React native参考手册
开始 | Getting Started
Networking
许多移动应用程序需要从远程URL加载资源。您可能希望向REST API发出POST请求,或者您可能只需从另一台服务器获取大量静态内容。
使用Fetch
React Native 为您的网络需求提供Fetch API。如果您以前使用过XMLHttpRequest
或其他网络API,Fetch看起来会很熟悉。有关更多信息,请参阅MDN的使用提取指南。
发出请求
为了从任意网址获取内容,只需传递网址即可获取:
fetch('https://mywebsite.com/mydata.json')
取指还采用可选的第二个参数,它允许您自定义HTTP请求。您可能需要指定其他标头,或发出POST请求:
fetch('https://mywebsite.com/endpoint/', {
method: 'POST',
headers: {
'Accept': 'application/json',
'Content-Type': 'application/json',
},
body: JSON.stringify({
firstParam: 'yourValue',
secondParam: 'yourOtherValue',
})
})
查看获取请求文档以获取完整的属性列表。
处理响应
以上示例显示了如何提出请求。在很多情况下,你会想要做出回应。
网络是一种固有的异步操作。提取方法将返回一个Promise,这使得它可以直接编写以异步方式工作的代码:
function getMoviesFromApiAsync() {
return fetch('https://facebook.github.io/react-native/movies.json')
.then((response) => response.json())
.then((responseJson) => {
return responseJson.movies;
})
.catch((error) => {
console.error(error);
});
}
您也可以在React Native应用程序中使用建议的ES2017 async
/ await
语法:
async function getMoviesFromApi() {
try {
let response = await fetch('https://facebook.github.io/react-native/movies.json');
let responseJson = await response.json();
return responseJson.movies;
} catch(error) {
console.error(error);
}
}
不要忘记捕捉任何可能被抛出的错误fetch
,否则它们会被默默地抛弃。
import React, { Component } from 'react';
import { ActivityIndicator, ListView, Text, View } from 'react-native';
export default class Movies extends Component {
constructor(props) {
super(props);
this.state = {
isLoading: true
}
}
componentDidMount() {
return fetch('https://facebook.github.io/react-native/movies.json')
.then((response) => response.json())
.then((responseJson) => {
let ds = new ListView.DataSource({rowHasChanged: (r1, r2) => r1 !== r2});
this.setState({
isLoading: false,
dataSource: ds.cloneWithRows(responseJson.movies),
}, function() {
// do something with new state
});
})
.catch((error) => {
console.error(error);
});
}
render() {
if (this.state.isLoading) {
return (
<View style={{flex: 1, paddingTop: 20}}>
<ActivityIndicator />
</View>
);
}
return (
<View style={{flex: 1, paddingTop: 20}}>
<ListView
dataSource={this.state.dataSource}
renderRow={(rowData) => <Text>{rowData.title}, {rowData.releaseYear}</Text>}
/>
</View>
);
}
}
默认情况下,iOS会阻止任何未使用SSL加密的请求。如果您需要从明文网址(以该网址开始
http
)获取数据,则首先需要添加App Transport Security异常。如果您事先知道需要访问的域名,那么为这些域名添加例外情况会更加安全; 如果域直到运行时才知道,则可以完全禁用ATS。不过请注意,从2017年1月起,苹果的App Store审查将需要合理的理由来禁用ATS。有关更多信息,请参阅Apple的文档。
使用其他网络库
在XMLHttpRequest的API是建立在反应原住民。这意味着您可以使用第三方库(例如依赖它的飞盘或axios),或者如果您愿意,可以直接使用XMLHttpRequest API。
var request = new XMLHttpRequest();
request.onreadystatechange = (e) => {
if (request.readyState !== 4) {
return;
}
if (request.status === 200) {
console.log('success', request.responseText);
} else {
console.warn('error');
}
};
request.open('GET', 'https://mywebsite.com/endpoint/');
request.send();
XMLHttpRequest的安全模型与Web上的安全模型不同,因为在本机应用程序中没有CORS的概念。
WebSocket支持
React Native还支持WebSockets,这是一种通过单个TCP连接提供全双工通信通道的协议。
var ws = new WebSocket('ws://host.com/path');
ws.onopen = () => {
// connection opened
ws.send('something'); // send a message
};
ws.onmessage = (e) => {
// a message was received
console.log(e.data);
};
ws.onerror = (e) => {
// an error occurred
console.log(e.message);
};
ws.onclose = (e) => {
// connection closed
console.log(e.code, e.reason);
};
举手击掌!
如果你通过阅读教程得到了线性的结果,那么你是一个非常令人印象深刻的人。恭喜。接下来,您可能想查看社区用React Native所做的所有酷炫事情。
开始 | Getting Started相关
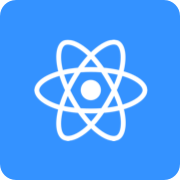
React Native 是一个 JavaScript 的框架,用来撰写实时的、可原生呈现 iOS 和 Android 的应用。
主页 | https://facebook.github.io/react-native/ |
源码 | https://github.com/facebook/react-native |
发布版本 | 0.49 |