Electron参考手册
浏览器窗口 | BrowserWindow
BrowserWindow
创建并控制浏览器窗口。
过程:Main
// In the main process.
const {BrowserWindow} = require('electron')
// Or use `remote` from the renderer process.
// const {BrowserWindow} = require('electron').remote
let win = new BrowserWindow({width: 800, height: 600})
win.on('closed', () => {
win = null
})
// Load a remote URL
win.loadURL('https://github.com')
// Or load a local HTML file
win.loadURL(`file://${__dirname}/app/index.html`)
无框窗口
要创建没有镶边的窗口或任意形状的透明窗口,可以使用无框窗口API。
优雅地显示窗口
当直接在窗口中加载页面时,用户可能会逐渐看到页面加载,这对于本机应用程序来说不是一个好的体验。为了使窗口显示没有视觉闪光,对于不同的情况有两种解决方案。
使用ready-to-show
事件
加载页面时,ready-to-show
如果窗口尚未显示,则渲染器进程首次渲染页面时会发出事件。在此事件之后显示窗口将没有可视闪光灯:
const {BrowserWindow} = require('electron')
let win = new BrowserWindow({show: false})
win.once('ready-to-show', () => {
win.show()
})
此事件通常在did-finish-load
事件发生后发出,但对于具有许多远程资源的页面,它可能在did-finish-load
事件发生之前发出。
设置 backgroundColor
对于复杂的应用程序,该ready-to-show
事件可能会发射得太晚,使应用程序感觉缓慢。在这种情况下,建议立即显示窗口,并使用backgroundColor
接近您的应用程序的背景:
const {BrowserWindow} = require('electron')
let win = new BrowserWindow({backgroundColor: '#2e2c29'})
win.loadURL('https://github.com')
请注意,即使对于使用ready-to-show
事件的应用程序,仍然建议设置backgroundColor
为使应用程序感觉更加本地化。
父窗口和子窗口
通过使用parent
选项,您可以创建子窗口:
const {BrowserWindow} = require('electron')
let top = new BrowserWindow()
let child = new BrowserWindow({parent: top})
child.show()
top.show()
该child
窗口将始终显示在top
窗口顶部。
模态窗口
模态窗口是禁用父窗口的子窗口,要创建模态窗口,必须设置两者parent
和modal
选项:
const {BrowserWindow} = require('electron')
let child = new BrowserWindow({parent: top, modal: true, show: false})
child.loadURL('https://github.com')
child.once('ready-to-show', () => {
child.show()
})
页面可见性
在网页浏览权限API的工作原理如下:
- 在所有平台上,可见性状态都会跟踪窗口是否隐藏/最小化。
- 另外,在macOS上,可见性状态还跟踪窗口遮挡状态。如果窗口被另一个窗口遮挡(即完全覆盖),则可见性状态将为
hidden
。在其他平台上,hidden
仅当窗口最小化或显式隐藏时才会显示状态win.hide()
。 - 如果使用a
BrowserWindow
创建ashow: false
,则visible
尽管窗口实际上处于隐藏状态,但仍会显示初始可见性状态。 - 如果
backgroundThrottling
被禁用,visible
即使窗口被最小化,遮挡或隐藏,可见性状态仍会保留。
建议您在可见性状态hidden
为了最小化功耗时暂停昂贵的操作。
平台通知
- 在macOS模态窗口将显示为附加到父窗口的图纸。
- 在macOS上,当父窗口移动时,子窗口将保持与父窗口的相对位置,而在Windows和Linux子窗口上不会移动。
- 在Windows上不支持动态更改父窗口。
- 在Linux上,模式窗口的类型将更改为
dialog
。 - 在Linux上,许多桌面环境不支持隐藏模式窗口。
类:BrowserWindow
创建并控制浏览器窗口。
过程:Main
BrowserWindow
是一个EventEmitter。
它创建一个新的BrowserWindow
具有原生属性的设置options
。
new BrowserWindow([options])
-
options
对象(可选)-
width
整数(可选) - 窗口的宽度(以像素为单位)。默认是800
。 -
height
整数(可选) - 窗口的高度(以像素为单位)。默认是600
。 -
x
整数(可选)(如果使用y,则为必需) - 窗口的左偏移屏幕。默认是将窗口居中。 -
y
整数(可选)(如果使用x,则为必需) - 窗口与屏幕的偏移量。默认是将窗口居中。 -
useContentSize
布尔(可选) -width
和height
将用作网页的大小,这意味着实际窗口的大小将包括窗口框架的大小并略大。默认是false
。 -
center
布尔(可选) - 在屏幕中心显示窗口。 -
minWidth
整数(可选) - 窗口的最小宽度。默认是0
。 -
minHeight
整数(可选) - 窗口的最小高度。默认是0
。 -
maxWidth
整数(可选) - 窗口的最大宽度。默认是没有限制的。 -
maxHeight
整数(可选) - 窗口的最大高度。默认是没有限制的。 -
resizable
布尔(可选) - 窗口是否可调整大小。默认是true
。 -
movable
布尔(可选) - 窗口是否可移动。这在Linux上没有实现。默认是true
。 -
minimizable
布尔(可选) - 窗口是否可以最小化。这在Linux上没有实现。默认是true
。 -
maximizable
布尔(可选) - 窗口是否可以最大化。这在Linux上没有实现。默认是true
。 -
closable
布尔(可选) - 窗口是否可关闭。这在Linux上没有实现。默认是true
。 -
focusable
布尔(可选) - 窗口是否可以聚焦。默认是true
。在Windows上设置focusable: false
也意味着设置skipTaskbar: true
。在Linux设置下focusable: false
,窗口停止与wm进行交互,所以窗口将始终保持在所有工作区的顶部。 -
alwaysOnTop
布尔(可选) - 窗口是否应始终保持在其他窗口之上。默认是false
。 -
fullscreen
布尔(可选) - 窗口是否应以全屏显示。当明确设置为false
全屏按钮时,将在macOS上隐藏或禁用。默认是false
