React native参考手册
Components: NavigatorIOS
NavigatorIOS
NavigatorIOS
是一个包装UINavigationController
,使您能够实现导航堆栈。它的工作原理与使用本机应用程序时UINavigationController
完全相同,从UIKit提供相同的动画和行为。
顾名思义,它只能在iOS上使用。查看React Navigation
JavaScript中的跨平台解决方案,或查看以下任一组件以获得本机解决方案:本机导航,反应本机导航。
要设置导航器,请为initialRoute
道具提供路径对象。路线对象用于描述您的应用导航到的每个场景。initialRoute
代表导航器中的第一条路线。
import PropTypes from 'prop-types';
import React, { Component } from 'react';
import { NavigatorIOS, Text } from 'react-native';
export default class NavigatorIOSApp extends Component {
render() {
return (
<NavigatorIOS
initialRoute={{
component: MyScene,
title: 'My Initial Scene',
}}
style={{flex: 1}}
/>
);
}
}
class MyScene extends Component {
static propTypes = {
title: PropTypes.string.isRequired,
navigator: PropTypes.object.isRequired,
}
_onForward = () => {
this.props.navigator.push({
title: 'Scene ' + nextIndex,
});
}
render() {
return (
<View>
<Text>Current Scene: { this.props.title }</Text>
<TouchableHighlight onPress={this._onForward}>
<Text>Tap me to load the next scene</Text>
</TouchableHighlight>
</View>
)
}
}
在此代码中,导航器呈现initialRoute中指定的组件,在本例中为MyScene
。该组件将收到代表导航器的route
道具和navigator
道具。导航器的导航栏将呈现当前场景的标题“我的初始场景”。
您可以选择将passProps
属性传递给您的initialRoute
。NavigatorIOS
将其作为道具传递给渲染组件:
initialRoute={{
component: MyScene,
title: 'My Initial Scene',
passProps: { myProp: 'foo' }
}}
然后你可以访问通过的道具{this.props.myProp}
。
处理导航
要触发导航功能(例如推送或弹出视图),您可以访问navigator
对象。该对象作为道具传递给渲染的任何组件NavigatorIOS
。然后,您可以调用相关方法来执行所需的导航操作:
class MyView extends Component {
_handleBackPress() {
this.props.navigator.pop();
}
_handleNextPress(nextRoute) {
this.props.navigator.push(nextRoute);
}
render() {
const nextRoute = {
component: MyView,
title: 'Bar That',
passProps: { myProp: 'bar' }
};
return(
<TouchableHighlight onPress={() => this._handleNextPress(nextRoute)}>
<Text style={{marginTop: 200, alignSelf: 'center'}}>
See you on the other nav {this.props.myProp}!
</Text>
</TouchableHighlight>
);
}
}
您也可以从NavigatorIOS
组件中触发导航器功能:
class NavvyIOS extends Component {
_handleNavigationRequest() {
this.refs.nav.push({
component: MyView,
title: 'Genius',
passProps: { myProp: 'genius' },
});
}
render() {
return (
<NavigatorIOS
ref='nav'
initialRoute={{
component: MyView,
title: 'Foo This',
passProps: { myProp: 'foo' },
rightButtonTitle: 'Add',
onRightButtonPress: () => this._handleNavigationRequest(),
}}
style={{flex: 1}}
/>
);
}
}
上面的代码添加了一个_handleNavigationRequest
私有方法,NavigatorIOS
当按下右侧导航栏项时,该方法从组件中调用。为了访问导航器功能,对其的引用保存在ref
道具中,并在稍后参考将新场景推入导航堆栈。
导航栏配置
传递的道具NavigatorIOS
将为导航栏设置默认配置。作为属性传递给路由对象的道具将设置该路线导航栏的配置,覆盖传递给该NavigatorIOS
组件的任何道具。
_handleNavigationRequest() {
this.refs.nav.push({
//...
passProps: { myProp: 'genius' },
barTintColor: '#996699',
});
}
render() {
return (
<NavigatorIOS
//...
