React native参考手册
Components: Text
Text
用于显示文本的React组件。
Text
支持嵌套,造型和触摸处理。
在以下示例中,嵌套标题和正文文本将继承fontFamily
from styles.baseText
,但标题提供了它自己的其他样式。由于字面换行符的缘故,标题和正文将相互叠加:
import React, { Component } from 'react';
import { AppRegistry, Text, StyleSheet } from 'react-native';
export default class TextInANest extends Component {
constructor(props) {
super(props);
this.state = {
titleText: "Bird's Nest",
bodyText: 'This is not really a bird nest.'
};
}
render() {
return (
<Text style={styles.baseText}>
<Text style={styles.titleText} onPress={this.onPressTitle}>
{this.state.titleText}{'\n'}{'\n'}
</Text>
<Text numberOfLines={5}>
{this.state.bodyText}
</Text>
</Text>
);
}
}
const styles = StyleSheet.create({
baseText: {
fontFamily: 'Cochin',
},
titleText: {
fontSize: 20,
fontWeight: 'bold',
},
});
// skip this line if using Create React Native App
AppRegistry.registerComponent('TextInANest', () => TextInANest);
嵌套文本
iOS和Android都允许您通过使用特定格式(如NSAttributedString
iOS 或SpannableString
Android)上的特定格式注释字符串范围来显示格式化文本。在实践中,这是非常乏味的。对于React Native,我们决定使用网络范例,您可以在其中嵌套文本以实现相同的效果。
import React, { Component } from 'react';
import { AppRegistry, Text } from 'react-native';
export default class BoldAndBeautiful extends Component {
render() {
return (
<Text style={{fontWeight: 'bold'}}>
I am bold
<Text style={{color: 'red'}}>
and red
</Text>
</Text>
);
}
}
// skip this line if using Create React Native App
AppRegistry.registerComponent('AwesomeProject', () => BoldAndBeautiful);
在幕后,React Native将其转换为单位NSAttributedString
或SpannableString
包含以下信息:
"I am bold and red"
0-9: bold
9-17: bold, red
Nested views (iOS only)
在iOS上,您可以在文本组件中嵌套视图。这是一个例子:
import React, { Component } from 'react';
import { AppRegistry, Text, View } from 'react-native';
export default class BlueIsCool extends Component {
render() {
return (
<Text>
There is a blue square
<View style={{width: 50, height: 50, backgroundColor: 'steelblue'}} />
in between my text.
</Text>
);
}
}
// skip this line if using Create React Native App
AppRegistry.registerComponent('AwesomeProject', () => BlueIsCool);
为了使用这个功能,你必须给视图a
width
和aheight
。
集装箱
该<Text>
元素相对于布局是特殊的:里面的所有内容不再使用flexbox布局,而是使用文本布局。这意味着a中的元素<Text>
不再是矩形,而是当它们看到行的末尾时换行。
<Text>
<Text>First part and </Text>
<Text>second part</Text>
</Text>
// Text container: all the text flows as if it was one
// |First part |
// |and second |
// |part |
<View>
<Text>First part and </Text>
<Text>second part</Text>
</View>
// View container: each text is its own block
// |First part |
// |and |
// |second part|
有限的风格继承
在网络上,为整个文档设置字体系列和大小的常用方法是利用继承的CSS属性,如下所示:
html {
font-family: 'lucida grande', tahoma, verdana, arial, sans-serif;
font-size: 11px;
color: #141823;
}
文档中的所有元素都将继承此字体,除非他们或其父母之一指定了新规则。
在React Native中,我们对它更加严格:必须将所有文本节点包装在 组件中。你不能直接在一个文本节点下。<Text>
<View>
// BAD: will raise exception, can't have a text node as child of a <View>
<View>
Some text
</View>
// GOOD
<View>
<Text>
Some text
</Text>
</View>
您也失去了为整个子树设置默认字体的能力。建议在应用程序中使用一致字体和大小的方法是创建一个MyAppText
包含它们的组件,并在应用程序中使用此组件。您还可以使用此组件来制作更多特定的组件,例如MyAppHeaderText
其他类型的文本。
<View>
<MyAppText>Text styled with the default font for the entire application</MyAppText>
<MyAppHeaderText>Text styled as a header</MyAppHeaderText>
</View>
假设这MyAppText
是一个组件,它只是将其子项渲染为Text
具有样式的组件,则MyAppHeaderText
可以如下定义:
class MyAppHeaderText extends Component {
render() {
<MyAppText>
<Text style={{fontSize: 20}}>
{this.props.children}
</Text>
</MyAppText>
}
}
MyAppText
用这种方式编写可以确保我们从顶层组件中获取样式,但让我们能够在特定用例中添加/覆盖它们。
React Native仍然具有样式继承的概念,但仅限于文本子树。在这种情况下,第二部分将是粗体和红色。
<Text style={{fontWeight: 'bold'}}>
I am bold
<Text style={{color: 'red'}}>
and red
</Text>
</Text>
我们相信,这种更受限制的文本样式将产生更好的应用程序:
- (开发人员)React组件设计时考虑到了强大的隔离性:您应该能够在应用程序的任何位置放置组件,相信只要道具相同,它的外观和行为方式都是相同的。可以从道具外部继承的文本属性将打破这种隔离。
- (Implementor)React Native的实现也被简化了。我们不需要
fontFamily
在每个元素上都有一个字段,并且每次显示文本节点时都不需要遍历树根。样式继承只在本地Text组件内进行编码,不会泄漏到其他组件或系统本身。
道具
accessible?: bool
设置为时true
,表示该视图是可访问性元素。Text
元素的默认值是true
。
请参阅无障碍指南了解更多信息。
allowFontScaling?: bool
指定字体是否应缩放以尊重文本大小可访问性设置。默认是true
。
ellipsizeMode?: enum('head', 'middle', 'tail', 'clip')
何时numberOfLines
设置,这个道具定义了文本将被截断的方式。numberOfLines
必须与此道具一起设置。
这可以是以下值之一:
-
head
- 显示该行以便末端适合容器,并且在行首的缺失文本由省略号字形表示。例如“... wxyz”
-
middle
- 显示的行使开始和结束符合容器,中间的缺失文本由省略号字形表示。“AB ... YZ”
-
tail
- 显示该行,以便开始适合容器,并且在行末尾缺少的文本由省略号字形表示。例如“abcd ...”
