JavaScript参考手册
声明 | Statements
label
标记语句可以和break
或continue
语句一起使用。标记就是在一条语句前面加个可以引用的标识符。
标记的循环或块非常罕见。通常可以使用函数调用而不是循环跳转。
语法
label :
statement
label
任何不是保留关键字的 JavaScript 标识符。statement
语句。break
可用于任何标记语句,而 continue
可用于循环标记语句。
描述
可使用一个标签来唯一标记一个循环,然后使用 break
或 continue
语句来指示程序是否中断循环或继续执行。
需要注意的是 JavaScript没有goto
语句,标记只能和 break
或 continue
一起使用。
在严格模式中,你不能使用“let
”作为标签名称。它会抛出一个SyntaxError
(let是一个保留的标识符)。
示例
for
循环中使用带标记 continue
var i, j;
loop1:
for (i = 0; i < 3; i++) { //The first for statement is labeled "loop1"
loop2:
for (j = 0; j < 3; j++) { //The second for statement is labeled "loop2"
if (i === 1 && j === 1) {
continue loop1;
}
console.log('i = ' + i + ', j = ' + j);
}
}
// Output is:
// "i = 0, j = 0"
// "i = 0, j = 1"
// "i = 0, j = 2"
// "i = 1, j = 0"
// "i = 2, j = 0"
// "i = 2, j = 1"
// "i = 2, j = 2"
// Notice how it skips both "i = 1, j = 1" and "i = 1, j = 2"
使用带标记 continue
语句
给定一组数据和一组测试,下面的例子统计通过测试的数据。
var itemsPassed = 0;
var i, j;
top:
for (i = 0; i < items.length; i++) {
for (j = 0; j < tests.length; j++) {
if (!tests[j].pass(items[i])) {
continue top;
}
}
itemsPassed++;
}
for
循环中使用带标记的break
var i, j;
loop1:
for (i = 0; i < 3; i++) { //The first for statement is labeled "loop1"
loop2:
for (j = 0; j < 3; j++) { //The second for statement is labeled "loop2"
if (i === 1 && j === 1) {
break loop1;
}
console.log('i = ' + i + ', j = ' + j);
}
}
// Output is:
// "i = 0, j = 0"
// "i = 0, j = 1"
// "i = 0, j = 2"
// "i = 1, j = 0"
// Notice the difference with the previous continue example
使用带标记 break
语句
给定一组数据和一组测试,下面的例子判断是否所有的数据均通过了测试。
var allPass = true;
var i, j;
top:
for (i = 0; items.length; i++)
for (j = 0; j < tests.length; i++)
if (!tests[j].pass(items[i])) {
allPass = false;
break top;
}
在标记块中使用 break
你可以在块中使用记,但只有break
语句可以使用非循环标记。
foo: {
console.log('face');
break foo;
console.log('this will not be executed');
}
console.log('swap');
// this will log:
// "face"
// "swap
标记函数声明
从ECMAScript 2015开始,标准的函数声明现在对规范的Web兼容性附件中的非严格代码进行了标准化。
L: function F() {}
在严格模式中,这会抛出SyntaxError
:
'use strict';
L: function F() {}
// SyntaxError: functions cannot be labelled
生成器函数既不能在严格模式中标记,也不能在非严格模式中标记:
L: function* F() {}
// SyntaxError: generator functions cannot be labelled
规范
Specification |
Status |
Comment |
---|---|---|
ECMAScript 3rd Edition (ECMA-262) |
Standard |
Initial definition. Implemented in JavaScript 1.2 |
ECMAScript 5.1 (ECMA-262)The definition of 'Labelled statement' in that specification. |
Standard |
|
ECMAScript 2015 (6th Edition, ECMA-262)The definition of 'Labelled statement' in that specification. |
Standard |
|
ECMAScript Latest Draft (ECMA-262)The definition of 'Labelled statement' in that specification. |
Draft |
|
浏览器兼容性
Feature |
Chrome |
Edge |
Firefox (Gecko) |
Internet Explorer |
Opera |
Safari |
---|---|---|---|---|---|---|
Basic support |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
Feature |
Android |
Chrome for Android |
Edge |
Firefox Mobile (Gecko) |
IE Mobile |
Opera Mobile |
Safari Mobile |
---|---|---|---|---|---|---|---|
Basic support |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
声明 | Statements相关
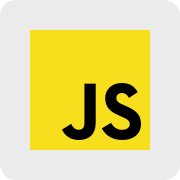
JavaScript 是一种高级编程语言,通过解释执行,是一门动态类型,面向对象(基于原型)的解释型语言。它已经由ECMA(欧洲电脑制造商协会)通过 ECMAScript 实现语言的标准化。它被世界上的绝大多数网站所使用,也被世界主流浏览器( Chrome、IE、FireFox、Safari、Opera )支持。JavaScript 是一门基于原型、函数先行的语言,是一门多范式的语言,它支持面向对象编程,命令式编程,以及函数式编程。它提供语法来操控文本、数组、日期以及正则表达式等,不支持 I/O,比如网络