。 -
fullscreenable
布尔(可选) - 窗口是否可以进入全屏模式。在macOS上,最大化/缩放按钮是否应该切换全屏模式或最大化窗口。默认是true
。 -
skipTaskbar
布尔(可选) - 是否在任务栏中显示窗口。默认是false
。 -
kiosk
布尔(可选) - 自助服务终端模式。默认是false
。 -
title
字符串(可选) - 默认窗口标题。默认是"Electron"
。 -
icon
(NativeImage |字符串)(可选) - 窗口图标。在Windows上,建议使用ICO
图标来获得最佳的视觉效果,您也可以将其保留为未定义的,以便使用可执行文件的图标。 -
show
布尔(可选) - 创建时是否显示窗口。默认是true
。 -
frame
布尔(可选) - 指定false
创建一个无框窗口。默认是true
。 -
parent
BrowserWindow(可选) - 指定父窗口。默认是null
。 -
modal
布尔(可选) - 是否是模态窗口。这只适用于窗口是子窗口的情况。默认是false
。 -
acceptFirstMouse
Boolean(可选) - Web视图是否接受同时激活窗口的单个鼠标按下事件。默认是false
。 -
disableAutoHideCursor
布尔(可选) - 是否在键入时隐藏光标。默认是false
。 -
autoHideMenuBar
布尔(可选) - 除非Alt
按下键,否则自动隐藏菜单栏。默认是false
。 -
enableLargerThanScreen
布尔(可选) - 使窗口的调整大小大于屏幕。默认是false
。 -
backgroundColor
字符串(可选) - 窗口的背景颜色为十六进制值,如#66CD00
or#FFF
或#80FFFFFF
(支持alpha)。默认是#FFF
(白色)。 -
hasShadow
布尔(可选) - 窗口是否应该有阴影。这只在macOS上实现。默认是true
。 -
darkTheme
布尔(可选) - 强制使用黑暗主题作为窗口,仅适用于某些GTK + 3桌面环境。默认是false
。 -
transparent
布尔(可选) - 使窗口透明。默认是false
。 -
type
字符串(可选) - 窗口的类型,默认为正常窗口。请参阅下面的更多信息。 -
titleBarStyle
字符串(可选) - 窗口标题栏的样式。默认是default
。可能的值是:-
default
- 导致标准灰色不透明的Mac标题栏。 -
hidden
- 导致隐藏标题栏和全尺寸内容窗口,但标题栏仍然在左上角具有标准窗口控件(“交通信号灯”)。 -
hidden-inset
- 弃用,hiddenInset
改为使用。 -
hiddenInset
- 在隐藏的标题栏中显示交通灯按钮稍微偏离窗口边缘的替代外观。 -
customButtonsOnHover
布尔(可选) - 在macOS无框窗口上绘制自定义关闭,最小化和全屏按钮。这些按钮不会显示,除非在窗口的左上角悬停。这些自定义按钮可防止与标准窗口工具栏按钮发生的鼠标事件相关的问题。注意:此选项目前是实验性的。
-
-
- `fullscreenWindowTitle` Boolean (optional) - Shows the title in the tile bar in full screen mode on macOS for all `titleBarStyle` options. Default is `false`.
- `thickFrame` Boolean (optional) - Use `WS_THICKFRAME` style for frameless windows on Windows, which adds standard window frame. Setting it to `false` will remove window shadow and window animations. Default is `true`.
- `vibrancy` String (optional) - Add a type of vibrancy effect to the window, only on macOS. Can be `appearance-based`, `light`, `dark`, `titlebar`, `selection`, `menu`, `popover`, `sidebar`, `medium-light` or `ultra-dark`.
- `zoomToPageWidth` Boolean (optional) - Controls the behavior on macOS when option-clicking the green stoplight button on the toolbar or by clicking the Window > Zoom menu item. If `true`, the window will grow to the preferred width of the web page when zoomed, `false` will cause it to zoom to the width of the screen. This will also affect the behavior when calling `maximize()` directly. Default is `false`.
- `tabbingIdentifier` String (optional) - Tab group name, allows opening the window as a native tab on macOS 10.12+. Windows with the same tabbing identifier will be grouped together. This also adds a native new tab button to your window’s tab bar and allows your `app` and window to receive the `new-window-for-tab` event.
- `webPreferences` Object (optional) - Settings of web page’s features.
- `devTools` Boolean (optional) - Whether to enable DevTools. If it is set to `false`, can not use `BrowserWindow.webContents.openDevTools()` to open DevTools. Default is `true`.
- `nodeIntegration` Boolean (optional) - Whether node integration is enabled. Default is `true`.
- `nodeIntegrationInWorker` Boolean (optional) - Whether node integration is enabled in web workers. Default is `false`. More about this can be found in [Multithreading](../../tutorial/multithreading/index).
