Electron参考手册
指南 | Guides
快速开始 | Quick Start
Electron使您可以通过为运行时提供丰富的本地(操作系统)API来使用纯JavaScript创建桌面应用程序。您可以将其看作是专注于桌面应用程序而不是Web服务器的Node.js运行时的变体。
这并不意味着Electron是图形用户界面(GUI)库的JavaScript绑定。相反,Electron使用网页作为其GUI,因此您可以将其视为由JavaScript控制的最小Chromium浏览器。
主流程
在电子,运行过程中package.json
的main
脚本被称为主过程。在主进程中运行的脚本可以通过创建网页来显示GUI。
渲染器进程
由于Electron使用Chromium来显示网页,因此Chromium的多进程架构也被使用。Electron中的每个网页都在其自己的进程中运行,这称为渲染器进程。
在普通浏览器中,网页通常运行在沙盒环境中,不允许访问本地资源。但是,电子用户有权在网页中使用Node.js API,从而实现较低级别的操作系统交互。
主流程和渲染器流程的区别
主进程管理所有网页及其相应的呈现程序进程。每个呈现程序进程都是孤立的,只关心在其中运行的网页。
主进程管理所有网页及其相应的渲染器进程。每个渲染器进程都是孤立的,只关心在其中运行的网页。
在网页中,不允许调用与本地GUI相关的API,因为管理网页中的本地GUI资源非常危险,并且很容易泄漏资源。如果要在网页中执行GUI操作,则网页的呈现器进程必须与主进程通信以请求主进程执行这些操作。
在Electron中,我们有几种方法在主流程和渲染器流程之间进行通信。像ipcRenderer
和ipcMain
用于发送消息的模块,以及用于RPC样式通信远程模块。还有关于如何在网页之间共享数据的FAQ条目。
写下你的第一个电子应用程序
通常,一个Electron应用程序的结构如下所示:
您的应用程序内/
├──package.json
├──main.js
└──index.html
其格式与package.json
Node的模块完全相同,并且该main
字段指定的脚本是应用程序的启动脚本,它将运行主进程。你的例子package.json
可能是这样的:
{
"name" : "your-app",
"version" : "0.1.0",
"main" : "main.js"
}
注意:如果该main
字段不存在package.json
,Electron会尝试加载一个index.js
。
本main.js
应创建窗口和处理系统事件,一个典型的例子是:
const {app,BrowserWindow} = require('electron')
const path = require('path')
const url = require('url')
//保持窗口对象的全局引用,如果你不这样做,窗口将会
//当JavaScript对象被垃圾收集时自动关闭。
让胜利
function createWindow () {
// Create the browser window.
win = new BrowserWindow({width: 800, height: 600})
// and load the index.html of the app.
win.loadURL(url.format({
pathname: path.join(__dirname, 'index.html'),
protocol: 'file:',
slashes: true
}))
// Open the DevTools.
win.webContents.openDevTools()
// Emitted when the window is closed.
win.on('closed', () => {
// Dereference the window object, usually you would store windows
// in an array if your app supports multi windows, this is the time
// when you should delete the corresponding element.
win = null
})
}
// This method will be called when Electron has finished
// initialization and is ready to create browser windows.
// Some APIs can only be used after this event occurs.
app.on('ready', createWindow)
// Quit when all windows are closed.
app.on('window-all-closed', () => {
// On macOS it is common for applications and their menu bar
// to stay active until the user quits explicitly with Cmd + Q
if (process.platform !== 'darwin') {
app.quit()
}
})
app.on('activate', () => {
// On macOS it's common to re-create a window in the app when the
// dock icon is clicked and there are no other windows open.
if (win === null) {
createWindow()
}
})
//在这个文件中,您可以包含应用程序的其他特定主流程
//代码。你也可以把它们放在单独的文件中并在这里要求它们。
最后index.html
是你想要显示的网页:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Hello World!</title>
</head>
<body>
<h1>Hello World!</h1>
We are using node <script>document.write(process.versions.node)</script>,
Chrome <script>document.write(process.versions.chrome)</script>,
and Electron <script>document.write(process.versions.electron)</script>.
</body>
</html>
运行你的应用
一旦你创建了你的初始main.js
,index.html
和package.json
文件,你可能会想尝试在本地运行你的应用来测试它,并确保它按预期工作。
electron
electron
是一个npm
包含Electron预编译版本的模块。
如果你已经在全局安装了它npm
,那么你只需要在应用程序的源代码目录中运行以下代码:
electron .
如果您已经在本地安装了它,然后运行:
macOS / Linux
$ ./node_modules/.bin/electron .
Windows
$ .\node_modules\.bin\electron .
手动下载电子二进制文件
如果您手动下载了Electron,则还可以使用包含的二进制文件直接执行您的应用程序。
macOS
$ ./Electron.app/Contents/MacOS/Electron your-app/
Linux
$ ./electron/electron your-app/
Windows
$ .\electron\electron.exe your-app\
Electron.app
这里是Electron发布包的一部分,你可以从这里下载。
作为发行版运行
编写完应用程序后,您可以按照“应用程序分发指南”创建分发版,然后执行打包的应用程序。
试试这个例子
使用electron/electron-quick-start
存储库克隆并运行本教程中的代码。
注意:运行此操作需要在系统上安装Git和Node.js(其中包含npm)。
# Clone the repository
$ git clone https://github.com/electron/electron-quick-start
# Go into the repository
$ cd electron-quick-start
# Install dependencies
$ npm install
# Run the app
$ npm start
指南 | Guides相关
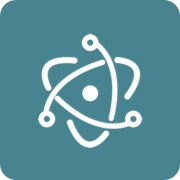
Electron 是一个使用 JavaScript, HTML 和 CSS 等 Web 技术创建原生程序的框架,它负责比较难搞的部分,你只需把精力放在你的应用的核心上即可。
主页 | https://electron.atom.io/ |
源码 | https://github.com/electron/electron |
发布版本 | 1.7.9 |