JavaScript参考手册
声明 | Statements
throw
throw
语句用来抛出一个用户自定义的异常。当前函数的执行将被停止(throw
之后的语句将不会执行),并且控制将被传递到调用堆栈中的第一个catch
块。如果调用者函数中没有catch
块,程序将会终止。
语法
throw expression;
expression
要抛出的表达式。
描述
使用throw
语句来抛出一个异常。当你抛出异常时,expression
指定了异常的内容。下面的每行都抛出了一个异常:
throw 'Error2'; // generates an exception with a string value
throw 42; // generates an exception with the value 42
throw true; // generates an exception with the value true
注意throw
语句同样受到自动分号插入(ASI)机制的控制,在throw
关键字和值之间不允许有行终止符。
示例
抛出一个对象
你可以在抛出异常时指定一个对象。然后可以在catch
块中引用对象的属性。以下示例创建一个类型为UserException
的对象,并在throw
语句中使用它。
function UserException(message) {
this.message = message;
this.name = 'UserException';
}
function getMonthName(mo) {
mo = mo - 1; // Adjust month number for array index (1 = Jan, 12 = Dec)
var months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul',
'Aug', 'Sep', 'Oct', 'Nov', 'Dec'];
if (months[mo] !== undefined) {
return months[mo];
} else {
throw new UserException('InvalidMonthNo');
}
}
try {
// statements to try
var myMonth = 15; // 15 is out of bound to raise the exception
var monthName = getMonthName(myMonth);
} catch (e) {
monthName = 'unknown';
console.log(e.message, e.name); // pass exception object to err handler
}
另一个抛出异常对象的示例
下面的示例中测试一个字符串是否是美国邮政编码。如果邮政编码是无效的,那么throw
语句将会抛出一个类型为ZipCodeFormatException
的异常对象实例。
/*
* Creates a ZipCode object.
*
* Accepted formats for a zip code are:
* 12345
* 12345-6789
* 123456789
* 12345 6789
*
* If the argument passed to the ZipCode constructor does not
* conform to one of these patterns, an exception is thrown.
*/
function ZipCode(zip) {
zip = new String(zip);
pattern = /[0-9]{5}([- ]?[0-9]{4})?/;
if (pattern.test(zip)) {
// zip code value will be the first match in the string
this.value = zip.match(pattern)[0];
this.valueOf = function() {
return this.value
};
this.toString = function() {
return String(this.value)
};
} else {
throw new ZipCodeFormatException(zip);
}
}
function ZipCodeFormatException(value) {
this.value = value;
this.message = 'does not conform to the expected format for a zip code';
this.toString = function() {
return this.value + this.message;
};
}
/*
* This could be in a script that validates address data
* for US addresses.
*/
const ZIPCODE_INVALID = -1;
const ZIPCODE_UNKNOWN_ERROR = -2;
function verifyZipCode(z) {
try {
z = new ZipCode(z);
} catch (e) {
if (e instanceof ZipCodeFormatException) {
return ZIPCODE_INVALID;
} else {
return ZIPCODE_UNKNOWN_ERROR;
}
}
return z;
}
a = verifyZipCode(95060); // returns 95060
b = verifyZipCode(9560); // returns -1
c = verifyZipCode('a'); // returns -1
d = verifyZipCode('95060'); // returns 95060
e = verifyZipCode('95060 1234'); // returns 95060 1234
重新抛出异常
你可以使用throw
来抛出异常。下面的例子捕捉了一个异常值为数字的异常,并在其值大于50后重新抛出异常。重新抛出的异常传播到闭包函数或顶层,以便用户看到它。
try {
throw n; // throws an exception with a numeric value
} catch (e) {
if (e <= 50) {
// statements to handle exceptions 1-50
} else {
// cannot handle this exception, so rethrow
throw e;
}
}
规范
Specification |
Status |
Comment |
---|---|---|
ECMAScript 3rd Edition (ECMA-262) |
Standard |
Initial definition. Implemented in JavaScript 1.4 |
ECMAScript 5.1 (ECMA-262)The definition of 'throw statement' in that specification. |
Standard |
|
ECMAScript 2015 (6th Edition, ECMA-262)The definition of 'throw statement' in that specification. |
Standard |
|
ECMAScript Latest Draft (ECMA-262)The definition of 'throw statement' in that specification. |
Living Standard |
|
浏览器兼容性
Feature |
Chrome |
Edge |
Firefox (Gecko) |
Internet Explorer |
Opera |
Safari |
---|---|---|---|---|---|---|
Basic support |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
Feature |
Android |
Chrome for Android |
Edge |
Firefox Mobile (Gecko) |
IE Mobile |
Opera Mobile |
Safari Mobile |
---|---|---|---|---|---|---|---|
Basic support |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
声明 | Statements相关
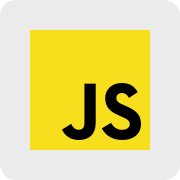
JavaScript 是一种高级编程语言,通过解释执行,是一门动态类型,面向对象(基于原型)的解释型语言。它已经由ECMA(欧洲电脑制造商协会)通过 ECMAScript 实现语言的标准化。它被世界上的绝大多数网站所使用,也被世界主流浏览器( Chrome、IE、FireFox、Safari、Opera )支持。JavaScript 是一门基于原型、函数先行的语言,是一门多范式的语言,它支持面向对象编程,命令式编程,以及函数式编程。它提供语法来操控文本、数组、日期以及正则表达式等,不支持 I/O,比如网络