- `preload` String (optional) - Specifies a script that will be loaded before other scripts run in the page. This script will always have access to node APIs no matter whether node integration is turned on or off. The value should be the absolute file path to the script. When node integration is turned off, the preload script can reintroduce Node global symbols back to the global scope. See example [here](../process/index#event-loaded).
- `sandbox` Boolean (optional) - If set, this will sandbox the renderer associated with the window, making it compatible with the Chromium OS-level sandbox and disabling the Node.js engine. This is not the same as the `nodeIntegration` option and the APIs available to the preload script are more limited. Read more about the option [here](../sandbox-option/index). **Note:** This option is currently experimental and may change or be removed in future Electron releases.
- `session` [Session](../session/index#class-session) (optional) - Sets the session used by the page. Instead of passing the Session object directly, you can also choose to use the `partition` option instead, which accepts a partition string. When both `session` and `partition` are provided, `session` will be preferred. Default is the default session.
- `partition` String (optional) - Sets the session used by the page according to the session’s partition string. If `partition` starts with `persist:`, the page will use a persistent session available to all pages in the app with the same `partition`. If there is no `persist:` prefix, the page will use an in-memory session. By assigning the same `partition`, multiple pages can share the same session. Default is the default session.
- `zoomFactor` Number (optional) - The default zoom factor of the page, `3.0` represents `300%`. Default is `1.0`.
- `javascript` Boolean (optional) - Enables JavaScript support. Default is `true`.
- `webSecurity` Boolean (optional) - When `false`, it will disable the same-origin policy (usually using testing websites by people), and set `allowRunningInsecureContent` to `true` if this options has not been set by user. Default is `true`.
- `allowRunningInsecureContent` Boolean (optional) - Allow an https page to run JavaScript, CSS or plugins from http URLs. Default is `false`.
- `images` Boolean (optional) - Enables image support. Default is `true`.
- `textAreasAreResizable` Boolean (optional) - Make TextArea elements resizable. Default is `true`.
- `webgl` Boolean (optional) - Enables WebGL support. Default is `true`.
- `webaudio` Boolean (optional) - Enables WebAudio support. Default is `true`.
- `plugins` Boolean (optional) - Whether plugins should be enabled. Default is `false`.
- `experimentalFeatures` Boolean (optional) - Enables Chromium’s experimental features. Default is `false`.
- `experimentalCanvasFeatures` Boolean (optional) - Enables Chromium’s experimental canvas features. Default is `false`.
- `scrollBounce` Boolean (optional) - Enables scroll bounce (rubber banding) effect on macOS. Default is `false`.
- `blinkFeatures` String (optional) - A list of feature strings separated by `,`, like `CSSVariables,KeyboardEventKey` to enable. The full list of supported feature strings can be found in the [RuntimeEnabledFeatures.json5](https://cs.chromium.org/chromium/src/third_party/WebKit/Source/platform/RuntimeEnabledFeatures.json5?l=62) file.
- `disableBlinkFeatures` String (optional) - A list of feature strings separated by `,`, like `CSSVariables,KeyboardEventKey` to disable. The full list of supported feature strings can be found in the [RuntimeEnabledFeatures.json5](https://cs.chromium.org/chromium/src/third_party/WebKit/Source/platform/RuntimeEnabledFeatures.json5?l=62) file.
- `defaultFontFamily` Object (optional) - Sets the default font for the font-family.
- `standard` String (optional) - Defaults to `Times New Roman`.
- `serif` String (optional) - Defaults to `Times New Roman`.
- `sansSerif` String (optional) - Defaults to `Arial`.
- `monospace` String (optional) - Defaults to `Courier New`.
- `cursive` String (optional) - Defaults to `Script`.
- `fantasy` String (optional) - Defaults to `Impact`.
- `defaultFontSize` Integer (optional) - Defaults to `16`.
- `defaultMonospaceFontSize` Integer (optional) - Defaults to `13`.
- `minimumFontSize` Integer (optional) - Defaults to `0`.
- `defaultEncoding` String (optional) - Defaults to `ISO-8859-1`.
- `backgroundThrottling` Boolean (optional) - Whether to throttle animations and timers when the page becomes background. This also affects the [Page Visibility API][#page-visibility]. Defaults to `true`.
- `offscreen` Boolean (optional) - Whether to enable offscreen rendering for the browser window. Defaults to `false`. See the [offscreen rendering tutorial](../../tutorial/offscreen-rendering/index) for more details.
- `contextIsolation` Boolean (optional) - Whether to run Electron APIs and the specified `preload` script in a separate JavaScript context. Defaults to `false`. The context that the `preload` script runs in will still have full access to the `document` and `window` globals but it will use its own set of JavaScript builtins (`Array`, `Object`, `JSON`, etc.) and will be isolated from any changes made to the global environment by the loaded page. The Electron API will only be available in the `preload` script and not the loaded page. This option should be used when loading potentially untrusted remote content to ensure the loaded content cannot tamper with the `preload` script and any Electron APIs being used. This option uses the same technique used by [Chrome Content Scripts](https://developer.chrome.com/extensions/content_scripts#execution-environment). You can access this context in the dev tools by selecting the ‘Electron Isolated Context’ entry in the combo box at the top of the Console tab. **Note:** This option is currently experimental and may change or be removed in future Electron releases.
- `nativeWindowOpen` Boolean (optional) - Whether to use native `window.open()`. Defaults to `false`. **Note:** This option is currently experimental.
