React native参考手册
Components: Modal
Modal
模态组件是在封闭视图上呈现内容的简单方法。
注意:如果您需要更多控制如何在应用程序的其他部分展示模块,请考虑使用顶级导航器。
import React, { Component } from 'react';
import { Modal, Text, TouchableHighlight, View } from 'react-native';
class ModalExample extends Component {
state = {
modalVisible: false,
}
setModalVisible(visible) {
this.setState({modalVisible: visible});
}
render() {
return (
<View style={{marginTop: 22}}>
<Modal
animationType="slide"
transparent={false}
visible={this.state.modalVisible}
onRequestClose={() => {alert("Modal has been closed.")}}
>
<View style={{marginTop: 22}}>
<View>
<Text>Hello World!</Text>
<TouchableHighlight onPress={() => {
this.setModalVisible(!this.state.modalVisible)
}}>
<Text>Hide Modal</Text>
</TouchableHighlight>
</View>
</View>
</Modal>
<TouchableHighlight onPress={() => {
this.setModalVisible(true)
}}>
<Text>Show Modal</Text>
</TouchableHighlight>
</View>
);
}
}
道具
animated?: bool
弃用
改用animationType
prop。
animationType?: enum('none', 'slide', 'fade')
该animationType
道具如何控制模态动画。
-
slide
从底部滑入
-
fade
淡入视野
-
none
没有动画出现
默认设置为none
。
onRequestClose?: (Platform.isTVOS || Platform.OS === 'android') ? PropTypes.func.isRequired : PropTypes.func
onRequestClose
当用户点击Android上的硬件返回按钮或Apple TV上的菜单按钮时,会调用该回调。
onShow?: function
该onShow
道具允许传递一个函数,一旦模态被显示,该函数将被调用。
transparent?: bool
该transparent
道具决定了你是否模式将填补整个视图。将其设置为true
将在透明背景上渲染模式。
visible?: bool
该visible
道具决定了你的模式是否可见。
androidhardwareAccelerated?: bool
该hardwareAccelerated
道具控制是否强制底层窗口的硬件加速。
iosonOrientationChange?: function
在onOrientationChange
当在显示模态方向变化回调被调用。提供的方向只是“肖像”或“风景”。不管当前的方向如何,此回调也在初始渲染时调用。
iospresentationStyle?: enum('fullScreen', 'pageSheet', 'formSheet', 'overFullScreen')
该presentationStyle
道具控制着模式的显示方式(通常用于iPad或大尺寸iPhone等较大的设备上)。有关详细信息,请参阅https://developer.apple.com/reference/uikit/uimodalpresentationstyle。
-
fullScreen
完全覆盖屏幕
-
pageSheet
涵盖居中的纵向宽度视图(仅适用于较大的设备)
-
formSheet
涵盖居中的窄视图(仅适用于较大的设备)
-
overFullScreen
完全覆盖屏幕,但允许透明度
默认设置为overFullScreen
或fullScreen
取决于transparent
属性。
iossupportedOrientations?: [enum('portrait', 'portrait-upside-down', 'landscape', 'landscape-left', 'landscape-right')]
所述supportedOrientations
支柱允许模式被旋转到任何指定的取向。在iOS上,模态仍受限于应用的Info.plist的UISupportedInterfaceOrientations字段中指定的内容。当使用presentationStyle
的pageSheet
还是formSheet
,这个属性会被iOS的被忽略。
Components: Modal相关
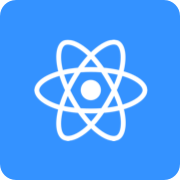
React Native 是一个 JavaScript 的框架,用来撰写实时的、可原生呈现 iOS 和 Android 的应用。
主页 | https://facebook.github.io/react-native/ |
源码 | https://github.com/facebook/react-native |
发布版本 | 0.49 |