React native参考手册
开始 | Getting Started
Props
大多数组件可以在创建时自定义,并具有不同的参数。这些创建参数被调用props
。
例如,一个基本的React Native组件是Image
。当您创建图像时,您可以使用一个指定的道具source
来控制它显示的图像。
import React, { Component } from 'react';
import { AppRegistry, Image } from 'react-native';
export default class Bananas extends Component {
render() {
let pic = {
uri: 'https://upload.wikimedia.org/wikipedia/commons/d/de/Bananavarieties.jpg'
};
return (
<Image source={pic} style={{width: 193, height: 110}}/>
);
}
}
// skip this line if using Create React Native App
AppRegistry.registerComponent('AwesomeProject', () => Bananas);
注意,{pic}
它被大括号包围,以便将该变量嵌入pic
到JSX中。您可以将任何JavaScript表达式放在JSX中的大括号内。
您自己的组件也可以使用props
。这使您可以制作应用中许多不同位置使用的单个组件,每个位置的属性略有不同。只需this.props
在您的render
功能中参考。这是一个例子:
import React, { Component } from 'react';
import { AppRegistry, Text, View } from 'react-native';
class Greeting extends Component {
render() {
return (
<Text>Hello {this.props.name}!</Text>
);
}
}
export default class LotsOfGreetings extends Component {
render() {
return (
<View style={{alignItems: 'center'}}>
<Greeting name='Rexxar' />
<Greeting name='Jaina' />
<Greeting name='Valeera' />
</View>
);
}
}
// skip this line if using Create React Native App
AppRegistry.registerComponent('AwesomeProject', () => LotsOfGreetings);
使用name
作为道具让我们自定义Greeting
组件,所以我们可以为每个问候重用该组件。这个例子也使用Greeting
JSX中的组件,就像内置组件一样。做到这一点的力量正是让React如此酷炫的原因 - 如果你发现自己希望有一组不同的用户界面原语可以使用,那么你只需要发明新的。
这里发生的另一件新事物是View
组件。一个View
可用作其他组件的容器,以帮助控制样式和布局。
使用props
和基本的Text
,Image
和View
组件,你可以建立各种各样的静态屏幕。要了解如何让您的应用随时间变化,您需要了解状态。
开始 | Getting Started相关
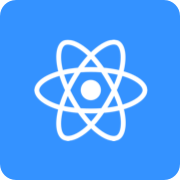
React Native 是一个 JavaScript 的框架,用来撰写实时的、可原生呈现 iOS 和 Android 的应用。
主页 | https://facebook.github.io/react-native/ |
源码 | https://github.com/facebook/react-native |
发布版本 | 0.49 |