React native参考手册
组件:图像 | Components: Image
Image
React组件,用于显示不同类型的图像,包括网络图像,静态资源,临时本地图像以及本地磁盘上的图像,例如相机胶卷。
这个例子展示了从本地存储器获取和显示图像,以及从网络获取图像,甚至从'data:'
uri模式提供的数据。
请注意,对于网络和数据图像,您需要手动指定图像的尺寸!
import React, { Component } from 'react';
import { AppRegistry, View, Image } from 'react-native';
export default class DisplayAnImage extends Component {
render() {
return (
<View>
<Image
source={require('./img/favicon.png')}
/>
<Image
style={{width: 50, height: 50}}
source={{uri: 'https://facebook.github.io/react/img/logo_og.png'}}
/>
<Image
style={{width: 66, height: 58}}
source={{uri: 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAADMAAAAzCAYAAAA6oTAqAAAAEXRFWHRTb2Z0d2FyZQBwbmdjcnVzaEB1SfMAAABQSURBVGje7dSxCQBACARB+2/ab8BEeQNhFi6WSYzYLYudDQYGBgYGBgYGBgYGBgYGBgZmcvDqYGBgmhivGQYGBgYGBgYGBgYGBgYGBgbmQw+P/eMrC5UTVAAAAABJRU5ErkJggg=='}}
/>
</View>
);
}
}
// skip this line if using Create React Native App
AppRegistry.registerComponent('DisplayAnImage', () => DisplayAnImage);
您还可以添加style
到图片:
import React, { Component } from 'react';
import { AppRegistry, View, Image, StyleSheet } from 'react-native';
const styles = StyleSheet.create({
stretch: {
width: 50,
height: 200
}
});
export default class DisplayAnImageWithStyle extends Component {
render() {
return (
<View>
<Image
style={styles.stretch}
source={require('./img/favicon.png')}
/>
</View>
);
}
}
// skip these lines if using Create React Native App
AppRegistry.registerComponent(
'DisplayAnImageWithStyle',
() => DisplayAnImageWithStyle
);
Android上的GIF和WebP支持
在构建自己的本机代码时,Android上默认不支持GIF和WebP。
android/app/build.gradle
根据应用程序的需要,您需要添加一些可选模块。
dependencies {
// If your app supports Android versions before Ice Cream Sandwich (API level 14)
compile 'com.facebook.fresco:animated-base-support:1.3.0'
// For animated GIF support
compile 'com.facebook.fresco:animated-gif:1.3.0'
// For WebP support, including animated WebP
compile 'com.facebook.fresco:animated-webp:1.3.0'
compile 'com.facebook.fresco:webpsupport:1.3.0'
// For WebP support, without animations
compile 'com.facebook.fresco:webpsupport:1.3.0'
}
另外,如果您在ProGuard中使用GIF,则需要在以下内容中添加此规则proguard-rules.pro
:
-keep class com.facebook.imagepipeline.animated.factory.AnimatedFactoryImpl {
public AnimatedFactoryImpl(com.facebook.imagepipeline.bitmaps.PlatformBitmapFactory, com.facebook.imagepipeline.core.ExecutorSupplier);
}
道具
blurRadius?: number
blurRadius:添加到图像的模糊滤镜的模糊半径
onError?: function
在加载错误时调用{nativeEvent: {error}}
。
onLayout?: function
在装载和布局更改时调用{nativeEvent: {layout: {x, y, width, height}}}
。
onLoad?: function
加载成功完成时调用。
onLoadEnd?: function
当加载成功或失败时调用。
onLoadStart?: function
在加载启动时调用。
e.g., onLoadStart={(e) => this.setState({loading: true})}
resizeMode?: enum('cover', 'contain', 'stretch', 'repeat', 'center')
确定在帧与原始图像尺寸不匹配时如何调整图像尺寸。
-
cover
:均匀缩放图像(保持图像的宽高比),使图像的两个尺寸(宽度和高度)等于或大于视图的相应尺寸(减去填充)。
-
contain
:均匀缩放图像(保持图像的宽高比),使图像的两个尺寸(宽度和高度)等于或小于视图的相应尺寸(减去填充)。
-
stretch
:独立缩放宽度和高度,这可能会改变src的宽高比。
-
repeat
:重复图像以覆盖视图的框架。图像将保持其尺寸和高宽比。(仅适用于iOS)
source?: ImageSourcePropType
图像源(远程URL或本地文件资源)。
这个道具还可以包含多个远程URL,它们的宽度和高度以及规模/其他URI参数一起指定。然后本机端将uri
根据图像容器的测量大小选择最佳显示方式。cache
可以添加一个属性来控制网络请求如何与本地缓存交互。
目前支持的格式是png
,jpg
,jpeg
,bmp
,gif
,webp
(仅限Android), psd
(仅限iOS)。
style?: style
Layout Props...