-
clip
- 线条不会通过文本容器的边缘绘制。
默认是tail
。
clip
仅适用于iOS
nativeID?: string
用于从本机代码查找此视图。
numberOfLines?: number
用于在计算文本布局(包括换行)后使用省略号截断文本,以使行总数不超过此数字。
这个道具是常用的ellipsizeMode
。
onLayout?: function
在装载和布局更改时调用
{nativeEvent: {layout: {x, y, width, height}}}
onLongPress?: function
该功能在长按时被调用。
e.g., onLongPress={this.increaseSize}>
onPress?: function
此功能在印刷机上被调用。
e.g., onPress={() => console.log('1st')}
pressRetentionOffset?: {top: number, left: number, bottom: number, right: number}
当滚动视图被禁用时,这将定义您的触摸离开按钮有多远,然后停用按钮。一旦停用,请尝试将其移回,并且您会看到该按钮再次被重新激活!在滚动视图禁用时,将它来回移动数次。确保你传入一个常量来减少内存分配。
selectable?: bool
让用户选择文本,以使用本机复制和粘贴功能。
style?: style
View#style...
color color
fontFamily string
fontSize number
fontStyle enum('normal', 'italic')
fontWeight enum('normal','bold','100','200','300','400','500','600','700','800','900')指定字体重量。大多数字体支持“正常”和“粗体”值。并非所有的字体都有各个数值的变体,在这种情况下,选择最接近的一个。
lineHeight号码
textAlign枚举('auto','left','right','center','justify')指定文本对齐。值'justify'只支持iOS和left
在Android 上回退。
textDecorationLine enum('none', 'underline', 'line-through', 'underline line-through')
textShadowColor color
textShadowOffset {width: number, height: number}
textShadowRadius number
androidincludeFontPadding bool设置为false
删除额外的字体填充,旨在为某些上行/ 下行空间腾出空间。使用一些字体时,这种填充可以使文本看起来在垂直居中时略微不对齐。为了最好的结果也设置textAlignVertical
为center
。默认值是true。
androidtextAlignVertical enum('auto', 'top', 'bottom', 'center')
iosfontVariant enum('small-caps', 'oldstyle-nums', 'lining-nums', 'tabular-nums', 'proportional-nums')
iosletterSpacing number
iostextDecorationColor color
iostextDecorationStyle enum('solid', 'double', 'dotted', 'dashed')
ioswritingDirection enum('auto', 'ltr', 'rtl')
testID?: string
用于在端到端测试中定位此视图。
androiddisabled?: bool
为测试目的指定文本视图的禁用状态
androidselectionColor?: color
文本的高亮颜色。
androidtextBreakStrategy?: enum('simple', 'highQuality', 'balanced')
在Android API等级23+设置文本突破策略,可能的值是simple
,highQuality
,balanced
默认值是highQuality
。
iosadjustsFontSizeToFit?: bool
指定字体是否应该自动缩小以适应给定的样式约束。
iosminimumFontScale?: number
指定启用adjustsFontSizeToFit时字体可以达到的最小可能缩放比例。(值为0.01-1.0)。
iossuppressHighlighting?: bool
当true
文本被按下时,没有视觉改变。默认情况下,灰色椭圆形突出显示按下时的文字。
Components: Text相关
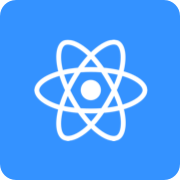
React Native 是一个 JavaScript 的框架,用来撰写实时的、可原生呈现 iOS 和 Android 的应用。
主页 | https://facebook.github.io/react-native/ |
源码 | https://github.com/facebook/react-native |
发布版本 | 0.49 |