React native参考手册
开始 | Getting Started
Handling Touches
用户主要通过触摸与移动应用进行交互。他们可以使用手势组合,例如点击按钮,滚动列表或缩放地图。React Native提供了处理各种常见手势的组件,以及一个综合的手势响应系统以允许更高级的手势识别,但是您最可能感兴趣的一个组件是基本Button。
显示一个基本按钮
按钮提供了一个基本的按钮组件,可以在所有平台上很好地呈现。显示按钮的最小示例如下所示:
<Button
onPress={() => { Alert.alert('You tapped the button!')}}
title="Press Me"
/>
这将在iOS上呈现蓝色标签,并在Android上呈现蓝色圆角矩形,并显示白色文字。按下按钮将会调用“onPress”功能,在这种情况下会显示一个警报弹出窗口。如果你喜欢,你可以指定一个“颜色”道具来改变你的按钮的颜色。
继续Button
使用下面的示例演示组件。您可以通过点击右下角的切换选择您的应用预览的平台,然后点击“点击播放”预览该应用。
import React, { Component } from 'react';
import { Alert, AppRegistry, Button, StyleSheet, View } from 'react-native';
export default class ButtonBasics extends Component {
_onPressButton() {
Alert.alert('You tapped the button!')
}
render() {
return (
<View style={styles.container}>
<View style={styles.buttonContainer}>
<Button
onPress={this._onPressButton}
title="Press Me"
/>
</View>
<View style={styles.buttonContainer}>
<Button
onPress={this._onPressButton}
title="Press Me"
color="#841584"
/>
</View>
<View style={styles.alternativeLayoutButtonContainer}>
<Button
onPress={this._onPressButton}
title="This looks great!"
/>
<Button
onPress={this._onPressButton}
title="OK!"
color="#841584"
/>
</View>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
},
buttonContainer: {
margin: 20
},
alternativeLayoutButtonContainer: {
margin: 20,
flexDirection: 'row',
justifyContent: 'space-between'
}
})
// skip this line if using Create React Native App
AppRegistry.registerComponent('AwesomeProject', () => ButtonBasics);
可触摸
如果基本按钮看起来不适合您的应用,则可以使用React Native提供的任何“可触摸”组件构建自己的按钮。“可触摸”组件提供捕捉敲击手势的功能,并且可以在识别手势时显示反馈。但是,这些组件不提供任何默认样式,因此您需要做一些工作才能让它们在您的应用中很好地显示。
您使用哪种“可触摸”组件取决于您想要提供的反馈类型:
- 一般来说,您可以在任何地方使用TouchableHighlight,在网页上使用按钮或链接。当用户按下按钮时,视图的背景会变暗。
- 您可以考虑在Android上使用TouchableNativeFeedback来显示响应用户触摸的墨水表面反应波纹。
- TouchableOpacity可用于通过减少按钮的不透明度来提供反馈,从而在用户按下时可以看到背景。
- 如果您需要处理轻按手势,但不想显示任何反馈,请使用TouchableWithoutFeedback。
在某些情况下,您可能需要检测用户何时按下并保持一定时间的视图。这些长时间按压可以通过将功能传递给onLongPress
任何“可触摸”组件的道具来处理。
让我们看看所有这些在行动:
import React, { Component } from 'react';
import { Alert, AppRegistry, Platform, StyleSheet, Text, TouchableHighlight, TouchableOpacity, TouchableNativeFeedback, TouchableWithoutFeedback, View } from 'react-native';
export default class Touchables extends Component {
_onPressButton() {
Alert.alert('You tapped the button!')
}
_onLongPressButton() {
Alert.alert('You long-pressed the button!')
}
render() {
return (
<View style={styles.container}>
<TouchableHighlight onPress={this._onPressButton} underlayColor="white">
<View style={styles.button}>
<Text style={styles.buttonText}>TouchableHighlight</Text>
</View>
</TouchableHighlight>
<TouchableOpacity onPress={this._onPressButton}>
<View style={styles.button}>
<Text style={styles.buttonText}>TouchableOpacity</Text>
</View>
</TouchableOpacity>
<TouchableNativeFeedback
onPress={this._onPressButton}
background={Platform.OS === 'android' ? TouchableNativeFeedback.SelectableBackground() : ''}>
<View style={styles.button}>
<Text style={styles.buttonText}>TouchableNativeFeedback</Text>
</View>
</TouchableNativeFeedback>
<TouchableWithoutFeedback
onPress={this._onPressButton}
>
<View style={styles.button}>
<Text style={styles.buttonText}>TouchableWithoutFeedback</Text>
</View>
</TouchableWithoutFeedback>
<TouchableHighlight onPress={this._onPressButton} onLongPress={this._onLongPressButton} underlayColor="white">
<View style={styles.button}>
<Text style={styles.buttonText}>Touchable with Long Press</Text>
</View>
</TouchableHighlight>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
paddingTop: 60,
alignItems: 'center'
},
button: {
marginBottom: 30,
width: 260,
alignItems: 'center',
backgroundColor: '#2196F3'
},
buttonText: {
padding: 20,
color: 'white'
}
})
// skip this line if using Create React Native App
AppRegistry.registerComponent('AwesomeProject', () => Touchables);
滚动列表,滑动页面和捏缩放
移动应用中常用的另一个手势是滑动或平移。该手势允许用户滚动浏览项目列表,或者滑过内容页面。为了处理这些和其他手势,我们将学习如何使用ScrollView。
开始 | Getting Started相关
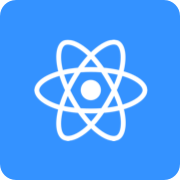
React Native 是一个 JavaScript 的框架,用来撰写实时的、可原生呈现 iOS 和 Android 的应用。
主页 | https://facebook.github.io/react-native/ |
源码 | https://github.com/facebook/react-native |
发布版本 | 0.49 |