Electron参考手册
指南 | Guides
使用Selenium和WebDriver | Using Selenium and WebDriver
WebDriver是一个开源工具,用于在许多浏览器上自动测试Web应用程序。它提供了导航到网页,用户输入,JavaScript执行等功能。ChromeDriver是一个独立的服务器,它为Chromium实现WebDriver的有线协议。它由Chromium和WebDriver团队的成员开发。
设置Spectron
Spectron是Electron官方支持的ChromeDriver测试框架。它建立在WebdriverIO之上,并且有助手在您的测试中访问Electron API并捆绑ChromeDriver。
$ npm install --save-dev spectron
// A simple test to verify a visible window is opened with a title
var Application = require('spectron').Application
var assert = require('assert')
var app = new Application({
path: '/Applications/MyApp.app/Contents/MacOS/MyApp'
})
app.start().then(function () {
// Check if the window is visible
return app.browserWindow.isVisible()
}).then(function (isVisible) {
// Verify the window is visible
assert.equal(isVisible, true)
}).then(function () {
// Get the window's title
return app.client.getTitle()
}).then(function (title) {
// Verify the window's title
assert.equal(title, 'My App')
}).catch(function (error) {
// Log any failures
console.error('Test failed', error.message)
}).then(function () {
// Stop the application
return app.stop()
})
使用WebDriverJs进行设置
WebDriverJs提供了一个用于测试Web驱动程序的节点包,我们将以此为例。
1.启动ChromeDriver
首先,您需要下载chromedriver
二进制文件,并运行它:
$ npm install electron-chromedriver
$ ./node_modules/.bin/chromedriver
Starting ChromeDriver (v2.10.291558) on port 9515
Only local connections are allowed.
记住端口号9515
,稍后会使用它
2.安装WebDriverJS
$ npm install selenium-webdriver
3.连接到ChromeDriver
selenium-webdriver
Electron 的使用与上游基本相同,不同之处在于您必须手动指定如何连接镀铬驱动程序以及在哪里找到Electron的二进制文件:
const webdriver = require('selenium-webdriver')
const driver = new webdriver.Builder()
// The "9515" is the port opened by chrome driver.
.usingServer('http://localhost:9515')
.withCapabilities({
chromeOptions: {
// Here is the path to your Electron binary.
binary: '/Path-to-Your-App.app/Contents/MacOS/Electron'
}
})
.forBrowser('electron')
.build()
driver.get('http://www.google.com')
driver.findElement(webdriver.By.name('q')).sendKeys('webdriver')
driver.findElement(webdriver.By.name('btnG')).click()
driver.wait(() => {
return driver.getTitle().then((title) => {
return title === 'webdriver - Google Search'
})
}, 1000)
driver.quit()
使用WebdriverIO进行设置
WebdriverIO提供了一个Node包用于使用Web驱动程序进行测试。
1.启动ChromeDriver
首先,您需要下载chromedriver
二进制文件,然后运行它:
$ npm install electron-chromedriver
$ ./node_modules/.bin/chromedriver --url-base=wd/hub --port=9515
Starting ChromeDriver (v2.10.291558) on port 9515
Only local connections are allowed.
记住端口号9515
,将在以后使用。
2.安装WebdriverIO
$ npm install webdriverio
3.连接到 chrome driver
const webdriverio = require('webdriverio')
const options = {
host: 'localhost', // Use localhost as chrome driver server
port: 9515, // "9515" is the port opened by chrome driver.
desiredCapabilities: {
browserName: 'chrome',
chromeOptions: {
binary: '/Path-to-Your-App/electron', // Path to your Electron binary.
args: [/* cli arguments */] // Optional, perhaps 'app=' + /path/to/your/app/
}
}
}
let client = webdriverio.remote(options)
client
.init()
.url('http://google.com')
.setValue('#q', 'webdriverio')
.click('#btnG')
.getTitle().then((title) => {
console.log('Title was: ' + title)
})
.end()
工作流程
要在不重建Electron的情况下测试您的应用程序,只需将您的应用程序源放入Electron的资源目录。
或者,传递参数以使用指向应用文件夹的电子二进制文件运行。这消除了将您的应用程序复制粘贴到Electron资源目录中的需要。
指南 | Guides相关
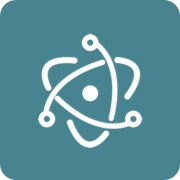
Electron 是一个使用 JavaScript, HTML 和 CSS 等 Web 技术创建原生程序的框架,它负责比较难搞的部分,你只需把精力放在你的应用的核心上即可。
主页 | https://electron.atom.io/ |
源码 | https://github.com/electron/electron |
发布版本 | 1.7.9 |