Electron参考手册
Tray
Tray
将图标和上下文菜单添加到系统的通知区域。
Process: Main
Tray
是一个 EventEmitter。
const {app, Menu, Tray} = require('electron')
let tray = null
app.on('ready', () => {
tray = new Tray('/path/to/my/icon')
const contextMenu = Menu.buildFromTemplate([
{label: 'Item1', type: 'radio'},
{label: 'Item2', type: 'radio'},
{label: 'Item3', type: 'radio', checked: true},
{label: 'Item4', type: 'radio'}
])
tray.setToolTip('This is my application.')
tray.setContextMenu(contextMenu)
})
Platform limitations:
- 在Linux上,应用程序指示符将在支持时使用,否则
GtkStatusIcon
将用于替代。 - 在仅支持应用程序指标的Linux发行版中,必须安装
libappindicator1
才能使托盘图标正常工作。 - 应用程序指示器仅在具有上下文菜单时才会显示。
- 当在Linux上使用应用程序指示符时,该
click
事件将被忽略。 - 在Linux上,为了使个人
MenuItem
的更改生效,您必须setContextMenu
再次调用。例如:
const {app, Menu, Tray} = require('electron')
let appIcon = null
app.on('ready', () => {
appIcon = new Tray('/path/to/my/icon')
const contextMenu = Menu.buildFromTemplate([
{label: 'Item1', type: 'radio'},
{label: 'Item2', type: 'radio'}
])
// Make a change to the context menu
contextMenu.items[1].checked = false
// Call this again for Linux because we modified the context menu
appIcon.setContextMenu(contextMenu)
})
- 在Windows上,建议使用
ICO
图标以获得最佳的视觉效果。
如果您想要在所有平台上保持完全相同的行为,则不应该依赖该click
事件并始终将上下文菜单附加到托盘图标上。
new Tray(image)
-
image
(NativeImage | String)
创建一个与该关联的新托盘图标image
。
Instance Events
该Tray
模块发出以下事件:
Event: ‘click’
-
event
事件-
altKey
布尔 -
shiftKey
布尔 -
ctrlKey
布尔 -
metaKey
布尔
-
-
bounds
矩形 - 托盘图标的边界
点击托盘图标时发出。
Event: ‘right-click’ macOS Windows
-
event
事件-
altKey
布尔 -
shiftKey
布尔 -
ctrlKey
布尔 -
metaKey
布尔
-
-
bounds
矩形 - 托盘图标的边界
右键单击托盘图标时发出。
Event: ‘double-click’ macOS Windows
-
event
事件-
altKey
布尔 -
shiftKey
布尔 -
ctrlKey
布尔 -
metaKey
布尔
-
-
bounds
矩形 - 托盘图标的边界
当双击托盘图标时发出。
Event: ‘balloon-show’ Windows
托盘气球显示时发出。
Event: ‘balloon-click’ Windows
点击托盘气球时发出。
Event: ‘balloon-closed’ Windows
当托盘气球由于超时而关闭时发出,或者用户手动关闭托盘气球。
Event: ‘drop’ macOS
当任何拖动项目被拖放到托盘图标上时发出。
Event: ‘drop-files’ macOS
-
event
Event -
files
String[] - The paths of the dropped files.
拖动文件放入托盘图标时发出。
Event: ‘drop-text’ macOS
-
event
Event -
text
String - the dropped text string
当拖动的文字被放入托盘图标时发出。
Event: ‘drag-enter’ macOS
当拖动操作进入托盘图标时发出。
Event: ‘drag-leave’ macOS
当拖动操作退出托盘图标时发出。
Event: ‘drag-end’ macOS
拖动操作在托盘上结束或在其他位置结束时发出。
Event: ‘mouse-enter’ macOS
-
event
事件-
altKey
布尔 -
shiftKey
布尔 -
ctrlKey
布尔 -
metaKey
布尔
-
-
position
Point - 事件的位置
鼠标进入托盘图标时发射。
Event: ‘mouse-leave’ macOS
-
event
事件-
altKey
布尔 -
shiftKey
布尔 -
ctrlKey
布尔 -
metaKey
布尔
-
-
position
Point - 事件的位置
鼠标退出托盘图标时发出。
Instance Methods
该Tray
班有以下方法:
tray.destroy()
立即销毁托盘图标。
tray.setImage(image)
-
image
(NativeImage | String)
设置image
与此托盘图标关联。
tray.setPressedImage(image)
macOS
-
image
NativeImage
image
在 macOS 上按下时设置与此托盘图标关联。
tray.setToolTip(toolTip)
-
toolTip
String
设置此托盘图标的悬停文本。
tray.setTitle(title)
macOS
-
title
String
在状态栏中将标题显示在托盘图标的旁边。
tray.setHighlightMode(mode)
macOS
-
mode
字符串 - 使用以下值之一突出显示模式:-
selection
- 单击时突出显示托盘图标,同时打开其上下文菜单。这是默认设置。 -
always
- 始终突出显示托盘图标。 -
never
- 永不突出显示托盘图标。
-
设置何时托盘的图标背景突出显示(蓝色)。
注意:您可以使用highlightMode
一个BrowserWindow
由之间切换'never'
和'always'
模式的可视窗口更改时。
const {BrowserWindow, Tray} = require('electron')
const win = new BrowserWindow({width: 800, height: 600})
const tray = new Tray('/path/to/my/icon')
tray.on('click', () => {
win.isVisible() ? win.hide() : win.show()
})
win.on('show', () => {
tray.setHighlightMode('always')
})
win.on('hide', () => {
tray.setHighlightMode('never')
})
tray.displayBalloon(options)
Windows
-
options
目的-
icon
(NativeImage | String) - (可选) -
title
字符串 - (可选) -
content
字符串 - (可选)
-
显示托盘 tray balloon。
tray.popUpContextMenu([menu, position])
macOS Windows
-
menu
菜单(可选) -
position
点(可选) - 弹出位置。
弹出托盘图标的上下文菜单。当menu
通过时,menu
将显示而不是托盘图标的上下文菜单。
在position
仅在Windows上使用,它在默认情况下是(0,0)。
tray.setContextMenu(menu)
-
menu
菜单
设置此图标的上下文菜单。
tray.getBounds()
macOS Windows
返回 Rectangle
在bounds
这个托盘图标为Object
。
tray.isDestroyed()
返回Boolean
- 托盘图标是否被销毁。
Tray | ||
---|---|---|
Tray | 详细 |
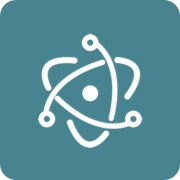
Electron 是一个使用 JavaScript, HTML 和 CSS 等 Web 技术创建原生程序的框架,它负责比较难搞的部分,你只需把精力放在你的应用的核心上即可。
主页 | https://electron.atom.io/ |
源码 | https://github.com/electron/electron |
发布版本 | 1.7.9 |