- `webviewTag` Boolean (optional) - Whether to enable the [`<webview>` tag](../webview-tag/index). Defaults to the value of the `nodeIntegration` option. **Note:** The `preload` script configured for the `<webview>` will have node integration enabled when it is executed so you should ensure remote/untrusted content is not able to create a `<webview>` tag with a possibly malicious `preload` script. You can use the `will-attach-webview` event on [webContents](../web-contents/index) to strip away the `preload` script and to validate or alter the `<webview>`’s initial settings.
使用minWidth
/ maxWidth
/ minHeight
/ 设置最小或最大窗口大小时maxHeight
,它仅限制用户。它不会阻止你传递的大小不符合尺寸约束setBounds
/ setSize
或的构造BrowserWindow
。
该type
选项的可能值和行为取决于平台。可能的值是:
- 在Linux上,可能的类型有
desktop
,dock
,toolbar
,splash
,notification
。 - 在macOS上,可能的类型是
desktop
,textured
。- 该
textured
类型添加了金属渐变外观(NSTexturedBackgroundWindowMask
)。 - 该
desktop
类型将窗口置于桌面背景窗口级别(kCGDesktopWindowLevel - 1
)。请注意,桌面窗口不会收到焦点,键盘或鼠标事件,但您可以使用它globalShortcut
来节省输入。
- 该
- 在Windows上,可能的类型是
toolbar
。
实例事件
创建的对象new BrowserWindow
会发出以下事件:
注意:某些事件仅在特定的操作系统上可用,并且被标记为这样。
事件:'page-title-updated'
返回:
-
event
事件 -
title
串
文档更改标题时发出,调用event.preventDefault()
将阻止本地窗口的标题更改。
事件:'close'
返回:
-
event
事件
当窗户将要关闭时发射。它在DOM beforeunload
和unload
事件之前发射。通话event.preventDefault()
将取消关闭。
通常你会想使用beforeunload
处理程序来决定窗口是否应该关闭,窗口重新加载时也会调用这个窗口。在Electron中,返回除了undefined
取消关闭以外的任何值。例如:
window.onbeforeunload = (e) => {
console.log('I do not want to be closed')
// Unlike usual browsers that a message box will be prompted to users, returning
// a non-void value will silently cancel the close.
// It is recommended to use the dialog API to let the user confirm closing the
// application.
e.returnValue = false
}
事件:'关闭'
窗户关闭时发射。收到此事件后,您应该删除对该窗口的引用并避免再使用它。
事件:'会话结束' Windows
窗口会话由于强制关机或机器重启或会话注销而结束时发出。
事件:'无响应'
当网页无响应时发出。
事件:'响应'
当无响应的网页再次变得响应时发出。
事件:'blur'
当窗口失去焦点时发射。
事件:'focus'
当窗口获得焦点时发射。
事件: ‘show’
显示窗口时发出。
事件: ‘hide’
隐藏窗口时发射。
事件: ‘ready-to-show’
当网页被渲染时(当没有被显示时)被发射,窗口可以在没有可视闪光的情况下被显示。
事件: ‘maximize’
窗口最大化时发射。
事件:'unmaximize'
窗口退出最大化状态时发出。
事件:'最小化'
当窗口最小化时发射。
事件: ‘restore’
窗口从最小化状态恢复时发出。
事件: ‘resize’
窗口大小调整时发射。
事件: ‘move’
窗口移动到新位置时发射。
注意:在macOS上,这个事件只是一个别名moved
。
事件: ‘moved’ macOS
窗口移动到新位置时发射一次。
事件: ‘enter-full-screen’
窗口进入全屏状态时发射。
事件: ‘leave-full-screen’
窗口离开全屏状态时发射。
事件: ‘enter-html-full-screen’
窗口进入由HTML API触发的全屏状态时发出。
事件: ‘leave-html-full-screen’
当窗口离开由HTML API触发的全屏状态时发出。
事件: ‘app-command’ Windows
返回:
-
event
事件 -
command
串
当应用程序命令(https://msdn.microsoft.com/en-us/library/windows/desktop/ms646275(v = vs.85%29.aspx))被调用时发出。这些通常与键盘媒体键或浏览器命令,以及内置于Windows上某些鼠标的“返回”按钮。
命令是小写的,下划线用连字符代替,并且APPCOMMAND_
前缀被剥离。例如APPCOMMAND_BROWSER_BACKWARD
被排出browser-backward
。
const {BrowserWindow} = require('electron')
let win = new BrowserWindow()
win.on('app-command', (e, cmd) => {
// Navigate the window back when the user hits their mouse back button
if (cmd === 'browser-backward' && win.webContents.canGoBack()) {
win.webContents.goBack()
}
})
事件: ‘scroll-touch-begin’ macOS
滚轮事件阶段开始时发出。
事件: ‘scroll-touch-end’ macOS
滚轮事件阶段结束时发出。
事件: ‘scroll-touch-edge’ macOS
当滚动轮事件相位到达元素的边缘时发出。
