React native参考手册
Components: WebView
WebView
WebView
以本地视图呈现网页内容。
import React, { Component } from 'react';
import { WebView } from 'react-native';
class MyWeb extends Component {
render() {
return (
<WebView
source={{uri: 'https://github.com/facebook/react-native'}}
style={{marginTop: 20}}
/>
);
}
}
您可以使用此组件在Web视图的历史记录中来回导航并配置Web内容的各种属性。
道具
ViewPropTypes props...
automaticallyAdjustContentInsets?: bool
控制是否调整放置在导航栏,选项卡栏或工具栏后面的Web视图的内容嵌入。默认值是true
。
contentInset?: {top: number, left: number, bottom: number, right: number}
网页浏览内容从滚动视图的边缘插入的量。默认为{top:0,left:0,bottom:0,right:0}。
html?: string
弃用
改用source
prop。
injectJavaScript?: function
接受一个字符串的函数,该字符串将被传递给WebView并作为JavaScript立即执行。
injectedJavaScript?: string
将其设置为提供在视图加载时将被注入网页的JavaScript。
mediaPlaybackRequiresUserAction?: bool
布尔值,用于确定HTML5音频和视频是否需要用户在开始播放之前点击它们。默认值是true
。
onError?: function
WebView
加载失败时调用的函数。
onLoad?: function
WebView
加载完成后调用的函数。
onLoadEnd?: function
WebView
加载成功或失败时调用的函数。
onLoadStart?: function
当WebView
开始加载时调用的函数。
onMessage?: function
在webview调用时调用的函数window.postMessage
。设置这个属性会postMessage
在你的webview中注入一个全局的,但仍然会调用预先存在的值postMessage
。
window.postMessage
接受一个参数,data
它将在事件对象上可用,event.nativeEvent.data
。data
必须是一个字符串。
onNavigationStateChange?: function
WebView
加载开始或结束时调用的函数。
renderError?: function
返回视图以显示是否有错误的函数。
renderLoading?: function
返回加载指示符的函数。
scalesPageToFit?: bool
布尔值,用于控制是否缩放网页内容以适应视图,并允许用户更改比例。默认值是true
。
source?: {uri: string, method: string, headers: object, body: string}, {html: string, baseUrl: string}, number
在WebView中加载静态html或uri(带有可选标题)。
startInLoadingState?: bool
强制WebView
在第一次加载时显示加载视图的布尔值。
style?: ViewPropTypes.style
该风格适用于WebView
。
url?: string
弃用
改用source
prop。
androiddomStorageEnabled?: bool
用于控制是否启用DOM存储的布尔值。仅用于Android。
androidjavaScriptEnabled?: bool
用于在JavaScript中启用JavaScript的布尔值WebView
。仅在Android上使用,因为iOS上默认启用JavaScript。默认值是true
。
androidmixedContentMode?: enum('never', 'always', 'compatibility')
指定混合内容模式。即WebView将允许一个安全的来源从任何其他来源加载内容。
可能的值mixedContentMode
是:
-
'never'
(默认) - WebView将不允许安全来源从不安全来源加载内容。
-
'always'
- WebView将允许安全来源加载来自任何其他来源的内容,即使该来源不安全。
-
'compatibility'
- WebView将尝试与现代Web浏览器在混合内容方面的兼容性。
androidthirdPartyCookiesEnabled?: bool
用于启用第三方cookie的布尔值WebView
。仅适用于Android棒棒糖及以上版本,因为默认情况下Android Kitkat及以下版本和iOS版本启用了第三方Cookie。默认值是true
。
androiduserAgent?: string
设置用户代理WebView
。
iosallowsInlineMediaPlayback?: bool
布尔值,用于确定HTML5视频是内联播放还是使用本机全屏控制器。默认值是false
。
注:为了使视频以内联方式播放,不仅需要将此属性设置为true
,而且HTML文档中的视频元素还必须包含该webkit-playsinline
属性。
iosbounces?: bool
布尔值,用于确定Web视图到达内容边缘时是否弹起。默认值是true
。
iosdataDetectorTypes?: enum('phoneNumber', 'link', 'address', 'calendarEvent', 'none', 'all'), [enum('phoneNumber', 'link', 'address', 'calendarEvent', 'none', 'all')]
确定在Web视图内容中转换为可点击网址的数据类型。默认情况下只检测到电话号码。
您可以提供一种类型或多种类型的数组。
Possible values for dataDetectorTypes
are:
'phoneNumber'
'link'
'address'
'calendarEvent'
'none'
'all'
iosdecelerationRate?: ScrollView.propTypes.decelerationRate
一个浮点数,用于确定用户抬起手指后滚动视图的减速速度。您也可以使用字符串快捷键"normal"
和"fast"
匹配底层的iOS设置,这UIScrollViewDecelerationRateNormal
和UIScrollViewDecelerationRateFast
分别为:
- normal: 0.998
- fast: 0.99 (the default for iOS web view)
iosonShouldStartLoadWithRequest?: function
允许自定义处理任何Web查看请求的函数。true
从函数返回以继续加载请求并false
停止加载。
iosscrollEnabled?: bool
用于确定是否启用滚动的布尔值WebView
。默认值是true
。
Components: WebView相关
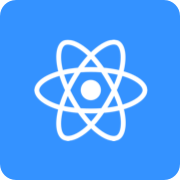
React Native 是一个 JavaScript 的框架,用来撰写实时的、可原生呈现 iOS 和 Android 的应用。
主页 | https://facebook.github.io/react-native/ |
源码 | https://github.com/facebook/react-native |
发布版本 | 0.49 |