Typescript参考手册
更多 | What's New
TypeScript 1.7
async
/ await
支持ES6目标(Node v4 +)
TypeScript现在支持对ES6生成器具有本机支持的引擎的异步功能,例如Node v4及更高版本。异步函数的前缀是async
关键字; await
暂停执行,直到完成异步函数返回许诺并解开Promise
返回的值。
例
在以下示例中,每个输入元素将以400毫秒的延迟一次打印出一个:
"use strict";
// printDelayed is a 'Promise<void>'
async function printDelayed(elements: string[]) {
for (const element of elements) {
await delay(200);
console.log(element);
}
}
async function delay(milliseconds: number) {
return new Promise<void>(resolve => {
setTimeout(resolve, milliseconds);
});
}
printDelayed(["Hello", "beautiful", "asynchronous", "world"]).then(() => {
console.log();
console.log("Printed every element!");
});
有关更多信息,请参阅异步函数博客文章。
使用--module支持--target ES6
TypeScript 1.7将ES6添加到可用于--module标志的选项列表中,并允许您在定位ES6时指定模块输出。 这提供了更多的灵活性,以在特定运行时准确定位您想要的功能。
例
{
"compilerOptions": {
"module": "amd",
"target": "es6"
}
}
this
-typing
this
从一个方法中返回当前对象(即)以创建流畅样式的API是一种常见模式。例如,考虑以下BasicCalculator
模块:
export default class BasicCalculator {
public constructor(protected value: number = 0) { }
public currentValue(): number {
return this.value;
}
public add(operand: number) {
this.value += operand;
return this;
}
public subtract(operand: number) {
this.value -= operand;
return this;
}
public multiply(operand: number) {
this.value *= operand;
return this;
}
public divide(operand: number) {
this.value /= operand;
return this;
}
}
用户可以表达2 * 5 + 1
为
import calc from "./BasicCalculator";
let v = new calc(2)
.multiply(5)
.add(1)
.currentValue();
这通常会打开编写代码的非常优雅的方式; 然而,对于那些想要扩展的类来说存在一个问题BasicCalculator
。设想一个用户想要开始写一个ScientificCalculator
:
import BasicCalculator from "./BasicCalculator";
export default class ScientificCalculator extends BasicCalculator {
public constructor(value = 0) {
super(value);
}
public square() {
this.value = this.value ** 2;
return this;
}
public sin() {
this.value = Math.sin(this.value);
return this;
}
}
因为TypeScript用于推断返回的BasicCalculator
每种方法的类型,所以类型系统会忘记每次使用方法时都会有。BasicCalculatorthisScientificCalculatorBasicCalculator
例如:
import calc from "./ScientificCalculator";
let v = new calc(0.5)
.square()
.divide(2)
.sin() // Error: 'BasicCalculator' has no 'sin' method.
.currentValue();
现在不再是这种情况了 - TypeScript现在推断它有一个特殊的类型,只要在一个类的实例方法内部就可以调用它。 这种类型是这样写的,基本上意思是“方法调用中点的左侧类型”。
该this
类型在描述库(例如Ember.js)中使用mixin样式模式描述继承的交集类型时也很有用:
interface MyType {
extend<T>(other: T): this & T;
}
ES7指数运算符
TypeScript 1.7支持即将推出的ES7 / ES2016指数运算符:**
和**=
。操作员将使用输出转换为ES3 / ES5 Math.pow
。
例
var x = 2 ** 3;
var y = 10;
y **= 2;
var z = -(4 ** 3);
将生成以下JavaScript输出:
var x = Math.pow(2, 3);
var y = 10;
y = Math.pow(y, 2);
var z = -(Math.pow(4, 3));
改进了对解构对象字面值的检查
TypeScript 1.7使用对象文字或数组文字初始值设定项来检查解构模式的刚性和直观性更低。
当对象字面量按对象绑定模式的隐含类型进行上下文类型化时:
- 对象绑定模式中具有默认值的属性在对象字面量中变为可选。
- 对象绑定模式中的对象文本中没有匹配的属性需要在对象绑定模式中具有默认值,并自动添加到对象文本类型中。
- 对象文本中的对象绑定模式中没有匹配的属性是错误的。
当数组文字通过数组绑定模式的隐含类型进行上下文类型化时:
- 数组绑定模式中数组文本中没有匹配的元素需要在数组绑定模式中具有默认值,并自动添加到数组文本类型中。
例
// Type of f1 is (arg?: { x?: number, y?: number }) => void
function f1({ x = 0, y = 0 } = {}) { }
// And can be called as:
f1();
f1({});
f1({ x: 1 });
f1({ y: 1 });
f1({ x: 1, y: 1 });
// Type of f2 is (arg?: (x: number, y?: number) => void
function f2({ x, y = 0 } = { x: 0 }) { }
f2();
f2({}); // Error, x not optional
f2({ x: 1 });
f2({ y: 1 }); // Error, x not optional
f2({ x: 1, y: 1 });
针对ES3时支持修饰器
目标ES3时,装饰者现在被允许。 TypeScript 1.7从__decorate helper中删除特定于ES5的reduceRight。 这些更改还以向后兼容的方式调用Object.getOwnPropertyDescriptor和Object.defineProperty,以允许清理ES5的发射,并稍后删除对前述Object方法的各种重复调用。
更多 | What's New相关
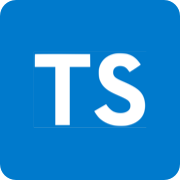
TypeScript 是 JavaScript 的类型的超集,它可以编译成纯 JavaScript。编译出来的 JavaScript 可以运行在任何浏览器上。
主页 | https://www.typescriptlang.org |
源码 | https://github.com/Microsoft/TypeScript |
发布版本 | 2.6.0 |