JavaScript参考手册
Operators
super
super关键字用于访问和调用一个对象的父对象上的函数。
super.prop和super[expr]表达式在类和对象字面量任何方法定义中都是有效的。
语法
super([arguments]); // calls the parent constructor.
super.functionOnParent([arguments]);
描述
在构造函数中使用时,super
关键字将单独出现,并且必须在使用this
关键字之前使用。super
关键字也可以用来调用父对象上的函数。
示例
在类中使用super
以下代码片段来自于 classes sample。
class Polygon {
constructor(height, width) {
this.name = 'Polygon';
this.height = height;
this.width = width;
}
sayName() {
console.log('Hi, I am a ', this.name + '.');
}
}
class Square extends Polygon {
constructor(length) {
this.height; // ReferenceError, super needs to be called first!
// Here, it calls the parent class' constructor with lengths
// provided for the Polygon's width and height
super(length, length);
// Note: In derived classes, super() must be called before you
// can use 'this'. Leaving this out will cause a reference error.
this.name = 'Square';
}
get area() {
return this.height * this.width;
}
set area(value) {
this.height = this.width = Math.sqrt(value);
}
}
调用父类上的静态方法
你也可以用 super 调用父类的静态方法。
class Human {
constructor() {}
static ping() {
return 'ping';
}
}
class Computer extends Human {
constructor() {}
static pingpong() {
return super.ping() + ' pong';
}
}
Computer.pingpong(); // 'ping pong'
删除 super 上的属性将抛出异常
你不能使用 delete 操作符 加 super.prop 或者 super[expr] 去删除父类的属性,这样做会抛出 ReferenceError。
class Base {
constructor() {}
foo() {}
}
class Derived extends Base {
constructor() {}
delete() {
delete super.foo;
}
}
new Derived().delete(); // ReferenceError: invalid delete involving 'super'.
Super.prop
不能覆写不可写属性
当使用 Object.defineProperty 定义一个属性为不可写时,super将不能重写这个属性的值。
class X {
constructor() {
Object.defineProperty(this, 'prop', {
configurable: true,
writable: false,
value: 1
});
}
f() {
super.prop = 2;
}
}
var x = new X();
x.f(); // TypeError: "prop" is read-only
console.log(x.prop); // 1
在对象字面量中使用super.prop
Super也可以在object initializer / literal 符号中使用。在下面的例子中,两个对象各定义了一个方法。在第二个对象中, 我们使用super调用了第一个对象中的方法。 当然,这需要我们先利用 Object.setPrototypeOf() 将obj2的原型加到obj1上,然后才能够使用super调用 obj1上的method1。
var obj1 = {
method1() {
console.log('method 1');
}
}
var obj2 = {
method2() {
super.method1();
}
}
Object.setPrototypeOf(obj2, obj1);
obj2.method2(); // logs "method 1"
规范
Specification |
Status |
Comment |
---|---|---|
ECMAScript 2015 (6th Edition, ECMA-262)The definition of 'super' in that specification. |
Standard |
Initial definition. |
ECMAScript Latest Draft (ECMA-262)The definition of 'super' in that specification. |
Living Standard |
|
浏览器兼容性
Feature |
Chrome |
Edge |
Firefox (Gecko) |
Internet Explorer |
Opera |
Safari |
---|---|---|---|---|---|---|
Basic support |
42.0 |
(Yes) |
45 (45) |
? |
? |
? |
Feature |
Android |
Android Webview |
Edge |
Firefox Mobile (Gecko) |
IE Mobile |
Opera Mobile |
Safari Mobile |
Chrome for Android |
---|---|---|---|---|---|---|---|---|
Basic support |
? |
42.0 |
(Yes) |
45.0 (45) |
? |
? |
? |
42.0 |
Operators相关
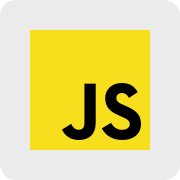
JavaScript 是一种高级编程语言,通过解释执行,是一门动态类型,面向对象(基于原型)的解释型语言。它已经由ECMA(欧洲电脑制造商协会)通过 ECMAScript 实现语言的标准化。它被世界上的绝大多数网站所使用,也被世界主流浏览器( Chrome、IE、FireFox、Safari、Opera )支持。JavaScript 是一门基于原型、函数先行的语言,是一门多范式的语言,它支持面向对象编程,命令式编程,以及函数式编程。它提供语法来操控文本、数组、日期以及正则表达式等,不支持 I/O,比如网络