JavaScript参考手册
Operators
delete
delete
去除对象的属性。
语法
delete expression
其中表达应评估的特性引用,例如:
delete object.property
delete object['property']
参数
object
对象的名称或评估对象的表达式。
property
要删除的属性。
返回值
true
适用于所有情况,除非属性是自己的不可配置属性,在这种情况下,false
将以非严格模式返回。
异常
如果该属性是自己的不可配置属性,则在严格模式下抛出Global_objects/SyntaxError
。
描述
与常见的看法不同,delete
运营商与直接释放内存无关。内存管理通过中断引用间接完成,请参阅内存管理页面以获取更多详细信息。
delete
操作者除去来自物体的给定属性。在成功删除后,它将返回,true,
否则false
将返回。但是,考虑以下情况很重要:
- 如果您试图删除的属性不存在,
delete
将不会有任何效果并将返回true
- 如果对象的原型链中存在同名的属性,那么在删除之后,对象将使用原型链中的属性(换句话说,
delete
只对自己的属性有影响)。 - 任何声明的属性
var
都不能从全局范围或函数的范围中删除。- 因此,
delete
不能删除全局范围内的任何函数(无论这是否是函数定义或函数表达式的一部分)。 - 作为一个对象的一部分的函数(除了全局作用域)可以被删除
delete
。
- 因此,
- 任何属于
let
或const
不能从定义范围内删除的属性。 - 不可配置的属性不能被删除。这包括内置对象一样的性质
Math
,Array
,Object
被非配置有类似的方法创建和属性Object.defineProperty()
。
以下片段给出了一个简单的例子:
var Employee = {
age: 28,
name: 'abc',
designation: 'developer'
}
console.log(delete Employee.name); // returns true
console.log(delete Employee.age); // returns true
// When trying to delete a property that does
// not exist, true is returned
console.log(delete Employee.salary); // returns true
不可配置的属性
当一个属性被标记为不可配置时,delete
不会有任何效果,并且会返回false
。在严格的模式下,这将提高SyntaxError
。
var Employee = {};
Object.defineProperty(Employee, 'name', {configurable: false});
console.log(delete Employee.name); // returns false
var
,let
并const
创建无法用delete
操作符删除的不可配置属性:
var nameOther = 'XYZ';
// We can access this global property using:
Object.getOwnPropertyDescriptor(window, 'nameOther');
// output: Object {value: "XYZ",
// writable: true,
// enumerable: true,
// configurable: false}
// Since "nameOther" is added using with the
// var keyword, it is marked as "non-configurable"
delete nameOther; // return false
在严格的模式下,这会引发一个例外。
严格模式与非严格模式
在严格模式下,如果delete
用于对变量,函数参数或函数名称的直接引用,则会抛出一个SyntaxError
。
任何定义的变量都var
被标记为不可配置。在以下示例中,它salary
是不可配置的,无法删除。在非严格模式下,delete
操作将返回false
。
function Employee() {
delete salary;
var salary;
}
Employee();
让我们来看看相同的代码在严格模式下的行为。false
声明提出了一个答案,而不是返回SyntaxError
。
"use strict";
function Employee() {
delete salary; // SyntaxError
var salary;
}
// Similarly, any direct access to a function
// with delete will raise a SyntaxError
function DemoFunction() {
//some code
}
delete DemoFunction; // SyntaxError
示例
// creates the property adminName on the global scope
adminName = 'xyz';
// creates the property empCount on the global scope
// Since we are using var, this is marked as non-configurable. The same is true of let and const.
var empCount = 43;
EmployeeDetails = {
name: 'xyz',
age: 5,
designation: 'Developer'
};
// adminName is a property of the global scope.
// It can be deleted since it is created without var.
// Therefore, it is configurable.
delete adminName; // returns true
// On the contrary, empCount is not configurable,
// since var was used.
delete empCount; // returns false
// delete can be used to remove properties from objects
delete EmployeeDetails.name; // returns true
// Even when the property does not exists, it returns "true"
delete EmployeeDetails.salary; // returns true
// delete does not affect built-in static properties
delete Math.PI; // returns false
// EmployeeDetails is a property of the global scope.
// Since it defined without "var", it is marked configurable
delete EmployeeDetails; // returns true
function f() {
var z = 44;
// delete doesn't affect local variable names
delete z; // returns false
}
delete
和原型链
在下面的例子中,我们删除了一个对象的属性,而同名的属性在原型链上可用:
function Foo() {
this.bar = 10;
}
Foo.prototype.bar = 42;
var foo = new Foo();
// Returns true, since the own property
// has been deleted on the foo object
delete foo.bar;
// foo.bar is still available, since it
// is available in the prototype chain.
console.log(foo.bar);
// We delete the property on the prototype
delete Foo.prototype.bar;
// logs "undefined" since the property
// is no longer inherited
console.log(foo.bar);
删除数组元素
删除数组元素时,数组长度不受影响。即使删除数组的最后一个元素,也是如此。
当delete
操作符移除数组元素时,该元素不再位于数组中。在下面的例子中,trees[3]
被delete
删除。
var trees = ['redwood', 'bay', 'cedar', 'oak', 'maple'];
delete trees[3];
if (3 in trees) {
// this does not get executed
}
如果你想要一个数组元素存在,但有一个未定义的值,请使用该undefined
值而不是delete
运算符。在下面的例子中,trees[3]
赋值为 undefined,但数组元素仍然存在:
var trees = ['redwood', 'bay', 'cedar', 'oak', 'maple'];
trees[3] = undefined;
if (3 in trees) {
// this gets executed
}
规范
Specification |
Status |
Comment |
---|---|---|
ECMAScript Latest Draft (ECMA-262)The definition of 'The delete Operator' in that specification. |
Living Standard |
|
ECMAScript 2015 (6th Edition, ECMA-262)The definition of 'The delete Operator' in that specification. |
Standard |
|
ECMAScript 5.1 (ECMA-262)The definition of 'The delete Operator' in that specification. |
Standard |
|
ECMAScript 1st Edition (ECMA-262)The definition of 'The delete Operator' in that specification. |
Standard |
Initial definition. Implemented in JavaScript 1.2. |
浏览器兼容性
Feature |
Chrome |
Edge |
Firefox (Gecko) |
Internet Explorer |
Opera |
Safari |
---|---|---|---|---|---|---|
Basic support |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
Temporal dead zone |
? |
? |
36 (36) |
? |
? |
? |
Feature |
Android |
Chrome for Android |
Edge |
Firefox Mobile (Gecko) |
IE Mobile |
Opera Mobile |
Safari Mobile |
---|---|---|---|---|---|---|---|
Basic support |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
(Yes) |
Temporal dead zone |
? |
? |
? |
36.0 (36) |
? |
? |
? |
Operators相关
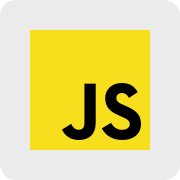
JavaScript 是一种高级编程语言,通过解释执行,是一门动态类型,面向对象(基于原型)的解释型语言。它已经由ECMA(欧洲电脑制造商协会)通过 ECMAScript 实现语言的标准化。它被世界上的绝大多数网站所使用,也被世界主流浏览器( Chrome、IE、FireFox、Safari、Opera )支持。JavaScript 是一门基于原型、函数先行的语言,是一门多范式的语言,它支持面向对象编程,命令式编程,以及函数式编程。它提供语法来操控文本、数组、日期以及正则表达式等,不支持 I/O,比如网络