TensorFlow Guide参考手册
教程 | Tutorials
Mandelbrot Set
Mandelbrot集的可视化与机器学习没有任何关系,但它提供了一个有趣的例子,说明如何将TensorFlow用于一般数学。这实际上是一个简洁的可视化的实现,但它也体现了这一点。(我们最终可能会提供一个更精细的实现方式来生成更加真实美观的图像。)
基本设置
我们需要一些导入来开始。
# Import libraries for simulation
import tensorflow as tf
import numpy as np
# Imports for visualization
import PIL.Image
from io import BytesIO
from IPython.display import Image, display
现在我们将定义一个函数来实际显示图像,一旦我们有迭代计数。
def DisplayFractal(a, fmt='jpeg'):
"""Display an array of iteration counts as a
colorful picture of a fractal."""
a_cyclic = (6.28*a/20.0).reshape(list(a.shape)+[1])
img = np.concatenate([10+20*np.cos(a_cyclic),
30+50*np.sin(a_cyclic),
155-80*np.cos(a_cyclic)], 2)
img[a==a.max()] = 0
a = img
a = np.uint8(np.clip(a, 0, 255))
f = BytesIO()
PIL.Image.fromarray(a).save(f, fmt)
display(Image(data=f.getvalue()))
会话和变量初始化
为了像这样,我们经常使用互动式会话,但常规会话也可以使用。
sess = tf.InteractiveSession()
我们可以自由地混合NumPy和TensorFlow,非常方便。
# Use NumPy to create a 2D array of complex numbers
Y, X = np.mgrid[-1.3:1.3:0.005, -2:1:0.005]
Z = X+1j*Y
现在我们定义并初始化TensorFlow张量。
xs = tf.constant(Z.astype(np.complex64))
zs = tf.Variable(xs)
ns = tf.Variable(tf.zeros_like(xs, tf.float32))
TensorFlow要求您在使用它们之前明确地初始化变量。
tf.global_variables_initializer().run()
定义和运行计算
现在我们指定更多的计算...
# Compute the new values of z: z^2 + x
zs_ = zs*zs + xs
# Have we diverged with this new value?
not_diverged = tf.abs(zs_) < 4
# Operation to update the zs and the iteration count.
#
# Note: We keep computing zs after they diverge! This
# is very wasteful! There are better, if a little
# less simple, ways to do this.
#
step = tf.group(
zs.assign(zs_),
ns.assign_add(tf.cast(not_diverged, tf.float32))
)
...并运行它几百步
for i in range(200): step.run()
让我们看看我们得到了什么。
DisplayFractal(ns.eval())
还不错!
教程 | Tutorials相关
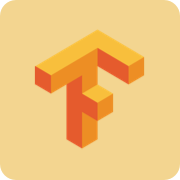
TensorFlow是谷歌基于DistBelief进行研发的第二代人工智能学习系统,其命名来源于本身的运行原理。Tensor(张量)意味着N维数组,Flow(流)意味着基于数据流图的计算,TensorFlow为张量从流图的一端流动到另一端计算过程。TensorFlow是将复杂的数据结构传输至人工智能神经网中进行分析和处理过程的系统。
主页 | https://www.tensorflow.org/ |
源码 | https://github.com/tensorflow/tensorflow |
版本 | Guide |
发布版本 | 1.4 |