Shadow Props...
Transforms...
backfaceVisibility enum('visible', 'hidden')
backgroundColor颜色
borderBottomLeftRadius号码
borderBottomRightRadius号码
borderColor颜色
borderRadius号码
borderTopLeftRadius号码
borderTopRightRadius号码
边框宽度数字
不透明度数字
溢出枚举('可见','隐藏')
resizeMode Object.keys(ImageResizeMode)
tintColor color将所有不透明像素的颜色更改为tintColor。
androidoverlayColor string当图像具有圆角时,指定overlayColor将导致角落中的剩余空间填充纯色。这对于Android实现圆角不支持的情况非常有用: - 某些调整大小模式,例如“包含” - 动画GIF
使用此道具的典型方法是将图像显示在纯色背景上,并将其设置overlayColor
为与背景相同的颜色。
有关这是如何工作的细节,请参阅http://frescolib.org/docs/rounded-corners-and-circles.html
ImageResizeMode
是Enum
针对不同的图像大小调整模式,通过组件上的resizeMode
样式属性进行设置Image
。该值是contain
,cover
,stretch
,center
,repeat
。
testID?: string
用于UI自动化测试脚本的此元素的唯一标识符。
androidresizeMethod?: enum('auto', 'resize', 'scale')
当图像的尺寸与图像视图的尺寸不同时应该用于调整图像大小的机制。默认为auto
。
-
auto
:使用启发式来选择resize
和scale
。
-
resize
:在解码之前改变存储器中编码图像的软件操作。这应该被用来代替scale
当图像比视图大得多时。
-
scale
:图像被缩小或放大。相比较而言resize
,scale
速度更快(通常是硬件加速),并生成更高质量的图像。如果图像小于视图,则应该使用此选项。如果图像比视图稍大,也应该使用它。
有关详细信息resize
,请scale
访问http://frescolib.org/docs/resizing-rotating.html。
iosaccessibilityLabel?: node
屏幕阅读器在用户与图像交互时读取的文本。
iosaccessible?: bool
如果为true,则表示该图像是可访问性元素。
ioscapInsets?: {top: number, left: number, bottom: number, right: number}
当图像被调整大小时,指定大小的角capInsets
将保持固定大小,但图像的中心内容和边界将被拉伸。这对创建可调整大小的舍入按钮,阴影和其他可调整大小的资产很有用。有关Apple官方文档的更多信息。
iosdefaultSource?: {uri: string, width: number, height: number, scale: number}, number
加载图像源时显示的静态图像。
-
uri
- 表示图像的资源标识符的字符串,应该是本地文件路径或静态图像资源的名称(应包装在require('./path/to/image.png')
函数中)。
-
width
,height
- 如果在构建时已知,则可以指定 - 在这种情况下,这些将用于设置默认<Image/>
组件维度。
-
scale
- 用于指示图像的比例因子。如果未指定,则默认为1.0,表示一个图像像素等于一个显示点/ DIP。
-
number
- 由类似返回的不透明类型require('./image.jpg')
。
iosonPartialLoad?: function
当图像的部分加载完成时调用。虽然这是针对渐进式JPEG加载的,但是构成“部分加载”的定义与加载器相关。
iosonProgress?: function
用下载进度调用{nativeEvent: {loaded, total}}
。
方法
static getSize(uri: string, success: function, failure?: function):
在显示图像之前检索图像的宽度和高度(以像素为单位)。如果无法找到图像或无法下载,此方法可能会失败。
为了检索图像尺寸,图像可能首先需要加载或下载,之后将被缓存。这意味着原则上,您可以使用此方法预先加载图像,但未针对此目的进行优化,未来可能会以未完全加载/下载图像数据的方式实施。预加载图像的正确支持方式将作为单独的API提供。
不适用于静态图像资源。
参数:
名称和类型 |
描述 |
---|---|
uriString中 |
图像的位置。 |
successfunction |
如果成功找到图像并检索宽度和高度,将调用该函数。 |
failurefunction |
如果发生错误,将会调用的函数,如未能检索图像。 |
static prefetch(url: string):
通过将远程映像下载到磁盘高速缓存中来预取一个远程映像供以后使用
参数:
名称和类型 |
描述 |
---|---|
urlstring |
图像的远程位置。 |
组件:图像 | Components: Image相关
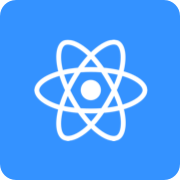
React Native 是一个 JavaScript 的框架,用来撰写实时的、可原生呈现 iOS 和 Android 的应用。
主页 | https://facebook.github.io/react-native/ |
源码 | https://github.com/facebook/react-native |
发布版本 | 0.49 |