JavaScript参考手册
声明 | Statements
if...else
当指定条件为真,if 语句会执行一段语句。如果条件为假,则执行另一段语句。
语法
if (condition)
statement1
[else
statement2]
condition
值为真或假的表达式statement1
当condition
为真时执行的语句。可为任意语句,包括更深层的内部if
语句。要执行多条语句,使用块语句({ ... })将这些语句分组;若不想执行语句,则使用空语句。 statement2
如果condition
为假且else
从句存在时执行的语句。可为任意语句,包括块语句和嵌套的if
语句。
描述
多层if...else
语句可使用else if
从句。注意:在 Javascript 中没有 elseif
(一个单词)关键字。
if (condition1)
statement1
else if (condition2)
statement2
else if (condition3)
statement3
...
else
statementN
要看看它如何工作,可以调整下嵌套的缩进:
if (condition1)
statement1
else
if (condition2)
statement2
else
if (condition3)
...
要在一个从句中执行多条语句,可使用语句块({ ... }
)。通常情况下,一直使用语句块是个好习惯,特别是在涉及嵌套if
语句的代码中:
if (condition) {
statements1
} else {
statements2
}
不要将原始布尔值的true
和false
与Boolean对象的真或假混淆。任何一个值,只要它不是 undefined
、null
、 0
、NaN
或空字符串(""
),那么无论是任何对象,即使是值为假的Boolean对象,在条件语句中都为真。例如:
var b = new Boolean(false);
if (b) // this condition is truthy
示例
使用if...else
if (cipher_char === from_char) {
result = result + to_char;
x++;
} else {
result = result + clear_char;
}
使用else if
注意,Javascript中没有elseif
语句。但可以使用else
和if
中间有空格的语句:
if (x > 5) {
/* do the right thing */
} else if (x > 50) {
/* do the right thing */
} else {
/* do the right thing */
}
条件表达式中的赋值运算
建议不要在条件表达式中单纯的使用赋值运算,因为粗看下赋值运算的代码很容易让人误认为是等性比较。比如,不要使用下面示例的代码:
if (x = y) {
/* do the right thing */
}
如果你需要在条件表达式中使用赋值运算,用圆括号包裹赋值运算。例如:
if ((x = y)) {
/* do the right thing */
}
规范
Specification |
Status |
Comment |
---|---|---|
ECMAScript Latest Draft (ECMA-262)The definition of 'if statement' in that specification. |
Draft |
|
ECMAScript 2015 (6th Edition, ECMA-262)The definition of 'if statement' in that specification. |
Standard |
|
ECMAScript 5.1 (ECMA-262)The definition of 'if statement' in that specification. |
Standard |
|
ECMAScript 3rd Edition (ECMA-262)The definition of 'if statement' in that specification. |
Standard |
|
ECMAScript 1st Edition (ECMA-262)The definition of 'if statement' in that specification. |
Standard |
Initial definition |
浏览器兼容性
Feature |
Chrome |
Edge |
Firefox (Gecko) |
Internet Explorer |
Opera |
Safari |
---|---|---|---|---|---|---|
Basic support |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
Feature |
Android |
Chrome for Android |
Edge |
Firefox Mobile (Gecko) |
IE Mobile |
Opera Mobile |
Safari Mobile |
---|---|---|---|---|---|---|---|
Basic support |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
声明 | Statements相关
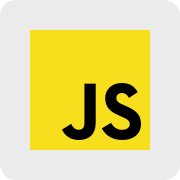
JavaScript 是一种高级编程语言,通过解释执行,是一门动态类型,面向对象(基于原型)的解释型语言。它已经由ECMA(欧洲电脑制造商协会)通过 ECMAScript 实现语言的标准化。它被世界上的绝大多数网站所使用,也被世界主流浏览器( Chrome、IE、FireFox、Safari、Opera )支持。JavaScript 是一门基于原型、函数先行的语言,是一门多范式的语言,它支持面向对象编程,命令式编程,以及函数式编程。它提供语法来操控文本、数组、日期以及正则表达式等,不支持 I/O,比如网络