事件: ‘swipe’ macOS
返回:
-
event
事件 -
direction
串
用3指轻扫发射。可能的方向有up
,right
,down
,left
。
事件: ‘sheet-begin’ macOS
窗口打开表单时发出。
事件: ‘sheet-end’ macOS
窗口关闭表单时发出。
事件: ‘new-window-for-tab’ macOS
点击原生新标签按钮时发出。
静态方法
该BrowserWindow
班有下列静态方法:
BrowserWindow.getAllWindows()
返回BrowserWindow[]
- 所有打开的浏览器窗口的数组。
BrowserWindow.getFocusedWindow()
返回BrowserWindow
- 在此应用程序中关注的窗口,否则返回null
。
BrowserWindow.fromWebContents(webContents)
-
webContents
WebContents
返回BrowserWindow
- 拥有给定的窗口webContents
。
BrowserWindow.fromId(id)
-
id
整数
返回BrowserWindow
- 给定的窗口id
。
BrowserWindow.addExtension(path)
-
path
串
添加位于的Chrome扩展程序path
,并返回扩展程序的名称。
如果扩展的清单缺失或不完整,该方法也不会返回。
注意:ready
在app
模块事件发出之前,不能调用此API 。
BrowserWindow.removeExtension(name)
-
name
串
按名称删除Chrome扩展程序。
注意:ready
在app
模块事件发出之前,不能调用此API 。
BrowserWindow.getExtensions()
返回Object
- 键是扩展名,每个值都是包含对象name
和version
属性。
注意:ready
在app
模块事件发出之前,不能调用此API 。
BrowserWindow.addDevToolsExtension(path)
-
path
串
添加位于的DevTools扩展path
,并返回扩展的名称。
该扩展将被记住,所以你只需要调用这个API一次,这个API不适合编程使用。如果您尝试添加已经加载的扩展,则此方法不会返回,而是将警告记录到控制台。
如果扩展的清单缺失或不完整,该方法也不会返回。
注意:ready
在app
模块事件发出之前,不能调用此API 。
BrowserWindow.removeDevToolsExtension(name)
-
name
串
按名称删除DevTools扩展。
注意:ready
在app
模块事件发出之前,不能调用此API 。
BrowserWindow.getDevToolsExtensions()
返回Object
- 键是扩展名,每个值都是包含对象name
和version
属性。
要检查是否安装了DevTools扩展,您可以运行以下命令:
const {BrowserWindow} = require('electron')
let installed = BrowserWindow.getDevToolsExtensions().hasOwnProperty('devtron')
console.log(installed)
注意:ready
在app
模块事件发出之前,不能调用此API 。
实例属性
创建的对象new BrowserWindow
具有以下属性:
const {BrowserWindow} = require('electron')
// In this example `win` is our instance
let win = new BrowserWindow({width: 800, height: 600})
win.loadURL('https://github.com')
win.webContents
WebContents
此窗口拥有的对象。所有网页相关的事件和操作都将通过它完成。
请参阅webContents
文档以了解其方法和事件。
win.id
一个Integer
表示窗口的唯一ID。
实例方法
创建的对象new BrowserWindow
具有以下实例方法:
注意:某些方法仅在特定操作系统上可用,并且被标记为这样。
win.destroy()
强制关闭车窗,unload
和beforeunload
事件将不会被发出的网页,并且close
事件也将不会发出此窗口,但它保证了closed
事件将被发射。
win.close()
尝试关闭窗口。这与用户手动点击窗口的关闭按钮具有相同的效果。网页可能会取消关闭。查看关闭事件。
win.focus()
专注于窗口。
win.blur()
从窗口中移除焦点。
win.isFocused()
返回Boolean
- 窗口是否被聚焦。
win.isDestroyed()
返回Boolean
- 窗口是否被销毁。
win.show()
显示并关注窗口。
win.showInactive()
显示窗口,但不关注它。
win.hide()
隐藏窗口。
win.isVisible()
返回Boolean
- 窗口是否对用户可见。
win.isModal()
返回Boolean
- 当前窗口是否是模态窗口。
win.maximize()
最大化窗口。如果窗口没有被显示,这也会显示(但不会聚焦)窗口。
win.unmaximize()
取消最大化窗口。
win.isMaximized()
返回Boolean
- 窗口是否被最大化。
win.minimize()
最小化窗口。在某些平台上,最小化窗口将显示在Dock中。
win.restore()
将窗口从最小化状态恢复到之前的状态。
win.isMinimized()
返回Boolean
- 窗口是否最小化。
win.setFullScreen(flag)
-
flag
布尔
设置窗口是否应处于全屏模式。
win.isFullScreen()
返回Boolean
- 窗口是否处于全屏模式。
win.setAspectRatio(aspectRatio[, extraSize])
macOS
-
aspectRatio
浮动 - 为内容视图的某个部分维护的宽高比。 -
extraSize
尺寸 - 在保持宽高比的情况下不包括额外尺寸。
这将使窗口保持纵横比。额外的大小允许开发人员在像素中指定空间,但不包含在宽高比计算中。此API已经考虑到窗口大小与其内容大小之间的差异。
考虑一个具有高清视频播放器和相关控件的正常窗口。也许左边有15个像素的控件,右边有25个像素控件,播放器下面有50个控件。为了在播放器本身内保持16:9的宽高比(HD @ 1920x1080的标准宽高比),我们将使用16/9和40,50的参数来调用此函数。第二个参数并不关心额外宽度和高度在内容视图内的位置 - 只有它们存在。只需在总体内容视图中总结任何额外的宽度和高度区域。
win.previewFile(path[, displayName])
macOS
-
path
字符串 - 使用QuickLook预览文件的绝对路径。这一点很重要,因为Quick Look使用路径上的文件名和文件扩展名来确定要打开的文件的内容类型。 -
