React native参考手册
Components: TextInput
TextInput
通过键盘输入文本到应用程序的基本组件。道具为几种功能提供了可配置性,如自动更正,自动大写,占位符文本以及不同的键盘类型,如数字键盘。
最简单的用例是放下a TextInput
并订阅onChangeText
事件以读取用户输入。还有其他事件,例如onSubmitEditing
和onFocus
可以订阅。一个简单的例子:
import React, { Component } from 'react';
import { AppRegistry, TextInput } from 'react-native';
export default class UselessTextInput extends Component {
constructor(props) {
super(props);
this.state = { text: 'Useless Placeholder' };
}
render() {
return (
<TextInput
style={{height: 40, borderColor: 'gray', borderWidth: 1}}
onChangeText={(text) => this.setState({text})}
value={this.state.text}
/>
);
}
}
// skip this line if using Create React Native App
AppRegistry.registerComponent('AwesomeProject', () => UselessTextInput);
通过native元素公开的两个方法是.focus()和.blur(),它们将以编程方式聚焦或模糊TextInput。
请注意,有些道具只适用于multiline={true/false}
。此外,适用于只有一个元素的侧边框样式(如borderBottomColor
,borderLeftWidth
等)不会被如果应用multiline=false
。为了达到同样的效果,你可以把你的包装TextInput
放在View
:
import React, { Component } from 'react';
import { AppRegistry, View, TextInput } from 'react-native';
class UselessTextInput extends Component {
render() {
return (
<TextInput
{...this.props} // Inherit any props passed to it; e.g., multiline, numberOfLines below
editable = {true}
maxLength = {40}
/>
);
}
}
export default class UselessTextInputMultiline extends Component {
constructor(props) {
super(props);
this.state = {
text: 'Useless Multiline Placeholder',
};
}
// If you type something in the text box that is a color, the background will change to that
// color.
render() {
return (
<View style={{
backgroundColor: this.state.text,
borderBottomColor: '#000000',
borderBottomWidth: 1 }}
>
<UselessTextInput
multiline = {true}
numberOfLines = {4}
onChangeText={(text) => this.setState({text})}
value={this.state.text}
/>
</View>
);
}
}
// skip these lines if using Create React Native App
AppRegistry.registerComponent(
'AwesomeProject',
() => UselessTextInputMultiline
);
TextInput
在其视图底部默认有一个边框。此边框的填充由系统提供的背景图像设置,并且不能更改。避免这种情况的解决方案是,不要明确设置高度,系统将负责在正确的位置显示边框,或者不要通过设置underlineColorAndroid
为透明来显示边框。
请注意,在Android上执行文本选择输入可以改变应用程序的活动windowSoftInputMode
参数adjustResize
。这可能会导致在键盘处于活动状态时位置为“绝对”的组件出现问题。为了避免这种行为,可以windowSoftInputMode
在AndroidManifest.xml(https://developer.android.com/guide/topics/manifest/activity-element.html)中指定或使用本地代码以编程方式控制此参数。
道具
ViewPropTypes props...
autoCapitalize?: enum('none', 'sentences', 'words', 'characters')
可以告诉TextInput
自动大写某些字符。
-
characters
:所有人物。
-
words
:每个单词的第一个字母。
-
sentences
:每个句子的首字母(默认)。
-
none
:不要自动大写任何东西。
自动更正?: bool
如果false
,禁用自动更正。默认值是true
。
自动对焦?: bool
如果true
,把注意力集中在componentDidMount
。默认值是false
。
blurOnSubmit?: bool
如果true
,提交时文本字段会模糊。单行字段的默认值为true,多行字段的默认值为false。请注意,对于多行字段,设置blurOnSubmit
为true
意味着按下回车会模糊字段并触发onSubmitEditing
事件,而不是将新行插入字段。
caretHidden?: bool
如果true
,输入光标被隐藏。默认值是false
。
默认值?: string
提供一个初始值,当用户开始输入时将会改变。对于不希望处理收听事件和更新值支持以保持受控状态同步的简单用例很有用。
editable?: bool
如果false
文本不可编辑。默认值是true
。
keyboardType?: enum('default', 'email-address', 'numeric', 'phone-pad', 'ascii-capable', 'numbers-and-punctuation', 'url', 'number-pad', 'name-phone-pad', 'decimal-pad', 'twitter', 'web-search')
确定要打开哪个键盘,例如numeric
。
以下值适用于各种平台:
default
numeric
email-address
phone-pad
maxHeight?: number
如果自动增长true
,则限制TextInput框可以增长到的高度。一旦达到这个高度,TextInput就会变成可滚动的。
maxLength?: number
限制可以输入的最大字符数。使用这个而不是在JS中实现逻辑来避免闪烁。
multiline?: bool
如果true
,文本输入可以是多行。默认值是false
。
onBlur?: function
当文本输入模糊时调用的回调函数。
onChange?: function
在文本输入文本更改时调用的回调函数。
onChangeText?: function
在文本输入文本更改时调用的回调函数。已更改的文本作为参数传递给回调处理程序。
onContentSizeChange?: function