style={{flex: 1}}
barTintColor='#ffffcc'
/>
);
}
在上面的示例中,导航栏颜色在推送新路线时发生更改。
Props
barStyle?: enum('default', 'black')
导航栏的样式。支持的值是“默认”,“黑色”。使用“黑色”而不是barTintColor
黑色设置。这会生成具有更高半透明度的原生iOS样式的导航栏。
barTintColor?: string
导航栏的默认背景颜色。
initialRoute: {component: function, title: string, titleImage: Image.propTypes.source, passProps: object, backButtonIcon: Image.propTypes.source, backButtonTitle: string, leftButtonIcon: Image.propTypes.source, leftButtonTitle: string, leftButtonSystemIcon: Object.keys(SystemIcons), onLeftButtonPress: function, rightButtonIcon: Image.propTypes.source, rightButtonTitle: string, rightButtonSystemIcon: Object.keys(SystemIcons), onRightButtonPress: function, wrapperStyle: ViewPropTypes.style, navigationBarHidden: bool, shadowHidden: bool, tintColor: string, barTintColor: string, barStyle: enum('default', 'black'), titleTextColor: string, translucent: bool}
NavigatorIOS使用route
对象来标识子视图,其道具和导航栏配置。诸如推送操作之类的导航操作期望路线看起来像这样initialRoute
。
interactivePopGestureEnabled?: bool
指示是否启用交互式弹出手势的布尔值。这对于启用/禁用向后滑动导航手势很有用。
如果未提供此道具,则默认行为是在导航栏显示时启用后向滑动手势,隐藏导航栏时禁用。一旦你提供了interactivePopGestureEnabled
道具,你永远不会恢复默认行为。
itemWrapperStyle?: ViewPropTypes.style
导航器中组件的默认包装器样式。一个常见的用例是backgroundColor
为每个场景设置。
navigationBarHidden?: bool
布尔值,指示导航栏是否默认隐藏。
shadowHidden?: bool
布尔值,指示是否默认隐藏1px发际线阴影。
tintColor?: string
导航栏中用于按钮的默认颜色。
titleTextColor?: string
导航栏标题的默认文本颜色。
translucent?: bool
布尔值,指示默认情况下导航栏是否半透明
方法
push(route: object)
转到新路线。
参数:
名称和类型 |
描述 |
---|---|
路由对象 |
要导航到的新路线。 |
popN(n: number)
一次返回N个场景。当N = 1时,行为匹配pop()
。
参数:
名称和类型 |
描述 |
---|---|
n已接收 |
要弹出的场景数量。 |
pop()
回到前一个场景。
replaceAtIndex(route: object, index: number)
在导航堆栈中替换路线。
参数:
名称和类型 |
描述 |
---|---|
路由对象 |
新的路线将取代指定的路线。 |
indexnumber |
进入堆栈的路线应该被替换。如果是负数,则从堆栈的后面开始计数。 |
replace(route: object)
替换当前场景的路由,并立即加载新路由的视图。
参数:
名称和类型 |
描述 |
---|---|
路由对象 |
要导航到的新路线。 |
replacePrevious(route: object)
替换前一个场景的路线/视图。
参数:
名称和类型 |
描述 |
---|---|
路由对象 |
新的路线将取代以前的场景。 |
popToTop()
返回导航堆栈中最顶端的项目。
popToRoute(route: object)
返回到特定路由对象的项目。
参数:
名称和类型 |
描述 |
---|---|
路由对象 |
要导航到的新路线。 |
replacePreviousAndPop(route: object)
替换之前的路线/视图并转换回它。
参数:
名称和类型 |
描述 |
---|---|
路由对象 |
取代以前场景的新路线。 |
resetTo(route: object)
替换顶部项目并弹出。
参数:
名称和类型 |
描述 |
---|---|
路由对象 |
新的路线将取代最上面的项目。 |
Components: NavigatorIOS相关
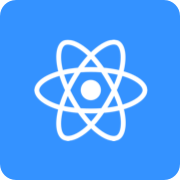
React Native 是一个 JavaScript 的框架,用来撰写实时的、可原生呈现 iOS 和 Android 的应用。
主页 | https://facebook.github.io/react-native/ |
源码 | https://github.com/facebook/react-native |
发布版本 | 0.49 |