displayName
字符串(可选) - 要在快速浏览模式视图中显示的文件的名称。这纯粹是可视化的,不会影响文件的内容类型。默认为path
。
使用快速查看预览给定路径上的文件。
win.closeFilePreview()
macOS
关闭当前打开的快速查看面板。
win.setBounds(bounds[, animate])
-
bounds
长方形 -
animate
布尔(可选)macOS
调整窗口大小并将窗口移动到提供的边界
win.getBounds()
返回 Rectangle
win.setContentBounds(bounds[, animate])
-
bounds
长方形 -
animate
布尔(可选)macOS
调整窗口的客户区域(例如网页)的大小并将其移动到提供的边界。
win.getContentBounds()
返回 Rectangle
win.setSize(width, height[, animate])
-
width
整数 -
height
整数 -
animate
布尔(可选)macOS
将窗口大小调整为width
和height
。
win.getSize()
返回Integer[]
- 包含窗口的宽度和高度。
win.setContentSize(width, height[, animate])
-
width
整数 -
height
整数 -
animate
布尔(可选)macOS
将窗口的客户区(例如网页)调整为width
和height
。
win.getContentSize()
返回Integer[]
- 包含窗口客户区的宽度和高度。
win.setMinimumSize(width, height)
-
width
整数 -
height
整数
将窗口的最小大小设置为width
和height
。
win.getMinimumSize()
返回Integer[]
- 包含窗口的最小宽度和高度。
win.setMaximumSize(width, height)
-
width
整数 -
height
整数
将窗口的最大尺寸设置为width
和height
。
win.getMaximumSize()
返回Integer[]
- 包含窗口的最大宽度和高度。
win.setResizable(resizable)
-
resizable
布尔
设置用户是否可以手动调整窗口大小。
win.isResizable()
返回Boolean
- 窗口是否可以由用户手动调整大小。
win.setMovable(movable)
macOS Windows
-
movable
布尔
设置用户是否可以移动窗口。在Linux上什么都不做。
win.isMovable()
macOS Windows
返回Boolean
- 窗口是否可以由用户移动。
在Linux上总是返回true
。
win.setMinimizable(minimizable)
macOS Windows
-
minimizable
布尔
设置用户是否可以手动将窗口最小化。在Linux上什么都不做。
win.isMinimizable()
macOS Windows
返回Boolean
- 窗口是否可以由用户手动最小化
在Linux上总是返回true
。
win.setMaximizable(maximizable)
macOS Windows
-
maximizable
布尔
设置用户是否可以手动最大化窗口。在Linux上什么都不做。
win.isMaximizable()
macOS Windows
返回Boolean
- 窗口是否可以由用户手动最大化。
在Linux上总是返回true
。
win.setFullScreenable(fullscreenable)
-
fullscreenable
布尔
设置最大化/缩放窗口按钮是切换全屏模式还是最大化窗口。
win.isFullScreenable()
返回Boolean
- 最大化/缩放窗口按钮切换全屏模式还是最大化窗口。
win.setClosable(closable)
macOS Windows
-
closable
布尔
设置用户是否可以手动关闭窗口。在Linux上什么都不做。
win.isClosable()
macOS Windows
返回Boolean
- 窗口是否可以由用户手动关闭。
在Linux上总是返回true
。
win.setAlwaysOnTop(flag[, level][, relativeLevel])
-
flag
布尔 -
level
字符串(可选)的MacOS -值包括normal
,floating
,torn-off-menu
,modal-panel
,main-menu
,status
,pop-up-menu
,screen-saver
,和
(不推荐)。默认是dockfloating
。有关更多详细信息,请参阅macOS文档。 -
relativeLevel
整数(可选)macOS - 设置此窗口相对于给定值更高的层数level
。默认是0
。请注意,Apple不鼓励设置级别高于1screen-saver
。
设置窗口是否应始终显示在其他窗口之上。设置完成后,窗口仍然是一个正常的窗口,而不是无法集中的工具箱窗口。
win.isAlwaysOnTop()
返回Boolean
- 窗口是否始终位于其他窗口之上。
win.center()
将窗口移动到屏幕中央。
win.setPosition(x, y[, animate])
-
x
整数 -
y
整数 -
animate
布尔(可选)macOS
将窗口移至x
和y
。
win.getPosition()
返回Integer[]
- 包含窗口的当前位置。
win.setTitle(title)
-
title
串
将本地窗口的标题更改为title
。
win.getTitle()
返回String
- 本机窗口的标题。
注意:网页的标题可能与本地窗口的标题不同。
win.setSheetOffset(offsetY[, offsetX])
macOS
-
offsetY
浮点 -
offsetX
浮点(可选)
更改macOS上图纸的附着点。默认情况下,工作表附加在窗口框架的正下方,但您可能希望将它们显示在HTML呈现的工具栏下方。例如:
const {BrowserWindow} = require('electron')
let win = new BrowserWindow()
let toolbarRect = document.getElementById('toolbar').getBoundingClientRect()
win.setSheetOffset(toolbarRect.height)
win.flashFrame(flag)
-
flag
布尔
开始或停止闪烁窗口以吸引用户的注意力。
win.setSkipTaskbar(skip)
-
skip
布尔
使窗口不显示在任务栏中。
win.setKiosk(flag)
-
flag
布尔
进入或离开信息亭模式。
win.isKiosk()
返回Boolean
- 窗口是否处于信息亭模式。
win.getNativeWindowHandle()