当文本输入内容大小改变时调用的回调。这将被称为{ nativeEvent: { contentSize: { width, height } } }
。
只需要多行文本输入。
onEndEditing?: function
当文本输入结束时调用的回调函数。
onFocus?: function
在文本输入焦点时调用的回调。
onLayout?: function
在装载和布局更改时调用{x, y, width, height}
。
onScroll?: function
在内容卷轴上调用{ nativeEvent: { contentOffset: { x, y } } }
。也可能包含来自ScrollEvent的其他属性,但在Android上,由于性能原因不提供contentSize。
onSelectionChange?: function
当文本输入选择被改变时被调用的回调。这将被称为{ nativeEvent: { selection: { start, end } } }
。
onSubmitEditing?: function
当文本输入的提交按钮被按下时调用的回调。无效如果multiline={true}
指定。
placeholder?: node
输入文字前输入的字符串。
placeholderTextColor?: color
占位符字符串的文本颜色。
returnKeyType?: enum('done', 'go', 'next', 'search', 'send', 'none', 'previous', 'default', 'emergency-call', 'google', 'join', 'route', 'yahoo')
确定返回键的外观。在Android上,您也可以使用returnKeyLabel
。
Cross platform
以下值适用于各种平台:
done
go
next
search
send
仅限Android
以下值仅适用于Android:
none
previous
iOS Only
以下值仅适用于iOS:
default
emergency-call
google
join
route
yahoo
secureTextEntry?: bool
如果true
输入的文本模糊了输入的文本,以便像密码这样的敏感文本保持安全。默认值是false
。
selectTextOnFocus?: bool
如果true
,所有文字将自动在焦点上选择。
selection?: {start: number, end: number}
文本输入选择的开始和结束。将开始和结束设置为相同的值以定位光标。
selectionColor?: color
文本输入的高亮和光标颜色。
style?: Text#style
请注意,并非所有文本样式都受支持,请参阅问题#7070以获取更多详细信息。
样式
value?: string
要为文本输入显示的值。TextInput
是一个受控组件,这意味着如果提供了本地值,它将被迫匹配这个值prop。对于大多数用途而言,这种方式效果很好,但在某些情况下,这可能会导致闪烁 - 一种常见原因是通过保持相同的值来防止编辑。除了设置相同的值之外,还可以进行设置editable={false}
或设置/更新maxLength
以防止无闪烁的不需要的编辑。
androidautoGrow?: bool
如果为true,则会在需要时增加文本框的高度。如果为false,则在达到高度时,文本框将变为可滚动。默认值是false。
androiddisableFullscreenUI?: bool
如果false
,如果有可用围绕一个文本输入(在手机上如横向)的少量空间,操作系统可以选择让用户编辑全屏幕文字输入模式中的文本。当true
此功能被禁用时,用户将总是直接在文本输入框内编辑文本。默认为false
。
androidinlineImageLeft?: string
如果已定义,则提供的图像资源将显示在左侧。图像资源必须在里面/android/app/src/main/res/drawable
并参考
<TextInput
inlineImageLeft='search_icon'
/>
androidinlineImagePadding?: number
内联图像(如果有)和文本输入本身之间进行填充。
androidnumberOfLines?: number
设置行数TextInput
。将其与多行设置为true
可以填充行。
androidreturnKeyLabel?: string
将返回键设置为标签。用它代替returnKeyType
。
androidtextBreakStrategy?: enum('simple', 'highQuality', 'balanced')
在Android API等级23+设置文本突破策略,可能的值是simple
,highQuality
,balanced
默认值是simple
。
androidunderlineColorAndroid?: color
TextInput
下划线的颜色。
iosclearButtonMode?: enum('never', 'while-editing', 'unless-editing', 'always')
当清除按钮应该出现在文本视图的右侧时。
iosclearTextOnFocus?: bool
如果true
,在编辑开始时自动清除文本字段。
iosdataDetectorTypes?: enum('phoneNumber', 'link', 'address', 'calendarEvent', 'none', 'all'), [enum('phoneNumber', 'link', 'address', 'calendarEvent', 'none', 'all')]
确定在文本输入中转换为可点击网址的数据类型。仅在multiline={true}
和时有效editable={false}
。默认情况下,没有检测到数据类型。
您可以提供一种类型或多种类型的数组。
可能的值dataDetectorTypes
是:
'phoneNumber'
'link'
'address'
'calendarEvent'
'none'
'all'
iosenablesReturnKeyAutomatically?: bool
如果true
键盘在没有文本时禁用返回键,并在有文本时自动启用。默认值是false
。
ioskeyboardAppearance?: enum('default', 'light', 'dark')
确定键盘的颜色。
iosonKeyPress?: function
当按下某个键时调用的回调函数。这将被称为与{ nativeEvent: { key: keyValue } }
哪里keyValue
是'Enter'
或'Backspace'
各自的键和输入的字符,否则包括' '
空间。在onChange
回调之前触发。
iosselectionState?: DocumentSelectionState
的实例DocumentSelectionState
,这是一些国家是负责维护选择信息的文档。
可以使用此实例执行的一些功能是:
blur()
focus()
update()
您可以参考
DocumentSelectionState
在vendor/document/selection/DocumentSelectionState.js
iosspellCheck?: bool
如果false
禁用拼写检查样式(即红色下划线)。默认值是继承自autoCorrect
。
方法
isFocused():
true
如果输入当前为焦点,则返回; false
除此以外。
clear()
从中删除所有文本TextInput
。
Components: TextInput相关
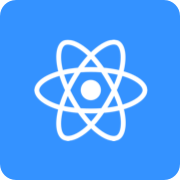
React Native 是一个 JavaScript 的框架,用来撰写实时的、可原生呈现 iOS 和 Android 的应用。
主页 | https://facebook.github.io/react-native/ |
源码 | https://github.com/facebook/react-native |
发布版本 | 0.49 |