Python参考手册
数据类型 | Data Types
sched
源代码: Lib / sched.py
该sched
模块定义了一个实现通用事件调度器的类:
class sched.scheduler(timefunc, delayfunc)
在scheduler
类定义一个通用的接口,调度事件。它需要两个功能来实际处理“外部世界” - timefunc应该可以在没有参数的情况下进行调用,并返回一个数字(任何单位的“时间”)。该delayfunc功能应该是可调用的一个参数,与输出兼容timefunc,并应延迟许多时间单位。在每个事件运行后,delayfunc也将被参数调用,0
以允许其他线程有机会在多线程应用程序中运行。
例:
>>> import sched, time
>>> s = sched.scheduler(time.time, time.sleep)
>>> def print_time(): print "From print_time", time.time()
...
>>> def print_some_times():
... print time.time()
... s.enter(5, 1, print_time, ())
... s.enter(10, 1, print_time, ())
... s.run()
... print time.time()
...
>>> print_some_times()
930343690.257
From print_time 930343695.274
From print_time 930343700.273
930343700.276
在多线程环境中,scheduler
该类在线程安全方面存在限制,无法在正在运行的调度程序中当前未决的新任务之前插入新任务,并且阻止主线程直到事件队列为空。相反,首选方法是使用threading.Timer
该类。
例:
>>> import time
>>> from threading import Timer
>>> def print_time():
... print "From print_time", time.time()
...
>>> def print_some_times():
... print time.time()
... Timer(5, print_time, ()).start()
... Timer(10, print_time, ()).start()
... time.sleep(11) # sleep while time-delay events execute
... print time.time()
...
>>> print_some_times()
930343690.257
From print_time 930343695.274
From print_time 930343700.273
930343701.301
1.调度程序对象
scheduler
实例具有以下方法和属性:
scheduler.enterabs(time, priority, action, argument)
安排新的活动。在时间参数应该是一个数字类型与返回值兼容timefunc传递给构造函数。计划在同一时间的活动将按其优先顺序执行。
执行事件意味着执行action(*argument)
。参数必须是一个保存动作参数的序列。
返回值是一个可用于事件后续取消的事件(请参阅参考资料cancel()
)。
scheduler.enter(delay, priority, action, argument)
安排延迟更多时间单位的事件。除了相对时间以外,其他参数,效果和返回值与那些相同enterabs()
。
scheduler.cancel(event)
从队列中删除事件。如果事件不是当前队列中的事件,则此方法将引发一个ValueError
。
scheduler.empty()
如果事件队列为空,则返回true。
scheduler.run()
运行所有计划的事件。该函数将等待(使用delayfunc()
传递给构造函数的函数)进行下一个事件,然后执行此操作,直到没有更多预定事件。
无论是动作还是delayfunc可引发异常。无论哪种情况,调度程序都将保持一致的状态并传播异常。如果通过操作引发异常,则将来的呼叫将不会尝试该事件run()
。
如果一系列事件的运行时间比下一个事件之前的可用时间长,那么调度器将会落后。没有事件会被丢弃; 调用代码负责取消不再相关的事件。
scheduler.queue
只读属性按照它们将运行的顺序返回即将发生的事件列表。每个事件都显示为具有以下字段的命名元组:时间,优先级,动作,参数。
2.6版本中的新功能。
数据类型 | Data Types相关
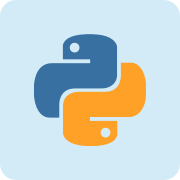
Python 是一种面向对象的解释型计算机程序设计语言,由荷兰人 Guido van Rossum 于1989年发明,第一个公开发行版发行于1991年。 Python 是纯粹的自由软件, 源代码和解释器 CPython 遵循 GPL 协议。Python 语法简洁清晰,特色之一是强制用空白符( white space )作为语句缩进。
主页 | https://www.python.org/ |
源码 | https://github.com/python/cpython |
版本 | 2.7 |
发布版本 | 2.7.13 |