返回Buffer
- 窗口的平台特定的句柄。
原生型手柄的是HWND
在Windows上,NSView*
在MacOS和Window
(unsigned long
)在Linux上。
win.hookWindowMessage(message, callback)
Windows
-
message
整数 -
callback
函数
钩住一个Windows消息。该callback
在的WndProc接收到消息时被调用。
win.isWindowMessageHooked(message)
Windows
-
message
整数
返回Boolean
- true
或false
取决于消息是否被挂钩。
win.unhookWindowMessage(message)
Windows
-
message
整数
解开窗口消息。
win.unhookAllWindowMessages()
Windows
解除所有窗口消息。
win.setRepresentedFilename(filename)
macOS
-
filename
字符串
设置窗口代表的文件的路径名,文件的图标将显示在窗口的标题栏中。
win.getRepresentedFilename()
macOS
返回String
- 窗口代表的文件的路径名。
win.setDocumentEdited(edited)
macOS
-
edited
布尔
指定窗口的文档是否已被编辑,并且设置为标题栏中的图标将变为灰色true
。
win.isDocumentEdited()
macOS
返回Boolean
- 窗口的文档是否已被编辑。
win.focusOnWebView()
win.blurWebView()
win.capturePage([rect, ]callback)
-
rect
矩形(可选) - 捕获的边界 -
callback
功能-
image
NativeImage
-
和webContents.capturePage([rect, ]callback)
一样。
win.loadURL(url[, options])
-
url
串 -
options
对象(可选)-
httpReferrer
字符串(可选) - HTTP引荐网址。 -
userAgent
字符串(可选) - 发起请求的用户代理。 -
extraHeaders
字符串(可选) - 额外的标题由“\ n”分隔 -
postData
(UploadRawData [] | UploadFile [] | UploadFileSystem [] | UploadBlob []) - (可选) -
baseURLForDataURL
字符串(可选) - 基础URL(带尾随路径分隔符)用于由数据URL加载的文件。只有当指定的url
是数据url并需要加载其他文件时,才需要此选项。
-
和webContents.loadURL(url[, options])
一样。
的url
可以是远程地址(例如http://
),或使用本地HTML文件的路径file://
协议。
为确保文件URL格式正确,建议使用Node的url.format
方法:
let url = require('url').format({
protocol: 'file',
slashes: true,
pathname: require('path').join(__dirname, 'index.html')
})
win.loadURL(url)
您可以POST
通过执行以下操作使用带有URL编码数据的请求加载URL :
win.loadURL('http://localhost:8000/post', {
postData: [{
type: 'rawData',
bytes: Buffer.from('hello=world')
}],
extraHeaders: 'Content-Type: application/x-www-form-urlencoded'
})
win.reload()
和webContents.reload
一样。
win.setMenu(menu)
Linux Windows
-
menu
菜单| 空值
设置menu
为窗口的菜单栏,将其设置为null
将删除菜单栏。
win.setProgressBar(progress[, options])
-
progress
双精度浮点数 -
options
对象(可选)-
mode
字符串Windows - 进度条的模式。可以none
,normal
,indeterminate
,error
,或paused
。
-
在进度条中设置进度值。有效范围是0,1.0。
进度<0时移除进度条; 进度> 1时更改为不确定模式。
在Linux平台上,仅支持统一的桌面环境,你需要指定*.desktop
文件名desktopName
字段package.json
。默认情况下,它会假设app.getName().desktop
。
在Windows上,可以传递一个模式。可接受的值是none
,normal
,indeterminate
,error
,和paused
。如果您setProgressBar
没有使用模式设置(但有效范围内的值),normal
则会被假定。
win.setOverlayIcon(overlay, description)
Windows
-
overlay
NativeImage - 显示在任务栏图标右下角的图标。如果这个参数是null
,覆盖被清除 -
description
字符串 - 将提供给辅助功能屏幕阅读器的说明
在当前任务栏图标上设置一个16 x 16像素叠加层,通常用于传达某种应用程序状态或被动地通知用户。
win.setHasShadow(hasShadow)
macOS
-
hasShadow
布尔
设置窗口是否应该有阴影。在Windows和Linux上什么都不做。
win.hasShadow()
macOS
返回Boolean
- 窗口是否有阴影。
在Windows和Linux上总是返回true
。
win.setThumbarButtons(buttons)
Windows
-
buttons
ThumbarButton[]
返回Boolean
- 按钮是否成功添加
在任务栏按钮布局中,将具有指定的一组按钮的缩略图工具栏添加到窗口的缩略图图像中。返回一个Boolean
对象指示缩略图是否已成功添加。
由于房间有限,缩略图工具栏中的按钮数量应不大于7个。一旦您设置了缩略图工具栏,由于平台的限制,无法移除工具栏。但是您可以使用空数组调用API来清理按钮。
这buttons
是一个Button
对象数组:
-
Button
目的-
icon
NativeImage - 缩略图工具栏中显示的图标。 -
click
功能 -
tooltip
字符串(可选) - 按钮工具提示的文本。 -
flags
字符串 - 控制按钮的特定状态和行为。默认情况下是['enabled']
。
-
该flags
数组可以包含以下String
s:
-
enabled
- 该按钮处于活动状态并可供用户使用。 -
disabled
- 该按钮被禁用。它存在,但有一个视觉状态表明它不会响应用户操作。 -
