JavaScript参考手册
对象 | Object
Object.prototype
Object.prototype
属性表示Object
的原型对象。
| Object.prototype
属性的属性特性 |
|:----|
| Writable | no |
| Enumerable | no |
| Configurable | no |
描述
几乎所有的 JavaScript 对象都是Object
的实例;一个典型的对象继承了Object.prototype
的属性(包括方法),尽管这些属性可能被遮蔽(也被称为覆盖)。然而,一个Object
可能是故意创建的,这是不确定的(例如通过Object.create(null)
),或者它可能被改变,所以这不再是准确的(例如Object.setPrototypeOf
)。
Object
原型的改变会传播到所有对象上,除非这些属性和方法被其他对原型链更里层的改动所覆盖。这提供了一个非常强大的,但有潜在危险的机制来覆盖或扩展对象行为。
属性
Object.prototype.constructor
特定的函数,用于创建一个对象的原型。
Object.prototype.__proto__
指向当对象被实例化的时候,用作原型的对象。
Object.prototype.__noSuchMethod__
当未定义的对象成员被调用作方法的时候,允许定义并执行的函数。Object.prototype.__count__用于直接返回用户定义的对象中可数的属性的数量。已被废除。
Object.prototype.__parent__用于指向对象的内容。已被废除。
方法
Object.prototype.__defineGetter__()
关联一个函数到一个属性。访问该函数时,执行该函数并返回其返回值。
Object.prototype.__defineSetter__()
关联一个函数到一个属性。设置该函数时,执行该修改属性的函数。
Object.prototype.__lookupGetter__()
返回使用__defineGetter__
定义的方法函数 。
Object.prototype.__lookupSetter__()
返回使用__defineSetter__
定义的方法函数。
Object.prototype.hasOwnProperty()
返回一个布尔值 ,表示某个对象是否含有指定的属性,而且此属性非原型链继承的。
Object.prototype.isPrototypeOf()
返回一个布尔值,表示指定的对象是否在本对象的原型链中。
Object.prototype.propertyIsEnumerable()
判断指定属性是否可枚举,内部属性设置参见ECMAScript [[Enumerable]] attribute。
Object.prototype.toSource()
返回字符串表示此对象的源代码形式,可以使用此字符串生成一个新的相同的对象。
Object.prototype.toLocaleString()
直接调用toString()
方法。Object.prototype.toString()
返回对象的字符串表示。
Object.prototype.unwatch()
移除对象某个属性的监听。
Object.prototype.valueOf()
返回指定对象的原始值。
Object.prototype.watch()
给对象的某个属性增加监听。
Object.prototype.eval()
在指定对象为上下文情况下执行javascript字符串代码,已经废弃。
示例
当改变现有的 Object.prototype method(方法)的行为时,考虑在现有逻辑之前或之后通过封装你的扩展来注入代码。例如,此(未测试的)代码将在内置逻辑或其他人的扩展执行之前 pre-conditionally(预条件地)执行自定义逻辑。
当一个函数被调用时,调用的参数被保留在类似数组 "变量" 的参数中。例如, 在调用 "myFn (a、b、c)"时, 在myFn 的主体内的参数将包含 3个类似数组的元素对应于 (a、b、c)。 使用钩子修改原型时,只需通过调用该函数的 apply (),将 this 与参数 (调用状态) 传递给当前行为。这种模式可以用于任何原型,如 Node.prototype、 Function.prototype 等.
var current = Object.prototype.valueOf;
// Since my property "-prop-value" is cross-cutting and isn't always
// on the same prototype chain, I want to modify Object.prototype:
Object.prototype.valueOf = function() {
if (this.hasOwnProperty('-prop-value')) {
return this['-prop-value'];
} else {
// It doesn't look like one of my objects, so let's fall back on
// the default behavior by reproducing the current behavior as best we can.
// The apply behaves like "super" in some other languages.
// Even though valueOf() doesn't take arguments, some other hook may.
return current.apply(this, arguments);
}
}
Since JavaScript doesn't exactly have sub-class objects, prototype is a useful workaround to make a “base class” object of certain functions that act as objects. For example:
var Person = function(name) {
this.name = name;
this.canTalk = true;
};
Person.prototype.greet = function() {
if (this.canTalk) {
console.log('Hi, I am ' + this.name);
}
};
var Employee = function(name, title) {
Person.call(this, name);
this.title = title;
};
Employee.prototype = Object.create(Person.prototype);
Employee.prototype.constructor = Employee;
Employee.prototype.greet = function() {
if (this.canTalk) {
console.log('Hi, I am ' + this.name + ', the ' + this.title);
}
};
var Customer = function(name) {
Person.call(this, name);
};
Customer.prototype = Object.create(Person.prototype);
Customer.prototype.constructor = Customer;
var Mime = function(name) {
Person.call(this, name);
this.canTalk = false;
};
Mime.prototype = Object.create(Person.prototype);
Mime.prototype.constructor = Mime;
var bob = new Employee('Bob', 'Builder');
var joe = new Customer('Joe');
var rg = new Employee('Red Green', 'Handyman');
var mike = new Customer('Mike');
var mime = new Mime('Mime');
bob.greet();
// Hi, I am Bob, the Builder
joe.greet();
// Hi, I am Joe
rg.greet();
// Hi, I am Red Green, the Handyman
mike.greet();
// Hi, I am Mike
mime.greet();
规范
Specification |
Status |
Comment |
---|---|---|
ECMAScript 1st Edition (ECMA-262) |
Standard |
Initial definition. Implemented in JavaScript 1.0. |
ECMAScript 5.1 (ECMA-262)The definition of 'Object.prototype' in that specification. |
Standard |
|
ECMAScript 2015 (6th Edition, ECMA-262)The definition of 'Object.prototype' in that specification. |
Standard |
|
ECMAScript Latest Draft (ECMA-262)The definition of 'Object.prototype' in that specification. |
Living Standard |
|
浏览器兼容
Feature |
Chrome |
Edge |
Firefox |
Internet Explorer |
Opera |
Safari |
---|---|---|---|---|---|---|
Basic Support |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
Feature |
Android |
Chrome for Android |
Edge mobile |
Firefox for Android |
IE mobile |
Opera Android |
iOS Safari |
---|---|---|---|---|---|---|---|
Basic Support |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
对象 | Object相关
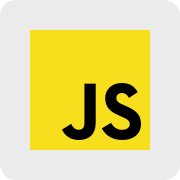
JavaScript 是一种高级编程语言,通过解释执行,是一门动态类型,面向对象(基于原型)的解释型语言。它已经由ECMA(欧洲电脑制造商协会)通过 ECMAScript 实现语言的标准化。它被世界上的绝大多数网站所使用,也被世界主流浏览器( Chrome、IE、FireFox、Safari、Opera )支持。JavaScript 是一门基于原型、函数先行的语言,是一门多范式的语言,它支持面向对象编程,命令式编程,以及函数式编程。它提供语法来操控文本、数组、日期以及正则表达式等,不支持 I/O,比如网络