JavaScript参考手册
对象 | Object
Object.getOwnPropertyDescriptor
Object.getOwnPropertyDescriptor()
方法返回指定对象上一个自有属性对应的属性描述符。(自有属性指的是直接赋予该对象的属性,不需要从原型链上进行查找的属性)
语法
Object.getOwnPropertyDescriptor(obj, prop)
参数
obj
需要查找的目标对象
prop
目标对象内属性名称(String类型)
返回值
如果指定的属性存在于对象上,则返回其属性描述符对象(property descriptor),否则返回 undefined
。
描述
该方法允许对一个属性的描述进行检索。在 Javascript 中, 属性 由一个字符串类型的“名字”(name)和一个“属性描述符”(property descriptor)对象构成。更多关于属性描述符类型以及他们属性的信息可以查看:Object.defineProperty
.
一个属性描述符是一个记录,由下面属性当中的某些组成的:
value
该属性的值(仅针对数据属性描述符有效)
writable当且仅当属性的值可以被改变时为true。(仅针对数据属性描述有效)
get
获取该属性的访问器函数(getter)。如果没有访问器, 该值为undefined。(仅针对包含访问器或设置器的属性描述有效)
set
获取该属性的设置器函数(setter)。 如果没有设置器, 该值为undefined。(仅针对包含访问器或设置器的属性描述有效)
configurable当且仅当指定对象的属性描述可以被改变或者属性可被删除时,为true。
enumerable
当且仅当指定对象的属性可以被枚举出时,为 true
。
示例
var o, d;
o = { get foo() { return 17; } };
d = Object.getOwnPropertyDescriptor(o, 'foo');
// d is {
// configurable: true,
// enumerable: true,
// get: /*the getter function*/,
// set: undefined
// }
o = { bar: 42 };
d = Object.getOwnPropertyDescriptor(o, 'bar');
// d is {
// configurable: true,
// enumerable: true,
// value: 42,
// writable: true
// }
o = {};
Object.defineProperty(o, 'baz', {
value: 8675309,
writable: false,
enumerable: false
});
d = Object.getOwnPropertyDescriptor(o, 'baz');
// d is {
// value: 8675309,
// writable: false,
// enumerable: false,
// configurable: false
// }
注意事项
在 ES5 中,如果该方法的第一个参数不是对象(而是原始类型),那么就会产生出现TypeError
。而在 ES2015,第一个的参数不是对象的话就会被强制转换为对象。
Object.getOwnPropertyDescriptor('foo', 0);
// TypeError: "foo" is not an object // ES5 code
Object.getOwnPropertyDescriptor('foo', 0);
// Object returned by ES2015 code: {
// configurable: false,
// enumerable: true,
// value: "f",
// writable: false
// }
规范
Specification |
Status |
Comment |
---|---|---|
ECMAScript 5.1 (ECMA-262)The definition of 'Object.getOwnPropertyDescriptor' in that specification. |
Standard |
Initial definition. Implemented in JavaScript 1.8.5. |
ECMAScript 2015 (6th Edition, ECMA-262)The definition of 'Object.getOwnPropertyDescriptor' in that specification. |
Standard |
|
ECMAScript Latest Draft (ECMA-262)The definition of 'Object.getOwnPropertyDescriptor' in that specification. |
Living Standard |
|
浏览器兼容性
Feature |
Chrome |
Edge |
Firefox |
Internet Explorer |
Opera |
Safari |
---|---|---|---|---|---|---|
Basic Support |
5 |
(Yes) |
4 |
8 |
12 |
5 |
Feature |
Android |
Chrome for Android |
Edge mobile |
Firefox for Android |
IE mobile |
Opera Android |
iOS Safari |
---|---|---|---|---|---|---|---|
Basic Support |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
对象 | Object相关
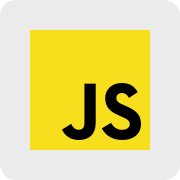
JavaScript 是一种高级编程语言,通过解释执行,是一门动态类型,面向对象(基于原型)的解释型语言。它已经由ECMA(欧洲电脑制造商协会)通过 ECMAScript 实现语言的标准化。它被世界上的绝大多数网站所使用,也被世界主流浏览器( Chrome、IE、FireFox、Safari、Opera )支持。JavaScript 是一门基于原型、函数先行的语言,是一门多范式的语言,它支持面向对象编程,命令式编程,以及函数式编程。它提供语法来操控文本、数组、日期以及正则表达式等,不支持 I/O,比如网络