dismissonclick
- 点击该按钮时,缩略图窗口立即关闭。 -
nobackground
- 不要绘制按钮边框,只使用图像。 -
hidden
- 该按钮不会显示给用户。 -
noninteractive
- 该按钮已启用,但不能互动; 没有按下按钮状态绘制。此值适用于在通知中使用按钮的情况。
win.setThumbnailClip(region)
Windows
-
region
矩形 - 窗口的区域
将窗口的区域设置为显示为悬停在任务栏窗口上时显示的缩略图图像。您可以通过指定一个空白区域来将缩略图重置为整个窗口:{x: 0, y: 0, width: 0, height: 0}
。
win.setThumbnailToolTip(toolTip)
Windows
-
toolTip
字符串
设置将鼠标悬停在任务栏中的窗口缩略图上时显示的工具提示。
win.setAppDetails(options)
Windows
-
options
目的-
appId
字符串(可选) - 窗口的应用用户模型ID(https://msdn.microsoft.com/en-us/library/windows/desktop/dd391569(v = vs.85%29.aspx)。它必须设置,否则其他选项将不起作用。 -
appIconPath
字符串(可选) - 窗口的重新启动图标(https://msdn.microsoft.com/en-us/library/windows/desktop/dd391573(v = vs.85%29.aspx)。 -
appIconIndex
整数(可选) - 中的图标索引appIconPath
。appIconPath
未设置时忽略。默认是0
。 -
relaunchCommand
字符串(可选) - 窗口的重新启动命令(https://msdn.microsoft.com/en-us/library/windows/desktop/dd391571(v = vs.85%29.aspx)。 -
relaunchDisplayName
字符串(可选) - 窗口的重新启动显示名称(https://msdn.microsoft.com/en-us/library/windows/desktop/dd391572(v = vs.85%29.aspx)。
-
设置窗口任务栏按钮的属性。
注意: relaunchCommand
并且relaunchDisplayName
必须始终设置在一起。如果其中一个属性未设置,则不会使用。
win.showDefinitionForSelection()
macOS
和webContents.showDefinitionForSelection()
一样。
win.setIcon(icon)
Windows Linux
-
icon
NativeImage
更改窗口图标。
win.setAutoHideMenuBar(hide)
-
hide
布尔
设置窗口菜单栏是否应该自动隐藏。一旦设置菜单栏将仅在用户按下单个Alt
键时显示。
如果菜单栏已经可见,则调用setAutoHideMenuBar(true)
不会立即隐藏。
win.isMenuBarAutoHide()
返回Boolean
- 菜单栏是否自动隐藏自身。
win.setMenuBarVisibility(visible)
Windows Linux
-
visible
布尔
设置菜单栏是否应该可见。如果菜单栏是自动隐藏的,用户仍然可以通过按下单个Alt
键来调出菜单栏。
win.isMenuBarVisible()
返回Boolean
- 菜单栏是否可见。
win.setVisibleOnAllWorkspaces(visible)
-
visible
布尔
设置窗口是否应在所有工作区上可见。
注意:此API在Windows上不执行任何操作。
win.isVisibleOnAllWorkspaces()
返回Boolean
- 窗口是否在所有工作区上可见。
注意:此API在Windows上始终返回false。
win.setIgnoreMouseEvents(ignore)
-
ignore
布尔
使窗口忽略所有鼠标事件。
此窗口中发生的所有鼠标事件都将传递到此窗口下方的窗口,但如果此窗口具有焦点,它仍将接收键盘事件。
win.setContentProtection(enable)
macOS Windows
-
enable
布尔
防止窗口内容被其他应用程序捕获。
在macOS上,它将NSWindow的sharingType设置为NSWindowSharingNone。在Windows上,它调用SetWindowDisplayAffinity WDA_MONITOR
。
win.setFocusable(focusable)
Windows
-
focusable
布尔
改变窗口是否可以集中。
win.setParentWindow(parent)
Linux macOS
-
parent
BrowserWindow
设置parent
为当前窗口的父窗口,传递null
将当前窗口变成顶层窗口。
win.getParentWindow()
返回BrowserWindow
- 父窗口。
win.getChildWindows()
返回BrowserWindow[]
- 所有子窗口。
win.setAutoHideCursor(autoHide)
macOS
-
autoHide
布尔
控制是否在输入时隐藏光标。
win.setVibrancy(type)
macOS
-
type
字符串-可以是appearance-based
,light
,dark
,titlebar
,selection
,menu
,popover
,sidebar
,medium-light
或ultra-dark
。有关更多详细信息,请参阅macOS文档。
为浏览器窗口添加活力效果。传递null
或空字符串将消除窗口上的活力影响。
win.setTouchBar(touchBar)
macOS 实验
-
touchBar
触摸栏
设置当前窗口的touchBar布局。指定null
或undefined
清除触摸栏。如果机器具有触摸栏并且在macOS 10.12.1+上运行,此方法只有效果。
注意: TouchBar API目前是实验性的,可能会在未来的Electron版本中更改或删除。
win.setBrowserView(browserView)
试验
-
browserView
BrowserView中
注意: BrowserView API目前是实验性的,可能会在未来的Electron版本中更改或删除。
浏览器窗口 | BrowserWindow相关
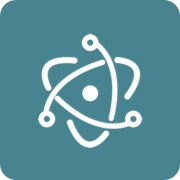
Electron 是一个使用 JavaScript, HTML 和 CSS 等 Web 技术创建原生程序的框架,它负责比较难搞的部分,你只需把精力放在你的应用的核心上即可。
主页 | https://electron.atom.io/ |
源码 | https://github.com/electron/electron |
发布版本 | 1.7.9 |