaining
end
each(&block) 显示源文件
将每一行的行作为标题和字段元组(就像迭代一个散列一样)。此方法返回用于链接的行。
如果没有给出块,则返回枚举器。
支持Enumerable。
# File lib/csv.rb, line 511
def each(&block)
block or return enum_for(__method__) { size }
@row.each(&block)
self # for chaining
end
fetch( header ) 显示源文件
fetch( header ) { |row| ... }
fetch( header, default )
该方法将通过获取字段值header
。它具有与Hash#fetch相同的行为:如果有给定的字段,header
则返回其值。否则,如果给出了一个块,它就会得到header
该结果并返回; 如果a default
作为第二个参数给出,则返回; 否则会引发KeyError。
# File lib/csv.rb, line 310
def fetch(header, *varargs)
raise ArgumentError, "Too many arguments" if varargs.length > 1
pair = @row.assoc(header)
if pair
pair.last
else
if block_given?
yield header
elsif varargs.empty?
raise KeyError, "key not found: #{header}"
else
varargs.first
end
end
end
field( header ) 显示源文件
field( header, offset )
field( index )
此方法将通过header
或返回字段值index
。如果找不到字段,nil
则返回。
提供时,offset
确保标题匹配发生在offset
索引上或晚于索引。您可以使用它来查找重复的标题,而不需要硬编码精确的索引。
# File lib/csv.rb, line 284
def field(header_or_index, minimum_index = 0)
# locate the pair
finder = (header_or_index.is_a?(Integer) || header_or_index.is_a?(Range)) ? :[] : :assoc
pair = @row[minimum_index..-1].send(finder, header_or_index)
# return the field if we have a pair
if pair.nil?
nil
else
header_or_index.is_a?(Range) ? pair.map(&:last) : pair.last
end
end
另外别名为:[]
field?(data) 显示源文件
如果data
与此行中的字段匹配则返回true
,false
否则返回。
# File lib/csv.rb, line 497
def field?(data)
fields.include? data
end
field_row?() 显示源文件
如果这是一个字段行,则返回true
。
# File lib/csv.rb, line 262
def field_row?
not header_row?
end
fields(*headers_and_or_indices) 显示源文件
此方法接受任意数量的参数,这些参数可以是标题,索引,任一范围或包含标题和偏移量的双元素数组。每个参数将被替换为字段查找,如#field中所述。
如果不带任何参数调用,则返回所有字段。
# File lib/csv.rb, line 450
def fields(*headers_and_or_indices)
if headers_and_or_indices.empty? # return all fields--no arguments
@row.map { |pair| pair.last }
else # or work like values_at()
headers_and_or_indices.inject(Array.new) do |all, h_or_i|
all + if h_or_i.is_a? Range
index_begin = h_or_i.begin.is_a?(Integer) ? h_or_i.begin :
index(h_or_i.begin)
index_end = h_or_i.end.is_a?(Integer) ? h_or_i.end :
index(h_or_i.end)
new_range = h_or_i.exclude_end? ? (index_begin...index_end) :
(index_begin..index_end)
fields.values_at(new_range)
else
[field(*Array(h_or_i))]
end
end
end
end
Also aliased as: values_at
has_key?(header) Show source
如何给定的字段header
,返回true
。
# File lib/csv.rb, line 327
def has_key?(header)
!!@row.assoc(header)
end
Also aliased as: include?, key?, member?
header?(name) 显示源文件
如果name
是此行的标题,则返回true
,false
否则返回。
# File lib/csv.rb, line 488
def header?(name)
headers.include? name
end
Also aliased as: include?
header_row?() 显示源文件
如果这是一个标题行,则返回true
。
# File lib/csv.rb, line 257
def header_row?
@header_row
end
headers() 显示源文件
返回此行的标题。
# File lib/csv.rb, line 267
def headers
@row.map { |pair| pair.first }
end
include?(header)
Alias for: has_key?
index( header ) 显示源文件
index( header, offset )
此方法将返回提供header
的字段的索引。offset
可用于定位的重复标题名称,如在#field说明。
# File lib/csv.rb, line 480
def index(header, minimum_index = 0)
# find the pair
index = headers[minimum_index..-1].index(header)
# return the index at the right offset, if we found one
index.nil? ? nil : index + minimum_index
end
inspect() 显示源文件
字段摘要,按照ASCII兼容的字符串标题。
# File lib/csv.rb, line 548
def inspect
str = ["#<", self.class.to_s]
each do |header, field|
str << " " << (header.is_a?(Symbol) ? header.to_s : header.inspect) <<
":" << field.inspect
end
str << ">"
begin
str.join('')
rescue # any encoding error
str.map do |s|
e = Encoding::Converter.asciicompat_encoding(s.encoding)
e ? s.encode(e) : s.force_encoding("ASCII-8BIT")
end.join('')
end
end
key?(header)
Alias for: has_key?
member?(header)
Alias for: has_key?
push(*args) 显示源文件
追加多个字段的快捷方式。相当于:
args.each { |arg| csv_row << arg }
此方法返回用于链接的行。
# File lib/csv.rb, line 399
def push(*args)
args.each { |arg| self << arg }
self # for chaining
end
to_csv(options = Hash.new) Show source
将该行作为CSV字符串返回。不使用标头。相当于:
csv_row.fields.to_csv( options )
# File lib/csv.rb, line 542
def to_csv(options = Hash.new)
fields.to_csv(options)
end
另外别名为:to_s
to_hash() Show source
将行折叠成简单的哈希。要警告的是,这放弃了现场秩序和clobbers重复的领域。
# File lib/csv.rb, line 532
def to_hash
# flatten just one level of the internal Array
Hash[*@row.inject(Array.new) { |ary, pair| ary.push(*pair) }]
end
to_s(options = Hash.new)
别名为:to_csv
values_at(*headers_and_or_indices)
别名为:字段
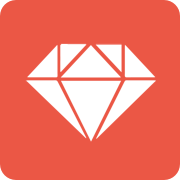
Ruby 是一种面向对象、命令式、函数式、动态的通用编程语言,是世界上最优美而巧妙的语言。
主页 | https://www.ruby-lang.org/ |
源码 | https://github.com/ruby/ruby |
版本 | 2.4 |
发布版本 | 2.4.1 |