React native参考手册
开始 | Getting Started
Handling Text Input
TextInput
是允许用户输入文本的基本组件。它有一个onChangeText
道具,它在每次文本改变onSubmitEditing
时都会调用一个函数,并在提交文本时使用一个支持调用函数的道具。
例如,假设用户键入的内容是将他们的单词翻译为不同的语言。在这种新语言中,每一个单词都以相同的方式写成:?。所以句子“你好,鲍勃”将被翻译为“???”。
import React, { Component } from 'react';
import { AppRegistry, Text, TextInput, View } from 'react-native';
export default class PizzaTranslator extends Component {
constructor(props) {
super(props);
this.state = {text: ''};
}
render() {
return (
<View style={{padding: 10}}>
<TextInput
style={{height: 40}}
placeholder="Type here to translate!"
onChangeText={(text) => this.setState({text})}
/>
<Text style={{padding: 10, fontSize: 42}}>
{this.state.text.split(' ').map((word) => word && '?').join(' ')}
</Text>
</View>
);
}
}
// skip this line if using Create React Native App
AppRegistry.registerComponent('AwesomeProject', () => PizzaTranslator);
在这个例子中,我们存储text
在状态中,因为它随时间而变化。
对于文本输入你可能想要做更多的事情。例如,您可以在用户输入时验证里面的文本。有关更详细的示例,请参阅受控组件上的React文档或TextInput的参考文档。
文本输入是用户与应用交互的方式之一。接下来,让我们看看另一种输入类型,并学习如何处理触摸。
开始 | Getting Started相关
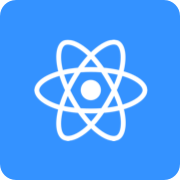
React Native 是一个 JavaScript 的框架,用来撰写实时的、可原生呈现 iOS 和 Android 的应用。
主页 | https://facebook.github.io/react-native/ |
源码 | https://github.com/facebook/react-native |
发布版本 | 0.49 |