JavaScript参考手册
对象 | Object
Object.getOwnPropertyNames
Object.getOwnPropertyNames()
方法返回一个由指定对象的所有自身属性的属性名(包括不可枚举属性但不包括Symbol值作为名称的属性)组成的数组。
语法
Object.getOwnPropertyNames(obj)
参数
obj
一个对象,其自身的可枚举和不可枚举属性的名称被返回。
返回值
在给定对象上找到的属性对应的字符串数组。
描述
Object.getOwnPropertyNames()
返回一个数组,该数组对元素是obj
自身拥有的枚举或不可枚举属性名称字符串。 数组中枚举属性的顺序与通过 for...in
循环(或Object.keys
)迭代该对象属性时一致。数组中不可枚举属性的顺序未定义。
示例
使用 Object.getOwnPropertyNames()
var arr = ['a', 'b', 'c'];
console.log(Object.getOwnPropertyNames(arr).sort());
// logs ["0", "1", "2", "length"]
// Array-like object
var obj = { 0: 'a', 1: 'b', 2: 'c' };
console.log(Object.getOwnPropertyNames(obj).sort());
// logs ["0", "1", "2"]
// Logging property names and values using Array.forEach
Object.getOwnPropertyNames(obj).forEach(
function (val, idx, array) {
console.log(val + ' -> ' + obj[val]);
}
);
// logs
// 0 -> a
// 1 -> b
// 2 -> c
// non-enumerable property
var my_obj = Object.create({}, {
getFoo: {
value: function() { return this.foo; },
enumerable: false
}
});
my_obj.foo = 1;
console.log(Object.getOwnPropertyNames(my_obj).sort());
// logs ["foo", "getFoo"]
如果你只要获取到可枚举属性,查看Object.keys
或用for...in
循环(还会获取到原型链上的可枚举属性,不过可以使用hasOwnProperty()
方法过滤掉)。
下面的例子演示了该方法不会获取到原型链上的属性:
function ParentClass() {}
ParentClass.prototype.inheritedMethod = function() {};
function ChildClass() {
this.prop = 5;
this.method = function() {};
}
ChildClass.prototype = new ParentClass;
ChildClass.prototype.prototypeMethod = function() {};
console.log(
Object.getOwnPropertyNames(
new ChildClass() // ["prop", "method"]
)
);
只获取不可枚举的属性
下面的例子使用了Array.prototype.filter()
方法,从所有的属性名数组(使用Object.getOwnPropertyNames()
方法获得)中去除可枚举的属性(使用Object.keys()
方法获得),剩余的属性便是不可枚举的属性了:
var target = myObject;
var enum_and_nonenum = Object.getOwnPropertyNames(target);
var enum_only = Object.keys(target);
var nonenum_only = enum_and_nonenum.filter(function(key) {
var indexInEnum = enum_only.indexOf(key);
if (indexInEnum == -1) {
// Not found in enum_only keys,
// meaning that the key is non-enumerable,
// so return true so we keep this in the filter
return true;
} else {
return false;
}
});
console.log(nonenum_only);
提示
在 ES5 中,如果参数不是一个原始对象类型,将抛出一个 TypeError
异常。在 ES2015 中,非对象参数被强制转换为对象 。
Object.getOwnPropertyNames('foo');
// TypeError: "foo" is not an object (ES5 code)
Object.getOwnPropertyNames('foo');
// ["0", "1", "2", "length"] (ES2015 code)
规范
Specification |
Status |
Comment |
---|---|---|
ECMAScript 5.1 (ECMA-262)The definition of 'Object.getOwnPropertyNames' in that specification. |
Standard |
Initial definition. Implemented in JavaScript 1.8.5. |
ECMAScript 2015 (6th Edition, ECMA-262)The definition of 'Object.getOwnPropertyNames' in that specification. |
Standard |
|
ECMAScript Latest Draft (ECMA-262)The definition of 'Object.getOwnPropertyNames' in that specification. |
Living Standard |
|
浏览器兼容性
Feature |
Chrome |
Edge |
Firefox |
Internet Explorer |
Opera |
Safari |
---|---|---|---|---|---|---|
Basic Support |
5 |
(Yes) |
4 |
9 |
12 |
5 |
Feature |
Android |
Chrome for Android |
Edge mobile |
Firefox for Android |
IE mobile |
Opera Android |
iOS Safari |
---|---|---|---|---|---|---|---|
Basic Support |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
对象 | Object相关
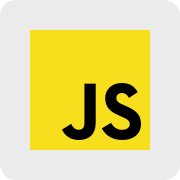
JavaScript 是一种高级编程语言,通过解释执行,是一门动态类型,面向对象(基于原型)的解释型语言。它已经由ECMA(欧洲电脑制造商协会)通过 ECMAScript 实现语言的标准化。它被世界上的绝大多数网站所使用,也被世界主流浏览器( Chrome、IE、FireFox、Safari、Opera )支持。JavaScript 是一门基于原型、函数先行的语言,是一门多范式的语言,它支持面向对象编程,命令式编程,以及函数式编程。它提供语法来操控文本、数组、日期以及正则表达式等,不支持 I/O,比如网络